‘Not’ Keyword in Python: Quick Reference
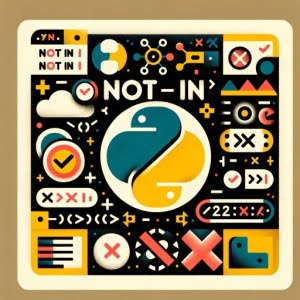
Are you finding the ‘not’ keyword in Python a bit confusing? You’re not alone. Like a traffic signal, ‘not’ can control the flow of your code, but its usage can sometimes be puzzling for beginners and even experienced developers.
Think of the ‘not’ keyword as a switch, flipping the truth value of any Python expression. It’s a fundamental part of Python’s logical operators, playing a crucial role in conditional statements and control flow.
In this guide, we will demystify the ‘not’ keyword in Python, covering everything from basic usage to advanced techniques. We’ll explore how ‘not’ works, delve into its applications, and even discuss common issues and their solutions.
Let’s get started!
TL;DR: How Do I Use the ‘not’ Keyword in Python?
The ‘not’ keyword in Python is a logical operator that inverses the truth value of the operand. If the operand is False, ‘not’ returns True, and vice versa.
Here’s a simple example:
x = False
print(not x)
# Output:
# True
In this example, we’ve assigned the boolean value False to the variable ‘x’. When we print ‘not x’, Python returns True because ‘not’ inverts the False value of ‘x’.
This is just a basic usage of the ‘not’ keyword in Python. There’s much more to learn about its applications and nuances. Continue reading for a more detailed exploration.
Table of Contents
- Demystifying the ‘not’ Keyword: Basic Usage in Python
- Advanced Use of ‘Not’ in Python: Logical Operators and Conditional Statements
- Alternative to ‘Not’ in Python: Exploring Other Methods
- Troubleshooting ‘Not’ in Python: Common Issues and Solutions
- Python Logical Operators: Understanding ‘Not’
- ‘Not’ in Python: Beyond the Basics
- Wrapping Up: Mastering the ‘Not’ Keyword in Python
Demystifying the ‘not’ Keyword: Basic Usage in Python
In its simplest form, the ‘not’ keyword in Python is used to reverse the truth value of the operand it precedes. If the operand is True, ‘not’ will return False, and if the operand is False, ‘not’ will return True. This is a fundamental aspect of Python’s logical operations.
Here is a basic example of using the ‘not’ keyword:
x = True
print(not x)
# Output:
# False
In this example, we’ve assigned the boolean value True to the variable ‘x’. When we use the ‘not’ keyword before ‘x’ in the print statement, Python returns False. This is because ‘not’ inverts the truth value of ‘x’.
The ‘not’ keyword is particularly useful when you want to check if a variable does not meet a certain condition. For instance, if you want to check if a variable ‘x’ is not equal to 10, you could use the ‘not’ keyword like so:
x = 5
print(x is not 10)
# Output:
# True
In this example, the ‘not’ keyword is used in conjunction with the ‘is’ keyword to check if ‘x’ is not 10. Since ‘x’ is 5, the statement ‘x is not 10’ returns True.
However, it’s important to be aware of potential pitfalls when using the ‘not’ keyword. One common issue is misunderstanding the order of operations. Python follows a specific order of operations, which can lead to unexpected results if not properly understood. We’ll delve into this in more detail in the ‘Troubleshooting and Considerations’ section.
Advanced Use of ‘Not’ in Python: Logical Operators and Conditional Statements
As you become more comfortable with Python, you’ll find that the ‘not’ keyword can be used in more complex ways, particularly with other logical operators (like ‘and’ and ‘or’) and in conditional statements.
‘Not’ with Other Logical Operators
In Python, ‘not’ can be used with other logical operators such as ‘and’ and ‘or’ to create more complex logical expressions. Here’s an example:
x = 10
y = 20
print(not(x < 5 and y > 15))
# Output:
# True
In this example, ‘x < 5 and y > 15’ is a logical expression that returns False because ‘x’ is not less than 5. However, when we apply ‘not’ to this expression, the result is inverted, and the print statement outputs True.
