Python Turtle Commands | Quick Start to Turtle Drawing
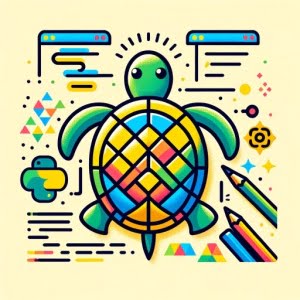
As we spend a lot of time working on the command line at IOFLOOD, we like to add fun python drawing in our scripts to liven the experience. The Python Turtle module is an excellent tool as it provides a simple interface for drawing shapes and patterns. Today’s article will cover the Python Turtle documentation, to help our customers looking to utilize their custom server configurations for graphical design.
In this guide, we’ll walk you through the process of using the Python turtle module, from the basics to more advanced techniques. We’ll cover everything from creating a turtle, moving it around the canvas, to using loops and conditionals to create complex shapes and designs.
Let’s get started!
TL;DR: How Do I Use the Python Turtle Module?
To use the turtle drawing module in Python, first import it with
import turtle
. Then use its methods like,t.penup()
,t.goto(-35, 35)
,t.pendown()
, andt.circle(10)
to make a python drawing.!
Here’s a basic example:
import turtle
my_turtle = turtle.Turtle()
my_turtle.forward(100)
turtle.done()
# Output:
# Draws a straight line of length 100
In this example, we first import the turtle module. Then, we create a turtle object using turtle.Turtle()
. The my_turtle.forward(100)
command makes the turtle move forward by 100 units, drawing a straight line. Finally, turtle.done()
indicates that we’re done drawing.
This is just the tip of the iceberg when it comes to Python’s turtle module. Keep reading for more detailed instructions, advanced usage examples, and some cool tricks you can do with Python turtle graphics!
Table of Contents
Getting Started with Python Turtle
Python turtle graphics is a popular choice for beginners to start learning about programming and graphics. It’s simple, fun, and incredibly powerful once you understand the basics. So, let’s dive into it.
Creating a Turtle
The first step in using the turtle module is to create a turtle. This is done using the Turtle()
function in the turtle module. Here’s how you do it:
import turtle
my_turtle = turtle.Turtle()
# Output:
# Creates a new turtle object
In this example, we first import the turtle module and then create a new turtle object, which we’ve named my_turtle
. This turtle object is what we’ll be using to draw on the canvas.
Moving the Turtle
Now that we have a turtle, let’s make it move. The turtle can move in a variety of ways, but the most basic command is forward(distance)
, which makes the turtle move forward by a specified distance. Let’s try it out:
my_turtle.forward(100)
# Output:
# Moves the turtle forward by 100 units
In this example, my_turtle.forward(100)
moves our turtle forward by 100 units, drawing a line as it moves.
Changing Turtle’s Shape and Color
Python turtle graphics allows you to change the shape and color of your turtle. This is done using the shape(shape_name)
and color(color_name)
methods. Let’s see how to do it:
my_turtle.shape('turtle')
my_turtle.color('red')
# Output:
# Changes the turtle's shape to a turtle and its color to red
In this example, my_turtle.shape('turtle')
changes the shape of our turtle to resemble a turtle. Similarly, my_turtle.color('red')
changes the color of our turtle to red.
These are just the basics of using Python’s turtle module. As you can see, it’s straightforward and intuitive. In the next section, we’ll explore some more advanced techniques you can use to create complex shapes and designs with Python turtle graphics.
Advanced Python Turtle Use
After getting comfortable with the basics of Python’s turtle module, you might be wondering, “How can I create more complex shapes and designs?”. The answer lies in the power of loops and conditionals in Python. Let’s explore how we can utilize these programming constructs to create intricate designs with Python turtle graphics.
Drawing with Loops
Loops in Python allow us to perform a task multiple times. This can be particularly useful when we want to draw a shape that has repeating patterns. For instance, let’s draw a square using a loop:
for i in range(4):
my_turtle.forward(100)
my_turtle.right(90)
# Output:
# Draws a square
In this example, we use a for
loop to repeat the commands my_turtle.forward(100)
and my_turtle.right(90)
four times. This effectively draws a square, with the turtle moving forward by 100 units and then turning right by 90 degrees at each iteration of the loop.
Creating Patterns with Conditionals
Conditionals allow us to control the flow of our program based on certain conditions. Let’s see how we can use a conditional to create a pattern:
for i in range(30):
if i % 2 == 0:
my_turtle.forward(100)
my_turtle.right(60)
else:
my_turtle.forward(50)
my_turtle.right(120)
# Output:
# Draws a complex pattern
In this example, we use a for
loop to repeat the commands 30 times. However, the commands that are executed depend on whether the current iteration number (i
) is even or odd. If i
is even, the turtle moves forward by 100 units and turns right by 60 degrees. If i
is odd, the turtle moves forward by 50 units and turns right by 120 degrees. This creates a complex pattern.
