Python Visualizer: Seeing Your Code and Data
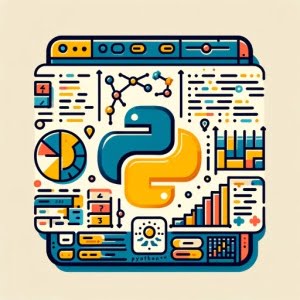
Are you finding it challenging to visualize your Python code or data? You’re not alone. Many developers find themselves in a similar situation, but there’s a tool that can bring clarity to your code and data.
Think of Python visualizers as a magnifying glass for your code and data – they bring the intricate details into focus, making it easier to understand and debug your programs.
In this guide, we’ll introduce you to Python visualizers, starting from the basics and gradually moving on to more advanced topics. We’ll cover everything from simple code visualization using tools like PyCharm, to data visualization using libraries like Matplotlib and Seaborn.
So, let’s dive in and start mastering Python visualizers!
TL;DR: What is a Python Visualizer?
A Python visualizer is a tool or library that helps you visualize your Python code execution or data. For code visualization, PyCharm is a popular choice. For data visualization, libraries like Matplotlib or Seaborn are commonly used.
Here’s a simple example of how you might use PyCharm for code visualization:
# Let's say we have the following Python code:
def hello_world():
print('Hello, world!')
hello_world()
# Output:
# 'Hello, world!'
In PyCharm, you can step through this code line by line, watching as the function hello_world()
is defined and then called, printing ‘Hello, world!’ to the console.
And here’s a basic example of data visualization with Matplotlib:
import matplotlib.pyplot as plt
# Let's say we have the following data:
data = [1, 2, 3, 4, 5]
plt.plot(data)
plt.show()
# Output:
# A line graph showing the numbers 1 through 5 on the y-axis, with the x-axis representing the index of each number in the list.
In this example, we import the Matplotlib library and use it to create a simple line graph of our data.
These are just the basics of Python visualizers, but there’s much more to learn. Continue reading for more detailed information and examples.
Table of Contents
Getting Started with Python Visualizer
Visualizing Code with PyCharm
PyCharm is a popular Integrated Development Environment (IDE) that offers excellent features for Python development, including code visualization. Let’s walk through a basic example of how to use PyCharm for code visualization.
Suppose we have a simple Python function that prints a greeting:
def greet(name):
print(f'Hello, {name}!')
greet('Alice')
# Output:
# 'Hello, Alice!'
In PyCharm, you can step through this code line by line, watching as the function greet()
is defined and then called, printing ‘Hello, Alice!’ to the console. This step-by-step visualization helps you understand the flow of your code and makes debugging easier.
Data Visualization with Matplotlib
Matplotlib is a powerful library for creating static, animated, and interactive visualizations in Python. Here’s a simple example of how you can use Matplotlib to visualize data.
import matplotlib.pyplot as plt
# Let's say we have the following data:
data = [1, 2, 3, 4, 5]
plt.plot(data)
plt.show()
# Output:
# A line graph showing the numbers 1 through 5 on the y-axis, with the x-axis representing the index of each number in the list.
In this example, we import the Matplotlib library and use it to create a simple line graph of our data. This visual representation of data can be incredibly useful in understanding patterns and trends in your data.
Advanced Python Visualizer Techniques
Advanced PyCharm Features
As you become more comfortable with PyCharm, you can start exploring its advanced features. For instance, PyCharm allows you to set breakpoints in your code, which lets you pause the execution of your program at specific points and inspect the current state.
Let’s look at a more complex example:
def factorial(n):
if n == 0:
return 1
else:
return n * factorial(n-1)
print(factorial(5))
# Output:
# 120
In this recursive function, you can set a breakpoint at the line return n * factorial(n-1)
. When you run the program in debug mode, PyCharm will pause at this line, allowing you to inspect the current value of n
and the output of factorial(n-1)
. This advanced use of PyCharm’s visualizer can be invaluable when debugging complex code.
Complex Plots with Matplotlib
Matplotlib is not just for simple line graphs. It’s a versatile library that can create a wide variety of plots, including histograms, scatter plots, and even 3D plots.
