JPA in Java: Guide to Java Persistence API
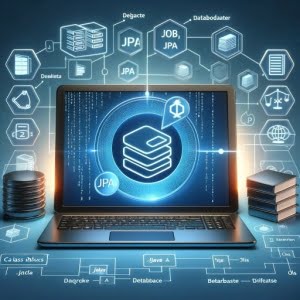
Are you wrestling with data persistence in Java? You’re not alone. Many developers find it challenging to manage data effectively in Java, but there’s a tool that can make this process a breeze.
Think of Java Persistence API (JPA) as a reliable librarian – it helps you manage your data efficiently, providing a standard interface for accessing databases in Java. From managing your data to ensuring its persistence, JPA is a game-changer.
In this guide, we’ll walk you through the process of using JPA, from the basics to more advanced techniques. We’ll cover everything from setting up JPA in your Java project, mapping Java objects to database tables, performing basic CRUD operations, to discussing more advanced features of JPA. We’ll also delve into common issues you might encounter when using JPA and provide solutions and best practices.
So, let’s get started and master JPA!
TL;DR: What is JPA in Java?
JPA, or Java Persistence API
, is a specification for object-relational mapping in Java. It provides a way to map Java objects to database tables, making it easier to manage and persist data in your Java applications.
Here’s a simple example of using JPA:
@Entity
public class Book {
@Id
private Long id;
private String title;
// getters and setters
}
In this example, we’ve defined a Book
entity with id
and title
fields. The @Entity
annotation tells JPA that this is an entity class, and the @Id
annotation is used to specify the primary key.
This is just a basic introduction to JPA in Java. There’s much more to learn about JPA, including how to perform CRUD operations, handle relationships, and more. Continue reading for a more detailed understanding and advanced usage scenarios.
Table of Contents
- Getting Started with JPA: Basic Use
- Exploring JPA: Advanced Features
- Exploring Alternatives to JPA
- Troubleshooting JPA: Common Pitfalls and Solutions
- JPA Fundamentals: Object-Relational Mapping, Persistence, and Transactions
- JPA in the Bigger Picture: Enterprise Applications and Beyond
- Wrapping Up: Java Persistence API (JPA)
Getting Started with JPA: Basic Use
The first step in using JPA in your Java project is setting it up. This involves adding the necessary dependencies to your project and configuring your persistence unit.
Here’s an example of how to add the JPA dependency to a Maven project:
<dependencies>
<dependency>
<groupId>org.hibernate</groupId>
<artifactId>hibernate-entitymanager</artifactId>
<version>5.4.27.Final</version>
</dependency>
</dependencies>
Next, you’ll need to configure your persistence unit. This is typically done in a persistence.xml
file, which is placed in the META-INF
directory of your project. Here’s a simple example:
<persistence version="2.2"
xmlns="http://xmlns.jcp.org/xml/ns/persistence" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/persistence http://xmlns.jcp.org/xml/ns/persistence/persistence_2_2.xsd">
<persistence-unit name="myPU" transaction-type="JTA">
<provider>org.hibernate.jpa.HibernatePersistenceProvider</provider>
<properties>
<property name="javax.persistence.jdbc.url" value="jdbc:mysql://localhost:3306/mydb"/>
<property name="javax.persistence.jdbc.user" value="user"/>
<property name="javax.persistence.jdbc.password" value="password"/>
<property name="javax.persistence.schema-generation.database.action" value="drop-and-create"/>
</properties>
</persistence-unit>
</persistence>
With the setup complete, you can now start using JPA to map Java objects to database tables and perform basic CRUD operations. Let’s walk through a simple example of mapping a Book
object to a database table:
@Entity
public class Book {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String title;
// getters and setters
}
In this example, we’ve defined a Book
entity with id
and title
fields. The @Entity
annotation tells JPA that this is an entity class, and the @Id
annotation is used to specify the primary key. The @GeneratedValue
annotation is used to specify that the id
field should be automatically generated.
With the Book
entity defined, we can now perform basic CRUD operations. Here’s an example of how to create a new Book
:
EntityManagerFactory emf = Persistence.createEntityManagerFactory("myPU");
EntityManager em = emf.createEntityManager();
em.getTransaction().begin();
Book book = new Book();
book.setTitle("JPA Guide");
em.persist(book);
em.getTransaction().commit();
em.close();
emf.close();
In this example, we first create an EntityManagerFactory
and an EntityManager
. We then start a transaction, create a new Book
, and persist it using the persist
method. Finally, we commit the transaction and close the EntityManager
and EntityManagerFactory
.
