JDBC in Java: A Complete Guide to Mastering JDBC
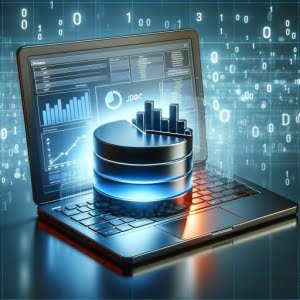
Are you grappling with JDBC in Java? You’re not alone. Many developers find JDBC a bit challenging, but it’s a crucial bridge that connects your Java application to a database, providing a vital link in your software.
In this guide, we will explore JDBC, its uses, and how to effectively leverage it in your Java applications. We’ll delve into everything from establishing a simple JDBC connection to executing complex queries and handling transactions. We’ll also touch on alternative approaches and common issues you might encounter along the way.
So, let’s dive in and start mastering JDBC in Java!
TL;DR: How Do I Use JDBC in Java?
JDBC (Java Database Connectivity)
is anAPI
in Java that allows applications to interact with databases. You can establish a JDBC connection, execute queries, and retrieve results using a few simple commands.
Here’s a simple example of a JDBC connection:
// Load JDBC driver
Class.forName('com.mysql.jdbc.Driver');
// Establish connection
Connection conn = DriverManager.getConnection('jdbc:mysql://localhost/test', 'user', 'password');
// Execute query
Statement stmt = conn.createStatement();
ResultSet rs = stmt.executeQuery('SELECT * FROM table');
In this example, we first load the JDBC driver, then establish a connection to the database. We create a statement object and execute a query, storing the result in a ResultSet object.
This is just a basic way to use JDBC in Java, but there’s much more to learn about executing complex queries, handling transactions, and dealing with different types of databases. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Establishing a JDBC Connection: A Beginner’s Guide
- JDBC Advanced Usage: PreparedStatement and Transactions
- Exploring Alternatives to JDBC: ORM Frameworks
- Troubleshooting JDBC: Common Issues and Solutions
- Understanding JDBC: The Architecture and Underlying Concepts
- JDBC in Large-Scale Applications: A Deeper Dive
- Wrapping Up: JDBC in Java
Establishing a JDBC Connection: A Beginner’s Guide
JDBC, or Java Database Connectivity, is a powerful tool that allows Java applications to interact with databases. In this section, we’ll walk you through the process of establishing a JDBC connection, executing queries, and retrieving results.
Establishing a JDBC Connection
The first step in using JDBC is to establish a connection to your database. Here’s a simple example of how you can do this:
// Load JDBC driver
Class.forName('com.mysql.jdbc.Driver');
// Establish connection
Connection conn = DriverManager.getConnection('jdbc:mysql://localhost/test', 'user', 'password');
In this code block, we first load the JDBC driver using the Class.forName()
method. This line is crucial because it loads the driver’s class file into memory so the JVM (Java Virtual Machine) can use it to establish a database connection.
Next, we establish a connection to the database using DriverManager.getConnection()
. This method takes in three arguments: a string representing the database URL, and two strings representing the username and password for the database.
Executing Queries and Retrieving Results
Once you have a connection, you can use it to create a Statement
object, which you can then use to execute SQL queries. Here’s an example of how to execute a query and retrieve results:
// Execute query
Statement stmt = conn.createStatement();
ResultSet rs = stmt.executeQuery('SELECT * FROM table');
// Process results
while (rs.next()) {
String name = rs.getString('name');
System.out.println(name);
}
# Output:
# 'John Doe'
# 'Jane Doe'
In this example, we first create a Statement
object using conn.createStatement()
. We then use the executeQuery()
method of the Statement
object to execute our SQL query. This method returns a ResultSet
object, which represents the result of our query.
We then use a while loop to iterate over the ResultSet
, retrieving each row of results. In this case, we’re retrieving a ‘name’ from each row and printing it to the console.
Advantages and Potential Pitfalls
The main advantage of using JDBC is its simplicity and direct control over the database. It allows you to execute any SQL query, providing you with the flexibility to interact with your database.
However, JDBC also has some potential pitfalls. One common issue is SQL injection, a security vulnerability that occurs when an attacker can insert malicious SQL code into your query. To mitigate this risk, always sanitize your inputs and use prepared statements, which we’ll cover in the next section.
The key to mastering JDBC is practice. As you become more comfortable with JDBC, you’ll be able to leverage its full power to interact with your databases.
JDBC Advanced Usage: PreparedStatement and Transactions
As you become more comfortable with JDBC, you can start to explore its more advanced features. This section will delve into the use of PreparedStatement
objects, handling transactions, and interacting with different types of databases.