Here’s an example of a more complex plot using Matplotlib:
import matplotlib.pyplot as plt
import numpy as np
# Generate some data
x = np.random.randn(1000)
# Create a histogram
plt.hist(x, bins=30)
plt.title('Histogram')
plt.show()
# Output:
# A histogram showing the distribution of 1000 random numbers.
In this example, we generate 1000 random numbers using NumPy and create a histogram using Matplotlib. The histogram provides a visual representation of the distribution of our data, which can be very useful in data analysis.
Exploring Alternative Python Visualizers
Online Python Tutor for Code Visualization
While PyCharm is a powerful tool for code visualization, there are alternatives worth considering. One such tool is Online Python Tutor, a web-based tool that allows you to visualize Python code execution.
Let’s take our factorial function as an example:
def factorial(n):
if n == 0:
return 1
else:
return n * factorial(n-1)
print(factorial(5))
# Output:
# 120
If you paste this code into Online Python Tutor and click ‘Visualize Execution’, you’ll see a step-by-step visualization of the code, including the recursive calls to the factorial
function. This can be a great way to understand complex code, especially if you’re learning Python or teaching it to others.
Seaborn for Data Visualization
When it comes to data visualization, Matplotlib is not the only game in town. Seaborn is another powerful Python library based on Matplotlib. It provides a high-level interface for creating attractive graphics.
For instance, let’s create a histogram with Seaborn:
import seaborn as sns
import numpy as np
# Generate some data
x = np.random.randn(1000)
# Create a histogram
sns.histplot(x, bins=30, kde=True)
plt.show()
# Output:
# A histogram showing the distribution of 1000 random numbers, with a kernel density estimate (KDE) overlay.
In this example, not only do we create a histogram, but we also add a kernel density estimate (KDE) overlay with the kde=True
parameter. This provides a smoothed estimate of the data distribution, which can be very useful in data analysis.
While these alternative tools can offer additional features or different approaches, it’s important to choose the tool that best fits your needs and preferences. Whether you’re visualizing code with Online Python Tutor or PyCharm, or visualizing data with Seaborn or Matplotlib, the key is to find a tool that helps you understand and communicate your code and data effectively.
Troubleshooting Python Visualizers
Common Issues and Solutions
As with any tool, you might encounter some issues while using Python visualizers. Here are some common problems and their solutions.
PyCharm Not Showing Visualizations
Sometimes, PyCharm might not display visualizations as expected. This could be due to a variety of reasons, such as incorrect settings or software bugs. A simple solution can be to update PyCharm to the latest version or check your debugger settings.
Matplotlib Not Displaying Plots
If Matplotlib is not displaying your plots, it could be because you forgot to call plt.show()
at the end of your plotting code. Here’s an example:
import matplotlib.pyplot as plt
data = [1, 2, 3, 4, 5]
plt.plot(data)
# Missing plt.show()
# Output:
# [<matplotlib.lines.Line2D object at 0x7f6c9c03a9d0>]
As you can see, the plot object is printed, but the plot is not displayed. To fix this, simply add plt.show()
at the end:
plt.plot(data)
plt.show()
# Output:
# A line graph showing the numbers 1 through 5 on the y-axis, with the x-axis representing the index of each number in the list.
Best Practices and Optimization
To get the most out of Python visualizers, here are some tips:
- Use the Right Tool for the Job: PyCharm is great for code visualization, but if you’re dealing with complex data, consider using a data visualization library like Matplotlib or Seaborn.
Leverage Advanced Features: Don’t limit yourself to the basic features. Explore advanced features like breakpoints in PyCharm or different types of plots in Matplotlib.
Keep Your Tools Updated: Software tools are constantly being improved. Make sure to keep your tools updated to benefit from the latest features and bug fixes.
The Power of Visualization in Python Programming
Visualizing your Python code execution or data isn’t just a neat trick—it’s a fundamental part of effective programming. Let’s delve deeper into why this is so important.
The Importance of Code Visualization
Code visualization provides a graphical representation of your program’s execution, which can make it easier to understand and debug your code. It’s especially useful when dealing with complex code structures, such as recursive functions or nested loops.
For instance, consider this recursive function to calculate the Fibonacci sequence:
def fibonacci(n):
if n <= 1:
return n
else:
return(fibonacci(n-1) + fibonacci(n-2))
print(fibonacci(9))
# Output:
# 34
Without visualization, understanding the flow of this function can be challenging. But with a tool like PyCharm or Online Python Tutor, you can visualize each recursive call, making the function’s execution much clearer.