Using loops and conditionals with Python’s turtle module can open up a world of possibilities for creating complex shapes and designs. Play around with these concepts and see what you can create!
List of Python Turtle Commands
To further empower yourself in creating intricate designs with Pythons turtle module, it’s essential to have a good grasp of all the turtle commands and their functions. Here’s a table of Python turtle commands you might find useful:
Command | Description |
---|---|
turtle.forward(x) or turtle.fd(x) | Moves the turtle forward by specified distance x . |
turtle.backward(x) or turtle.bk(x) | Moves the turtle backward by specified distance x . |
turtle.right(x) or turtle.rt(x) | Turns the turtle right by specified angle x . |
turtle.left(x) or turtle.lt(x) | Turns the turtle left by specified angle x . |
turtle.penup() or turtle.pu() | Lifts the pen such that moving turtle doesn’t draw on the screen. |
turtle.pendown() or turtle.pd() | Puts the pen down such that moving turtle draws on the screen. |
turtle.pensize(x) or turtle.width(x) | Sets the pen size to the provided width x . |
turtle.color("color") | Changes the color of the turtle’s pen to the provided color. |
turtle.speed(x) | Sets the speed of the turtle’s movement to the specified x . |
turtle.shape("shape") | Changes the shape of the turtle to the provided shape (e.g., “turtle”, “arrow”, “circle”, etc.). |
After mastering these commands, together with loops and conditionals, you’ll have all the necessary tools to start creating more complex designs. The real magic stems from combining these tools in innovative ways, so don’t shy away from experimenting with different combinations of commands, loops, and conditionals to achieve your desired design.
Alternative Python Drawing Tools
While Python’s turtle module is a fantastic tool for creating graphics, it’s not the only option out there. In this section, we’ll explore two other popular Python libraries for creating graphics: Pygame and Matplotlib.
Pygame: A Game Developer’s Friend
Pygame is a set of Python modules designed for writing video games. However, it’s also great for creating interactive graphics. Here’s a simple example of how to create a window with Pygame:
import pygame
pygame.init()
win = pygame.display.set_mode((500, 500))
# Output:
# Creates a new window with a width and height of 500
In this example, we first import the pygame module and initialize all its modules using pygame.init()
. Then, we create a new window with a width and height of 500 using pygame.display.set_mode((500, 500))
.
The benefits of using Pygame include its flexibility and the ability to handle user input, sound, and more. However, it can be more complex and harder to learn than the turtle module.
Matplotlib: The Scientist’s Choice
Matplotlib is a plotting library for Python that is widely used in the scientific community. It’s great for creating static, animated, and interactive plots in Python. Here’s an example of how to create a simple line plot with Matplotlib:
import matplotlib.pyplot as plt
plt.plot([1, 2, 3, 4], [1, 4, 9, 16])
plt.show()
# Output:
# Creates a line plot with the x-values [1, 2, 3, 4] and the y-values [1, 4, 9, 16]
In this example, we first import the pyplot
module from Matplotlib. Then, we create a line plot with the x-values [1, 2, 3, 4] and the y-values [1, 4, 9, 16] using plt.plot([1, 2, 3, 4], [1, 4, 9, 16])
. Finally, we display the plot using plt.show()
.
The benefits of using Matplotlib include its extensive functionality and the ability to create a wide variety of plots. However, it’s not as intuitive as the turtle module and might be overkill for simple graphics.
In conclusion, while the turtle module is a great tool for creating graphics in Python, Pygame and Matplotlib offer alternative approaches that might be more suitable depending on your needs.
Troubleshooting Python Turtle
While working with Python turtle graphics, you may encounter a few common errors or obstacles. Don’t worry – we’ve got you covered. In this section, we’ll discuss some of these common issues, their solutions, and some best practices to optimize your Python turtle experience.
Common Errors and Their Solutions
One common error is forgetting to initialize the turtle module. This can result in an error message like NameError: name 'turtle' is not defined
. To solve this, make sure you import the turtle module at the beginning of your script:
import turtle
# Output:
# Imports the turtle module
Another common issue is trying to move the turtle before it’s been created. This can result in an error message like AttributeError: 'module' object has no attribute 'forward'
. To solve this, ensure you create your turtle object before trying to move it:
my_turtle = turtle.Turtle()
my_turtle.forward(100)
# Output:
# Creates a turtle and moves it forward by 100 units
Best Practices and Optimization Tips
Here are some tips to help you make the most of Python’s turtle module:
- Always clean up: It’s a good practice to end your turtle script with
turtle.done()
. This ensures that your window waits for a user action before it closes. Use loops for repetition: If you find yourself writing the same turtle commands over and over, consider using a loop. It’ll make your code cleaner and easier to read.
Experiment with speed: You can control the speed of your turtle using the
speed()
method. This can be useful if you want to slow down your turtle to see exactly what it’s doing.