The persist
method is used to create a new Book
in the database. To read, update, or delete a Book
, you would use the find
, merge
, and remove
methods, respectively.
And that’s it! You’ve successfully set up JPA in your Java project and performed basic CRUD operations. Keep reading for more advanced usage scenarios.
Exploring JPA: Advanced Features
Once you’ve mastered the basics of JPA, it’s time to delve into its more advanced features. These include handling relationships between entities, inheritance, and querying.
Handling Relationships with JPA
JPA provides several annotations to handle relationships between entities. These include @OneToOne
, @OneToMany
, @ManyToOne
, and @ManyToMany
. Let’s look at an example of a one-to-many relationship between Author
and Book
entities:
@Entity
public class Author {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
@OneToMany(mappedBy = "author")
private List<Book> books;
// getters and setters
}
@Entity
public class Book {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String title;
@ManyToOne
@JoinColumn(name = "author_id")
private Author author;
// getters and setters
}
In this example, an Author
can have many Book
s, but each Book
can have only one Author
. The mappedBy
attribute in the @OneToMany
annotation indicates that the Book
entity owns the relationship.
Inheritance in JPA
JPA also supports inheritance, allowing you to define a superclass entity and subclass entities. Here’s an example:
@Entity
@Inheritance(strategy = InheritanceType.SINGLE_TABLE)
public abstract class Vehicle {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String manufacturer;
// getters and setters
}
@Entity
public class Car extends Vehicle {
private int seats;
// getters and setters
}
@Entity
public class Bike extends Vehicle {
private boolean hasPedals;
// getters and setters
}
In this example, Vehicle
is a superclass entity, and Car
and Bike
are subclass entities. The @Inheritance
annotation is used to specify the inheritance strategy.
Querying with JPA
JPA provides a powerful query language known as JPQL (Java Persistence Query Language), which allows you to perform complex queries on your entities. Here’s an example of how to use JPQL to get all Book
s by a specific Author
:
EntityManagerFactory emf = Persistence.createEntityManagerFactory("myPU");
EntityManager em = emf.createEntityManager();
String jpql = "SELECT b FROM Book b WHERE b.author.name = :authorName";
TypedQuery<Book> query = em.createQuery(jpql, Book.class);
query.setParameter("authorName", "John Doe");
List<Book> books = query.getResultList();
em.close();
emf.close();
In this example, we first create a JPQL query string. We then create a TypedQuery
using the createQuery
method and set the authorName
parameter. Finally, we execute the query using the getResultList
method.
These are just a few of the advanced features of JPA. With these tools at your disposal, you can handle complex data persistence tasks in your Java applications with ease.
Exploring Alternatives to JPA
While JPA is a powerful tool for handling data persistence in Java, it’s not the only option. Depending on your specific needs and circumstances, you might find that alternatives such as raw SQL, JDBC, or other ORM tools like Hibernate are more suitable. Let’s take a closer look at these alternatives and discuss their pros and cons.
Raw SQL
Before JPA and other ORM tools, developers had to write raw SQL queries to interact with databases. Here’s an example of how to execute a raw SQL query in Java using JDBC:
Connection conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydb", "user", "password");
Statement stmt = conn.createStatement();
ResultSet rs = stmt.executeQuery("SELECT * FROM Book");
while (rs.next()) {
System.out.println(rs.getString("title"));
}
# Output:
# 'JPA Guide'
# 'Java for Beginners'
In this example, we first establish a connection to the database, create a Statement
, and then execute a SQL query. We then iterate over the result set and print the title of each Book
.
While raw SQL gives you complete control over your queries, it can be verbose and error-prone. You also have to handle the mapping between SQL results and Java objects manually.
