Python Ternary Operator | Usage Guide with Examples
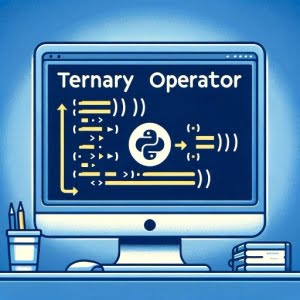
Struggling with Python’s ternary operator? Like a traffic light, the ternary operator can help control the flow of your code.
It’s a powerful tool that, when used correctly, can make your code more concise and easier to read. But, it can also be a bit tricky to master, especially if you’re new to Python or programming in general.
In this guide, we’ll demystify the ternary operator in Python. We’ll start with the basics, explaining what it is and how it works. Then, we’ll move on to more advanced topics, like how to use it effectively in your code and what pitfalls to avoid.
By the end of this article, you’ll have a solid understanding of the Python ternary operator and how to use it to write cleaner, more efficient code.
TL;DR: How Do I Use the Ternary Operator in Python?
The ternary operator in Python is a one-line shorthand for an if-else statement. It allows you to quickly test a condition and return a value based on whether that condition is true or false.
Here’s a simple example:
x = 10
print('Even' if x % 2 == 0 else 'Odd')
# Output:
# Even
In this example, the condition x % 2 == 0
checks if the number x
is even. If it is, the string ‘Even’ is printed. If not, the string ‘Odd’ is printed. The ternary operator allows us to do this in a single, concise line of code.
Keep reading for a more detailed explanation of how the ternary operator works in Python, as well as advanced usage scenarios and best practices.
Table of Contents
- Unraveling the Python Ternary Operator
- Exploring Nested Ternary Operations in Python
- Alternative Ways to Implement Conditional Logic in Python
- Troubleshooting Common Issues with the Python Ternary Operator
- Understanding Operators in Python
- The Ternary Operator in Real-World Applications
- Exploring Further
- Recap: Mastering the Python Ternary Operator
Unraveling the Python Ternary Operator
The ternary operator in Python is a compact way of writing an if-else statement. It’s used when you want to return two different values based on the truth value of a condition. The syntax is:
value_if_true if condition else value_if_false
Let’s take a look at a simple example:
x = 7
message = 'Even' if x % 2 == 0 else 'Odd'
print(message)
# Output:
# Odd
In this example, the condition x % 2 == 0
checks if x
is even. If it is, ‘Even’ is assigned to the variable message
. If not, ‘Odd’ is assigned. Thus, the print statement outputs ‘Odd’ as x
is 7, which is an odd number.
Advantages and Pitfalls of the Ternary Operator
The ternary operator can make your code more concise and easier to read when used appropriately. It’s great for simple, quick decisions in your code. However, it can become difficult to read and understand when overused or used for complex conditions. It’s best to use the ternary operator sparingly and for simple, clear conditions.
Exploring Nested Ternary Operations in Python
As you become more comfortable with the ternary operator, you might find yourself wondering if you can nest them. The answer is yes, you can, but with caution. Nested ternary operations can make your code compact, but they can also make it harder to read if overused.
Here’s an example of a nested ternary operation:
x = 15
message = 'Divisible by 5' if x % 5 == 0 else 'Even' if x % 2 == 0 else 'Odd'
print(message)
# Output:
# Divisible by 5
In this example, we first check if x
is divisible by 5. If it is, we print ‘Divisible by 5’. If it’s not, we move on to the next condition, checking if x
is even. If it is, we print ‘Even’. If it’s not even (and not divisible by 5), we print ‘Odd’.
Readability Concerns and Best Practices
While nested ternary operations can be useful, they can also make your code more complex and harder to read. As a best practice, avoid nesting ternary operations more than once. If your code requires more complex conditional logic, consider using traditional if-else statements for clarity. Remember, the goal of using the ternary operator should be to make your code more readable and efficient, not less.
Alternative Ways to Implement Conditional Logic in Python
While the ternary operator is a powerful tool, Python offers other ways to implement conditional logic. Understanding these alternatives can help you write code that’s easier to read and maintain, especially for complex conditions.
Traditional If-Else Statements
The most straightforward alternative to the ternary operator is a traditional if-else statement. Here’s how you’d write the earlier example using an if-else statement:
x = 15
if x % 5 == 0:
message = 'Divisible by 5'
elif x % 2 == 0:
message = 'Even'
else:
message = 'Odd'
print(message)
# Output:
# Divisible by 5
This code does exactly the same thing as the nested ternary operation example, but it’s arguably easier to read, especially for beginners.
Switch-Case Statements Using Dictionaries
Python doesn’t have built-in switch-case statements like some other languages, but you can mimic them using dictionaries. Here’s an example:
x = 15
switch = {
0: 'Divisible by 5',
1: 'Odd',
2: 'Even'
}
message = switch.get(x % 5, 'Not divisible by 5')
print(message)
# Output:
# Divisible by 5
In this example, we create a dictionary where each key-value pair corresponds to a case and its result. We use the get
method to retrieve the value for the key x % 5
. If x % 5
isn’t a key in the dictionary, it returns ‘Not divisible by 5’.
While these alternatives might be more verbose than the ternary operator, they can make your code easier to read and understand, which is crucial when you’re working on complex projects or collaborating with other developers.
