Python Zipfile Module | Guide To Managing Zip Files
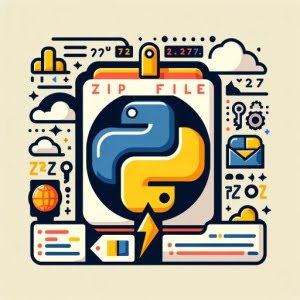
Are you struggling to manage ZIP files in Python? You’re not alone. Many developers find themselves in a maze when it comes to handling ZIP files in Python, but we’re here to help.
Think of Python’s zipfile module as a skilled archivist – it allows you to create, extract, and manipulate ZIP files with ease. It’s an incredibly versatile tool that can make your life significantly easier when dealing with ZIP files.
In this guide, we’ll walk you through the process of working with the zipfile module in Python, from the basics to more advanced techniques. We’ll cover everything from creating and extracting ZIP files, to handling common issues and exploring alternative approaches.
Let’s dive in and start mastering Python zipfile!
TL;DR: How Do I Create and Extract ZIP Files in Python?
Python’s zipfile module allows you to easily create and extract ZIP files with the
write()
andextractall()
functions. Here’s a simple example:
import zipfile
# Create a ZIP file
with zipfile.ZipFile('example.zip', 'w') as zipf:
zipf.write('example.txt')
# Extract a ZIP file
with zipfile.ZipFile('example.zip', 'r') as zipf:
zipf.extractall()
# Output:
# A file named 'example.zip' is created with 'example.txt' inside it.
# The file 'example.txt' is then extracted from 'example.zip'.
In this example, we first import the zipfile module. We then create a ZIP file named ‘example.zip’ and write a file ‘example.txt’ into it. After that, we open the same ZIP file in read mode and extract all its contents.
This is a basic way to use the zipfile module in Python, but there’s much more to learn about creating, extracting, and manipulating ZIP files. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
Getting Started with Python Zipfile Module
As a Python developer, you’ll often find yourself needing to work with ZIP files, whether it’s for archiving, data compression, or distributing multiple files as a single package. Python’s zipfile module makes this process straightforward and efficient.
Creating a ZIP File
To create a ZIP file, you need to use the ZipFile
function from the zipfile module. Here’s a simple example:
import zipfile
# Create a new ZIP file
with zipfile.ZipFile('my_archive.zip', 'w') as my_zip:
my_zip.write('my_file.txt')
# Output:
# A new ZIP file named 'my_archive.zip' is created with 'my_file.txt' inside it.
In this code, we first import the zipfile module. We then create a new ZIP file named ‘my_archive.zip’ in write (‘w’) mode. Inside this ZIP file, we store a file named ‘my_file.txt’.
Extracting a ZIP File
To extract a ZIP file, you can use the extractall
method. Here’s how you do it:
import zipfile
# Extract all files from a ZIP file
with zipfile.ZipFile('my_archive.zip', 'r') as my_zip:
my_zip.extractall()
# Output:
# All files inside 'my_archive.zip' are extracted to the current directory.
In this example, we open ‘my_archive.zip’ in read (‘r’) mode and use the extractall
method to extract all files inside it to the current directory.
These are the basics of creating and extracting ZIP files using Python’s zipfile module. However, there’s much more you can do with this versatile module, as we’ll explore in the following sections.
Advanced Python Zipfile Techniques
While the basic operations of creating and extracting ZIP files with Python’s zipfile module are straightforward, there are more advanced techniques that can be incredibly useful in certain situations. Let’s delve into some of these techniques.
Adding Multiple Files to a ZIP File
If you want to add multiple files to a ZIP file, you can do so by calling the write
method multiple times. Here’s an example:
import zipfile
# Create a new ZIP file and add multiple files
with zipfile.ZipFile('my_archive.zip', 'w') as my_zip:
my_zip.write('my_file1.txt')
my_zip.write('my_file2.txt')
my_zip.write('my_file3.txt')
# Output:
# A new ZIP file named 'my_archive.zip' is created with 'my_file1.txt', 'my_file2.txt', and 'my_file3.txt' inside it.
In this code, we create a new ZIP file named ‘my_archive.zip’ and add three files (‘my_file1.txt’, ‘my_file2.txt’, and ‘my_file3.txt’) to it.
Creating Password-Protected ZIP Files
You can also create password-protected ZIP files using the zipfile module, although this requires using a third-party module called pyminizip. Here’s an example:
import pyminizip
# Create a password-protected ZIP file
pyminizip.compress('my_file.txt', None, 'my_archive.zip', 'mypassword', 0)
# Output:
# A new password-protected ZIP file named 'my_archive.zip' is created with 'my_file.txt' inside it. The password is 'mypassword'.
In this example, we use the compress
method from the pyminizip module to create a password-protected ZIP file named ‘my_archive.zip’. The file ‘my_file.txt’ is compressed into this ZIP file, and the password is set to ‘mypassword’.
