Date Formatting Methods in Bash Scripting
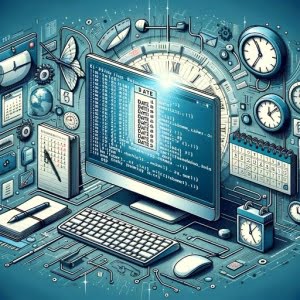
Are you finding it challenging to format dates in Bash? You’re not alone. Many developers find themselves puzzled when it comes to handling date formatting in Bash, but we’re here to help.
Think of Bash’s date formatting as a time machine – allowing us to display dates in any format you desire, providing a versatile and handy tool for various tasks.
In this guide, we’ll walk you through the process of formatting dates in Bash, from their creation, manipulation, and usage. We’ll cover everything from the basics of date formatting to more advanced techniques, as well as alternative approaches.
Let’s get started!
TL;DR: How Do I Format Dates in Bash?
The Bash
date
command, combined with a format string, is your key to manipulating and displaying dates. For instance,date '+%Y-%m-%d'
will output the current date in the ‘YYYY-MM-DD’ format.
Here’s a simple example:
date '+%Y-%m-%d'
# Output:
# 2022-03-14
In this example, we’ve used the date
command with a format string '+%Y-%m-%d'
. The +
sign is used to tell the date command that we want to format the output. The %Y
represents the year in four digits, %m
represents the month, and %d
represents the day. This command will output the current date in the ‘YYYY-MM-DD’ format.
This is just a basic way to format dates in Bash, but there’s much more to learn about manipulating and displaying dates in Bash. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Understanding the Basics of Bash Date Formatting
- Diving Deeper: Advanced Bash Date Formatting Techniques
- Exploring Alternatives: Other Commands for Date Formatting
- Navigating Challenges: Troubleshooting Bash Date Formatting
- Unix Time: The Heart of Bash Date Formatting
- Expanding Horizons: Bash Date Formatting in Larger Contexts
- Wrapping Up: Mastering Bash Date Formatting
Understanding the Basics of Bash Date Formatting
The date
command in Bash is a powerful tool for manipulating and displaying dates. It’s an essential part of any developer’s toolkit, particularly when dealing with scripts that require date or time operations.
Let’s start with a simple example of how to use the date
command to display the current date:
date
# Output:
# Mon Mar 14 12:34:56 UTC 2022
In this command, date
without any arguments displays the current date and time. The output includes the day of the week, month, date, time in hh:mm:ss format, time zone, and the year.
The real power of the date
command comes when you start using format strings. A format string allows you to control how the date is displayed. These are special codes that start with a %
symbol. For example, %Y
represents the year, %m
represents the month, and %d
represents the day.
Here’s how you can use a format string with the date
command:
date '+%Y-%m-%d %H:%M:%S'
# Output:
# 2022-03-14 12:34:56
In this command, we’ve used the date
command with a format string '+%Y-%m-%d %H:%M:%S'
. This command will output the current date in the ‘YYYY-MM-DD’ format, followed by the current time in ‘HH:MM:SS’ format.
The date
command is a versatile tool, but it’s important to remember that it relies on the system’s time settings. If your system’s time zone or clock is incorrect, the date
command will also display incorrect information. It’s also worth noting that the date
command only displays the current date and time by default. If you need to work with different dates or times, you’ll need to use additional options or commands, which we’ll cover in the next sections.
Diving Deeper: Advanced Bash Date Formatting Techniques
As you become more familiar with the date
command and its basic usage, you can explore more complex date formatting techniques. These can include using different format strings or manipulating the output with other Bash commands.
For example, you can use the date
command to display the date for a specific day in the past or future. This can be done using the -d
or --date
option. Here’s how you can display the date for 5 days ago:
date -d '5 days ago'
# Output:
# Wed Mar 9 12:34:56 UTC 2022
In this command, -d '5 days ago'
tells the date
command to calculate the date for 5 days in the past from the current date. The output is similar to the basic date
command, but the date is from 5 days ago.
But, what if you want to display the date in a different format? That’s where format strings come in handy. Let’s say you want to display the date for 5 days ago in ‘YYYY-MM-DD’ format. Here’s how you can do it:
date -d '5 days ago' '+%Y-%m-%d'
# Output:
# 2022-03-09
In this command, -d '5 days ago'
is used to calculate the date for 5 days in the past, and '+%Y-%m-%d'
is used to format the output date. The output is the date for 5 days ago in ‘YYYY-MM-DD’ format.
