How to Add Colors in Java: A Step-by-Step Guide
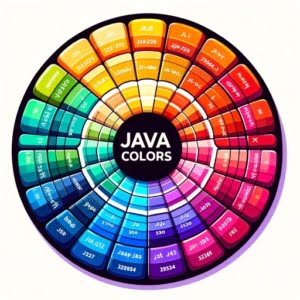
Are you finding it challenging to add a splash of color to your Java applications? You’re not alone. Many developers find themselves puzzled when it comes to handling colors in Java, but we’re here to help.
Think of Java’s color handling as an artist’s palette – allowing us to mix and manipulate colors, providing a versatile and handy tool for various tasks.
In this guide, we’ll walk you through the process of working with colors in Java, from their creation, manipulation, and usage. We’ll cover everything from the basics of the java.awt.Color class to more advanced techniques, such as setting colors in a GUI, and even discuss alternative approaches.
Let’s get started and start mastering colors in Java!
TL;DR: How Do I Use Colors in Java?
There are multiple ways to use Colors in Java. The simplest method is through the java.awt.Color class. You can create a color by specifying the Red, Green, and Blue (RGB) values. For example, to create a bright red color, you would do:
Color myColor = new Color(0, 255, 0);
This code creates a new Color object, myColor
, and assigns it the RGB values for bright green (0, 255, 0).
This is just a basic way to use colors in Java, but there’s much more to learn about creating and manipulating colors. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
Utilizing the java.awt.Color Class
Java provides the java.awt.Color class for creating and manipulating colors. This class is part of the Abstract Window Toolkit (AWT) and provides methods to work with RGB colors.
Creating Colors with the java.awt.Color Class
To create a color, we instantiate a new Color object and pass in the desired RGB values as parameters. Each parameter (Red, Green, and Blue) can range from 0 to 255. For instance, to create a bright red color, you would do:
Color myColor = new Color(255, 0, 0);
In this case, myColor
is a new Color object that represents bright red.
Advantages of the java.awt.Color Class
The java.awt.Color class simplifies working with colors in Java. It provides predefined color constants for quick access to common colors, and methods to manipulate colors according to your needs.
Potential Pitfalls
While the java.awt.Color class is handy, it’s crucial to remember that the RGB color model doesn’t cover all colors visible to the human eye. Also, different screens may render the same RGB values differently, leading to slight color variations.
Remember, mastering colors in Java is like learning to mix paints as an artist. It takes time and practice, but with the java.awt.Color class, you’re well on your way!
Advanced Color Handling in Java
As you grow more comfortable with using the java.awt.Color class, you can begin to explore more advanced uses of colors in Java. Let’s delve into some of these techniques.
Setting Colors in a GUI
Java’s Swing library allows you to create graphical user interfaces (GUIs) where you can apply your newfound color skills. For instance, you can change the background color of a JPanel:
JPanel panel = new JPanel();
panel.setBackground(new Color(255, 0, 0));
In this code, we create a JPanel and then use the setBackground()
method to change the panel’s background color to red.
Using Different Color Spaces
In addition to the RGB model, java.awt.Color supports other color spaces like HSB (Hue, Saturation, Brightness). You can create a color using HSB values using the Color.getHSBColor()
method:
Color hsbColor = Color.getHSBColor(0.9f, 1f, 1f);
This code creates a color with a hue of 0.9, full saturation, and full brightness.
Manipulating Colors
The java.awt.Color class provides methods to manipulate colors. For example, you can darken a color by decreasing its brightness:
Color darkColor = new Color(myColor.getRed(), myColor.getGreen(), myColor.getBlue()).darker();
This code creates a new color (darkColor
) that is a darker version of myColor
.
Mastering these advanced techniques will allow you to use colors more effectively in your Java applications.
Exploring Alternative Methods for Color Handling in Java
While the java.awt.Color class is a powerful tool for color manipulation in Java, there are additional methods that can offer more flexibility or functionality, particularly for more complex projects. One such method is the use of third-party libraries.
Leveraging Third-Party Libraries
Third-party libraries, such as Apache’s Commons Imaging library, offer a broad range of color handling capabilities that extend beyond the standard Java libraries. For example, the Imaging library provides support for a wider range of color spaces and more sophisticated color manipulation methods.
import org.apache.commons.imaging.color.ColorHsl;
import org.apache.commons.imaging.color.ColorRgb;
ColorRgb colorRgb = new ColorRgb(255, 0, 0);
ColorHsl colorHsl = ColorHsl.rgb2hsl(colorRgb);
// Output:
// ColorHsl { H: 0.0, S: 1.0, L: 0.5 }
In this code, we use the Commons Imaging library to convert an RGB color to HSL (Hue, Saturation, Lightness). The output shows the HSL values for the red color we defined.
Weighing the Pros and Cons
Third-party libraries can offer more advanced features, but they also come with their own set of considerations. On the one hand, they can provide more flexibility and functionality, which can be particularly useful for complex projects. On the other hand, they add an external dependency to your project and may have a steeper learning curve than using the built-in Java classes.