These are just a few examples of the more advanced techniques you can use with Python’s zipfile module. As with any tool, the more you practice and experiment with it, the more you’ll discover its capabilities.
Exploring Alternatives to Python’s Zipfile Module
While the zipfile module is a powerful tool for managing ZIP files in Python, it’s not the only option. There are other modules and techniques that you can use to work with ZIP files. Let’s take a look at some of these alternatives.
Using the Tarfile Module
Python’s tarfile module is another built-in module that you can use to read and write tar archives, a popular file format in Unix-based systems. Here’s a quick example of how you can use it to create a tar file:
import tarfile
# Create a new tar file
with tarfile.open('my_archive.tar', 'w') as my_tar:
my_tar.add('my_file.txt')
# Output:
# A new tar file named 'my_archive.tar' is created with 'my_file.txt' inside it.
In this example, we first import the tarfile module. We then create a new tar file named ‘my_archive.tar’ and add a file named ‘my_file.txt’ to it.
Using Third-Party Libraries
There are also several third-party libraries that you can use to work with ZIP files in Python. For example, the pyminizip library allows you to create password-protected ZIP files, as we saw in the previous section.
import pyminizip
# Create a password-protected ZIP file
pyminizip.compress('my_file.txt', None, 'my_archive.zip', 'mypassword', 0)
# Output:
# A new password-protected ZIP file named 'my_archive.zip' is created with 'my_file.txt' inside it. The password is 'mypassword'.
In this code, we use the compress
method from the pyminizip module to create a password-protected ZIP file named ‘my_archive.zip’. The file ‘my_file.txt’ is compressed into this ZIP file, and the password is set to ‘mypassword’.
While the zipfile module is often sufficient for most needs, these alternative approaches can provide additional functionality or a different way of working with ZIP files in Python. It’s worth exploring these options to find the one that best suits your specific needs.
Troubleshooting Common Zipfile Issues
Working with ZIP files in Python using the zipfile module is generally straightforward, but you may encounter some common issues. Let’s delve into these potential problems and discuss how to resolve them.
Handling Large Files
When dealing with large files, you might run into memory issues. To avoid this, you can read and write data in chunks. Here’s an example of how you can write large files in chunks:
import zipfile
def write_large_file_to_zip(zip_name, file_name, chunk_size=1024):
with zipfile.ZipFile(zip_name, 'w', zipfile.ZIP_DEFLATED) as zf:
with open(file_name, 'rb') as f:
while True:
chunk = f.read(chunk_size)
if not chunk:
break
zf.writestr(file_name, chunk)
# Usage:
write_large_file_to_zip('my_archive.zip', 'large_file.txt')
# Output:
# A new ZIP file named 'my_archive.zip' is created with 'large_file.txt' inside it, written in chunks.
In this example, we define a function write_large_file_to_zip
that writes a large file to a ZIP file in chunks. This function takes three arguments: the name of the ZIP file, the name of the file to be written, and the chunk size (defaulting to 1024 bytes).
Dealing with Corrupted ZIP Files
Sometimes, you might have to deal with corrupted ZIP files. The zipfile module provides the is_zipfile
function to check if a file is a ZIP file and the testzip
method to find the first corrupted file in a ZIP file. Here’s how you can use them:
import zipfile
# Check if a file is a ZIP file
if zipfile.is_zipfile('corrupted.zip'):
print('This is a ZIP file.')
else:
print('This is not a ZIP file.')
# Find the first corrupted file in a ZIP file
with zipfile.ZipFile('corrupted.zip', 'r') as my_zip:
corrupted_file = my_zip.testzip()
if corrupted_file is None:
print('No corrupted file found.')
else:
print(f'Corrupted file found: {corrupted_file}')
# Output:
# 'This is a ZIP file.'
# 'Corrupted file found: corrupted_file.txt'
In this example, we first check if ‘corrupted.zip’ is a ZIP file. We then open this ZIP file in read mode and use the testzip
method to find the first corrupted file. If a corrupted file is found, its name is printed; otherwise, a message indicating no corrupted file was found is printed.
These are just a few examples of the issues you might encounter when using the zipfile module in Python. However, with the right knowledge and tools, you can effectively troubleshoot these problems and work with ZIP files efficiently.
Understanding ZIP Files and Python’s Zipfile Module
Before we delve deeper into the zipfile module, it’s essential to understand what ZIP files are and why they’re so widely used.
What are ZIP Files?
ZIP files are archives that store multiple files and directories in one compressed file. This compression makes it easier to share, distribute, and manage large files and groups of files. It’s like packing a suitcase – you can fit more into a small space by compressively organizing your belongings.
import zipfile
# Create a ZIP file
with zipfile.ZipFile('example.zip', 'w') as zipf:
zipf.write('example.txt')
# Output:
# A file named 'example.zip' is created with 'example.txt' inside it.
In this code block, we create a ZIP file named ‘example.zip’ and add ‘example.txt’ to it. This ZIP file is a single compressed file that contains ‘example.txt’.
