Python Reduce Function: Guide (With Examples)
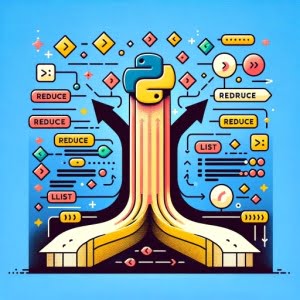
Are you finding it challenging to understand the reduce function in Python? Don’t worry, you’re not alone. Many developers find themselves puzzled when it comes to using this powerful function.
Think of Python’s reduce function as a master chef – it skillfully combines elements of a list using a function you provide, resulting in a single output.
In this guide, we’ll walk you through the process of using the reduce function in Python, from the basics to more advanced techniques. We’ll cover everything from the functools
module, basic usage of reduce, as well as alternative approaches and common issues.
Let’s get started!
TL;DR: How Do I Use the Reduce Function in Python?
The reduce function is a part of Python’s
functools
module. It’s used to apply a function to all elements of a list, effectively ‘reducing’ the list to a single output. Here’s a simple example:
from functools import reduce
numbers = [1, 2, 3, 4, 5]
result = reduce(lambda x, y: x+y, numbers)
print(result)
# Output:
# 15
In this example, we import the reduce
function from the functools
module. We then define a list of numbers. The reduce
function is used with a lambda function that adds two elements (x
and y
) together. It applies this lambda function to all elements of the list, resulting in the sum of all numbers in the list, which is 15.
This is a basic way to use the
reduce
function in Python, but there’s much more to learn about it. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Understanding the Basics of Python’s Reduce Function
- Applying Reduce Function to Complex Scenarios
- Exploring Alternatives to Python’s Reduce Function
- Navigating Common Pitfalls with Python’s Reduce Function
- Diving Deep into Python’s functools Module
- Understanding the Concept of Reduction
- Python’s reduce() Function and Functional Programming
- The Power of Python’s Reduce in Advanced Topics
- Wrapping Up: Mastering Python’s Reduce Function
Understanding the Basics of Python’s Reduce Function
The reduce()
function is a powerful tool in Python that operates on a list (or any iterable), applies a function to its elements, and ‘reduces’ them to a single output. It’s part of the functools
module, which needs to be imported before you can use reduce()
.
Let’s break down how it works with a simple example:
from functools import reduce
# Here's a list of numbers
numbers = [1, 2, 3, 4, 5]
# We'll use reduce to find the product of these numbers
product = reduce((lambda x, y: x * y), numbers)
print(product)
# Output:
# 120
In this example, we’re using reduce()
to find the product of all numbers in a list. The reduce()
function takes two arguments: a function and an iterable. The function here is a lambda function that takes two arguments (x
and y
) and multiplies them. The iterable is our list of numbers.
The reduce()
function starts from the beginning of the list. It takes the first two elements, 1 and 2, and applies the lambda function to them, resulting in 2. Then it takes this result and the next number in the list (3), applies the lambda function again, and gets 6. This process continues until all elements in the list have been handled, resulting in the product of all numbers in the list, which is 120.
Advantages of Using Reduce
The reduce()
function is a concise and efficient way to apply a function to all elements of a list and get a single output. It can simplify your code and make it more readable.
Potential Pitfalls
While reduce()
is powerful, it can also be a bit tricky to understand, especially for beginners. It’s also worth noting that reduce()
isn’t always the best tool for the job. For some tasks, using a simple loop or list comprehension can be more straightforward and easier to understand. We’ll explore these alternatives later in this guide.
Applying Reduce Function to Complex Scenarios
While the reduce()
function is commonly used with lists of numbers, it’s also versatile enough to handle more complex data structures and functions. Let’s explore some of these advanced usages.
Using Reduce with Strings
For instance, you can use reduce()
to concatenate a list of strings into a single string. Here’s how:
from functools import reduce
# Here's a list of strings
words = ['Python', 'Reduce', 'Function', 'Tutorial']
# We'll use reduce to concatenate these strings
sentence = reduce((lambda x, y: x + ' ' + y), words)
print(sentence)
# Output:
# 'Python Reduce Function Tutorial'
In this example, the lambda function takes two arguments (x
and y
) and concatenates them with a space in between. reduce()
applies this function to all elements in the list, resulting in a single string that combines all words in the list.