PreparedStatement: An Advanced Tool
A PreparedStatement
is a precompiled SQL statement that can be executed multiple times without the cost of recompiling the SQL statement for each execution. It also provides a defense against SQL injection attacks. Here’s an example of using a PreparedStatement
:
// Prepare statement
String query = 'SELECT * FROM table WHERE id = ?';
PreparedStatement pstmt = conn.prepareStatement(query);
// Set parameter
pstmt.setInt(1, 10);
// Execute query
ResultSet rs = pstmt.executeQuery();
while (rs.next()) {
String name = rs.getString('name');
System.out.println(name);
}
# Output:
# 'John Doe'
In this example, we first prepare the SQL statement, leaving a question mark (?) as a placeholder for the id. We then set the parameter value using pstmt.setInt()
. Finally, we execute the query and process the results as before.
Handling Transactions
JDBC allows you to handle transactions, which are a series of actions that are treated as a single unit of work. Transactions ensure that either all actions are carried out, or none are, maintaining the integrity of your database. Here’s an example of handling transactions with JDBC:
try {
// Start transaction
conn.setAutoCommit(false);
// Execute statements
Statement stmt = conn.createStatement();
stmt.executeUpdate('INSERT INTO table VALUES (1, 'John')');
stmt.executeUpdate('INSERT INTO table VALUES (2, 'Jane')');
// Commit transaction
conn.commit();
} catch (SQLException e) {
// Rollback transaction
conn.rollback();
}
In this example, we first disable auto-commit for the connection, starting a new transaction. We then execute two INSERT
statements. If both statements execute successfully, we commit the transaction using conn.commit()
. If either statement throws a SQLException
, we rollback the transaction using conn.rollback()
, undoing any changes made during the transaction.
JDBC with Different Databases
JDBC allows you to interact with many different types of databases. The process is largely the same for each database—you just need to use the appropriate driver and connection string. For example, to connect to a PostgreSQL database, you would do the following:
// Load PostgreSQL driver
Class.forName('org.postgresql.Driver');
// Establish connection
Connection conn = DriverManager.getConnection('jdbc:postgresql://localhost/test', 'user', 'password');
In this code block, we load the PostgreSQL driver and establish a connection to a PostgreSQL database. The rest of the JDBC code (creating statements, executing queries, etc.) would remain the same.
Mastering these advanced features of JDBC will allow you to write more efficient and secure Java applications. Remember to always practice safe coding habits, such as using PreparedStatement
s and handling transactions properly.
Exploring Alternatives to JDBC: ORM Frameworks
While JDBC is a powerful tool for interacting with databases, it’s not the only option. Object-Relational Mapping (ORM) frameworks like Hibernate and Java Persistence API (JPA) provide alternative methods for database connectivity. These frameworks can simplify database operations and reduce the amount of boilerplate code you need to write.
Hibernate: Simplifying Database Operations
Hibernate is an ORM framework that maps Java classes to database tables and Java data types to SQL data types. It simplifies database operations by allowing you to interact with your database using Java objects and methods.
Here’s an example of using Hibernate to retrieve data from a database:
// Create a Configuration object
Configuration cfg = new Configuration();
// Load configuration file
cfg.configure('hibernate.cfg.xml');
// Create SessionFactory
SessionFactory sf = cfg.buildSessionFactory();
// Create Session
Session session = sf.openSession();
// Create Criteria
Criteria crit = session.createCriteria(Employee.class);
// Execute query
List<Employee> employees = crit.list();
for (Employee employee : employees) {
System.out.println(employee.getName());
}
# Output:
# 'John Doe'
# 'Jane Doe'
In this example, we first create a Configuration
object and load a configuration file. We then create a SessionFactory
and Session
. We create a Criteria
object, which represents a query, and execute the query using crit.list()
. We then process the results, which are returned as a list of Employee
objects.
JPA: A Standardized Approach
JPA, or Java Persistence API, is a standardized API for ORM in Java. It provides a simple, flexible way to map Java objects to database tables. Here’s an example of using JPA to retrieve data from a database:
// Create EntityManagerFactory
EntityManagerFactory emf = Persistence.createEntityManagerFactory('my-pu');
// Create EntityManager
EntityManager em = emf.createEntityManager();
// Create Query
Query q = em.createQuery('SELECT e FROM Employee e');
// Execute query
List<Employee> employees = q.getResultList();
for (Employee employee : employees) {
System.out.println(employee.getName());
}
# Output:
# 'John Doe'
# 'Jane Doe'
In this example, we first create an EntityManagerFactory
and EntityManager
. We create a Query
object, which represents a query, and execute the query using q.getResultList()
. We then process the results, which are returned as a list of Employee
objects.
