Python Optional Arguments | Guide (With Examples)
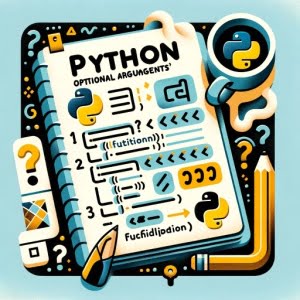
Are you finding it challenging to work with optional arguments in Python? You’re not alone. Many developers find themselves in a similar situation, but there’s a tool that can make this process a breeze.
Think of Python’s optional arguments as a Swiss army knife – they can make your Python functions more flexible and powerful, providing a versatile and handy tool for various tasks.
This guide will walk you through the use of optional arguments in Python, from basic usage to advanced techniques. We’ll cover everything from defining and using optional arguments, handling different types of arguments, to troubleshooting common issues.
So, let’s dive in and start mastering Python optional arguments!
TL;DR: How Do I Use Optional Arguments in Python?
In Python, you can make function arguments optional by assigning them a default value, such as
def greet(name='World'):
. This allows the function to be called with fewer arguments than it is defined to allow.
Here’s a simple example:
def greet(name='World'):
print(f'Hello, {name}!')
greet()
greet('Python')
# Output:
# 'Hello, World!'
# 'Hello, Python!'
In this example, the function greet
is defined with one argument name
that has a default value of ‘World’. When greet()
is called without any argument, it uses the default value. When we call greet('Python')
, it overrides the default value with the provided argument.
This is just a basic introduction to using optional arguments in Python. There’s much more to learn, including advanced usage and best practices. So, let’s dive deeper!
Table of Contents
- Grasping the Basics of Python Optional Arguments
- Mixing Optional Arguments with Positional Arguments
- Exploring Alternative Methods for Optional Arguments
- Navigating Common Challenges with Python Optional Arguments
- Unraveling the Fundamentals of Python Function Arguments
- The Power of Optional Arguments in Larger Python Projects
- Wrapping Up: Mastering Python Optional Arguments
Grasping the Basics of Python Optional Arguments
At its core, an optional argument in Python is a function argument that assumes a default value if a value is not provided in the function call for that argument. It’s a powerful tool that can make your functions more versatile and easier to use.
Defining and Using Optional Arguments
Defining optional arguments in Python is straightforward. You simply assign a default value to the argument in the function definition. Let’s take a look at a simple example:
def greet(name='World'):
print(f'Hello, {name}!')
greet()
greet('Python')
# Output:
# 'Hello, World!'
# 'Hello, Python!'
In this example, we defined a function greet
with one argument name
. The name
argument has a default value of ‘World’. When we call greet()
without any arguments, it uses the default value and prints ‘Hello, World!’. When we call greet('Python')
, it overrides the default value with the provided argument and prints ‘Hello, Python!’.
Advantages and Potential Pitfalls
Using optional arguments in Python can make your functions more flexible. They allow you to create functions that can handle a varying number of arguments, making your code more reusable and easier to maintain.
However, there are some potential pitfalls to be aware of. One common mistake is forgetting that the default value is only evaluated once, at the point of function definition.
This can lead to unexpected behavior when using mutable default values, like lists or dictionaries.
For instance, consider the following code:
def append_to(num, target=[]):
target.append(num)
return target
print(append_to(1))
print(append_to(2))
# Output:
# [1]
# [1, 2]
You might expect the function to return [1]
and then [2]
but it doesn’t. This is because the default value for target
is evaluated once and the same list is used in each successive call.
To avoid this pitfall, it’s recommended to use None
as the default value and create a new object inside the function, if necessary.
Mixing Optional Arguments with Positional Arguments
Python allows you to use optional arguments alongside positional arguments. Positional arguments are those whose values are assigned based on their order in the function call. Let’s look at an example:
def greet(greeting, name='World'):
print(f'{greeting}, {name}!')
greet('Hello')
greet('Hello', 'Python')
# Output:
# 'Hello, World!'
# 'Hello, Python!'
In this example, the greet
function has two arguments: greeting
(positional argument) and name
(optional argument). When we call greet('Hello')
, it assigns ‘Hello’ to greeting
and uses the default value for name
. When we call greet('Hello', 'Python')
, it assigns ‘Hello’ to greeting
and ‘Python’ to name
.
