Bash ‘If Not’ Condition: A Negation Operator Guide
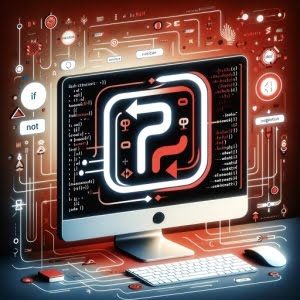
Are you finding the ‘if not’ command in bash scripting a bit perplexing? You’re not alone. Many developers find themselves in a tangle when it comes to handling ‘if not’ in bash scripting, but we’re here to help.
Think of ‘if not’ in bash scripting as a gatekeeper, a sentinel standing guard, allowing or denying access based on certain conditions. It’s a powerful tool that can help you control the flow of your scripts with precision.
In this guide, we’ll walk you through the ins and outs of using ‘if not’ in bash scripting, from its basic use to advanced techniques. We’ll explore its core functionality, delve into its advanced features, and even discuss common issues and their solutions.
So, let’s dive in and start mastering ‘if not’ in bash scripting!
TL;DR: How Do I Use ‘If Not’ in Bash Scripting?
'If not'
is used in bash scripting to check if a condition is not true.Not
is represented by the!
character and is used with the syntax,if [ ! {flag} {condition}].
It’s a powerful tool that can help you control the flow of your scripts with precision.
Here’s a simple example:
if [ ! -f /tmp/testfile ]; then
echo 'File does not exist'
fi
# Output:
# 'File does not exist'
In this example, the script checks if the file ‘/tmp/testfile’ does not exist and prints a message if it doesn’t. The ‘!’ symbol is used to represent ‘not’ in bash scripting, and ‘-f’ checks for the existence of a file. So, the condition ‘[ ! -f /tmp/testfile ]’ checks if the file ‘/tmp/testfile’ does not exist.
This is a basic way to use ‘if not’ in bash scripting, but there’s much more to learn about controlling the flow of your scripts. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Getting Started with ‘If Not’ in Bash Scripting
- Advanced ‘If Not’ Usage in Bash Scripting
- Exploring Alternative Approaches in Bash Scripting
- Troubleshooting ‘If Not’ in Bash Scripting
- Bash Scripting: The Fundamentals
- Expanding ‘If Not’ in Larger Bash Scripts
- Wrapping Up: Mastering ‘If Not’ in Bash Scripting
Getting Started with ‘If Not’ in Bash Scripting
Bash scripting is a powerful tool for automating tasks on Linux systems. One of the fundamental concepts in bash scripting is the use of conditional statements, among which ‘if not’ holds a pivotal role.
The ‘if not’ statement in bash scripting is used to check if a condition is not true. This can be particularly useful when we want to execute a block of code only when a certain condition is not met.
Let’s consider a simple example of checking if a string is not empty.
string="Hello, World!"
if [ ! "$string" ]; then
echo "String is empty"
else
echo "String is not empty"
fi
# Output:
# 'String is not empty'
In this example, we have a string variable named ‘string’ with the value ‘Hello, World!’. The ‘if not’ condition checks if the string is empty. Since our string is not empty, the script prints ‘String is not empty’.
The ‘if not’ condition in bash scripting is a powerful tool for controlling the flow of your scripts. It allows you to execute specific blocks of code based on whether a certain condition is not met. However, it’s important to use it correctly to avoid potential pitfalls, such as incorrect condition checks or unexpected behavior.
Advanced ‘If Not’ Usage in Bash Scripting
As you become more comfortable with bash scripting, you’ll find that the ‘if not’ condition can be combined with other conditions for more complex logic. This is often done using logical operators like ‘&&’ (AND) and ‘||’ (OR).
Combining ‘If Not’ with Other Conditions
Let’s look at an example where we combine ‘if not’ with the ‘&&’ operator.
string="Hello, World!"
file="/tmp/testfile"
if [ ! "$string" ] && [ ! -f "$file" ]; then
echo "String is empty and file does not exist"
else
echo "At least one condition is not met"
fi
# Output:
# 'At least one condition is not met'
In this script, we have a string variable ‘string’ and a file path ‘file’. The ‘if not’ conditions check if the string is empty and if the file does not exist. If both conditions are true, the script echoes ‘String is empty and file does not exist’. Otherwise, it echoes ‘At least one condition is not met’.
In this case, our string is not empty and the file exists, so the script prints ‘At least one condition is not met’. This example shows how you can combine ‘if not’ with other conditions to create more complex logic in your bash scripts.
Exploring Alternative Approaches in Bash Scripting
While ‘if not’ is a powerful tool in bash scripting, there are other commands and functions that can accomplish similar tasks. Understanding these alternatives can expand your scripting toolkit and help you write more efficient and readable code.
Using ‘case’ Statements
The ‘case’ statement in bash scripting provides a way to choose among multiple options based on the value of a variable or expression. This can be a more readable alternative to multiple ‘if not’ conditions.
string="Hello, World!"
case "$string" in
"") echo "String is empty" ;;
*) echo "String is not empty" ;;
esac
# Output:
# 'String is not empty'
In this script, the ‘case’ statement checks the value of the ‘string’ variable. If the string is empty, it echoes ‘String is empty’. Otherwise, it echoes ‘String is not empty’. This can be a more readable and efficient alternative to using ‘if not’ when you have multiple conditions to check.
Using ‘test’ Command
The ‘test’ command in bash scripting provides a way to check conditions without using ‘if’ statements. This can be a more concise alternative to ‘if not’.
string="Hello, World!"
test -z "$string" && echo "String is empty" || echo "String is not empty"
# Output:
# 'String is not empty'
In this script, the ‘test’ command checks if the string is empty. If the string is empty, it echoes ‘String is empty’. Otherwise, it echoes ‘String is not empty’. This can be a more concise and efficient alternative to using ‘if not’ for simple condition checks.