JDBC
JDBC (Java Database Connectivity) is a Java API for connecting and executing queries on databases. It provides more abstraction than raw SQL, but less than JPA or other ORM tools. Here’s an example of how to use JDBC to execute a SQL query:
Connection conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydb", "user", "password");
PreparedStatement pstmt = conn.prepareStatement("SELECT * FROM Book WHERE author = ?");
pstmt.setString(1, "John Doe");
ResultSet rs = pstmt.executeQuery();
while (rs.next()) {
System.out.println(rs.getString("title"));
}
# Output:
# 'JPA Guide'
In this example, we use a PreparedStatement
to execute a SQL query with a parameter. This provides some protection against SQL injection attacks.
While JDBC provides more control than JPA, it can be more verbose and requires more boilerplate code. You also have to handle the mapping between SQL results and Java objects manually.
Hibernate
Hibernate is a popular ORM tool that provides more features than JPA. It can be used as a JPA implementation, but it also provides additional features beyond the JPA specification. Here’s an example of how to use Hibernate to execute a HQL (Hibernate Query Language) query:
Session session = sessionFactory.openSession();
Query query = session.createQuery("FROM Book WHERE author = :authorName");
query.setParameter("authorName", "John Doe");
List<Book> books = query.list();
# Output:
# 'JPA Guide'
In this example, we use a Session
to create a HQL query and execute it. Unlike JPQL, HQL supports polymorphic queries, which can be useful in some cases.
While Hibernate provides more features than JPA, it can be more complex and has a steeper learning curve. It’s also less standardized than JPA, which can lead to issues if you need to switch to a different ORM tool in the future.
In conclusion, while JPA is a powerful tool for data persistence in Java, it’s not the only option. Depending on your specific needs and circumstances, you might find that alternatives such as raw SQL, JDBC, or Hibernate are more suitable. Each approach has its pros and cons, so it’s important to choose the one that best fits your needs.
Troubleshooting JPA: Common Pitfalls and Solutions
While JPA provides a powerful and convenient way to handle data persistence in Java, it’s not without its challenges. In this section, we’ll discuss some common issues you might encounter when using JPA, along with solutions and best practices.
Performance Issues
One common issue when using JPA is performance. If not used correctly, JPA can lead to inefficient database queries, which can slow down your application.
For example, fetching large amounts of data can lead to performance issues. To mitigate this, you can use pagination to fetch data in smaller chunks:
String jpql = "SELECT b FROM Book b";
TypedQuery<Book> query = em.createQuery(jpql, Book.class);
query.setFirstResult(0);
query.setMaxResults(10);
List<Book> books = query.getResultList();
In this example, we use the setFirstResult
and setMaxResults
methods to fetch the first 10 Book
s.
Lazy Loading
Another common issue is lazy loading. By default, JPA fetches relationships lazily, meaning it only fetches the related entities when you access them. This can lead to the N+1 select issue, where JPA executes an additional SQL query for each related entity.
To mitigate this, you can use the JOIN FETCH
clause in your JPQL queries to fetch the related entities in the same query:
String jpql = "SELECT a FROM Author a JOIN FETCH a.books WHERE a.name = :authorName";
TypedQuery<Author> query = em.createQuery(jpql, Author.class);
query.setParameter("authorName", "John Doe");
Author author = query.getSingleResult();
In this example, we use the JOIN FETCH
clause to fetch the Author
and their Book
s in the same query.
Best Practices
When using JPA, there are several best practices you should follow. These include using the EntityManager
in a try-with-resources statement to ensure it’s closed properly, using TypedQuery
to avoid SQL injection attacks, and using the version
field for optimistic locking.
In conclusion, while JPA is a powerful tool for data persistence in Java, it’s not without its challenges. By being aware of these potential issues and following best practices, you can use JPA effectively in your Java applications.
JPA Fundamentals: Object-Relational Mapping, Persistence, and Transactions
Before diving deeper into JPA, it’s important to understand the fundamental concepts that underpin it: object-relational mapping, persistence, and transactions.
Object-Relational Mapping in JPA
Object-Relational Mapping (ORM) is a technique that lets you interact with your database, like you would with SQL. In other words, it allows you to manipulate and access your data as objects.
@Entity
public class Book {
@Id
private Long id;
private String title;
// getters and setters
}
In the above example, the Book
class is annotated with @Entity
, indicating that it’s a JPA entity. This means that the Book
objects can be automatically persisted to the database.
Understanding Persistence in JPA
Persistence, in the context of JPA, is about storing data between application sessions. When an object’s state is stored in a database, it’s said to be ‘persistent’.
