Java Duration Class Explained: Basics to Advanced
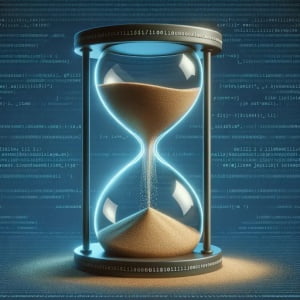
Are you finding it challenging to handle durations in Java? You’re not alone. Many developers find themselves grappling with this task, but there’s a tool in Java that can make this process a breeze.
Think of Java’s Duration class as a precise stopwatch – it allows you to measure time spans with great accuracy, providing a versatile and handy tool for various tasks.
This guide will walk you through the ins and outs of working with durations in Java, from basic usage to advanced techniques. We’ll cover everything from creating and manipulating Duration objects, to more complex uses and even troubleshooting common issues.
So, let’s dive in and start mastering Java Duration!
TL;DR: How Do I Work with Durations in Java?
Java provides the
Duration
class in thejava.time
package for working with durations. You can create a duration using theofHours
method like this:Duration duration = Duration.ofHours(5);
Here’s a simple example:
import java.time.Duration;
public class Main {
public static void main(String[] args) {
Duration duration = Duration.ofHours(5);
System.out.println(duration);
}
}
# Output:
# PT5H
In this example, we import the java.time.Duration
class and use the ofHours
method to create a duration of 2 hours. The System.out.println(duration);
line prints the duration, and the output is PT5H
, which stands for ‘Period of Time: 5 Hours’.
This is a basic way to use the
Duration
class in Java, but there’s much more to learn about creating and manipulating durations. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Understanding Java Duration: The Basics
- Manipulating and Comparing Durations in Java
- Exploring Alternatives: The Period Class
- Troubleshooting Java Duration: Common Issues and Solutions
- The Core of Java Time Measurement: The java.time Package
- Real-World Applications of Java Duration
- Further Resources for Mastering Java Duration
- Wrapping Up: Java Duration for Precise Time Measurement
Understanding Java Duration: The Basics
Java’s Duration
class is a versatile tool that allows you to measure time spans in the most precise way possible. It’s part of the java.time
package, which was introduced in Java 8 to address the shortcomings of the older date and time libraries.
Creating a Duration
To create a Duration
object, you use one of the static factory methods provided by the Duration
class. These methods include ofDays
, ofHours
, ofMinutes
, ofSeconds
, ofMillis
, and ofNanos
, each of which creates a duration of a specific length.
Here’s a simple example of creating a duration of 2 hours:
import java.time.Duration;
public class Main {
public static void main(String[] args) {
Duration duration = Duration.ofHours(2);
System.out.println(duration);
}
}
# Output:
# PT2H
In this code, we’re creating a Duration
object that represents a time span of 2 hours. The System.out.println(duration);
line then prints the duration, resulting in the output PT2H
.
Advantages of Using Duration
The Duration
class provides several advantages. It offers a high level of precision, allowing you to measure time spans down to the nanosecond. It’s also immutable, which means that once a Duration
object is created, it cannot be changed. This makes Duration
objects safe to use in a multithreaded environment.
Potential Pitfalls
While the Duration
class is powerful, it’s not without its potential pitfalls. One common issue is the loss of precision when converting a large duration to a smaller unit. For example, if you have a duration of 1 day and you convert it to nanoseconds, the result might not be exact due to the limitations of the long
data type used to store the duration.
Another potential pitfall is the use of the between
method to create a duration. This method measures the time difference between two temporal objects, but it can be misleading if those objects represent points in time that are subject to daylight saving time changes. In such cases, the between
method does not account for the extra or missing hour, which can lead to unexpected results.
Manipulating and Comparing Durations in Java
As you become more familiar with the Duration
class, you’ll find that it offers a lot more than just creating durations. You can also manipulate and compare durations, which can be incredibly useful in a variety of scenarios.
Manipulating Durations
The Duration
class provides several methods for manipulating durations, such as plus
, minus
, multipliedBy
, dividedBy
, and negated
. These methods return a new Duration
object, leaving the original duration unchanged.
Here’s an example of how you can manipulate durations:
import java.time.Duration;
public class Main {
public static void main(String[] args) {
Duration duration = Duration.ofHours(2);
Duration longerDuration = duration.plus(Duration.ofHours(1));
System.out.println(longerDuration);
}
}
# Output:
# PT3H
In this code, we first create a duration of 2 hours. We then use the plus
method to add 1 hour to this duration, creating a new duration of 3 hours. The output is PT3H
, which stands for ‘Period of Time: 3 Hours’.