While ‘if not’ is a powerful tool in bash scripting, understanding and utilizing these alternatives can help you write more efficient and readable scripts.
Troubleshooting ‘If Not’ in Bash Scripting
As with any programming or scripting language, you may encounter errors or obstacles while using ‘if not’ in bash scripting. Understanding these common issues and their solutions can save you a lot of time and frustration.
Problem: Unmatched Square Brackets
One common error is forgetting to close the square brackets when using ‘if not’. This will result in a syntax error.
string="Hello, World!"
if [ ! "$string"
echo "String is not empty"
fi
# Output:
# bash: syntax error near unexpected token `echo'
As you can see, the script throws a syntax error because the square brackets in the ‘if not’ condition are not properly closed. The solution is to make sure you always close your square brackets.
string="Hello, World!"
if [ ! "$string" ]; then
echo "String is not empty"
fi
# Output:
# 'String is not empty'
Problem: Neglecting Quotation Marks
Another common error is neglecting to use quotation marks around variable names in conditions. This can lead to unexpected behavior if the variable is empty or contains spaces.
string=""
if [ ! $string ]; then
echo "String is empty"
else
echo "String is not empty"
fi
# Output:
# bash: [: too many arguments
As you can see, the script throws an error because the ‘if not’ condition is not properly formatted. The solution is to always use quotation marks around variable names in conditions.
string=""
if [ ! "$string" ]; then
echo "String is empty"
else
echo "String is not empty"
fi
# Output:
# 'String is empty'
Remember, bash scripting is a powerful tool, but it requires attention to detail and understanding of its syntax and conventions. By understanding these common errors and their solutions, you can write more reliable and efficient scripts.
Bash Scripting: The Fundamentals
Bash scripting is a powerful tool for automating tasks on Unix-like operating systems. It’s a command processor that allows users to type commands and execute them. Bash stands for ‘Bourne Again SHell’, an improvement of the original Bourne Shell (sh).
Conditional Statements in Bash
Conditional statements are a fundamental part of bash scripting, allowing you to control the flow of execution based on certain conditions. The ‘if not’ statement is one such conditional statement, and it’s used to check if a condition is not true.
if [ ! $condition ]; then
# Code to execute if the condition is not true
fi
In this example, the ‘if not’ statement checks if the condition is not true. If it’s not true, the code inside the ‘if’ statement is executed. This can be particularly useful for error checking or controlling the flow of your scripts.
The Importance of ‘If Not’
The ‘if not’ statement in bash scripting is a powerful tool for controlling the flow of your scripts. It allows you to execute specific blocks of code only when a certain condition is not met. This can be particularly useful for error checking or controlling the flow of your scripts.
Remember, bash scripting is a powerful tool, but it requires a solid understanding of its syntax and conventions. By mastering the ‘if not’ statement and other conditional statements, you can write more efficient and reliable bash scripts.
Expanding ‘If Not’ in Larger Bash Scripts
As you become more comfortable with the ‘if not’ statement in bash scripting, you’ll find that it’s not just useful for simple scripts, but can also be a powerful tool in larger scripts or projects. It’s versatility allows it to easily integrate with other commands and functions for more complex scenarios.
Integrating ‘If Not’ with Other Commands
In larger scripts, ‘if not’ often goes hand in hand with commands like ‘for’, ‘while’, and ‘case’. This allows you to create more sophisticated scripts that can handle a variety of scenarios.
for file in /tmp/*; do
if [ ! -f "$file" ]; then
echo "$file does not exist"
fi
done
# Output:
# '/tmp/testfile1 does not exist'
# '/tmp/testfile2 does not exist'
In this example, we use a ‘for’ loop to iterate over all files in the ‘/tmp’ directory. The ‘if not’ statement checks if each file exists, and if it doesn’t, it prints a message. This is a simple example of how ‘if not’ can be used in larger scripts or projects.
Further Resources for Bash Scripting Mastery
If you’re interested in learning more about bash scripting and expanding your knowledge, here are a few resources that you might find useful:
- Advanced Bash-Scripting Guide: An in-depth exploration of the art of shell scripting.
- Bash Guide for Beginners: A comprehensive guide for those new to bash scripting.
- Bash Script Negate If: Baeldung’s article shows how to use the negation operator “!” in Bash scripting.
Wrapping Up: Mastering ‘If Not’ in Bash Scripting
This comprehensive guide has taken you on a journey through the usage of ‘if not’ in bash scripting, a fundamental aspect of controlling script flow based on certain conditions.
We started with the basics, learning how to use ‘if not’ in its simplest form. We then delved into more advanced usage, combining ‘if not’ with other conditions and logical operators for more complex scenarios. Along the way, we addressed common issues and their solutions, ensuring you’re well-equipped to handle any obstacles that might come your way.
We also explored alternative approaches to ‘if not’, such as ‘case’ statements and the ‘test’ command, providing you with a broader toolkit for your bash scripting needs. Here’s a quick comparison of these methods:
Method | Pros | Cons |
---|---|---|
‘If not’ | Powerful, versatile | Can lead to complex code |
‘Case’ statement | Readable, efficient for multiple conditions | Less flexible than ‘if not’ |
‘Test’ command | Concise, efficient for simple conditions | Less intuitive than ‘if not’ |
Whether you’re just starting out with bash scripting or you’re looking to enhance your existing skills, we hope this guide has given you a deeper understanding of ‘if not’ and its capabilities.
With its versatility and power, ‘if not’ is a fundamental tool in any bash scripter’s arsenal. Now, you’re well equipped to harness its capabilities. Happy scripting!