Web Scraping with Cheerio | npm Package Guide
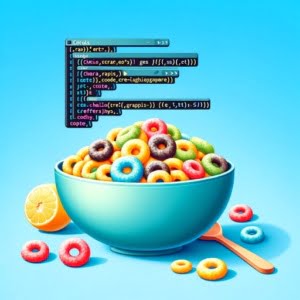
Diving into web scraping with Node.js? Like a skilled craftsman, Cheerio.js allows you to manipulate and traverse HTML documents with ease. Whether you’re a beginner or an experienced developer, you will be able to learn more about HTML parsing with Cheerio in today’s article.
When developing software for IOFLOOD, efficiently extracting data from HTML content is crucial for server-side operations. To assist our bare metal server customers, and other Node.js developers, grappling with HTML parsing challenges, we’ve compiled our insights into this comprehensive Cheerio guide.
This guide will walk you through using Cheerio with npm to unlock powerful web scraping capabilities. Get ready to transform the way you handle web content, making your development process more efficient and your data more accessible.
Let’s dive in and harness the capabilities of Cheerio to streamline HTML parsing in your Node.js projects.
TL;DR: How Do I Use Cheerio with npm for Web Scraping?
To use Cheerio for web scraping, first install it via npm with
npm install cheerio
. Then, load your HTML and use Cheerio’s jQuery-like syntax to select and manipulate elements.
Here’s a quick example:
const cheerio = require('cheerio');
const $ = cheerio.load('<h2 class="title">Hello world</h2>');
$('h2.title').text('Hello there!');
$('h2').addClass('welcome');
# Output:
# <h2 class="title welcome">Hello there!</h2>
In this example, we start by requiring the Cheerio module and loading an HTML string. We then use Cheerio’s jQuery-like syntax to change the text of an <h2>
element and add a class to it. This demonstrates how straightforward it is to manipulate HTML elements with Cheerio, making it an invaluable tool for web scraping tasks.
Ready to dive deeper into Cheerio’s capabilities and best practices for web scraping? Keep reading for a comprehensive guide that will transform your approach to handling web content.
Table of Contents
Getting Started with Cheerio
Embarking on your web scraping journey begins with a simple yet powerful step: installing Cheerio. Cheerio npm, as it’s commonly referred to, bridges the gap between server-side logic and front-end ease, making it a go-to for developers looking to manipulate HTML content efficiently.
Installation
To get started, you’ll need to have Node.js installed on your machine. Once that’s set, installing Cheerio is as easy as running a single command in your terminal:
npm install cheerio
Loading HTML Content
With Cheerio installed, the next step is to load the HTML content you wish to work with. This can be HTML from a website, a file, or even a string. Here’s how you can load HTML content into Cheerio for manipulation:
const cheerio = require('cheerio');
const htmlContent = '<div class="greeting">Hello, Cheerio!</div>';
const $ = cheerio.load(htmlContent);
Basic Manipulations and Selections
Now that you have your HTML content loaded, let’s perform some basic manipulations. Say you want to change the text inside the <div>
tag. Here’s how you can do it:
$('div.greeting').text('Hello, world!');
# Output:
# <div class="greeting">Hello, world!</div>
In this example, we used the .text()
method to change the text content of the <div>
element with the class greeting
. This demonstrates the simplicity and power of Cheerio’s jQuery-like syntax for selecting and manipulating HTML elements.
Through these steps, you’ve seen how to install Cheerio, load HTML, and perform basic manipulations. This foundation will serve you well as you dive deeper into web scraping with Cheerio npm.
Advanced Cheerio Techniques
After mastering the basics of Cheerio npm, it’s time to delve into more sophisticated uses. Cheerio’s real power shines when you start manipulating complex HTML structures, handling dynamic content, and utilizing advanced selectors to pinpoint exactly what you need from a sea of markup.
