Bash Boolean Variables: A Shell Scripting Syntax Guide
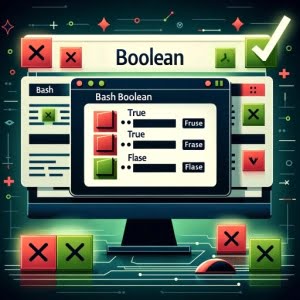
Are you finding it challenging to use boolean variables in Bash? You’re not alone. Many developers find themselves puzzled when it comes to handling boolean variables in Bash, but we’re here to help.
Think of Bash boolean variables as a traffic light – controlling the flow of your script, providing a versatile and handy tool for various tasks.
In this guide, we’ll walk you through the process of working with boolean variables in Bash, from their declaration, manipulation, and usage. We’ll cover everything from the basics of boolean variables to more advanced techniques, as well as alternative approaches.
Let’s get started!
TL;DR: How Do I Use Boolean Variables in Bash?
In Bash,
boolean variables
are delcared by assigning values such as0
, fortrue
, or 1, forfalse
. You must then utilize conditional statements likeif
to check the value of a boolean variable, such asif [ $is_true -eq 0 ]; then echo 'True'
. Here’s a simple example of how to use boolean variables in Bash:
is_true=0
if [ $is_true -eq 0 ]; then
echo 'True'
else
echo 'False'
fi
# Output:
# 'True'
In this example, we declare a variable is_true
and assign it a value of 0 (true). We then use a conditional if
statement to check the value of is_true
. If is_true
equals 0, the script prints ‘True’. Otherwise, it prints ‘False’. In this case, since is_true
is indeed 0, our output is ‘True’.
This is a basic way to use boolean variables in Bash, but there’s much more to learn about controlling the flow of your scripts with boolean logic. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Basic Use of Boolean Variables in Bash
- Advanced Usage of Boolean Variables in Bash
- Alternative Techniques to Boolean Variables in Bash
- Troubleshooting Boolean Variables in Bash
- Best Practices and Optimization Tips
- Understanding Bash Boolean Variables
- The Importance of Boolean Variables in Bash
- Expanding the Scope: Boolean Variables in Larger Bash Projects
- Wrapping Up: Mastering Boolean Variables in Bash
Basic Use of Boolean Variables in Bash
Let’s start with the basics. In Bash, boolean variables are represented by the numbers 0 and 1. The number 0 stands for true, and the number 1 stands for false. This might seem counterintuitive if you’re used to other programming languages where 1 is true and 0 is false, but it’s a convention that comes from the Unix world, where a program that exits with 0 is considered to have completed successfully (true), and a non-zero exit code indicates an error (false).
Here’s an example of how to declare and use boolean variables in Bash:
# Declare a boolean variable
is_valid=0
# Use the boolean variable in an if statement
if [ $is_valid -eq 0 ]; then
echo 'Valid'
else
echo 'Invalid'
fi
# Output:
# 'Valid'
In this example, we declare a variable is_valid
and assign it a value of 0 (true). We then use a conditional if
statement to check the value of is_valid
. If is_valid
equals 0, the script prints ‘Valid’. Otherwise, it prints ‘Invalid’. Since is_valid
is 0, our output is ‘Valid’.
Pros and Cons of Using Boolean Variables in Bash
Boolean variables in Bash are a powerful tool for controlling the flow of your scripts. They allow you to make decisions and perform different actions based on the truthiness of certain conditions. However, they also come with some caveats.
Pros:
- Simplicity: Boolean variables are simple and straightforward to use. Once you understand their logic, you can easily incorporate them into your scripts.
Control: They provide excellent control over the flow of your scripts, allowing you to dictate what happens under specific conditions.
Cons:
- Confusion: The fact that 0 is true and 1 is false in Bash can be confusing, especially for beginners or those coming from other programming languages.
Limited scope: Boolean variables can only hold two states, true or false. This can be limiting in situations where you need to represent more complex states or conditions.
Advanced Usage of Boolean Variables in Bash
As you grow more comfortable with using boolean variables in Bash, you can start incorporating them into more complex constructs, such as conditional statements and loops. This allows you to create more dynamic and flexible scripts.
Using Boolean Variables in Conditional Statements
Boolean variables can be used to control the flow of your script through conditional statements. Here’s an example of how you can use a boolean variable in an if-else
statement:
# Declare a boolean variable
file_exists=0
# Use the boolean variable in an if-else statement
if [ $file_exists -eq 0 ]; then
echo 'The file exists.'
else
echo 'The file does not exist.'
fi
# Output:
# 'The file exists.'
In this example, we declare a variable file_exists
and assign it a value of 0 (true). We then use a conditional if-else
statement to check the value of file_exists
. If file_exists
equals 0, the script prints ‘The file exists.’ Otherwise, it prints ‘The file does not exist.’ Since file_exists
is 0, our output is ‘The file exists.’