Combining Optional Arguments with Variable-Length Arguments
Python also gives you the ability to define functions with a variable number of arguments. These are known as variable-length arguments and are defined using *args for positional arguments and **kwargs for keyword arguments. Here’s how you can use them with optional arguments:
def func(required_arg, *args, optional_arg='default'):
print(f'Required argument: {required_arg}')
print(f'Optional argument: {optional_arg}')
for arg in args:
print(f'Additional argument: {arg}')
func('Hello', 'World', 'Python', optional_arg='not default')
# Output:
# Required argument: Hello
# Optional argument: not default
# Additional argument: World
# Additional argument: Python
In this example, func
has a required argument, an optional argument, and a variable-length argument. The variable-length argument *args
collects any additional positional arguments and packs them into a tuple. The optional_arg
uses its default value unless specified otherwise in the function call.
Best Practices
While Python’s flexibility with arguments can be powerful, it’s important to use these tools judiciously to keep your code clear and maintainable. Here are a few best practices:
- Keep the number of arguments reasonable. Too many can make your function calls difficult to read and understand.
Use optional arguments to provide sensible defaults but remember that the default value is only evaluated once, at the point of function definition.
When using a mix of positional, optional, and variable-length arguments, order them correctly in the function definition: required positional arguments, then optional arguments, and finally
*args
and/or**kwargs
.
Exploring Alternative Methods for Optional Arguments
Python’s flexibility doesn’t stop at basic optional arguments. There are more advanced techniques you can use to make your functions even more powerful and flexible. Two such techniques involve the use of *args
and **kwargs
.
Using *args and **kwargs
*args
and **kwargs
are special syntaxes in Python used in function definitions to allow for variable numbers of arguments. *args
is used to pass a non-keyworded, variable-length argument list, while **kwargs
allows you to pass keyworded, variable-length arguments. Let’s see how this works in practice:
def func(*args, **kwargs):
for arg in args:
print(f'Non-keyworded argument: {arg}')
for key, value in kwargs.items():
print(f'Keyworded argument: {key} = {value}')
func('Hello', 'World', greeting='Hello', name='Python')
# Output:
# Non-keyworded argument: Hello
# Non-keyworded argument: World
# Keyworded argument: greeting = Hello
# Keyworded argument: name = Python
In this example, func
takes any number of non-keyworded and keyworded arguments. *args
collects the non-keyworded arguments into a tuple, and **kwargs
collects the keyworded arguments into a dictionary.
Advantages and Disadvantages
Using *args
and **kwargs
can make your functions incredibly flexible, as they can handle any number of arguments. This can be especially useful when you’re not sure how many arguments your function might need, or when you’re working with APIs or libraries that require such functionality.
However, there are also disadvantages to consider. Functions that accept *args
and **kwargs
can be more difficult to understand and debug, as it’s not immediately clear what arguments they accept. They can also lead to less explicit and self-documenting code, which can be an issue in large codebases or collaborative environments.
Recommendations
While *args
and **kwargs
are powerful tools, they should be used judiciously. Use them when they provide clear benefits, but avoid overusing them, as they can make your code harder to understand and maintain. Always strive to write clear, explicit code that other developers (or you in the future) can easily understand and work with.
While optional arguments in Python can be extremely beneficial, they also come with a few potential pitfalls. Let’s discuss some common issues you might encounter and how to overcome them.
Incorrect Argument Order
One common issue is incorrect argument order. In Python, positional arguments must always precede keyword arguments (including optional arguments). Failing to adhere to this order will result in a SyntaxError:
def greet(greeting, name='World'):
print(f'{greeting}, {name}!')
greet(name='Python', 'Hello')
# Output:
# SyntaxError: positional argument follows keyword argument
In the above example, we incorrectly placed the positional argument ‘Hello’ after the keyword argument name='Python'
. To correct this, simply ensure that all positional arguments precede keyword arguments:
def greet(greeting, name='World'):
print(f'{greeting}, {name}!')
greet('Hello', name='Python')
# Output:
# 'Hello, Python!'
Missing Arguments
Another common issue is missing arguments. If a function expects an argument (whether positional or optional) and you forget to provide it, Python will raise a TypeError:
def greet(greeting, name='World'):
print(f'{greeting}, {name}!')
greet()
# Output:
# TypeError: greet() missing 1 required positional argument: 'greeting'
In this example, we forgot to provide the required positional argument greeting
when calling the function greet()
. To fix this, make sure to provide all required arguments in your function calls.
Remember, Python optional arguments can make your code more flexible and powerful, but they require careful handling. Always keep track of your argument order and make sure all required arguments are provided to avoid these common issues.
Unraveling the Fundamentals of Python Function Arguments
To fully grasp the concept of optional arguments in Python, it’s crucial to understand the fundamentals of function arguments in Python, including positional and keyword arguments, and the concept of default values.