‘Not’ in Conditional Statements
The ‘not’ keyword is also commonly used in conditional statements. Here’s an example:
x = []
if not x:
print('The list is empty.')
# Output:
# The list is empty.
In this example, we’ve assigned an empty list to ‘x’. The ‘if not x’ statement checks if ‘x’ is empty. If ‘x’ is empty, it’s considered False in a boolean context. But ‘not’ inverts this to True, so the print statement is executed, and ‘The list is empty.’ is printed.
Understanding these advanced uses of the ‘not’ keyword can significantly enhance your Python programming skills, allowing you to write more complex and efficient code.
Alternative to ‘Not’ in Python: Exploring Other Methods
While the ‘not’ keyword is a powerful tool in Python, there are alternative ways to achieve similar results. Understanding these alternatives can provide more flexibility in your code and help you choose the most efficient method for your specific needs.
Using Comparison Operators
One alternative to using the ‘not’ keyword is to use comparison operators. For example, instead of using ‘not’ to check if a value is not equal to another, you can use the ‘!=’ operator.
Here’s an example:
x = 5
# Using 'not'
print(x is not 10)
# Using '!='
print(x != 10)
# Output:
# True
# True
In both cases, the output is True because ‘x’ is not equal to 10. However, using the ‘!=’ operator can be more straightforward and readable in some cases.
Using ‘len()’ Function for Empty Lists
When checking if a list is empty, you can use the ‘len()’ function instead of the ‘not’ keyword. Here’s an example:
x = []
# Using 'not'
if not x:
print('The list is empty.')
# Using 'len()'
if len(x) == 0:
print('The list is empty.')
# Output:
# The list is empty.
# The list is empty.
Both methods return the same result, but using ‘len()’ can make your code more explicit and easier to understand.
While these alternatives can be useful in certain situations, it’s important to remember that the ‘not’ keyword has its own advantages, such as simplicity and readability in many cases. The best method depends on your specific use case and coding style.
Troubleshooting ‘Not’ in Python: Common Issues and Solutions
While the ‘not’ keyword in Python is relatively straightforward, it’s not without its potential pitfalls. In this section, we’ll discuss some common issues you might encounter and how to address them.
Unexpected Results Due to Operator Precedence
One common issue arises from Python’s operator precedence, which determines the order in which operations are performed. ‘Not’ has a higher precedence than ‘and’ and ‘or’, which can lead to unexpected results.
Here’s an example:
x = True
y = False
print(not x or y)
# Output:
# False
You might expect this expression to return True because ‘y’ is False and ‘not x or y’ seems like it should be equivalent to ‘(not x) or y’. However, due to operator precedence, it’s actually equivalent to ‘not (x or y)’, which returns False.
To avoid this issue, you can use parentheses to explicitly specify the order of operations:
x = True
y = False
print((not x) or y)
# Output:
# False
In this case, ‘(not x) or y’ returns False, as expected.
Using ‘Not’ with Non-Boolean Values
Another potential pitfall is using the ‘not’ keyword with non-boolean values. In Python, non-boolean values can be evaluated in a boolean context to be True or False, a concept known as truthiness and falsiness.
Here’s an example:
x = ''
print(not x)
# Output:
# True
In this example, ‘x’ is an empty string, which is considered False in a boolean context. Therefore, ‘not x’ returns True.
Understanding these issues and how to address them can help you use the ‘not’ keyword more effectively and avoid common pitfalls.
Python Logical Operators: Understanding ‘Not’
To fully grasp the ‘not’ keyword in Python, it’s important to understand the concept of logical operators. Logical operators in Python are used to combine conditional statements. The three logical operators in Python are ‘and’, ‘or’, and ‘not’.
