Java Color: Usage Guide for Java.awt.Color Class
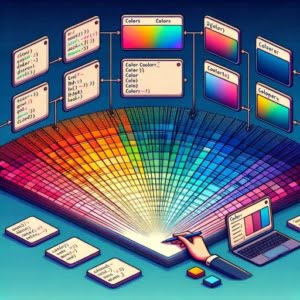
Are you finding it challenging to add a splash of color to your Java applications? You’re not alone. Many developers find themselves puzzled when it comes to handling colors in Java, but we’re here to help.
Think of the AWT Color class in Java as an artist’s palette – allowing you to paint your applications in any color you can imagine. This class provides a versatile and handy tool for various tasks.
In this guide, we’ll walk you through the process of using the AWT Color class in Java, from creating your own custom colors to using predefined ones. We’ll cover everything from the basics of the Color class to more advanced techniques, as well as alternative approaches.
Let’s get started and start mastering the Color class in Java!
TL;DR: How Do I Use the Color Class in Java?
To use the AWT Color class in Java, you first need to import it from the java.awt package, with the syntax
import java.awt.Color;
. You can then create a new Color object using one of the constructors provided by the class.
Here’s a simple example:
import java.awt.Color;
Color myColor = new Color(0, 255, 0);
In this example, we create a new Color object named ‘myColor’. The parameters 0, 255, 0 represent the RGB values for the color green.
This is a basic way to use the Color class in Java, but there’s much more to learn about creating and manipulating colors. Continue reading for more detailed information and examples.
Table of Contents
- Understanding the Color Class in Java
- Drawing Colored Shapes with Graphics Class
- Leveraging Predefined Colors in Java
- Exploring Alternative Color Handling in Java
- Weighing the Pros and Cons
- Addressing Common Issues with Java’s Color Class
- Delving into the RGB Color Model
- Unleashing the Power of Java Color Class
- Wrapping Up: Mastering the Color Class in Java
Understanding the Color Class in Java
The Color class in Java is a part of the java.awt package. It encapsulates colors in the default sRGB color space or colors in arbitrary color spaces identified by a ColorSpace. Every color has an implicit alpha value of 1.0 or an explicit one provided in the constructor. The alpha value defines the transparency of a color and can be represented by a float value in the range 0.0 – 1.0 or 0 – 255. An alpha value of 1.0 or 255 means that the color is completely opaque and an alpha value of 0 or 0.0 means that the color is completely transparent.
Creating a New Color Object
The Color class has several constructors. The most commonly used constructor accepts three parameters: red, green, and blue. Each of these parameters is an integer that can range from 0 to 255.
Here’s how you can create a new Color object:
import java.awt.Color;
Color myColor = new Color(255, 0, 0);
// Output:
// This creates a new Color object that represents the color red.
In this example, we import the Color class from the java.awt package and create a new Color object named ‘myColor’. The parameters 255, 0, 0 represent the RGB values for the color red.
Setting the Background Color of a JFrame
Now, let’s see how you can use the Color object to set the background color of a JFrame:
import javax.swing.JFrame;
import java.awt.Color;
public class Main {
public static void main(String[] args) {
JFrame frame = new JFrame();
frame.setSize(300, 200);
frame.getContentPane().setBackground(new Color(255, 0, 0));
frame.setVisible(true);
}
}
// Output:
// This will display a JFrame with a red background.
In this example, we create a JFrame and set its background color to red using the setBackground method and the Color object.
Understanding the RGB Color Model
The RGB (Red, Green, Blue) color model is a standard model used in digital graphics. In this model, each color is represented as a combination of the three primary colors: red, green, and blue. The intensity of each of these colors can be varied from 0 to 255, allowing for more than 16 million different colors (256x256x256).
In the Color class, the RGB color model is used to create custom colors. For instance, the color red is represented as (255, 0, 0), green as (0, 255, 0), and blue as (0, 0, 255).
