If-Else in Python: Learn Program Control Syntax
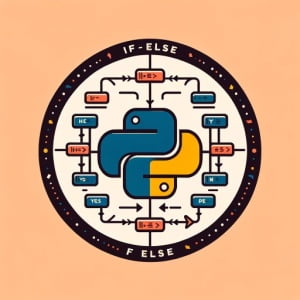
Are you finding it challenging to grasp if-else statements in Python? You’re not alone. Many beginners struggle with these essential control flow statements. Think of if-else statements as the traffic signals of your code, directing the flow and ensuring everything runs smoothly.
In this guide, we aim to demystify if-else statements in Python, taking you from the very basics to more advanced techniques. Whether you’re a novice programmer or looking to brush up on your Python skills, this comprehensive guide will serve as your roadmap to mastering if-else statements in Python.
TL;DR: How Do I Use If-Else Statements in Python?
In Python, if-else statements are used to control the flow of your code. They allow your program to make decisions based on certain conditions. Here’s a simple example:
x = 10
if x > 5:
print('x is greater than 5')
else:
print('x is not greater than 5')
# Output:
# 'x is greater than 5'
In this example, the program checks if the variable x
is greater than 5. Since x
is 10, which is indeed greater than 5, the program prints ‘x is greater than 5’. If x
was not greater than 5, the program would print ‘x is not greater than 5’.
This is just the tip of the iceberg when it comes to if-else statements in Python. Keep reading for a more detailed explanation and advanced usage scenarios.
Table of Contents
- Understanding Basic If-Else Statements in Python
- Delving Deeper: Nested If-Else and Logical Operators
- Exploring Alternatives: Ternary Operators and Switch-Case Statements
- Common Pitfalls and Solutions with If-Else Statements
- Understanding Control Flow and Conditional Programming
- If-Else Statements in Larger Projects
- Wrapping Up: Mastering If-Else in Python
Understanding Basic If-Else Statements in Python
Let’s start with the basics of if-else statements in Python. The if-else statement is a fundamental concept in Python, and it’s used to perform different computations or actions depending on whether a specific condition is true or not.
Here’s a simple example of an if-else statement:
age = 18
if age >= 18:
print('You are eligible to vote.')
else:
print('You are not eligible to vote.')
# Output:
# 'You are eligible to vote.'
In this example, the program checks if the variable age
is greater than or equal to 18. If the condition is true, it prints ‘You are eligible to vote.’ If the condition is false (i.e., age
is less than 18), it prints ‘You are not eligible to vote.’
The advantage of using if-else statements is that they make your code more flexible and dynamic. They allow your program to react differently based on the input or the situation, which is a fundamental aspect of programming.
However, there are a few potential pitfalls to watch out for. One common mistake is forgetting to use the colon (:
) at the end of the if
and else
lines. This will result in a syntax error. Another thing to keep in mind is that Python uses indentation to determine the scope of the code blocks. If your code is not properly indented, it will not run as expected.
Delving Deeper: Nested If-Else and Logical Operators
As you become more comfortable with if-else statements in Python, you can start to explore more complex uses. Two such advanced techniques are nested if-else statements and the use of logical operators.
Nested If-Else Statements
Nested if-else statements are essentially if-else statements within if-else statements. They allow you to check for multiple conditions and make your code more flexible. Here’s an example:
age = 20
if age >= 18:
if age <= 35:
print('You are eligible to vote and also in the youth demographic.')
else:
print('You are eligible to vote but not in the youth demographic.')
else:
print('You are not eligible to vote.')
# Output:
# 'You are eligible to vote and also in the youth demographic.'
In this example, the program first checks if the variable age
is greater than or equal to 18. If this condition is true, it enters another if-else statement to check if age
is less than or equal to 35. The nested if-else statement allows the program to give a more specific response based on the age.