The ‘Not’ Operator
The ‘not’ operator in Python is a logical operator that returns the inverse of the truth value of the operand it precedes. If the operand is True, ‘not’ will return False, and if the operand is False, ‘not’ will return True.
x = True
print(not x)
# Output:
# False
In this example, the ‘not’ operator inverts the truth value of ‘x’, so ‘not x’ returns False.
Truthiness and Falsiness in Python
In Python, values are not just classified as True or False, but can also be evaluated as ‘truthy’ or ‘falsy’. This means that non-boolean values can be evaluated in a boolean context.
For example, in Python, an empty list, an empty string, zero, and None are all considered ‘falsy’ because they are equivalent to False in a boolean context. On the other hand, non-empty lists, non-empty strings, non-zero numbers, and most other objects are considered ‘truthy’ because they are equivalent to True in a boolean context.
x = []
if not x:
print('x is falsy')
# Output:
# x is falsy
In this example, ‘x’ is an empty list, which is considered ‘falsy’. Therefore, ‘not x’ returns True, and ‘x is falsy’ is printed.
Understanding the concept of truthiness and falsiness in Python is crucial for effectively using the ‘not’ keyword and other logical operators.
‘Not’ in Python: Beyond the Basics
The ‘not’ keyword in Python isn’t just for simple logical operations. Its applications extend to more complex Python programs, including data analysis and web development.
‘Not’ in Data Analysis
In data analysis, the ‘not’ keyword can be used to filter out specific data. For example, if you’re working with a dataset of employees and want to exclude those with a certain job title, you could use the ‘not’ keyword.
import pandas as pd
df = pd.read_csv('employees.csv')
filtered_df = df[df['Job Title'] != 'Manager']
print(filtered_df)
# Output:
# A DataFrame excluding rows where 'Job Title' is 'Manager'
In this example, df['Job Title'] != 'Manager'
is a boolean series that is True for rows where ‘Job Title’ is not ‘Manager’. This series is used to filter the DataFrame, excluding rows where ‘Job Title’ is ‘Manager’.
‘Not’ in Web Development
In web development, the ‘not’ keyword can be used in various ways, such as validating user input or controlling the flow of the program based on certain conditions.
username = input('Enter username: ')
if not username:
print('Username cannot be empty.')
# Output:
# 'Username cannot be empty.' if no username is entered
In this example, ‘not username’ checks if the username is an empty string. If it’s empty, it’s considered ‘falsy’, and ‘not username’ returns True, so the error message is printed.
Further Resources for Mastering ‘Not’ in Python
To delve deeper into the ‘not’ keyword and related topics in Python, check out the following resources:
- IOFlood’s Conditional If Statement in Python provides a comprehensive guide on the Python if statement and its role in conditional code execution.
Exploring if-else Conditional Statements – Learn the fundamental “if-else” construct and how it controls Python program flow.
XOR in Python: Practical Examples – Harnes the power of using XOR for binary manipulation and data encryption in Python.
Python’s Official Documentation on Logical Operators reviews Python’s logical operators and their usage.
Real Python’s Guide to ‘not’ Operator – A comprehensive guide exploring the usage of the ‘not’ operator in Python.
Control Flow in Python Tutorial – A tutorial by W3Schools focusing on control flow and conditional statements in Python.
Wrapping Up: Mastering the ‘Not’ Keyword in Python
In this comprehensive guide, we’ve delved into the ‘not’ keyword in Python, exploring its usage, common issues, and their solutions.
We started with the basics, learning how to use the ‘not’ keyword in simple logical operations. We then moved on to more advanced applications, such as using ‘not’ with other logical operators and in conditional statements. We also explored alternative ways to achieve similar results without using the ‘not’ keyword.
Along the way, we tackled common challenges that you might face when using the ‘not’ keyword, such as unexpected results due to operator precedence and using ‘not’ with non-boolean values. We provided solutions and workarounds for each issue, equipping you with the knowledge to use the ‘not’ keyword effectively.
Whether you’re a beginner just starting out with Python or an experienced developer looking to brush up your skills, we hope this guide has given you a deeper understanding of the ‘not’ keyword in Python and its applications. Happy coding!