Comparing Durations
The Duration
class also provides methods for comparing durations, such as isZero
, isNegative
, compareTo
, and equals
. These methods can be used to check if a duration is zero, negative, or equal to another duration, or to compare two durations.
Here’s an example of comparing durations:
import java.time.Duration;
public class Main {
public static void main(String[] args) {
Duration duration1 = Duration.ofHours(2);
Duration duration2 = Duration.ofHours(3);
System.out.println(duration1.compareTo(duration2));
}
}
# Output:
# -1
In this code, we create two durations: one of 2 hours and another of 3 hours. We then use the compareTo
method to compare these durations. The output is -1
, indicating that the first duration is less than the second duration.
Best Practices
When working with the Duration
class, it’s important to keep in mind a few best practices. First, always use the Duration
class when you need to measure a time span in terms of hours, minutes, seconds, or smaller units. For larger units like days, months, and years, consider using the Period
class instead.
Second, remember that the Duration
class does not account for daylight saving time changes. If you need to measure a time span that spans a daylight saving time change, consider using the ZonedDateTime
class instead.
Finally, always be aware of the potential loss of precision when converting a large duration to a smaller unit. To avoid this, try to keep your durations in the largest unit that makes sense for your application.
Exploring Alternatives: The Period Class
While the Duration
class is a powerful tool for measuring time spans in terms of hours, minutes, and seconds, it’s not the only tool available in Java. For larger time spans, especially those involving days, months, and years, you might find the Period
class to be more suitable.
Using the Period Class
The Period
class, like the Duration
class, is part of the java.time
package. It allows you to represent a quantity of time in terms of years, months, and days.
Here’s how you can create a period of 1 year, 2 months, and 3 days:
import java.time.Period;
public class Main {
public static void main(String[] args) {
Period period = Period.of(1, 2, 3);
System.out.println(period);
}
}
# Output:
# P1Y2M3D
In this code, we’re using the of
method of the Period
class to create a period. The output is P1Y2M3D
, which stands for ‘Period: 1 Year, 2 Months, 3 Days’.
Advantages and Disadvantages of the Period Class
The Period
class has several advantages over the Duration
class for certain use cases. It’s more human-readable, as it uses years, months, and days instead of hours, minutes, and seconds. It also takes into account leap years when calculating the number of days in a period.
However, the Period
class also has its disadvantages. It does not support time units smaller than a day, and it does not take into account daylight saving time changes. For these scenarios, the Duration
class might be a better choice.
Recommendations
When choosing between the Duration
and Period
classes, consider the nature of the time span you need to represent. If it’s a short time span or if you need a high level of precision, use the Duration
class. If it’s a long time span involving days, months, or years, use the Period
class.
Troubleshooting Java Duration: Common Issues and Solutions
While Duration
class is a powerful tool, like any other class, it has its quirks and potential issues. Understanding these will help you use it more effectively and avoid common pitfalls.
Precision Loss When Converting Units
One common issue when working with durations is the loss of precision when converting a large unit to a smaller unit. For example, converting a long duration in hours to milliseconds may not give an exact number due to the limitations of the long
data type.
Consider the following example:
import java.time.Duration;
public class Main {
public static void main(String[] args) {
Duration duration = Duration.ofHours(50000);
long milliseconds = duration.toMillis();
System.out.println(milliseconds);
}
}
# Output:
# 180000000000
In this code, we’re creating a large duration of 50,000 hours and converting it to milliseconds. The output might not be the exact number of milliseconds, due to the precision limitations of the long
data type.
To avoid this issue, try to keep your durations in the largest unit that makes sense for your application. If you need to convert durations, be aware of the potential for precision loss and plan accordingly.
Daylight Saving Time Considerations
Another issue to be aware of when working with durations is the impact of daylight saving time changes. The Duration
class does not account for these changes, which can lead to unexpected results.
If you need to measure a time span that spans a daylight saving time change, consider using the ZonedDateTime
class instead. This class does account for daylight saving time changes, providing a more accurate measure of the time span.
Final Tips
When working with the Duration
class, always remember that it’s a tool for measuring time spans in terms of hours, minutes, and seconds. For larger units like days, months, and years, consider using the Period
class instead. And always be aware of the potential issues and quirks, and plan your code accordingly.