Logical Operators
Logical operators like and
, or
, and not
can be used in conjunction with if-else statements to check multiple conditions at once. Here’s an example using the and
operator:
age = 20
citizenship = 'USA'
if age >= 18 and citizenship == 'USA':
print('You are eligible to vote in the USA.')
else:
print('You are not eligible to vote in the USA.')
# Output:
# 'You are eligible to vote in the USA.'
In this example, the program checks if the variable age
is greater than or equal to 18 and if the variable citizenship
equals ‘USA’. Both conditions must be true for the program to print ‘You are eligible to vote in the USA.’ If either condition is false, it prints ‘You are not eligible to vote in the USA.’
Exploring Alternatives: Ternary Operators and Switch-Case Statements
While if-else statements are a fundamental part of Python, there are alternative methods to control your code’s flow. Two such advanced techniques are ternary operators and switch-case statements using dictionaries.
Ternary Operators
A ternary operator allows you to condense an if-else statement into a single line of code. It’s a more concise, albeit less readable, way of writing an if-else statement. Here’s an example:
age = 20
message = 'Eligible to vote.' if age >= 18 else 'Not eligible to vote.'
print(message)
# Output:
# 'Eligible to vote.'
In this example, the program uses a ternary operator to determine the value of the message
variable. If age
is greater than or equal to 18, message
is set to ‘Eligible to vote.’ If age
is less than 18, message
is set to ‘Not eligible to vote.’
Switch-Case Statements Using Dictionaries
Python does not have a built-in switch-case construct like some other programming languages. However, you can achieve similar functionality using dictionaries. Here’s an example:
def switch_case(value):
return {
1: 'One',
2: 'Two',
3: 'Three'
}.get(value, 'Unknown')
print(switch_case(2))
# Output:
# 'Two'
In this example, the program uses a dictionary to map values (1, 2, and 3) to strings (‘One’, ‘Two’, ‘Three’). The get
method is used to retrieve the string associated with the provided value. If the value is not in the dictionary, it returns ‘Unknown’.
Both ternary operators and switch-case statements using dictionaries can make your code more concise. However, they can also make your code harder to read, especially for beginners. Therefore, it’s important to use them judiciously and always consider readability when writing your code.
Common Pitfalls and Solutions with If-Else Statements
While if-else statements are a powerful tool in Python, they can also lead to some common issues, particularly for beginners. Let’s explore some of these potential pitfalls and how to solve them.
Indentation Errors
Python uses indentation to determine the scope of code blocks. If your code is not properly indented, you may encounter an IndentationError
. Here’s an example:
age = 20
if age >= 18:
print('You are eligible to vote.')
# Output:
# IndentationError: expected an indented block
In this example, the print
statement is not indented, so Python raises an IndentationError
. To fix this, you need to indent the print
statement:
age = 20
if age >= 18:
print('You are eligible to vote.')
# Output:
# 'You are eligible to vote.'
Logical Errors
Logical errors occur when your code runs without any syntax errors, but it doesn’t produce the expected output. These can be tricky to debug because the problem is with the program’s logic, not its syntax. Here’s an example:
age = 17
if age > 18:
print('You are eligible to vote.')
else:
print('You are not eligible to vote.')
# Output:
# 'You are not eligible to vote.'
In this example, the program incorrectly checks if age
is greater than 18, instead of greater than or equal to 18. As a result, it prints ‘You are not eligible to vote.’ even though 18-year-olds are eligible to vote. To fix this, you need to change the condition to age >= 18
.
Understanding these common issues and how to resolve them can help you write more effective if-else statements in Python and debug your code more efficiently.
Understanding Control Flow and Conditional Programming
Before we delve deeper into if-else statements, it’s crucial to understand the concept of control flow and conditional programming in Python.
Control flow refers to the order in which the program’s code executes. The flow of control is determined by conditional statements (like if-else), loops, and function calls. These structures decide when and how specific blocks of code are executed based on certain conditions.
In Python, if-else statements are a key part of this control flow. They allow your program to respond differently based on whether a specific condition is met.