Using Boolean Variables in Loops
Boolean variables can also be used to control the execution of loops. Here’s an example of how you can use a boolean variable in a while
loop:
# Declare a boolean variable
keep_looping=0
# Initialize a counter
counter=0
# Use the boolean variable in a while loop
while [ $keep_looping -eq 0 ]; do
counter=$((counter + 1))
echo "Loop $counter"
if [ $counter -ge 5 ]; then
keep_looping=1
fi
done
# Output:
# 'Loop 1'
# 'Loop 2'
# 'Loop 3'
# 'Loop 4'
# 'Loop 5'
In this example, we declare a variable keep_looping
and assign it a value of 0 (true). We then use a while
loop that continues to run as long as keep_looping
equals 0. Inside the loop, we increment a counter and print its value. If the counter reaches 5, we change the value of keep_looping
to 1 (false), which causes the loop to exit. Our output is the numbers 1 through 5, each on a new line.
Alternative Techniques to Boolean Variables in Bash
While boolean variables are a powerful tool in Bash, there are alternative techniques that can accomplish similar tasks. One such approach is using string comparison instead of boolean variables.
Using String Comparison in Bash
In Bash, you can compare strings using the ==
operator within an if
statement. This can serve as an alternative to using boolean variables. Here’s an example:
# Declare a string variable
status="success"
# Use the string variable in an if statement
if [ "$status" == "success" ]; then
echo 'Operation was successful.'
else
echo 'Operation failed.'
fi
# Output:
# 'Operation was successful.'
In this example, we declare a variable status
and assign it a string value of ‘success’. We then use a conditional if
statement to compare the value of status
to the string ‘success’. If they match, the script prints ‘Operation was successful.’ Otherwise, it prints ‘Operation failed.’ Since status
is indeed ‘success’, our output is ‘Operation was successful.’
Benefits and Drawbacks of Using String Comparison
Like boolean variables, string comparison in Bash has its pros and cons.
Pros:
- Flexibility: String comparison can handle more than just two states, unlike boolean variables which are limited to true or false.
Readability: String comparison can make your code more readable, as the strings can convey more information than simple true or false values.
Cons:
- Performance: Comparing strings can be slower than comparing integer values, especially for long strings or in scripts with a lot of comparisons.
Error-prone: String comparison can be more error-prone, as it requires an exact match. A small typo can lead to unexpected results.
When deciding whether to use boolean variables or string comparison in your Bash scripts, consider the specific requirements of your task and the trade-offs of each approach.
Troubleshooting Boolean Variables in Bash
Working with boolean variables in Bash can sometimes lead to unexpected results or errors. Let’s look at some common issues and how to solve them.
Incorrectly Interpreting True and False
A common mistake when using boolean variables in Bash is to interpret 1 as true and 0 as false, which is the opposite of the Bash convention. Here’s an example of this mistake:
# Declare a boolean variable
is_valid=1
# Use the boolean variable in an if statement
if [ $is_valid -eq 0 ]; then
echo 'Valid'
else
echo 'Invalid'
fi
# Output:
# 'Invalid'
In this example, we declare a variable is_valid
and assign it a value of 1, intending it to represent true. However, in the if
statement, we check if is_valid
equals 0, which leads to the script printing ‘Invalid’, not ‘Valid’ as we might expect.
Forgetting to Quote Variable Substitutions
Another common mistake is forgetting to quote variable substitutions in test constructs, which can lead to syntax errors if the variable is unset or empty. Here’s an example:
# Declare a boolean variable without a value
is_valid=
# Use the boolean variable in an if statement
if [ $is_valid -eq 0 ]; then
echo 'Valid'
else
echo 'Invalid'
fi
# Output:
# bash: [: -eq: unary operator expected
In this example, we declare a variable is_valid
but don’t assign it a value. When we try to use is_valid
in the if
statement, Bash throws a syntax error because it’s trying to compare nothing ($is_valid
) to 0.
To avoid this error, you should quote your variable substitutions in test constructs, like this: if [ "$is_valid" -eq 0 ]
.
Best Practices and Optimization Tips
- Always initialize your boolean variables: This ensures that they have a known value and prevents syntax errors caused by unset or empty variables.
Use descriptive variable names: This makes your code more readable and maintainable.