The Role of Python’s Zipfile Module
Python’s zipfile module is a powerful tool for creating, reading, writing, appending, and extracting data from ZIP files. It provides a high-level interface that makes it easy to work with ZIP files in Python.
import zipfile
# Extract a ZIP file
with zipfile.ZipFile('example.zip', 'r') as zipf:
zipf.extractall()
# Output:
# The file 'example.txt' is extracted from 'example.zip'.
In this example, we open ‘example.zip’ in read mode and extract ‘example.txt’ from it. This operation is made simple and efficient with Python’s zipfile module.
Understanding the fundamentals of ZIP files and the zipfile module is key to effectively working with ZIP files in Python. With this foundation, you can better understand and utilize the various functions and methods provided by the zipfile module.
Python Zipfile: Beyond the Basics
The zipfile module in Python is an incredibly versatile tool. While we’ve covered the basics and some advanced uses, there are many other ways you can utilize this module in larger projects. Let’s explore a couple of these possibilities.
Automating File Backups
One of the most common uses for ZIP files is to store backups. With Python’s zipfile module, you can automate this process. Here’s a simple example of how you might create a backup of several files:
import zipfile
import os
def backup_files(file_names, backup_name):
with zipfile.ZipFile(backup_name, 'w') as backup_zip:
for file_name in file_names:
if os.path.isfile(file_name):
backup_zip.write(file_name)
# Usage:
backup_files(['file1.txt', 'file2.txt', 'file3.txt'], 'backup.zip')
# Output:
# A new ZIP file named 'backup.zip' is created with 'file1.txt', 'file2.txt', and 'file3.txt' inside it.
In this example, we define a function backup_files
that takes a list of file names and a name for the backup ZIP file. It then creates a ZIP file and adds each file to it. This can be used to automate backups in larger projects.
Distributing Software
ZIP files are also commonly used to distribute software. With the zipfile module, you can create a ZIP file containing all the necessary files for your software, making it easy for users to download and install your program.
import zipfile
# Create a ZIP file for software distribution
with zipfile.ZipFile('my_software.zip', 'w') as software_zip:
software_zip.write('setup.py')
software_zip.write('requirements.txt')
software_zip.write('my_software.py')
# Output:
# A new ZIP file named 'my_software.zip' is created with 'setup.py', 'requirements.txt', and 'my_software.py' inside it.
In this code, we create a ZIP file named ‘my_software.zip’ and add the necessary files for software distribution (‘setup.py’, ‘requirements.txt’, and ‘my_software.py’) to it.
Further Resources for Mastering Python Zipfile
If you’re interested in learning more about Python’s zipfile module and how you can use it in your projects, here are a few resources that you might find helpful:
- Exploring Essential Python Modules – Learn how to import and utilize modules to extend Python’s functionality.
Efficient Data Management with Python’s heapq – Dive deep into using heapq for efficient data manipulation in Python.
Data Aggregation Made Easy with Python’s Reduce – Dive into functional programming and data reduction techniques.
Python’s Official Documentation on the Zipfile Module – An overview of the zipfile module and its functions.
Real Python’s Guide on Reading and Writing Files in Python covers reading and writing files and ZIP files in Python.
PythonForBeginners’ Tutorial on the Zipfile Module provides an introduction to working with files and ZIP files in Python.
Wrapping Up: Mastering Python’s Zipfile Module
In this comprehensive guide, we’ve journeyed through the world of managing ZIP files using Python’s zipfile module. From creating and extracting ZIP files to handling common issues and exploring alternative approaches, we’ve covered a wide range of topics to help you navigate the zipfile module with ease.
We began with the basics, demonstrating how to create and extract ZIP files using simple examples. We then ventured into more advanced territory, exploring how to add multiple files to a ZIP file and how to create password-protected ZIP files.
Along the way, we tackled common challenges you might encounter when using the zipfile module, such as handling large files and dealing with corrupted ZIP files, providing you with solutions and workarounds for each issue.
We also looked at alternative approaches to managing ZIP files in Python, comparing the zipfile module with other modules like tarfile and third-party libraries like pyminizip. Here’s a quick comparison of these methods:
Method | Versatility | Complexity | Use Case |
---|---|---|---|
Python’s zipfile module | High | Low to Moderate | General ZIP file operations |
Python’s tarfile module | Moderate | Low | Working with tar files |
Pyminizip library | Moderate | Moderate | Creating password-protected ZIP files |
Whether you’re just starting out with the zipfile module or you’re looking to level up your Python skills, we hope this guide has given you a deeper understanding of how to work with ZIP files in Python using the zipfile module.
With its balance of versatility, ease of use, and power, the zipfile module is a fantastic tool for managing ZIP files in Python. Happy coding!