Python Function Arguments
In Python, a function argument is a value that is passed into a function when it’s called. There are two types of function arguments in Python: positional arguments and keyword arguments.
def greet(greeting, name):
print(f'{greeting}, {name}!')
greet('Hello', 'Python')
# Output:
# 'Hello, Python!'
In the above example, ‘Hello’ and ‘Python’ are positional arguments. They are called ‘positional’ because their values are assigned based on their position in the function call.
Positional vs Keyword Arguments
The main difference between positional and keyword arguments lies in how they are passed to a function. Positional arguments are passed in the order in which they are defined, while keyword arguments are passed by explicitly naming each argument and its value.
def greet(greeting, name):
print(f'{greeting}, {name}!')
greet(name='Python', greeting='Hello')
# Output:
# 'Hello, Python!'
In this example, ‘Hello’ and ‘Python’ are keyword arguments. Notice that we can change the order of the arguments in the function call, because we are explicitly naming each argument.
Understanding Default Values
In Python, you can assign a default value to a function argument. This makes the argument optional, because the function can be called without providing a value for this argument. The function will then use the default value.
def greet(greeting='Hello', name='World'):
print(f'{greeting}, {name}!')
greet()
greet(name='Python', greeting='Hi')
# Output:
# 'Hello, World!'
# 'Hi, Python!'
In this example, both greeting
and name
have default values. The greet()
function can be called without any arguments, in which case it uses the default values. Alternatively, we can override the default values by providing our own arguments.
By understanding these fundamentals, you’ll have a solid foundation for mastering the use of optional arguments in Python.
The Power of Optional Arguments in Larger Python Projects
Python optional arguments are not just for small scripts or simple functions. They play a crucial role in larger Python projects and libraries as well. They can make your code more flexible, easier to read, and maintain.
Optional Arguments in Python Libraries
Many popular Python libraries make extensive use of optional arguments. For example, libraries like Pandas and NumPy, which are widely used for data analysis and manipulation, have numerous functions with optional arguments. This allows these libraries to provide a high level of flexibility and customization, while keeping their APIs clean and easy to use.
Exploring Related Concepts
Once you’ve mastered Python optional arguments, there are many related concepts to explore. For instance, function overloading allows you to define multiple functions with the same name but different arguments. Python doesn’t support function overloading in the same way as languages like C++ or Java, but you can achieve similar functionality using optional arguments, *args
, and **kwargs
.
Decorators are another powerful feature in Python that can work with optional arguments. A decorator is a function that takes another function and extends the behavior of the latter function without explicitly modifying it. This can be particularly useful when you want to modify the behavior of a function based on its arguments.
Further Resources for Mastering Python Optional Arguments
If you’re interested in diving deeper into Python optional arguments and related concepts, here are some resources you might find useful:
- Python’s official documentation on defining functions provides a thorough overview of function arguments, including optional arguments.
Real Python’s guide on function arguments offers an in-depth look at positional arguments, keyword arguments,
*args
, and**kwargs
.Python’s official documentation on decorators provides a concise explanation of decorators and how they work.
By understanding and mastering Python optional arguments, you’ll be well-equipped to write more flexible, maintainable, and powerful Python code.
Wrapping Up: Mastering Python Optional Arguments
In this comprehensive guide, we’ve delved into the world of optional arguments in Python. We’ve explored their basic usage, how they can be used alongside positional arguments, and even more advanced techniques involving *args
and **kwargs
.
We began with the basics, explaining how to define and use optional arguments in Python functions. We then moved on to more advanced territory, discussing how to use optional arguments with positional arguments and variable-length arguments.
We also explored alternative approaches, such as using *args
and **kwargs
, providing you with a wide range of tools to make your Python functions more flexible and powerful.
Along the way, we tackled common challenges you might face when using optional arguments, such as incorrect argument order and missing arguments, providing you with solutions and workarounds for each issue.
Here’s a quick comparison of the methods we’ve discussed:
Method | Flexibility | Complexity |
---|---|---|
Basic Optional Arguments | Moderate | Low |
Mixed Positional and Optional Arguments | High | Moderate |
Using *args and **kwargs | Very High | High |
Whether you’re just starting out with Python or you’re looking to level up your skills, we hope this guide has given you a deeper understanding of optional arguments and their capabilities.
Mastering Python optional arguments can make your code more flexible, robust, and easier to maintain. With the knowledge you’ve gained from this guide, you’re well-equipped to tackle any challenge that comes your way. Happy coding!