Using Reduce with Custom Functions
You’re not limited to using reduce()
with simple lambda functions. You can also use it with more complex, custom functions. For example, you could define a function that performs some complex operation, and then use reduce()
to apply this function to all elements in a list.
from functools import reduce
# A custom function that performs a complex operation
def complex_operation(x, y):
# (This is a placeholder for your complex operation)
return x * y
# Here's a list of numbers
numbers = [1, 2, 3, 4, 5]
# We'll use reduce to apply our custom function to these numbers
result = reduce(complex_operation, numbers)
print(result)
# Output:
# 120
In this example, complex_operation
is a custom function that takes two arguments and returns their product. We use reduce()
to apply this function to all numbers in the list, resulting in the product of all numbers, which is 120.
These are just a few examples of the advanced uses of reduce()
. As you can see, reduce()
is a versatile tool that you can use in a variety of scenarios.
Exploring Alternatives to Python’s Reduce Function
While the reduce()
function is a powerful tool in Python, it’s not always the best or the most efficient choice. Understanding alternative approaches can give you more flexibility in your coding. Let’s explore some of these alternatives and their pros and cons.
Using Loops
One of the simplest alternatives to reduce()
is using a loop. Here’s how you can achieve the same result as our previous examples using a loop:
# Here's a list of numbers
numbers = [1, 2, 3, 4, 5]
# We'll use a loop to find the product of these numbers
product = 1
for num in numbers:
product *= num
print(product)
# Output:
# 120
In this example, we initialize a variable product
to 1. Then we iterate over the list of numbers, multiplying product
by each number. This gives us the product of all numbers in the list, which is 120.
While loops are more verbose than reduce()
, they can be easier to understand, especially for beginners. However, they can also be less efficient than reduce()
, especially for large lists.
Using List Comprehensions
Another alternative to reduce()
is using list comprehensions. However, keep in mind that list comprehensions can’t always replace reduce()
, as they can’t ‘reduce’ a list to a single output. But they can be a more efficient and readable alternative for some tasks. Here’s an example:
# Here's a list of numbers
numbers = [1, 2, 3, 4, 5]
# We'll use a list comprehension to square these numbers
squares = [num ** 2 for num in numbers]
print(squares)
# Output:
# [1, 4, 9, 16, 25]
In this example, we’re using a list comprehension to square each number in a list. The list comprehension is more concise and arguably more readable than a loop. However, it can’t replace reduce()
for tasks that require reducing a list to a single output.
Making the Right Choice
Choosing between reduce()
, loops, and list comprehensions depends on your specific task, your performance requirements, and your personal coding style. While reduce()
is a powerful tool, it’s not always the best choice. Understanding these alternatives can help you write more efficient and readable code.
While Python’s reduce()
function is a powerful tool, it’s not without its quirks. Here, we’ll discuss some common issues you may encounter when using reduce()
, along with solutions and workarounds.
Handling Incorrect Function Arguments
One common issue with reduce()
is errors due to incorrect function arguments. Remember, the function you pass to reduce()
must take exactly two arguments. If it takes more or fewer arguments, you’ll get a TypeError
.
Here’s an example:
from functools import reduce
# This function takes only one argument
def square(x):
return x ** 2
numbers = [1, 2, 3, 4, 5]
try:
result = reduce(square, numbers)
except TypeError as e:
print(e)
# Output:
# square() takes 1 positional argument but 2 were given
In this example, we’re trying to use reduce()
with a function that takes only one argument. This results in a TypeError
. To fix this, we need to ensure our function takes exactly two arguments.
Dealing with Incorrect Data Types
Another common issue with reduce()
is errors due to incorrect data types. The function you pass to reduce()
must be able to handle the data type of the elements in your iterable. If it can’t, you’ll get an error.
Here’s an example:
from functools import reduce
# Here's a list of strings
words = ['Python', 'Reduce', 'Function', 'Tutorial']
# We'll try to use reduce to find the product of these strings
try:
result = reduce((lambda x, y: x * y), words)
except TypeError as e:
print(e)
# Output:
# can't multiply sequence by non-int of type 'str'
In this example, we’re trying to use reduce()
to find the product of a list of strings. This results in a TypeError
because you can’t multiply strings in Python. To fix this, we need to ensure our function can handle the data type of our iterable elements.
These are just a few examples of the issues you may encounter when using reduce()
. Understanding these potential pitfalls can help you use reduce()
more effectively and avoid common errors.
Diving Deep into Python’s functools
Module
Python’s functools
module is a treasure trove of higher-order functions. Higher-order functions are those that can accept other functions as arguments and/or return functions as results. The reduce()
function, which we’ve been discussing, is a part of this module.
The functools
module is a part of Python’s standard library, which means it comes with Python and you don’t need to install it separately. You just need to import it before you can use its functions.
import functools
Understanding the Concept of Reduction
In the context of programming, reduction refers to the process of reducing a list (or any iterable) to a single output by applying a function to its elements. This is exactly what the reduce()
function does.
