Matplotlib Mastery: A Comprehensive Python Guide
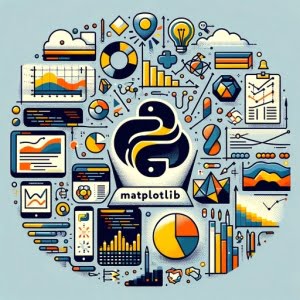
Are you grappling with the challenge of creating visually appealing graphs in Python? Imagine if you could wield Matplotlib like an artist’s brush, painting a vivid picture with your data. This guide is designed to help you do just that.
Matplotlib, a powerful plotting library in Python, is your canvas for data visualization. Whether you’re a beginner just starting out or an advanced user looking to refine your skills, this guide will walk you through the process of mastering Matplotlib.
So, if you’re ready to transform your data into compelling visuals, let’s dive into the world of Matplotlib.
TL;DR: How Do I Use Matplotlib in Python?
To use Matplotlib in Python, you first import the library, then create a plot, and finally show the plot. Here’s a simple example:
import matplotlib.pyplot as plt
plt.plot([1, 2, 3, 4])
plt.show()
# Output:
# This will create a simple line graph with values 1, 2, 3, and 4 plotted on the y-axis.
In this example, we first import the Matplotlib library with import matplotlib.pyplot as plt
. Next, we create a plot using plt.plot([1, 2, 3, 4])
which plots the values 1, 2, 3, and 4 on the y-axis. Finally, we display the plot with plt.show()
. This simple process is the foundation of using Matplotlib in Python.
Intrigued? Keep reading for a more detailed exploration and advanced usage scenarios of Matplotlib.
Table of Contents
- Matplotlib Basics: Line Graphs, Bar Charts, and Scatter Plots
- Advanced Matplotlib: Histograms, 3D Plots, and Heatmaps
- Exploring Alternatives to Matplotlib: Seaborn, Plotly, and Bokeh
- Matplotlib Troubleshooting and Considerations
- Matplotlib: A Deep Dive into Python’s Powerful Plotting Library
- Data Visualization: A Vital Tool in Data Analysis and Machine Learning
- Wrapping Up: Matplotlib Mastery for Python
Matplotlib Basics: Line Graphs, Bar Charts, and Scatter Plots
Matplotlib offers a broad spectrum of basic plots to visualize your data. Let’s start with the most common ones: line graphs, bar charts, and scatter plots.
Creating Line Graphs with Matplotlib
Line graphs are a staple in data visualization, perfect for showing trends over time. Here’s how you create one with Matplotlib:
import matplotlib.pyplot as plt
x = [1, 2, 3, 4, 5]
y = [2, 3, 5, 7, 11]
plt.plot(x, y)
plt.show()
# Output:
# This will create a line graph with 'x' values on the x-axis and 'y' values on the y-axis.
In the code above, we first define our x and y data points. Then, we call plt.plot(x, y)
to create the line graph. The plt.show()
function then displays our graph.
Crafting Bar Charts with Matplotlib
Bar charts are great for comparing quantities across different categories. Here’s a simple bar chart example:
import matplotlib.pyplot as plt
categories = ['A', 'B', 'C', 'D', 'E']
values = [7, 12, 15, 10, 8]
plt.bar(categories, values)
plt.show()
# Output:
# This will create a bar chart with categories on the x-axis and their corresponding values on the y-axis.
We create a bar chart by calling plt.bar(categories, values)
. The categories
list represents the x-axis and values
list represents the y-axis.
Scatter Plots: Visualizing Relationships in Matplotlib
Scatter plots are ideal for visualizing relationships between two variables. Here’s how to create a scatter plot:
import matplotlib.pyplot as plt
x = [1, 2, 3, 4, 5]
y = [2, 3, 5, 7, 11]
plt.scatter(x, y)
plt.show()
# Output:
# This will create a scatter plot with 'x' values on the x-axis and 'y' values on the y-axis.
To create a scatter plot, we use plt.scatter(x, y)
. This plots individual data points for each pair of x and y values.
These are just the basics, but they should give you a solid foundation to start visualizing your data with Matplotlib. As you become more comfortable, you can start exploring more complex visualizations and customizations.