Handling Dynamic Content
One of the challenges of web scraping is dealing with dynamic content. While Cheerio itself doesn’t execute JavaScript, it can work in tandem with other tools to handle dynamically generated content. Here’s how you might approach this:
First, use a tool like Axios to fetch the HTML content. Then, load this content into Cheerio for manipulation.
const axios = require('axios');
const cheerio = require('cheerio');
async function fetchAndParse(url) {
const { data } = await axios.get(url);
const $ = cheerio.load(data);
// Now you can use Cheerio to manipulate the HTML
const dynamicContent = $('.dynamic-content').text();
console.log(dynamicContent);
}
fetchAndParse('https://example.com');
# Output:
# 'This is dynamically generated content'
In this snippet, we’re fetching HTML content using Axios, then loading it into Cheerio. This approach allows us to access and manipulate even the content that was dynamically generated by JavaScript on the server-side.
Advanced Selectors
Cheerio’s selector engine is incredibly powerful, enabling you to traverse and manipulate the DOM with precision. Let’s explore using advanced CSS selectors to extract specific information:
const $ = cheerio.load('<ul class="items"><li class="item active">Item 1</li><li class="item">Item 2</li></ul>');
const activeItemText = $('li.item.active').text();
console.log(activeItemText);
# Output:
# 'Item 1'
This code demonstrates how to use Cheerio’s selectors to target elements with specific classes. By combining class selectors, we can pinpoint the exact element we’re interested in, showcasing the flexibility and power of Cheerio’s selection capabilities.
Through these examples, you’ve seen how Cheerio npm can be used to handle more complex web scraping tasks. By combining Cheerio with other tools like Axios and leveraging advanced selectors, you can tackle a wide range of scraping challenges.
Elevating Cheerio with npm Packages
As you venture further into the world of web scraping with Cheerio npm, you’ll find that combining it with other npm packages can significantly enhance its capabilities. This section explores how integrating Cheerio with Axios for HTTP requests and Puppeteer for handling JavaScript-rendered pages can take your web scraping projects to the next level.
Cheerio and Axios for HTTP Requests
Axios is a promise-based HTTP client for the browser and node.js, making it a perfect companion for Cheerio when fetching web pages. Here’s how you can use them together:
const axios = require('axios');
const cheerio = require('cheerio');
async function fetchPage(url) {
const { data } = await axios.get(url);
const $ = cheerio.load(data);
const pageTitle = $('title').text();
console.log(pageTitle);
}
fetchPage('https://example.com');
# Output:
# 'Example Domain'
In this example, Axios fetches the HTML from ‘https://example.com’, and Cheerio loads the fetched HTML. We then extract the page title using Cheerio’s simple, jQuery-like syntax. This demonstrates the seamless integration between Axios and Cheerio, allowing for efficient HTML fetching and manipulation.
Cheerio and Puppeteer for JavaScript Pages
Puppeteer is a Node library which provides a high-level API to control Chrome or Chromium over the DevTools Protocol. It’s especially useful for scraping JavaScript-rendered content. Here’s an example of how Puppeteer can be used in conjunction with Cheerio:
const puppeteer = require('puppeteer');
const cheerio = require('cheerio');
(async () => {
const browser = await puppeteer.launch();
const page = await browser.newPage();
await page.goto('https://example-dynamic.com');
const content = await page.content();
const $ = cheerio.load(content);
const dynamicText = $('.dynamic-text').text();
console.log(dynamicText);
await browser.close();
})();
# Output:
# 'This is dynamically generated text'
This snippet illustrates how Puppeteer navigates to a page with dynamically generated content, retrieves the full HTML content, and then Cheerio is used to parse and manipulate the HTML. It highlights the powerful combination of Puppeteer for rendering dynamic content and Cheerio for efficient DOM manipulation.
Through these examples, we’ve explored advanced techniques that combine Cheerio with other powerful npm packages. These combinations unlock new possibilities for web scraping, allowing developers to handle a wider range of scenarios with ease.
Cheerio Troubleshooting Guide
When diving into web scraping with Cheerio npm, you’re bound to encounter some hurdles. Understanding how to navigate these challenges will ensure your scraping projects are both efficient and robust. Let’s explore common issues and their solutions.
Handling Encoding Issues
A frequent issue when scraping web content is dealing with different character encodings. Cheerio, by default, operates with UTF-8 encoding, which might not match the encoding of the scraped website, leading to garbled text output.