Think of reduction as a process of condensation or summarization. For example, if you have a list of numbers and you want to find their sum, you’re essentially reducing the list to one number (the sum).
Python’s reduce()
Function and Functional Programming
The reduce()
function is a key concept in functional programming, a programming paradigm that treats computation as the evaluation of mathematical functions and avoids changing-state and mutable data.
In Python, reduce()
applies a function of two arguments cumulatively to the elements of an iterable in a way that it reduces the iterable to a single output. This style of computation is directly related to concepts in functional programming like folding and recursion.
Here’s a simple example of how reduce()
works:
from functools import reduce
numbers = [1, 2, 3, 4, 5]
# The lambda function adds two elements
sum = reduce((lambda x, y: x + y), numbers)
print(sum)
# Output:
# 15
In this example, reduce()
applies the lambda function to the first two elements of the list, then to the result and the next element, and so on, until it goes through all elements of the list. The final result is the sum of all numbers in the list, which is 15.
The Power of Python’s Reduce in Advanced Topics
The reduce()
function is not just a basic tool for list operations. Its relevance extends to advanced Python topics like data analysis, machine learning, and more.
Reduce in Data Analysis
In the field of data analysis, the reduce()
function can be used to perform computations on lists that represent datasets. For example, you might use reduce()
to calculate the sum or average of a dataset, or to apply a custom function to all elements of a dataset.
Reduce in Machine Learning
Machine learning often involves working with large datasets and performing complex computations on them. The reduce()
function can be a powerful tool in this context. For example, you might use reduce()
to preprocess your data, or to apply a machine learning algorithm to your dataset.
Exploring Related Concepts
If you’re interested in Python’s reduce()
function, you might also want to explore related concepts like the map()
and filter()
functions. These functions also operate on lists (or any iterables), and they can be used in combination with reduce()
to perform complex operations on lists.
Here’s a simple example that uses map()
, filter()
, and reduce()
together:
from functools import reduce
numbers = [1, 2, 3, 4, 5]
# Use map to square the numbers
squares = map(lambda x: x ** 2, numbers)
# Use filter to keep only the squares that are greater than 10
big_squares = filter(lambda x: x > 10, squares)
# Use reduce to find the sum of the big squares
sum = reduce(lambda x, y: x + y, big_squares)
print(sum)
# Output:
# 41
In this example, we’re using map()
to square each number in a list, filter()
to keep only the squares that are greater than 10, and reduce()
to find the sum of these big squares.
Further Resources for Mastering Python’s Reduce Function
To deepen your understanding of Python’s reduce()
function and related concepts, consider exploring the following resources:
- Simplifying Python Module Selection – Dive into the benefits of modular programming in Python development.
Working with ZIP Files in Python: A Quick Tutorial – Discover Python’s “zipfile” module for working with zip archives.
Python Turtle Graphics: Creative Drawing in Python – Explore Python’s “turtle” module for graphics and drawing.
Python’s functools module documentation provides information about the
reduce()
function and thefunctools
module.Real Python offers a wealth of Python tutorials and articles on topics like
reduce()
,map()
, andfilter()
.This Python Course offers a guide on Python, including a detailed section on lambda, map, filter, and reduce.
Wrapping Up: Mastering Python’s Reduce Function
In this comprehensive guide, we’ve delved into the depths of Python’s reduce()
function, a powerful tool for reducing a list (or any iterable) to a single output by applying a function to its elements.
We’ve explored how reduce()
is used, from basic examples to more complex scenarios, and we’ve also touched on related concepts like the map()
and filter()
functions.
We began with the basics, understanding how reduce()
works with a simple list of numbers. We then ventured into more advanced territory, using reduce()
with complex data structures and custom functions. We also explored alternatives to reduce()
, such as loops and list comprehensions, giving you a broader toolkit for handling similar tasks.
Along the way, we tackled common issues that you might encounter when using reduce()
, such as errors due to incorrect function arguments or data types, providing you with solutions and workarounds for each issue. We also delved into the functools
module, the home of the reduce()
function, and discussed the concept of reduction and its relevance to functional programming.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
Reduce | Powerful, versatile | Can be tricky to understand |
Loops | Easy to understand | Less efficient for large lists |
List Comprehensions | Efficient, readable | Can’t reduce a list to a single output |
Whether you’re a beginner just starting out with Python’s reduce()
function or an experienced developer looking to deepen your understanding, we hope this guide has given you a clearer picture of how to use reduce()
effectively. Happy coding!