Advanced Matplotlib: Histograms, 3D Plots, and Heatmaps
Once you’re comfortable with the basics of Matplotlib, it’s time to delve into more complex visualizations. In this section, we’ll explore histograms, 3D plots, and heatmaps.
Crafting Histograms with Matplotlib
Histograms allow us to visualize the distribution of a data set. Here’s how you create a histogram with Matplotlib:
import matplotlib.pyplot as plt
data = [2, 4, 4, 4, 5, 5, 7, 9]
plt.hist(data, bins=4)
plt.show()
# Output:
# This will create a histogram with 4 bins, distributing the 'data' values across these bins.
In this example, plt.hist(data, bins=4)
creates a histogram using the data
list. The bins
parameter divides the data into four bins, helping us visualize the distribution.
Creating 3D Plots in Matplotlib
3D plots can add an extra dimension to our data visualization. Here’s a simple 3D scatter plot example:
from mpl_toolkits.mplot3d import Axes3D
import matplotlib.pyplot as plt
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
x = [1, 2, 3, 4, 5]
y = [5, 7, 9, 11, 13]
z = [2, 3, 5, 7, 11]
ax.scatter(x, y, z)
plt.show()
# Output:
# This will create a 3D scatter plot with 'x', 'y', and 'z' values.
In the above code, we first create a figure and an Axes3D object. We then plot x, y, and z values using ax.scatter(x, y, z)
.
Heatmaps: Visualizing Density in Matplotlib
Heatmaps are a powerful tool for visualizing data density. Here’s how to create a heatmap:
import matplotlib.pyplot as plt
import numpy as np
# Generate random data
ndata = np.random.rand(10,10)
plt.imshow(ndata, cmap='hot', interpolation='nearest')
plt.show()
# Output:
# This will create a heatmap using the randomly generated data in 'ndata'.
In this example, plt.imshow(ndata, cmap='hot', interpolation='nearest')
creates a heatmap using the ndata
array. The cmap
parameter sets the color map to ‘hot’, and interpolation='nearest'
sets the interpolation method.
These advanced plotting techniques can provide deeper insights into your data. As you continue to explore Matplotlib, you’ll uncover even more ways to visualize and understand your data.
Exploring Alternatives to Matplotlib: Seaborn, Plotly, and Bokeh
While Matplotlib is a powerful tool for data visualization in Python, it’s not the only game in town. Other libraries such as Seaborn, Plotly, and Bokeh offer additional features and unique advantages. Let’s explore these alternatives and see how they compare to Matplotlib.
Seaborn: Statistical Data Visualization
Seaborn is a Python data visualization library based on Matplotlib. It provides a high-level interface for drawing attractive and informative statistical graphics. Here’s a simple Seaborn example:
import seaborn as sns
df = sns.load_dataset('iris')
sns.pairplot(df, hue='species')
# Output:
# This will create a pairplot of the 'iris' dataset, with different species color-coded.
In the above code, we first load the ‘iris’ dataset using sns.load_dataset('iris')
. Then, we create a pairplot using sns.pairplot(df, hue='species')
. The pairplot shows relationships between pairs of features in the iris dataset.
Plotly: Interactive Graphing
Plotly is a library that allows you to create interactive plots that you can use in dashboards or websites. Here’s a simple Plotly example:
import plotly.express as px
df = px.data.iris()
fig = px.scatter(df, x='sepal_width', y='sepal_length', color='species')
fig.show()
# Output:
# This will create an interactive scatter plot of the 'iris' dataset, with different species color-coded.
In the above code, we first load the ‘iris’ dataset using px.data.iris()
. Then, we create an interactive scatter plot using px.scatter(df, x='sepal_width', y='sepal_length', color='species')
.
Bokeh: Interactive Visualization Library
Bokeh is a Python library for creating interactive visualizations for modern web browsers. It’s designed to help you create interactive plots, dashboards, and data applications. Here’s a simple Bokeh example:
from bokeh.plotting import figure, show
p = figure(plot_width=400, plot_height=400)
p.circle([1, 2, 3, 4, 5], [6, 7, 2, 4, 5], size=20, color='navy', alpha=0.5)
show(p)
# Output:
# This will create an interactive circle plot with given x and y values.
In the above code, we first create a figure using figure(plot_width=400, plot_height=400)
. Then, we add a circle glyph to the figure using p.circle([1, 2, 3, 4, 5], [6, 7, 2, 4, 5], size=20, color='navy', alpha=0.5)
.