Book book = new Book();
book.setTitle("JPA Guide");
em.persist(book);
In this example, we create a new Book
object and set its title. By calling the persist
method, we’re telling JPA to manage the Book
object, making it persistent.
Transactions in JPA
A transaction is a set of operations that are executed as a single unit of work. If all operations succeed, the transaction is committed. If any operation fails, the transaction is rolled back, and no changes are made to the database.
em.getTransaction().begin();
Book book = new Book();
book.setTitle("JPA Guide");
em.persist(book);
em.getTransaction().commit();
In this example, we start a transaction, persist a Book
object, and then commit the transaction. If the persist
operation fails for any reason, the transaction will be rolled back, and the Book
object will not be stored in the database.
By understanding these fundamentals, you’ll be better equipped to use JPA effectively in your Java applications.
JPA in the Bigger Picture: Enterprise Applications and Beyond
JPA is not just for small projects or simple applications. Its power and flexibility make it an ideal choice for larger projects, such as enterprise applications. Moreover, its compatibility with other frameworks and libraries allows it to be part of a larger ecosystem, providing a comprehensive solution for your Java persistence needs.
JPA in Enterprise Applications
In enterprise applications, where the complexity and scale of data are much larger, JPA shines with its robustness and versatility. It simplifies the data layer by providing a standardized approach to object-relational mapping, making it easier to manage complex data models and relationships.
@Entity
public class Employee {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
@ManyToOne
private Department department;
// getters and setters
}
In this example, we have an Employee
entity that is linked to a Department
entity. This kind of relationship is common in enterprise applications, and JPA makes it easy to handle.
JPA with Spring Data and Hibernate
JPA works well with other popular Java libraries and frameworks. For instance, Spring Data JPA is a popular library that adds an extra layer of functionality on top of JPA, making it even easier to work with data in Spring applications.
Hibernate is another powerful ORM tool that implements the JPA specification. It offers additional features beyond JPA, making it a popular choice for many Java developers.
Further Resources for Mastering JPA
To help you further your understanding of JPA and its use in larger projects, here are some resources you might find useful:
- Java Interface Use Cases Explored – Explore examples of interfaces in standard Java libraries.
Exploring JDBC in Java – Learn about JDBC drivers, connections, statements, and result sets.
JDBC Connection Usage – Master JDBC connection setup for efficient and reliable database interactions.
Java Persistence API: A Beginner’s Guide – An introductory guide to JPA from Oracle, the creators of Java.
Pro JPA 2 in Java EE 8 – A comprehensive book on using JPA in Java EE applications.
Hibernate ORM – The official website for Hibernate, a powerful JPA implementation with additional features.
By exploring these resources and experimenting with JPA in your projects, you’ll be well on your way to mastering JPA and effectively handling data persistence in your Java applications.
Wrapping Up: Java Persistence API (JPA)
In this comprehensive guide, we’ve delved into the world of Java Persistence API (JPA), a standard interface for accessing databases in Java. We’ve explored its basic to advanced usage, its alternatives, and even the common issues you might encounter while using it.
We began with the basics, learning how to set up and use JPA in a Java project. We then delved into more intricate aspects of JPA, such as handling relationships between entities, inheritance, and querying. We also discussed alternatives to JPA, such as raw SQL, JDBC, and other ORM tools like Hibernate, providing a sense of the broader landscape of tools for data persistence in Java.
Along the way, we tackled common challenges you might face when using JPA, such as performance issues and lazy loading, providing you with solutions and best practices for each issue.
Here’s a quick comparison of JPA and its alternatives:
Method | Pros | Cons |
---|---|---|
JPA | Standardized, Easy to use | Might require troubleshooting |
Raw SQL | Complete control, Direct interaction with DB | Verbose, Manual object mapping |
JDBC | More control than JPA, Protection against SQL injections | More boilerplate code, Manual object mapping |
Hibernate | More features than JPA, Supports polymorphic queries | More complex, Less standardized |
Whether you’re just starting out with JPA or you’re looking to level up your data persistence skills, we hope this guide has given you a deeper understanding of JPA and its capabilities.
With its balance of standardization and ease of use, JPA is a powerful tool for data persistence in Java. Now, you’re well equipped to handle data persistence in your Java applications effectively. Happy coding!