Advantages and Disadvantages
ORM frameworks like Hibernate and JPA have several advantages over JDBC. They can simplify database operations, reduce boilerplate code, and provide a more object-oriented approach to database interaction. They also provide features like caching and lazy loading, which can improve performance.
However, ORM frameworks also have their disadvantages. They can be more complex to set up and use than JDBC, and they may not provide the same level of control over your database operations. Additionally, while ORM frameworks can improve performance in some cases, they can also introduce performance overhead in others.
When choosing between JDBC and an ORM framework, consider the complexity of your database operations, the performance requirements of your application, and your comfort level with each tool. In general, JDBC may be a better choice for simpler, performance-critical applications, while ORM frameworks may be more suitable for complex applications with extensive database operations.
Troubleshooting JDBC: Common Issues and Solutions
Working with JDBC is not always a smooth journey. You may encounter several issues, such as connection failures and SQL exceptions. In this section, we’ll discuss these common problems and provide solutions, workarounds, and tips to help you navigate your JDBC journey.
Connection Failures
One of the most common issues you may encounter is a failure to establish a connection to the database. This could be due to a variety of reasons, such as incorrect connection details or a network issue.
try {
// Establish connection
Connection conn = DriverManager.getConnection('jdbc:mysql://localhost/test', 'user', 'wrongpassword');
} catch (SQLException e) {
// Handle exception
System.out.println('Could not establish a connection.');
e.printStackTrace();
}
# Output:
# 'Could not establish a connection.'
# java.sql.SQLException: Access denied for user 'user'@'localhost' (using password: YES)
In this example, we’re trying to establish a connection with an incorrect password. As a result, a SQLException
is thrown. Always ensure you have the correct connection details and that the database is accessible from your application.
SQL Exceptions
Another common issue is encountering SQL exceptions. These exceptions are thrown when there’s an error in your SQL code.
try {
// Execute query
Statement stmt = conn.createStatement();
ResultSet rs = stmt.executeQuery('SELECT * FROM non_existent_table');
} catch (SQLException e) {
// Handle exception
System.out.println('Could not execute query.');
e.printStackTrace();
}
# Output:
# 'Could not execute query.'
# java.sql.SQLSyntaxErrorException: Table 'test.non_existent_table' doesn't exist
In this example, we’re trying to execute a query on a table that doesn’t exist. As a result, a SQLSyntaxErrorException
is thrown. Always ensure your SQL code is correct and that the tables and columns you’re referencing exist in the database.
Other Considerations
When using JDBC, it’s also important to consider the performance of your application. Executing SQL queries can be expensive, so it’s crucial to optimize your queries as much as possible. Also, always close your ResultSet
, Statement
, and Connection
objects when you’re done with them to free up resources.
// Close resources
rs.close();
stmt.close();
conn.close();
In this example, we’re closing the ResultSet
, Statement
, and Connection
objects. This is a good practice to follow to prevent resource leaks in your application.
Remember, every problem you encounter is an opportunity to learn and grow as a developer. So, keep these tips in mind, and you’ll be a JDBC master in no time!
Understanding JDBC: The Architecture and Underlying Concepts
Before we delve deeper into JDBC, it’s crucial to understand its architecture and the underlying concepts. This will give you a better grasp of how JDBC works, and why it’s such an essential tool for Java developers.
The JDBC API: An Overview
JDBC, short for Java Database Connectivity, is an API (Application Programming Interface) for the Java programming language. It provides methods for querying and updating data in a database, and is oriented towards relational databases.
The JDBC API includes several interfaces and classes, such as DriverManager
, Connection
, Statement
, and ResultSet
. These classes and interfaces provide methods for tasks like establishing a connection to a database, sending SQL statements, and processing the results.
JDBC Architecture: A Closer Look
The JDBC architecture consists of two layers: the JDBC API, which provides the application-to-JDBC Manager connection, and the JDBC Driver API, which supports the JDBC Manager-to-Driver Connection.
// Application-to-JDBC Manager Connection
Connection conn = DriverManager.getConnection(url, user, password);
// JDBC Manager-to-Driver Connection
Driver driver = DriverManager.getDriver(url);
In this example, the DriverManager.getConnection()
method establishes a connection between the application and the JDBC manager, while the DriverManager.getDriver()
method retrieves the driver object that will facilitate the connection between the JDBC manager and the driver.