Library | Advantages | Disadvantages |
---|---|---|
Matplotlib | Versatile, control over every element of a plot, widely used | Can be complex, not interactive |
Seaborn | Built on Matplotlib, easier to use, good for statistical plots | Less control than Matplotlib, less versatile |
Plotly | Interactive, easy to use, good for dashboards and web apps | Less control than Matplotlib, requires internet connection |
Bokeh | Interactive, good for large datasets, can build complex dashboards | Less control than Matplotlib, more complex than Plotly |
While Matplotlib is a powerful tool, these alternative libraries offer unique features and advantages. Depending on your specific needs, you might find one of these alternatives to be a better fit for your project.
Matplotlib Troubleshooting and Considerations
Data visualization with Matplotlib is not always a smooth journey. You might encounter some bumps along the road. Let’s explore some common issues and their solutions.
Dealing with Incorrect Data Types
One common issue is dealing with incorrect data types. Matplotlib expects numerical data for plotting, but what if your data includes non-numerical types?
import matplotlib.pyplot as plt
x = [1, 2, 'three', 4, 5]
y = [2, 3, 5, 7, 11]
try:
plt.plot(x, y)
plt.show()
except TypeError:
print('Error: Non-numerical data in the list')
# Output:
# Error: Non-numerical data in the list
In this example, the x list contains a string ‘three’, which causes a TypeError. The solution is to clean your data and convert non-numerical types to numerical ones before plotting.
Handling Missing Values
Missing values in your data can also cause problems. Let’s see how to handle them:
import matplotlib.pyplot as plt
import numpy as np
x = [1, 2, np.nan, 4, 5]
y = [2, 3, 5, 7, 11]
plt.plot(x, y)
plt.show()
# Output:
# This will create a line graph, but the line will be broken where the x value is missing (np.nan).
In this example, the x list contains a missing value (np.nan
). Matplotlib handles this by breaking the line at the missing value. If this is not the desired behavior, you can fill missing values with a suitable value or remove them before plotting.
Other Considerations
Other considerations when using Matplotlib include understanding your data, choosing the right plot for your data, and customizing your plots to convey information effectively. Remember, the goal of data visualization is not just to create pretty pictures, but to understand and communicate data.
By understanding these common issues and their solutions, you can avoid pitfalls and create effective visualizations with Matplotlib.
Matplotlib: A Deep Dive into Python’s Powerful Plotting Library
Before we delve further into the practical usage of Matplotlib, let’s take a moment to understand the library at a deeper level. This will not only enhance your understanding of the tool but also enable you to leverage its full potential.
Understanding Matplotlib’s Architecture
Matplotlib’s architecture is made up of three main layers: the Scripting Layer, the Artist Layer, and the Backend Layer.
- Scripting Layer: This is the layer that we interact with most of the time. It provides a simple way to generate plots quickly using pyplot, a module in Matplotlib.
Artist Layer: This is where much of the heavy lifting happens. Everything you see on a Matplotlib plot is an Artist object, whether it’s the text, lines, tick labels, or other elements.
Backend Layer: This is the layer that does the drawing onto your screen or into a file. There are different backends that Matplotlib can use, each with different capabilities and uses.
Matplotlib’s Relationship with Python Data Structures
Matplotlib is designed to work well with many of the core data structures in Python. For example, you can easily create plots using lists, as we’ve seen in previous examples. Matplotlib also works seamlessly with NumPy arrays, which are commonly used for storing data in Python. Furthermore, if you’re working with tabular data, Matplotlib integrates well with pandas, a powerful data manipulation library in Python.
Different Types of Plots and Their Use Cases
Matplotlib supports a wide array of plots, each with its own use case. Here are a few examples:
- Line Graph: Ideal for showing trends over time. For example, you could use a line graph to display a company’s revenue growth over the years.