Quote your variable substitutions in test constructs: This prevents syntax errors if the variable is unset or empty.
Comment your code: Especially when using boolean variables, it’s helpful to comment your code to explain what each variable represents and how it’s used in the flow of your script.
Understanding Bash Boolean Variables
To truly master the use of boolean variables in Bash, it’s essential to understand what they are and how they work at a fundamental level.
A boolean variable in Bash is a variable that can hold one of two values – 0 or 1. In the context of Bash, 0 represents true, and 1 represents false. This is a convention that comes from the Unix world, where a program that exits with 0 is considered to have completed successfully (true), and a non-zero exit code indicates an error (false).
Here’s an example to illustrate this concept:
# Declare a boolean variable
is_connected=0
# Use the boolean variable in an if statement
if [ $is_connected -eq 0 ]; then
echo 'Connected'
else
echo 'Not connected'
fi
# Output:
# 'Connected'
In this example, we declare a variable is_connected
and assign it a value of 0 (true). We then use a conditional if
statement to check the value of is_connected
. If is_connected
equals 0, the script prints ‘Connected’. Otherwise, it prints ‘Not connected’. Since is_connected
is 0, our output is ‘Connected’.
The Importance of Boolean Variables in Bash
Boolean variables play a crucial role in controlling the flow of a script. They allow your script to make decisions based on the truthiness of certain conditions. For example, you can use a boolean variable to check if a file exists before trying to access it, or to verify that a network connection is active before trying to send data.
In essence, boolean variables provide a way for your script to make decisions and react to different situations, making your scripts more dynamic and robust.
Expanding the Scope: Boolean Variables in Larger Bash Projects
As you become more comfortable with boolean variables, you’ll find that they are not just useful for small scripts but are also a fundamental part of larger Bash projects. The ability to control the flow of your scripts using boolean logic is a powerful tool that can help you write more efficient and effective code.
Incorporating Boolean Variables in Complex Scripts
Consider a scenario where you’re developing a script to automate system updates on a Linux server. In this case, boolean variables can be used to check whether the system is currently running any critical services that shouldn’t be interrupted, or whether there’s enough disk space to download and install updates. This type of conditional logic is crucial in ensuring the smooth operation of your scripts and the systems they manage.
Related Topics to Explore
Alongside boolean variables, there are several related topics that you might find interesting:
- Conditional Statements in Bash: If you’re using boolean variables, you’re likely using them within conditional statements. Understanding the various types of conditional statements in Bash can help you write more complex and dynamic scripts.
Looping Constructs in Bash: Loops are another area where boolean variables come in handy. Whether it’s a
for
loop, awhile
loop, or anuntil
loop, boolean variables can control the flow and repetition of your code.
Further Resources for Mastering Bash Boolean Variables
If you’re interested in diving deeper into the world of Bash scripting and boolean variables, here are some resources that you might find useful:
- Advanced Bash-Scripting Guide: This comprehensive guide covers all aspects of Bash scripting, including an in-depth section on variables.
Bash Guide for Beginners: This guide is perfect for those just starting out with Bash. It provides a solid foundation that you can build upon.
Boolean Bash Variables Guide: An in-depth guide by Cyberciti on declaring and using boolean variables in scripting.
Wrapping Up: Mastering Boolean Variables in Bash
In this comprehensive guide, we’ve unraveled the intricacies of using boolean variables in Bash, a fundamental concept in scripting that can control the flow of your scripts.
We embarked on this journey by understanding the basics of boolean variables and how to declare and use them in Bash. We then delved into more advanced techniques, using boolean variables in more complex constructs like conditional statements and loops. We also explored alternative approaches such as using string comparison instead of boolean variables, expanding our toolkit for Bash scripting.
Along the way, we addressed common issues that you might encounter when using boolean variables in Bash, providing solutions and best practices to avoid potential pitfalls. We also looked at how boolean variables fit into the larger picture of Bash scripting, discussing their role in larger Bash projects and related topics to explore further.
Method | Pros | Cons |
---|---|---|
Boolean Variables | Simple, Control over script flow | Can be confusing, Limited scope |
String Comparison | Flexibility, Readability | Can be slower, More error-prone |
Whether you’re just starting out with Bash or you’re looking to level up your scripting skills, we hope this guide has shed light on the use of boolean variables in Bash and equipped you with the knowledge to use them effectively.
The mastery of boolean variables opens up a new level of control in your Bash scripts, allowing you to create more dynamic, efficient, and robust scripts. With this guide, you’re well on your way to becoming a Bash scripting pro. Happy coding!