Ultimately, the best method for handling colors in Java will depend on your specific needs and the requirements of your project.
Troubleshooting Java Color Issues
Working with colors in Java can sometimes lead to unexpected results or issues. Let’s discuss some common problems and their solutions.
Handling Color Clashes in GUIs
When designing GUIs, color clashes can occur, making the interface hard to read or visually unappealing. To avoid this, we can use complementary colors or different shades of the same color.
JPanel panel = new JPanel();
panel.setBackground(new Color(255, 0, 0)); // Setting the background to red
JLabel label = new JLabel("Hello, World!");
label.setForeground(new Color(0, 0, 255)); // Setting the text color to blue
panel.add(label);
In this example, we’ve set the background color of a JPanel to red and the text color to blue, which are complementary colors. This contrast makes the text easier to read.
Dealing with Different Color Spaces
Different color spaces can cause colors to appear differently than expected. For example, a color defined in the RGB color space might look different when converted to the HSB color space. To avoid this, always ensure you’re working in the correct color space.
ColorRgb colorRgb = new ColorRgb(255, 0, 0);
ColorHsl colorHsl = ColorHsl.rgb2hsl(colorRgb);
// Output:
// ColorHsl { H: 0.0, S: 1.0, L: 0.5 }
This code converts an RGB color to HSL. The output shows that the red color in RGB translates to a hue of 0.0, full saturation, and half lightness in HSL.
Remember, handling colors in Java is an art as much as it is a science. It requires a good understanding of both the technical aspects and the visual aesthetics. But with practice and a bit of creativity, you can create visually stunning Java applications.
Understanding Java Colors: The Fundamentals
To effectively use colors in Java, it’s important to understand the fundamentals that underpin color handling in the language. This includes the java.awt.Color class, the concept of color spaces, and the RGB color model.
The java.awt.Color Class
The java.awt.Color class is a part of Java’s Abstract Window Toolkit (AWT) and is the primary tool for creating and manipulating colors in Java. It uses the RGB color model, where colors are defined using Red, Green, and Blue values.
Color myColor = new Color(255, 0, 0); // Creating a red color
In this code, we’re creating a new Color object, myColor
, and assigning it the RGB values for bright red.
Color Spaces and the RGB Model
A color space is a specific organization of colors, and the RGB color model is one such space. In RGB, colors are defined as a mix of Red, Green, and Blue light, with values ranging from 0 (no light) to 255 (maximum intensity).
Color greenColor = new Color(0, 255, 0); // Creating a green color
In this example, we’re creating a new Color object, greenColor
, and assigning it the RGB values for bright green.
Understanding these fundamentals is key to mastering color handling in Java. With this knowledge, you can create, manipulate, and apply colors with confidence in your Java applications.
Java Colors: Beyond the Basics
Mastering Java colors opens up a world of possibilities for developing diverse applications. Let’s explore some of these applications and related concepts you might want to delve into.
Color Handling in Game Development
In game development, color handling plays a crucial role in creating immersive and visually appealing environments. From defining the colors of game characters to setting the mood with background colors, understanding Java colors is a valuable skill for any game developer.
Data Visualization Tools
Data visualization tools often rely on colors to differentiate between data sets and make the data more understandable. Mastering Java colors can allow you to create more effective and visually appealing data visualizations.
Image Processing and GUI Design
In image processing and GUI design, colors are essential for tasks like filtering images, creating custom themes, and more. A solid understanding of Java colors can significantly enhance your ability to work in these areas.
Further Resources for Using Java Colors
To continue your journey in mastering Java colors, here are some resources that you might find helpful:
- Exploring Java Classes: Key Concepts – Learn Java classes basics quickly and efficiently.
Java Color Basics – Delve into Java’s color representation and manipulation techniques for graphic design.
Java Duration Basics – Master Java’s Duration class for representing time spans and durations accurately.
Oracle’s Java Tutorials cover a wide range of topics, including graphics and color handling.
Java2s’ Color Tutorial provides a detailed look at the Color class in JavaFX.
Java AWT Color Class Tutorial by GeeksforGeeks dives into the Color class in Java’s Abstract Window Toolkit.
Wrapping Up: Using Colors in Java
In this comprehensive guide, we’ve delved into the vibrant world of colors in Java, covering everything from the basics to advanced techniques.
We began with the fundamentals, learning how to create and manipulate colors using the java.awt.Color class. We then explored more advanced techniques, such as setting colors in a GUI and using different color spaces. Along the way, we tackled the common challenges you might encounter when working with colors in Java, offering solutions and workarounds for each issue.
We also examined alternative methods for handling colors in Java, including the use of third-party libraries, weighing the pros and cons of these alternatives. Here’s a quick comparison of the methods we’ve discussed:
Method | Flexibility | Complexity |
---|---|---|
java.awt.Color | Moderate | Low |
Third-party libraries | High | High |
Whether you’re just starting out with Java colors or you’re looking to level up your skills, we hope this guide has given you a deeper understanding of colors in Java and their potential applications.
With a solid grasp of Java colors, you’re well-equipped to bring a splash of color to your Java applications. Happy coding!