Here’s a simple example:
x = 5
if x > 0:
print('x is positive')
else:
print('x is not positive')
# Output:
# 'x is positive'
In this example, the program checks if x
is greater than 0. If the condition is true, it prints ‘x is positive’. If the condition is false, it prints ‘x is not positive’. This decision-making is the essence of control flow and conditional programming.
The Importance of Indentation in Python
Python uses indentation to define the scope of code blocks. This is different from many other programming languages, which often use brackets for this purpose. In Python, all the code that should be executed within the if block or else block needs to be indented by the same amount.
Here’s an example:
x = 5
if x > 0:
print('x is positive')
print('This is also part of the if block')
else:
print('x is not positive')
print('This is outside the if-else block')
# Output:
# 'x is positive'
# 'This is also part of the if block'
# 'This is outside the if-else block'
In this example, both print statements inside the if block are indented by the same amount. The final print statement, which is not indented, is outside the if-else block and is always executed, regardless of the condition.
Proper indentation is crucial in Python. If your code is not correctly indented, Python will raise an IndentationError
and your program will not run. This emphasis on indentation contributes to Python’s readability and clarity.
If-Else Statements in Larger Projects
If-else statements aren’t just for simple scripts; they also play a crucial role in larger projects. They are the building blocks that allow your program to make decisions and react to different scenarios.
The Power of Loops and Functions
Beyond if-else statements, loops and functions are other important concepts in Python that can significantly enhance your code’s functionality. Loops allow your program to perform repetitive tasks efficiently, while functions enable you to encapsulate a piece of code and reuse it throughout your program.
Here’s an example of how you might use a loop and a function with an if-else statement in a larger script:
def check_eligibility(age):
if age >= 18:
return 'Eligible to vote.'
else:
return 'Not eligible to vote.'
ages = [16, 20, 22, 15, 30]
for age in ages:
message = check_eligibility(age)
print(f'Age {age}: {message}')
# Output:
# 'Age 16: Not eligible to vote.'
# 'Age 20: Eligible to vote.'
# 'Age 22: Eligible to vote.'
# 'Age 15: Not eligible to vote.'
# 'Age 30: Eligible to vote.'
In this example, the program defines a function check_eligibility
that uses an if-else statement to check if a given age is eligible to vote. It then uses a for loop to check the eligibility of each age in the ages
list.
Further Resources for Mastering Python Control Flow
For those looking to delve deeper into Python control flow, here are some excellent resources:
- The Ultimate Resource for Python If Statement – Complete guide to nested if statements and intricate decision-making processes.
Python’s “not” Operator – Master advanced conditionals by using “not” for effective membership testing in Python.
Python’s Ternary Operator – Streamline conditional statements with the ternary operator in Python.
The Official Python Tutorial – This Python document provides a thorough explanation of all control flow statements.
Conditional Logic and Control Flow (Overview) – This guide by Real Python covers all aspects of control flow in Python.
Embrace these materials and continue to hone your skills with Python conditionals.
Wrapping Up: Mastering If-Else in Python
In this guide, we’ve taken a deep dive into if-else statements in Python, starting with the basics and progressing to more advanced techniques.
We’ve seen how if-else statements, akin to the traffic signals of your code, control the flow of your program based on specific conditions.
We’ve explored how to use simple if-else statements and discussed common pitfalls such as forgetting the colon :
at the end of the if
and else
lines, and the importance of correct indentation. We’ve also delved into more advanced uses like nested if-else statements and the use of logical operators and
, or
, and not
.
For those looking for alternative methods to control the flow of code, we’ve introduced ternary operators and switch-case statements using dictionaries. These techniques can make your code more concise, but they may also make it harder to read for beginners, so use them judiciously.
Finally, we’ve discussed the relevance of if-else statements in larger scripts or projects and suggested related concepts like loops and functions. We’ve also provided further resources for those looking to delve deeper into Python control flow.
Remember, mastering if else python
statements is a fundamental part of becoming proficient in Python. Keep practicing, and soon you’ll be able to use these control flow statements with ease and confidence.