To handle encoding issues, you can use the iconv-lite
package to convert the encoding before loading the content into Cheerio:
const axios = require('axios');
const iconv = require('iconv-lite');
const cheerio = require('cheerio');
async function fetchAndConvert(url) {
const response = await axios({
url,
responseType: 'arraybuffer'
});
const content = iconv.decode(Buffer.from(response.data), 'win1251'); // Example for Cyrillic content
const $ = cheerio.load(content);
console.log($('title').text());
}
fetchAndConvert('https://example-cyrillic.com');
# Output:
# 'Пример домена'
In this example, axios
fetches the HTML content as an array buffer, which iconv-lite
then decodes from Windows-1251 (a common encoding for Cyrillic scripts) to UTF-8. This ensures that when the content is loaded into Cheerio, it’s correctly encoded, and text manipulation yields the expected results.
Dealing with Malformed HTML
Another common challenge is scraping sites with malformed HTML. Cheerio is built on top of the forgiving, fast HTML parsing library htmlparser2
, which can handle a wide range of HTML quirks. However, some issues might still need manual intervention.
When you encounter malformed HTML, consider using the sanitize-html
package before loading it into Cheerio. This package can clean up the HTML, making it easier for Cheerio to parse:
const cheerio = require('cheerio');
const sanitizeHtml = require('sanitize-html');
const dirtyHtml = '<div><p>Unclosed paragraph<div><p>Another paragraph</div></div>';
const cleanHtml = sanitizeHtml(dirtyHtml);
const $ = cheerio.load(cleanHtml);
console.log($('p').length);
# Output:
# 2
This snippet demonstrates using sanitize-html
to clean up malformed HTML, ensuring that Cheerio can accurately parse and manipulate the content. By sanitizing the HTML, we ensure that all elements are correctly closed, and Cheerio can accurately select and manipulate them, showcasing its flexibility and robustness in handling real-world web scraping challenges.
Cheerio and jQuery: A Powerful Duo
When embarking on a web scraping project using Cheerio npm, it’s crucial to understand the technology’s core and its relationship with jQuery. Cheerio provides an API that mirrors jQuery, one of the most popular front-end libraries, making it incredibly intuitive for those familiar with jQuery’s syntax. However, unlike jQuery, which operates in the browser and manipulates the DOM of web pages directly, Cheerio works on the server-side, allowing for the manipulation of HTML documents fetched from the web.
Why Choose Cheerio for Server-Side DOM Manipulation?
Cheerio’s ability to parse, manipulate, and render HTML on the server-side makes it an ideal choice for web scraping. It’s lightweight, fast, and doesn’t require a DOM to be present, unlike browser-based libraries. Here’s a simple demonstration of Cheerio loading an HTML string and manipulating it:
const cheerio = require('cheerio');
const html = '<ul id="fruits"><li class="apple">Apple</li><li class="orange">Orange</li></ul>';
const $ = cheerio.load(html);
$('.apple', '#fruits').text('Green Apple');
console.log($.html());
# Output:
# <ul id="fruits"><li class="apple">Green Apple</li><li class="orange">Orange</li></ul>
In this code block, we start by requiring Cheerio and loading an HTML string. We then use Cheerio’s jQuery-like syntax to select the element with the class apple
and change its text to ‘Green Apple’. This demonstrates how Cheerio allows for easy manipulation of HTML elements, similar to jQuery, but on the server-side.
The Importance of Web Scraping
Web scraping is a powerful tool for data extraction, allowing developers to collect information from websites that might not provide an API. It’s used in a variety of applications, from data analysis to automated testing. However, it’s important to approach web scraping with ethical considerations in mind. Always respect the website’s robots.txt
rules and seek permission when necessary to avoid legal issues.
Understanding the fundamentals of Cheerio and its relationship with jQuery not only equips you with the skills to manipulate HTML effectively but also encourages responsible use of web scraping technologies. As you delve deeper into using Cheerio npm for your projects, remember the importance of ethical web scraping practices and the powerful capabilities Cheerio brings to server-side DOM manipulation.
Expanding Cheerio’s Horizons
As you become more adept at using Cheerio npm for web scraping, you’ll likely start to think about its application in larger, more complex projects. Whether it’s building a comprehensive web scraper service or integrating scraped data into databases for analysis, the possibilities are vast. Let’s explore how Cheerio can be a cornerstone in these endeavors and where you can go to learn even more.