Drawing Colored Shapes with Graphics Class
The Color class in Java isn’t just for setting background colors—it can also be used in conjunction with the Graphics class to draw colored shapes. Let’s see an example of how to draw a colored rectangle on a JPanel.
import javax.swing.JFrame;
import javax.swing.JPanel;
import java.awt.Color;
import java.awt.Graphics;
public class Main {
public static void main(String[] args) {
JFrame frame = new JFrame();
frame.setSize(300, 200);
frame.add(new JPanel() {
public void paintComponent(Graphics g) {
super.paintComponent(g);
g.setColor(new Color(255, 0, 0));
g.fillRect(50, 50, 100, 100);
}
});
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
}
}
// Output:
// This will display a JFrame with a red rectangle drawn on it.
In this example, we create a JFrame and add a JPanel to it. Inside the JPanel, we override the paintComponent
method. In this method, we first set the color of the Graphics object to red using the setColor
method and the Color object. Then, we draw a filled rectangle using the fillRect
method. The parameters for fillRect
are the x and y coordinates of the upper left corner of the rectangle, and the width and height of the rectangle.
Leveraging Predefined Colors in Java
The Color class in Java comes with a set of predefined colors that you can use directly without having to remember their RGB values. These are static final constants that represent some of the most commonly used colors.
Here’s how you can use a predefined color:
import java.awt.Color;
Color c = Color.RED;
// Output:
// Creates a new Color object that represents the color red.
In this example, we create a new Color object named ‘c’ and set it to the predefined color red. There are many predefined colors available, including but not limited to RED, BLUE, GREEN, YELLOW, PINK, CYAN, MAGENTA, ORANGE, GRAY, and BLACK.
Exploring Alternative Color Handling in Java
While the Color class is a versatile tool for color manipulation in Java, there are alternative approaches that can provide more specific functionalities or cater to different use cases.
SystemColor Class in Java
One such alternative is the SystemColor
class. This class extends the Color
class and provides system-dependent color values. These colors reflect the colors of the native system’s user interface.
Here’s a code example showing how to use the SystemColor
class:
import java.awt.SystemColor;
Color c = SystemColor.desktop;
// Output:
// Creates a new Color object that represents the color of the desktop.
In this example, we create a new Color object named ‘c’ and set it to the color of the desktop. The SystemColor
class provides access to a number of system colors, including desktop
, activeCaption
, textHighlight
, and menu
among others.
Creating Custom Color Models
Another advanced technique involves creating custom color models. Java provides the ColorModel
and IndexColorModel
classes for this purpose. These classes allow you to create and manipulate custom color spaces, which can be useful in certain applications such as image processing.
Weighing the Pros and Cons
While these alternative approaches can be powerful, they also come with their own set of challenges. For instance, system colors can vary greatly between different systems, making it difficult to ensure a consistent look across all platforms. Custom color models, on the other hand, can be complex to implement and may be overkill for simple applications.
In conclusion, while the Color
class provides a straightforward and versatile way to handle colors in Java, alternative approaches such as the SystemColor
class and custom color models can provide additional flexibility when necessary.
Addressing Common Issues with Java’s Color Class
While the Color class in Java is quite straightforward to use, there are a few common issues that you might encounter. Let’s discuss some of these problems and how to solve them.
Incorrect RGB Values
One of the most common issues is the use of incorrect RGB values. Remember, each RGB value must be an integer between 0 and 255. If you try to use a value outside of this range, Java will throw an IllegalArgumentException
.
Here’s an example:
import java.awt.Color;
public class Main {
public static void main(String[] args) {
Color myColor = new Color(300, 0, 0);
}
}
// Output:
// Exception in thread "main" java.lang.IllegalArgumentException: Color parameter outside of expected range
In this example, we tried to create a new Color object with an RGB value of 300, which is outside of the valid range. As a result, Java throws an IllegalArgumentException
.
To solve this issue, always ensure that your RGB values are within the correct range.
Issues with Color Visibility
Another common issue is color visibility. If you choose colors that are too similar, it can be difficult to distinguish between different elements on the screen. This can be especially problematic for users with color vision deficiency.