The Core of Java Time Measurement: The java.time Package
The java.time
package is a core part of Java’s standard library. Introduced in Java 8, it provides classes for dates, times, instants, and durations, offering a comprehensive framework for dealing with time in your Java applications.
The Concept of Durations in Programming
In programming, a duration is a measure of time. It can be as short as a few nanoseconds or as long as several centuries. Durations are used in a wide variety of applications, from timing operations for performance testing to scheduling tasks in real-world time.
In Java, durations are represented by the Duration
class. This class provides methods for creating, manipulating, and comparing durations, making it a versatile tool for time measurement.
Here’s a simple example of creating a duration of 2 hours and 30 minutes:
import java.time.Duration;
public class Main {
public static void main(String[] args) {
Duration duration = Duration.ofHours(2).plusMinutes(30);
System.out.println(duration);
}
}
# Output:
# PT2H30M
In this code, we’re using the ofHours
method to create a duration of 2 hours, and then adding 30 minutes to it using the plusMinutes
method. The output is PT2H30M
, which stands for ‘Period of Time: 2 Hours 30 Minutes’.
The Importance of Precise Time Measurement
Precise time measurement is crucial in many applications. For example, in performance testing, you need to know exactly how long an operation takes to execute. In real-world scheduling tasks, you need to be able to measure time spans accurately to ensure that tasks occur at the correct times.
The Duration
class provides a high level of precision, allowing you to measure time spans down to the nanosecond. This makes it a valuable tool for any application that requires precise time measurement.
Real-World Applications of Java Duration
The Duration
class in Java is not just a theoretical concept; it has practical applications in the real world. It’s used in a wide range of scenarios, from creating timers and stopwatches to benchmarking code performance.
Timers and Stopwatches
One common use of the Duration
class is to create timers and stopwatches. For example, you might want to measure how long a user takes to complete a task in your application. By creating a Duration
at the start of the task and another at the end, you can calculate the time taken to complete the task with great precision.
Benchmarking
Another common use of the Duration
class is benchmarking, which involves measuring the performance of your code. By creating a Duration
before and after a piece of code, you can measure exactly how long that code takes to execute. This can be incredibly useful for identifying performance bottlenecks and optimizing your code.
Exploring Related Concepts
If you’re interested in further exploring time handling in Java, there are several related concepts that you might find useful. For example, the Instant
class represents a specific point in time, while the Period
class represents a quantity of time in terms of years, months, and days.
Further Resources for Mastering Java Duration
To deepen your understanding of Java Duration and related concepts, here are a few resources that you might find useful:
- Java Classes Explained: Basic to Advanced – Boost your Java skills with in-depth classes coverage.
Mastering JList Usage – Explore Java’s JList component for building interactive lists in graphical user interfaces.
Understanding Java Colors – Explore Java’s color handling capabilities for creating visually appealing user interfaces.
Oracle’s Official Java Documentation – The official documentation for the
Duration
class, and all of its methods.Java 8 Date and Time Tutorial provides an intro to the
java.time
package and theDuration
class.JavaCodeGeeks’ Java Duration Tutorial provides examples of using the
Duration
in thejava.time
package.
Wrapping Up: Java Duration for Precise Time Measurement
In this comprehensive guide, we’ve delved into the depths of the Duration
class in Java, a powerful tool for measuring time spans with great precision.
We began with the basics, learning how to create Duration
objects and understanding the advantages and potential pitfalls of using this class. We then explored more advanced use cases, such as manipulating and comparing durations, and discussed best practices to follow when using the Duration
class.
We also looked at alternative approaches to handling durations, introducing the Period
class for dealing with larger time spans. We discussed the advantages and disadvantages of these two classes, equipping you with the knowledge to choose the right tool for your specific needs.
Here’s a quick comparison of these methods:
Method | Precision | Units | Best for |
---|---|---|---|
Duration | Nanosecond | Hours, Minutes, Seconds | Short time spans, high precision |
Period | Day | Years, Months, Days | Long time spans, human-readable |
We tackled common issues you might encounter when working with durations, such as precision loss and daylight saving time considerations, and provided solutions to help you overcome these challenges. We also provided a deeper understanding of the java.time
package and its importance in Java programming.
Whether you’re just starting out with Java Duration
or you’re looking to level up your skills, we hope this guide has given you a deeper understanding of Duration
and its capabilities. With its balance of precision and versatility, Duration
is a powerful tool for time measurement in Java. Happy coding!