Building a Web Scraper Service
Imagine creating a service that regularly scrapes data from multiple sources, processes it, and then offers this consolidated data through an API. Cheerio, combined with Node.js frameworks like Express, can make this a reality. Here’s a basic example of how you might set up a simple scraping service:
const express = require('express');
const cheerio = require('cheerio');
const axios = require('axios');
const app = express();
app.get('/scrape', async (req, res) => {
const { data } = await axios.get('https://example.com');
const $ = cheerio.load(data);
const pageTitle = $('title').text();
res.json({ title: pageTitle });
});
app.listen(3000, () => console.log('Scraper service running on port 3000'));
# Output:
# 'Scraper service running on port 3000'
This snippet showcases a simple server that scrapes the title of ‘https://example.com’ and returns it as JSON. By expanding on this basic structure, you can build out a service that offers a variety of scraped data endpoints.
Integrating with Databases
Storing and analyzing scraped data can provide valuable insights. Integrating Cheerio with a database, such as MongoDB, allows you to persist scraped data for future analysis. Here’s an example of saving the title of a webpage into a MongoDB database:
// This example assumes MongoDB setup and mongoose package installed
const mongoose = require('mongoose');
const cheerio = require('cheerio');
const axios = require('axios');
// Define a simple schema for titles
const TitleSchema = new mongoose.Schema({
pageTitle: String
});
const Title = mongoose.model('Title', TitleSchema);
async function saveTitle(url) {
const { data } = await axios.get(url);
const $ = cheerio.load(data);
const pageTitle = $('title').text();
const titleInstance = new Title({ pageTitle });
await titleInstance.save();
console.log('Title saved');
}
// Connect to MongoDB and save a title
mongoose.connect('your_mongodb_connection_string', { useNewUrlParser: true, useUnifiedTopology: true });
saveTitle('https://example.com');
# Output:
# 'Title saved'
This code demonstrates fetching a webpage, extracting its title, and then saving that title into a MongoDB database. This kind of integration is foundational for building out larger data collection and analysis projects.
Further Resources for Cheerio Mastery
To deepen your understanding and skills in web scraping with Cheerio npm, here are three invaluable resources:
- Cheerio Official Documentation – The go-to resource for a comprehensive understanding of Cheerio’s API and capabilities.
Scotch.io Tutorials – Practical guides and tutorials on web development, encompassing usage of Cheerio for web scraping.
The Net Ninja YouTube Channel – Easy-to-follow video tutorials on web development, featuring sessions on utilizing Cheerio for server-side manipulation.
By exploring these resources, you’ll gain a deeper understanding of web scraping and how to leverage Cheerio npm in your projects, pushing your skills beyond the basics and into the realm of advanced data manipulation and analysis.
Recap: Web Scraping with Cheerio
In this comprehensive guide, we’ve explored the ins and outs of using Cheerio with npm to harness the full potential of web scraping. From a simple installation to navigating through HTML documents with ease, Cheerio has proven to be an invaluable tool for developers looking to extract and manipulate web content.
We began with the basics, demonstrating how to install Cheerio via npm and load HTML content for manipulation. We then progressed to performing basic manipulations and selections, showcasing Cheerio’s jQuery-like syntax for easy element selection and content alteration.
Moving forward, we delved into more advanced topics, such as handling dynamic content with Axios and utilizing Puppeteer for scraping JavaScript-rendered pages. These techniques opened up new possibilities for scraping more complex websites.
Finally, we explored alternative approaches for enhancing Cheerio’s capabilities, including integrating with other npm packages for expanded functionality. This allowed for the creation of more sophisticated and robust web scraping solutions.
Feature | Description |
---|---|
Basic Manipulation | Easy element selection and content alteration with jQuery-like syntax |
Dynamic Content Handling | Use of Axios and Puppeteer for scraping dynamic and JavaScript-rendered pages |
Integration with npm Packages | Enhancing functionality by combining Cheerio with other packages |
Whether you’re just starting out with Cheerio or looking to expand your web scraping skills, this guide has provided you with the knowledge and tools necessary to tackle a wide range of scraping tasks. Cheerio’s lightweight, flexible nature makes it a powerful choice for developers looking to efficiently manipulate HTML content on the server-side.
With its balance of simplicity and power, Cheerio npm is a cornerstone for any developer’s web scraping toolkit. Happy scraping!