These are just a few examples of the advanced techniques you can use with the date
command. By combining different options and format strings, you can manipulate and display dates in a wide variety of ways.
Exploring Alternatives: Other Commands for Date Formatting
While the date
command is a powerful tool for formatting dates in Bash, it’s not the only one. There are other commands and functions that can accomplish similar tasks, providing alternative approaches to date formatting.
The strftime
Function
One such alternative is the strftime
function, a part of the awk
command. The strftime
function allows you to format dates and times based on a format string, much like the date
command.
Here’s an example of how you can use the strftime
function to display the current date and time:
awk 'BEGIN {print strftime("%Y-%m-%d %H:%M:%S")}'
# Output:
# 2022-03-14 12:34:56
In this command, awk 'BEGIN {print strftime("%Y-%m-%d %H:%M:%S")}'
uses the awk
command to print the current date and time in ‘YYYY-MM-DD HH:MM:SS’ format. The strftime
function formats the output based on the format string.
While the strftime
function provides similar functionality to the date
command, it does have some differences. For one, it’s a part of the awk
command, which means you need to be familiar with awk
to use it effectively. Additionally, it doesn’t support all the options and format strings that the date
command does, which can limit its versatility.
The printf
Command
Another alternative is the printf
command. While it’s primarily used for formatting and printing data, it can also be used for date formatting in combination with the date
command.
Here’s an example of how you can use the printf
command to display the current date:
printf "Today is %s\n" "$(date '+%Y-%m-%d')"
# Output:
# Today is 2022-03-14
In this command, printf "Today is %s\n" "$(date '+%Y-%m-%d')"
uses the printf
command to print a formatted string. The $(date '+%Y-%m-%d')
part is a command substitution that runs the date
command and replaces itself with the output.
The printf
command provides a different approach to date formatting. It allows you to include formatted dates in larger strings, which can be useful in scripts. However, it’s not as straightforward as the date
command, and it requires additional syntax to use effectively.
These are just a few of the alternative approaches to date formatting in Bash. Each method has its benefits and drawbacks, and the best one to use depends on your specific needs and circumstances.
While Bash’s date
command is a powerful tool, it’s not without its challenges. Here are some common errors or obstacles you might encounter when formatting dates in Bash, along with their solutions and best practices for optimization.
Incorrect Date Format
One common issue is getting the date format incorrect. This can happen if you use the wrong format string or if your system’s locale settings are different from what you expect.
For example, you might try to display the date in ‘MM/DD/YYYY’ format, but end up with an error:
date '+%m/%d/%Y'
# Output:
# bash: syntax error near unexpected token `'/''
In this case, the /
character is causing an issue because it’s interpreted as a special character by Bash. To resolve this, you can escape the /
character with a backslash (\
):
date '+%m\/%d\/%Y'
# Output:
# 03/14/2022
Timezone Issues
Another common issue is dealing with different timezones. The date
command displays the date and time based on the system’s timezone. If you need to display the date in a different timezone, you can use the TZ
environment variable.
For instance, to display the date in New York (Eastern Standard Time), you can do:
TZ='America/New_York' date
# Output:
# Mon Mar 14 08:34:56 EDT 2022
In this command, TZ='America/New_York' date
sets the TZ
environment variable to ‘America/New_York’ for the duration of the date
command. The output is the current date and time in New York.
Best Practices and Optimization
When using the date
command, it’s important to follow best practices for code readability and efficiency.
- Use clear and descriptive format strings. This makes your code easier to read and understand.
Handle errors and edge cases. Always check the return status of the
date
command and handle any errors that might occur.Use the
date
command sparingly in scripts. Thedate
command can be slow, especially in loops. If you need to display the date multiple times in a script, consider storing the date in a variable and reusing it.
By keeping these tips in mind, you can use the date
command effectively and avoid common pitfalls.
Unix Time: The Heart of Bash Date Formatting
To truly understand Bash date formatting, it’s important to delve into the underlying concept of Unix Time. Unix Time, also known as Epoch Time, is a system for tracking time that measures the number of seconds that have elapsed since the Unix Epoch – 00:00:00 Coordinated Universal Time (UTC), Thursday, 1 January 1970, not counting leap seconds.