Troubleshooting Common Issues with the Python Ternary Operator
Like any feature in a programming language, the ternary operator has its quirks and pitfalls. Understanding these can help you avoid bugs and write more effective code. Let’s go over some common issues you might encounter when using the ternary operator in Python.
Operator Precedence Issues
One common issue arises from the fact that the ternary operator has lower precedence than most other operators in Python. This means that in an expression with multiple operators, the ternary operation will be performed last unless you use parentheses to change the order of operations.
Consider the following example:
x = 5
y = 10
result = x / y * 100 if x < y else 0
print(result)
# Output:
# 0
You might expect this code to print ‘50.0’, but it actually prints ‘0’. That’s because x / y * 100
is evaluated first due to operator precedence, and then the ternary operation is performed.
To get the expected result, you need to use parentheses like so:
x = 5
y = 10
result = (x / y * 100) if x < y else 0
print(result)
# Output:
# 50.0
By using parentheses, you ensure that the division and multiplication are performed before the ternary operation, giving you the correct result.
Understanding operator precedence and using parentheses appropriately are key to avoiding bugs and confusion when using the ternary operator in Python.
Understanding Operators in Python
Before we dive deeper into the ternary operator, let’s take a step back and understand what operators are in Python. Operators are special symbols in Python that carry out arithmetic or logical computation. The value or variable that the operator works on is called the operand.
For example, in the expression 5 + 3
, 5
and 3
are operands, and +
is an operator.
Categories of Operators in Python
Python has several types of operators, including arithmetic operators (+
, -
, *
, etc.), comparison operators (==
, !=
, <
, etc.), and logical operators (and
, or
, not
).
The ternary operator is a type of conditional operator. It’s a shortcut for the if-else
statement and is used to make decisions in your code.
The Role of the Ternary Operator in Python
The ternary operator in Python allows you to evaluate an expression based on a condition being true or false. It significantly shortens the amount of code you need to write and improves readability for simple conditions.
Here’s an example of how you might use it:
x = 5
message = 'Hello' if x > 0 else 'Goodbye'
print(message)
# Output:
# Hello
In this example, the ternary operator checks if x
is greater than 0
. If it is, it assigns the string ‘Hello’ to message
. If not, it assigns the string ‘Goodbye’. The ternary operator allows us to do this in a single line of code, making our code more concise and readable.
The Ternary Operator in Real-World Applications
The ternary operator isn’t just a theoretical concept—it has practical applications in many areas of Python programming. Let’s explore a few of them.
Data Analysis
In data analysis, you often need to categorize or transform data based on certain conditions. The ternary operator makes this process much more streamlined. For instance, you might want to categorize a dataset of heights into ‘Tall’ and ‘Short’. The ternary operator can do this in a single line of code.
height = 180 # in centimeters
category = 'Tall' if height > 170 else 'Short'
print(category)
# Output:
# Tall
Web Development
In web development, the ternary operator can be used to control the flow of your application. For instance, you might want to display a different message to the user based on whether they’re logged in or not.
is_logged_in = True # this would typically be determined by your application logic
message = 'Welcome back!' if is_logged_in else 'Please log in.'
print(message)
# Output:
# Welcome back!
Exploring Further
The ternary operator is part of a larger concept in Python—control flow. Control flow is all about making decisions and controlling the execution of your code. If you’re interested in learning more about control flow and decision making in Python, here are some online resources:
- Click Here for an in-depth overview on conditional statements in Python and how they enable dynamic code.
Using if-else in Python – A practical guide on structuring Python code with “if-else” for decision-making and branching.
Python’s “if not” Statements – Master the art of leveraging “if not” for advanced Python conditionals.
Python Booleans: The Definitive Guide – A guide by W3Schools that focuses on working with booleans in Python.
All About Conditional Execution in Python – A Medium article that delves deep into conditional execution in Python.
Python If-Else Statement – A FreeCodeCamp guide providing explanations on the use of conditional “if-else” statements in Python.
Mastering the ternary operator and other control flow tools will make you a more efficient Python programmer and open up new possibilities in your coding projects.
Recap: Mastering the Python Ternary Operator
In this comprehensive guide, we’ve explored the ternary operator in Python, from its basic usage to advanced scenarios. We’ve learned that it’s a shorthand for the if-else statement, allowing us to write more concise and efficient code.
We’ve also delved into common issues, such as operator precedence, which can lead to unexpected results. Remember, using parentheses can help avoid these issues and ensure your ternary operations work as intended.
Additionally, we’ve looked at alternative approaches to conditional logic in Python, including traditional if-else statements and mimicking switch-case statements using dictionaries. While the ternary operator is a powerful tool, these alternatives can provide better readability and clarity in complex situations.
Here’s a quick comparison of the methods we’ve discussed:
Method | Conciseness | Readability | Complexity |
---|---|---|---|
Ternary Operator | High | Medium | Low to Medium |
If-Else Statement | Medium | High | Low |
Dictionary Switch-Case | Medium | Medium | Medium to High |
As we’ve seen, the ternary operator is a versatile tool in Python, useful in various real-world applications like data analysis and web development. However, its power comes with the responsibility to use it wisely and appropriately. Happy coding!