Bar Chart: Great for comparing quantities across different categories. For example, you could use a bar chart to compare the population of different countries.
Histogram: Perfect for visualizing the distribution of a data set. For example, you could use a histogram to visualize the distribution of student grades in a class.
Scatter Plot: Best suited for visualizing relationships between two variables. For example, you could use a scatter plot to display the correlation between advertising spend and sales.
Heatmap: Useful for visualizing data density. For example, you could use a heatmap to display the density of traffic accidents in different parts of a city.
Understanding the fundamentals of Matplotlib will help you to use the library more effectively and create more meaningful visualizations. Remember, the best data visualization is not necessarily the most complex, but the one that communicates the data most effectively.
Data Visualization: A Vital Tool in Data Analysis and Machine Learning
Data visualization, with tools like Matplotlib, plays a crucial role in various fields of data science, including data analysis and machine learning. It’s not just about creating visually appealing plots; it’s about uncovering insights, identifying patterns, and communicating complex data in a simple, digestible manner.
Unveiling Insights with Matplotlib
In data analysis, visualizing your data can help you understand it better. For instance, a bar chart can reveal the most common categories in your data, or a scatter plot might show the correlation between two variables. With Matplotlib, you can create these plots with just a few lines of code.
import matplotlib.pyplot as plt
# Sample data
x = [1, 2, 3, 4, 5]
y = [2, 4, 1, 3, 5]
# Create a scatter plot
plt.scatter(x, y)
plt.show()
# Output:
# This will create a scatter plot, showing the correlation between 'x' and 'y'.
In this example, we’re creating a scatter plot to visualize the correlation between x
and y
. The resulting plot can help us understand the relationship between these two variables.
Matplotlib in Machine Learning
In machine learning, visualizations can help in model selection and evaluation. For example, a line graph can show the performance of a model over time or across different hyperparameters, aiding in model selection.
Exploring Related Concepts
As you continue your data science journey, you might want to explore related concepts like data preprocessing and statistical analysis. Data preprocessing involves cleaning and transforming your data to improve your models’ performance. On the other hand, statistical analysis can help you understand your data and make informed predictions.
Further Resources for Matplotlib Mastery
For a deeper understanding of Matplotlib and data visualization, here are a few resources you might find useful:
- Creating Histograms with Matplotlib – A quick tutorial on histogram plotting and visualization in Python.
Matplotlib’s official documentation – A comprehensive resource with detailed explanations and examples.
Python Data Science Handbook by Jake VanderPlas – An excellent book with a section dedicated to data visualization with Matplotlib.
Data Visualization with Python and Matplotlib on Coursera – An online course that covers data visualization in depth.
Remember, mastering Matplotlib and data visualization is a journey. Take your time, practice regularly, and don’t be afraid to experiment and create your own unique visualizations.
Wrapping Up: Matplotlib Mastery for Python
In this guide, we’ve journeyed through the world of Matplotlib, Python’s powerful data visualization library.
We’ve explored the basics, such as creating line graphs, bar charts, and scatter plots with plt.plot()
, plt.bar()
, and plt.scatter()
. We delved into more advanced visualizations like histograms, 3D plots, and heatmaps, and tackled common issues like incorrect data types and missing values.
Along the way, we discovered alternative libraries for data visualization, including Seaborn, Plotly, and Bokeh, each with their unique advantages. For instance, Seaborn excels in statistical graphics, Plotly in interactive plots, and Bokeh in handling large datasets and building complex dashboards.
Library | Strengths |
---|---|
Matplotlib | Versatile, granular control over plots |
Seaborn | High-level interface, excellent for statistical plots |
Plotly | Interactive, great for dashboards and web apps |
Bokeh | Handles large datasets well, ideal for complex dashboards |
Remember, the choice of tool often depends on the task at hand. While Matplotlib is a versatile and powerful tool, these alternatives can sometimes offer more specialized features.
Data visualization is a crucial skill in data science, aiding in data analysis and machine learning. As you continue your journey, don’t forget to explore related concepts like data preprocessing and statistical analysis.
Most importantly, keep practicing and experimenting with your data visualizations. Happy plotting!