Data Visualization: A Key to Data Analysis
When it comes to data analysis, visualization is key. It allows you to see patterns, trends, and outliers in your data that might not be apparent from raw numbers.
For example, let’s say you have a list of numbers, and you want to know their distribution. You could look at the raw numbers, but it would be hard to get a sense of their overall distribution. With a histogram, however, the distribution becomes clear:
import matplotlib.pyplot as plt
# Let's say we have the following data:
data = [1, 2, 2, 3, 3, 3, 4, 4, 4, 4, 5, 5, 5, 5, 5]
plt.hist(data, bins=5)
plt.show()
# Output:
# A histogram showing the distribution of the numbers, with the y-axis showing the frequency and the x-axis showing the numbers.
As you can see, data visualization with Python can provide powerful insights into your data, making it an essential tool in your data analysis toolkit.
Debugging with Python Visualizers
Debugging can be a challenging task, but Python visualizers can make it easier. By allowing you to step through your code line by line and inspect the current state of your program at each step, visualizers can help you quickly identify and fix bugs in your code.
In conclusion, whether you’re visualizing your code to better understand its flow, visualizing your data to uncover insights, or debugging your program, Python visualizers are an invaluable tool for every Python programmer.
Expanding Your Horizons with Python Visualizers
Python Visualizers in Large-Scale Projects
Python visualizers aren’t just for simple scripts or data analysis tasks—they can also be invaluable in larger projects. For instance, if you’re working on a complex web application with Django, you can use PyCharm’s visualizer to step through your views and models, helping you understand the flow of your application and debug any issues.
Similarly, if you’re working on a machine learning project with libraries like scikit-learn or TensorFlow, data visualization with Matplotlib or Seaborn can help you understand your data and the output of your models. For instance, you can create plots to visualize the distribution of your data, or use heatmaps to understand the correlation between different features.
Exploring Related Topics
Once you’re comfortable with Python visualizers, there are many related topics you can explore to further enhance your Python skills. For instance, you might want to delve deeper into machine learning with Python, where data visualization plays a crucial role in understanding your data and interpreting your models.
Web development with Python is another interesting area to explore. Tools like Django or Flask allow you to build powerful web applications, and Python visualizers can be a great help in understanding and debugging your code.
Further Resources for Mastering Python Visualizers
To continue your journey with Python visualizers, here are some resources that provide more in-depth information:
- IOFlood’s Matplotlib Article explains how to add labels, titles, and annotations to your plots.
Matplotlib Line Plots with plt.plot(): A Quick Guide – Dive into line plot creation, styling, and customization with Matplotlib.
Creating Python Scatter Plots with plt.scatter() – Dive into scatter plot customization and data representation techniques.
Real Python offers a wealth of Python tutorials and articles on data visualization and using IDEs like PyCharm.
Python.org – The official Python documentation includes some detailed tutorials that cover visualization techniques.
DataCamp provides online courses on data science and machine learning, including some courses on Python, Matplotlib, and Seaborn for data visualization in Python.
Wrapping Up: Mastering Python Visualizers
In this comprehensive guide, we’ve navigated through the realm of Python visualizers, tools that bring clarity to your code and data.
We began with the basics, introducing Python visualizers and their fundamental uses. We then moved on to more advanced topics, exploring how to use PyCharm for code visualization and Matplotlib for data visualization.
We also discussed alternative approaches, such as using Online Python Tutor for code visualization and Seaborn for data visualization.
Along the way, we addressed common issues and their solutions when using Python visualizers and provided some best practices and optimization tips.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
PyCharm | Robust, great for code visualization | May require some time to learn all features |
Matplotlib | Powerful, versatile for data visualization | Can be complex for beginners |
Online Python Tutor | Web-based, easy to use | Limited features compared to PyCharm |
Seaborn | High-level interface, attractive graphics | Less flexible than Matplotlib |
Whether you’re just starting out with Python visualizers or you’re looking to level up your skills, we hope this guide has given you a deeper understanding of Python visualizers and their capabilities.
With the power to visualize your code execution and data, Python visualizers are a game-changer in your programming journey. Happy coding!