To avoid this issue, always ensure that there is enough contrast between different colors in your application. There are many online tools available that can help you choose a color palette with sufficient contrast.
In conclusion, while the Color class in Java is a powerful tool, it’s important to use it correctly. By being aware of these common issues and knowing how to solve them, you can make your applications more robust and user-friendly.
Delving into the RGB Color Model
Before we can fully harness the power of the Color class in Java, it’s important to understand the RGB color model, which is the foundation of color handling in Java and many other programming languages.
What is the RGB Color Model?
The RGB color model is a standard model used in digital graphics. In this model, each color is represented as a combination of the three primary colors: red, green, and blue. The intensity of each of these colors can be varied from 0 to 255, allowing for more than 16 million different colors (256x256x256).
Here’s a simple example to illustrate this concept:
import java.awt.Color;
public class Main {
public static void main(String[] args) {
Color myColor = new Color(255, 0, 0);
System.out.println(myColor);
}
}
// Output:
// java.awt.Color[r=255,g=0,b=0]
In this example, we create a new Color object with the RGB values for red (255, 0, 0). When we print this color, we can see the RGB values that make up the color.
How Does the RGB Color Model Relate to the Color Class?
The Color class in Java uses the RGB color model to create custom colors. Each instance of the Color class represents a color in the default sRGB color space. The class provides several methods to manipulate colors, such as getting the RGB values, adjusting the brightness, and mixing colors.
By understanding the RGB color model, you can create and manipulate colors more effectively using the Color class in Java.
Unleashing the Power of Java Color Class
The Color class in Java is not limited to just setting colors and drawing shapes. It can be used in more complex applications, such as creating gradients or working with images.
Creating Gradients with the Color Class
One of the ways to use the Color class in Java is to create gradients. Gradients are a smooth transition between two or more colors. In Java, you can create gradients using the GradientPaint
class. This class uses instances of the Color class to define the start and end colors of the gradient.
Working with Images
Another advanced use of the Color class is in image processing. The BufferedImage
class in Java allows you to manipulate images at the pixel level. Each pixel in an image can be represented as an instance of the Color class, allowing you to read and modify the color of individual pixels.
Further Resources for Mastering Java Color
If you want to dive deeper into the world of colors in Java, here are some resources that you might find helpful:
- Essential Tips for Java Classes – Unlock the power of Java classes in your projects.
Handling Color in Java – Discover Java’s built-in color functionalities for vibrant graphical user interfaces.
Mastering Java Timer Usage – Explore Java’s timer for time-based operations in applications.
Oracle’s Java Tutorials provide a guide to working with colors in Java.
Java2s’ Color Tutorial provides many examples of how to use the Color class in Java.
Java AWT Color Class Guide by GeeksforGeeks details the Color class in Java’s Abstract Window Toolkit.
Remember, mastering the Color class in Java, like any other skill, requires practice. So, don’t be afraid to experiment and create your own colorful applications!
Wrapping Up: Mastering the Color Class in Java
In this comprehensive guide, we’ve ventured into the colorful world of Java’s Color class, exploring its capabilities and applications in depth.
We began with the basics, understanding how to create custom colors using the RGB color model and applying these colors to Java components. We then delved deeper, learning how to draw colored shapes using the Graphics class and leveraging predefined colors for ease of use.
From there, we ventured into more advanced territory, exploring alternative approaches like the SystemColor class and creating custom color models. We also tackled common challenges that you might face when working with the Color class, providing solutions and workarounds for each issue.
Approach | Flexibility | Complexity | Use Case |
---|---|---|---|
Color Class | High | Low-Moderate | General color manipulation |
SystemColor Class | Medium | Moderate | System-dependent color values |
Custom Color Models | High | High | Specific applications like image processing |
Whether you’re just starting out with the Color class in Java or looking to enhance your color manipulation skills, we hope this guide has been a useful resource. The Color class is a powerful tool in Java, allowing you to add a splash of color to your applications and make them more visually appealing.
With this knowledge at your disposal, you’re well-equipped to paint your Java applications in any color you can imagine. Happy coding!