Unix Time is the standard method for tracking time in most computing systems, including Bash. When you use the date
command in Bash without any arguments, it displays the current date and time based on Unix Time.
Here’s an example of how you can display the current Unix Time using the date
command:
date +%s
# Output:
# 1647360894
In this command, date +%s
uses the %s
format string to display the current Unix Time. The output is the number of seconds that have elapsed since the Unix Epoch.
Unix Time is a fundamental concept in Bash date formatting. By understanding Unix Time, you can better understand how the date
command works and how to use it effectively.
However, it’s also important to note that Unix Time is not without its limitations. For instance, it does not account for leap seconds, which are added to Coordinated Universal Time (UTC) to keep it within 0.9 seconds of mean solar time. Furthermore, Unix Time is set to ‘overflow’ (i.e., run out of numbers and start over from a negative number) in 2038, a problem known as the Year 2038 problem or Y2K38.
Despite these limitations, Unix Time is a fundamental part of Bash and many other computing systems. By understanding Unix Time, you can more effectively work with dates and times in Bash.
Expanding Horizons: Bash Date Formatting in Larger Contexts
As you grow more comfortable with Bash date formatting, you’ll start to see its potential in larger scripts or projects. The date
command and its various options can be a powerful tool in a developer’s arsenal, providing the ability to manipulate and display dates in a wide variety of ways.
For instance, you might use Bash date formatting in a script that generates log files, with each file named according to the date it was created. Or, you might use it in a script that schedules tasks or events based on the date.
In these situations, the date
command often works in tandem with other Bash commands or functions. For example, you might use the touch
command to create a new file with a name based on the current date:
filename="$(date '+%Y-%m-%d').log"
touch "$filename"
# Output:
# Creates a new file named '2022-03-14.log'
In this command, filename="$(date '+%Y-%m-%d').log"
uses command substitution to run the date
command and assign its output to the filename
variable. The touch "$filename"
command then creates a new file with the name stored in filename
.
This is just one example of how Bash date formatting can be applied in larger scripts or projects. The possibilities are virtually endless, limited only by your needs and imagination.
Further Resources for Bash Date Formatting Mastery
If you’re interested in learning more about Bash date formatting and related topics, here are some resources that offer more in-depth information:
- GNU Coreutils: Date Command: This is the official documentation for the
date
command from the GNU Coreutils manual. It provides a comprehensive overview of thedate
command and its various options and format strings. The Art of Command Line: This is a comprehensive guide to the command line for beginners and experienced users alike. It covers a wide range of topics, including date formatting and other Bash commands.
Bash Scripting Guide: This guide offers a thorough introduction to Bash scripting, including topics like date formatting, control structures, and more. It’s a great resource for anyone looking to write more complex scripts in Bash.
Wrapping Up: Mastering Bash Date Formatting
In this comprehensive guide, we’ve traversed the landscape of Bash date formatting, starting from the basics and moving towards more advanced techniques.
We began with an introduction to Bash date formatting, learning how to use the date
command and format strings to display dates in a variety of formats. We then delved into more advanced usage, exploring how to manipulate the output with other Bash commands and how to display dates for specific days in the past or future.
We also tackled common challenges that you might face when formatting dates in Bash, such as incorrect date formats and timezone issues, providing you with solutions and best practices for each issue. Additionally, we explored alternative approaches to date formatting in Bash, such as the strftime
function and the printf
command, giving you a broader perspective on date formatting in Bash.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
Bash date command | Versatile, supports many format strings | Relies on system’s time settings |
strftime function | Part of the awk command, suitable for complex scripts | Requires familiarity with awk , less versatile than date command |
printf command | Can include formatted dates in larger strings | Requires additional syntax, less straightforward than date command |
We hope this guide has provided you with a deeper understanding of Bash date formatting and its applications. Whether you’re a beginner just starting out with Bash, or an experienced developer looking to brush up on your skills, mastering Bash date formatting is an essential part of your toolkit.
With its versatility and wide range of options, the date
command in Bash is a powerful tool for manipulating and displaying dates. Now, you’re well equipped to tackle any date formatting challenges that come your way. Happy scripting!