SQL and Databases: The Foundation of JDBC
At the heart of JDBC is SQL, or Structured Query Language. SQL is a standard language for managing and manipulating relational databases. When you use JDBC, you’re essentially sending SQL statements to a database and processing the results.
// Execute SQL statement
Statement stmt = conn.createStatement();
stmt.execute('CREATE TABLE employees (id INT, name VARCHAR(30))');
# Output:
# Table 'employees' created.
In this example, we’re sending a SQL CREATE TABLE
statement to the database. This statement creates a new table named ’employees’ with two columns: ‘id’ and ‘name’.
Understanding JDBC, its architecture, and the underlying concepts of SQL and databases is the first step towards mastering JDBC. With this knowledge, you’ll be able to use JDBC effectively and leverage its full power in your Java applications.
JDBC in Large-Scale Applications: A Deeper Dive
JDBC’s power and flexibility make it an essential tool for building large-scale, database-driven applications. Its ability to interact with virtually any relational database and execute complex SQL queries gives developers the freedom to design and build robust, data-intensive applications.
Connection Pooling: A Key to Performance
In large-scale applications, performance is a key concern. One way to boost the performance of your JDBC operations is through connection pooling. Connection pooling is a technique used to manage and reuse database connections, reducing the overhead of establishing a new connection for each query.
// Create a connection pool
HikariConfig config = new HikariConfig();
config.setJdbcUrl('jdbc:mysql://localhost/test');
config.setUsername('user');
config.setPassword('password');
HikariDataSource ds = new HikariDataSource(config);
// Get a connection from the pool
Connection conn = ds.getConnection();
In this example, we’re using HikariCP, a popular connection pooling library. We first configure the connection pool, then retrieve a connection from the pool. This connection can be reused, saving the cost of establishing a new connection each time.
Database Design: Laying the Foundation
Proper database design is crucial in large-scale applications. A well-designed database can improve performance, ensure data integrity, and make your application easier to maintain. Considerations like table structure, indexing, and normalization all play a part in database design.
-- Create a well-structured table
CREATE TABLE employees (
id INT PRIMARY KEY,
name VARCHAR(30) NOT NULL,
department_id INT,
FOREIGN KEY (department_id) REFERENCES departments(id)
);
In this SQL statement, we’re creating a well-structured ’employees’ table. It includes a primary key, a not-null constraint, and a foreign key, all of which help ensure data integrity.
Further Resources for Mastering JDBC
To further your understanding of JDBC and its role in large-scale applications, consider exploring these resources:
- Enhancing Code Design with Java Interface – Understand the importance of documenting interfaces in Java code.
Java Serialization Guide – Understand the Java Serializable interface for object serialization.
JPA Usage in Java – Master JPA for seamless integration between Java objects and relational databases.
Oracle’s Official JDBC Documentation: A comprehensive guide to JDBC, including its architecture, API, and various features.
Baeldung’s Guide to JDBC: A series of tutorials covering various aspects of JDBC, from basic usage to advanced features.
JDBC Quick Guide by Tutorialspoint covers various topics of JDBC usage, including database connections and handling exceptions.
Wrapping Up: JDBC in Java
In this comprehensive guide, we’ve embarked on a journey to explore JDBC (Java Database Connectivity), a vital tool that provides a link between your Java application and a database. We’ve dug deep into its functionalities, usage, and how to effectively leverage it in your Java applications.
We began with the basics, elucidating how to establish a JDBC connection, execute queries, and retrieve results. We then ventured into more advanced usage, discussing complex uses of JDBC, such as handling transactions with PreparedStatements, and dealing with different types of databases. We also explored alternative methods for database connectivity, such as using ORM frameworks like Hibernate or JPA.
In addition, we tackled common issues one may encounter during JDBC usage, such as connection failures, SQL exceptions, and provided solutions and workarounds for each issue. We also took a deep dive into the JDBC API, its architecture, and the underlying concepts to give you a better understanding of its workings.
Here’s a quick comparison of the methods we’ve discussed:
Method | Flexibility | Complexity | Control over Database Operations |
---|---|---|---|
JDBC | High | Moderate | High |
Hibernate | Moderate | High | Moderate |
JPA | Moderate | High | Moderate |
Whether you’re a beginner just starting out with JDBC or an intermediate developer looking to level up your skills, we hope this guide has given you a deeper understanding of JDBC and its capabilities.
The ability to interact with databases is a powerful tool in the world of Java development. With this knowledge, you’re now well-equipped to leverage JDBC in your Java applications. Happy coding!