Python’s turtle module is a powerful tool for creating graphics, but like any tool, it has its quirks. By understanding these common errors and best practices, you can make your journey with Python turtle graphics smoother and more enjoyable.
History of Turtle Drawing
Before we delve deeper into Python’s turtle module, let’s take a moment to understand the history and fundamentals of turtle graphics. This knowledge will not only give you a deeper appreciation of the tool you’re using but also help you use it more effectively.
The Origins of Turtle Graphics
The concept of turtle graphics dates back to the 1960s and the Logo programming language. The idea was to control a small robot, called a ‘turtle’, that moved around the floor drawing shapes. The turtle had a pen attached to it, and by moving it forward, backward, or rotating it, the turtle could draw intricate designs.
Python’s turtle module is a modern implementation of this concept, with the turtle being a virtual object on a graphical canvas instead of a physical robot.
Object-Oriented Programming and the Turtle Module
The turtle module in Python is a classic example of object-oriented programming (OOP). In OOP, we structure our program using objects that combine data and functionality. In the case of the turtle module, the turtle object has data (like its position and color) and methods (like forward()
and right()
) that act on this data.
Here’s an example of how we create and manipulate a turtle object:
import turtle
# Create a turtle object
my_turtle = turtle.Turtle()
# Use the turtle's methods
my_turtle.forward(100)
my_turtle.right(90)
# Output:
# Creates a turtle object and moves it forward by 100 units, then turns it right by 90 degrees
In this example, my_turtle
is an object of the Turtle
class provided by the turtle module. We can use the methods of this class to move the turtle around the canvas, change its color, and much more.
Understanding the object-oriented nature of the turtle module can help you use it more effectively and also gives you a glimpse into the powerful world of OOP in Python.
Practical Uses: Python Turtle Drawing
Python’s turtle module is more than just a tool for drawing shapes and designs. It’s an excellent resource for teaching programming concepts, creating games, and much more. In this section, we’ll explore some of the broader applications of the turtle module and suggest related topics for further learning.
Teaching Programming Concepts with Turtle
The turtle module’s simplicity and visual nature make it an excellent tool for teaching programming concepts. By drawing shapes and patterns, learners can get immediate visual feedback on their code, making abstract concepts like loops and conditionals more tangible.
Here’s an example of how you might use the turtle module to teach loops:
for i in range(4):
my_turtle.forward(100)
my_turtle.right(90)
# Output:
# Draws a square, demonstrating the concept of a loop
In this example, the learner can see how the for
loop repeats the commands four times to draw a square, providing a concrete example of how loops work.
Creating Games with Turtle
While the turtle module might not be as powerful as game-specific libraries like Pygame, it’s still possible to create simple games with it. For instance, you could create a ‘snake’ game, where the player controls a constantly moving turtle that needs to avoid obstacles.
Event-Driven Programming and Game Development
Working with the turtle module can also lead to more advanced topics like event-driven programming, a programming paradigm where the flow of the program is determined by events like mouse clicks or key presses. This is a crucial concept in game development and GUI applications.
Further Resources for Python Turtle Mastery
If you’re interested in diving deeper into Python’s turtle module, here are some resources that you might find helpful:
- Python Modules Essentials: Quick Guide – Discover the standard library modules that come built-in with Python.
Simplifying Data Reduction with Python Reduce – Discover how to simplify complex operations using Python’s “reduce.”
Data Security with Python’s hashlib – Dive into hashing algorithms and data security in Python.
Python’s Official Turtle Module Documentation is the most comprehensive resource available.
Python Turtle Graphics Tutorial by Real Python is a great introduction to the turtle module and includes a lot of examples.
Python Turtle Tutorial provides an insight into Python’s Turtle module, with a blend of theory and practical examples.
Recap: Python Turtle Documentation
In this comprehensive guide, we’ve explored the ins and outs of Python’s turtle module, a powerful tool for creating graphics in Python.
We began with the basics, learning how to create a turtle and manipulate it to draw simple shapes. We then dove into more advanced techniques, using loops and conditionals to create complex designs and patterns. Along the way, we tackled common challenges you might face when using the turtle module, providing you with solutions and best practices to overcome these hurdles.
We also ventured beyond the turtle module, exploring alternative Python libraries for creating graphics, such as Pygame and Matplotlib. Here’s a quick comparison of these libraries:
Library | Flexibility | Ease of Use |
---|---|---|
Python Turtle | Moderate | High |
Pygame | High | Moderate |
Matplotlib | High | Low |
Whether you’re a beginner just starting out with Python’s turtle module or an experienced developer looking to level up your graphics creation skills, we hope this guide has given you a deeper understanding of Python’s turtle module and its capabilities.
With its balance of simplicity, versatility, and power, Python’s turtle module is a fantastic tool for creating graphics in Python. Happy coding!