Java ‘var’ Keyword: A Detailed Usage Guide
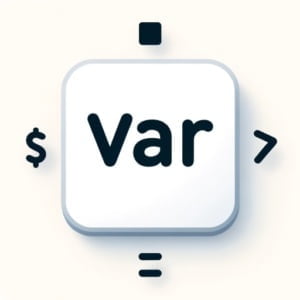
Are you finding it challenging to understand the ‘var’ keyword in Java? You’re not alone. Many developers find themselves puzzled when it comes to handling ‘var’ in Java, but we’re here to help.
Think of ‘var’ in Java as a chameleon – it adapts its type based on the variable it’s assigned to, providing a versatile and handy tool for various tasks.
In this guide, we’ll walk you through the process of working with ‘var’ in Java, from its basic usage, advanced techniques, to alternative approaches. We’ll cover everything from the basics of type inference to more complex uses of ‘var’, as well as when not to use ‘var’ and common pitfalls.
Let’s get started on mastering ‘var’ in Java!
TL;DR: What is ‘var’ in Java?
'var'
is a keyword introduced in Java 10 that allows you to declare a variable without specifying its type. The type is inferred from the variable’s initial value, for examplevar age = 10;
is inferred to be an integer. This feature is known as type inference and it can make your code more concise and easier to read.
Here’s a simple example:
var name = 'John';
System.out.println(name);
# Output:
# 'John'
In this example, we’ve used the ‘var’ keyword to declare a variable named ‘name’ and assign it the value ‘John’. The type of the ‘name’ variable is inferred to be a String based on its initial value. When we print the value of ‘name’, the output is ‘John’.
This is just a basic way to use ‘var’ in Java, but there’s much more to learn about type inference and other advanced uses of ‘var’. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
Basic Usage of ‘var’ in Java
The ‘var’ keyword in Java allows you to declare a variable without specifying its type. This feature is known as type inference and it’s a powerful tool that can make your code more concise and easier to read.
Here’s an example of how to declare and use variables with ‘var’:
var number = 10;
var greeting = 'Hello, world!';
System.out.println(number);
System.out.println(greeting);
# Output:
# 10
# 'Hello, world!'
In this example, we’ve declared two variables using ‘var’: ‘number’ and ‘greeting’. The types of these variables are inferred based on their initial values. The ‘number’ variable is inferred to be an integer, while ‘greeting’ is inferred to be a String.
The main advantage of using ‘var’ is that it can make your code more concise and easier to read, especially when dealing with complex types. However, it’s important to be aware of potential pitfalls. For instance, ‘var’ can only be used when a variable is initialized in the same statement in which it’s declared. If you try to declare a variable with ‘var’ without initializing it, you’ll get a compilation error.
var name; // Compilation error
Another thing to keep in mind is that ‘var’ is not a keyword that denotes a ‘dynamic’ or ‘loosely-typed’ variable. The type of the variable is still statically checked at compile time. If you try to assign a value of a different type to the variable later, you’ll get a compilation error.
var message = 'Hello, world!';
message = 123; // Compilation error
In this example, we’ve declared a variable ‘message’ with an initial value of ‘Hello, world!’, so its type is inferred to be a String. Later, we try to assign an integer to ‘message’, which results in a compilation error because an integer is not compatible with a String.
Advanced Usage of ‘var’ in Java
As you become more comfortable with the ‘var’ keyword in Java, you can start to explore some of its more advanced uses. This includes using ‘var’ with lambda expressions or streams, which are powerful features in Java that can greatly enhance your code.
Using ‘var’ with Lambda Expressions
Lambda expressions in Java are a way to represent anonymous functions, and they can be used with ‘var’ to make your code more concise. Here’s an example:
var list = List.of(1, 2, 3, 4, 5);
var sum = list.stream().reduce(0, (a, b) -> a + b);
System.out.println(sum);
# Output:
# 15
In this example, we’ve used ‘var’ with a lambda expression in the ‘reduce’ method. The ‘reduce’ method is used to perform a reduction on the elements of the stream, in this case to calculate the sum of the numbers in the list. The lambda expression ‘(a, b) -> a + b’ is used to specify how the reduction should be performed.
Using ‘var’ with Streams
Streams in Java are a way to work with sequences of elements, such as collections, in a functional programming style. You can use ‘var’ when working with streams to make your code more concise. Here’s an example:
var list = List.of('apple', 'banana', 'cherry');
var uppercaseList = list.stream().map(String::toUpperCase).collect(Collectors.toList());
System.out.println(uppercaseList);
# Output:
# [APPLE, BANANA, CHERRY]
In this example, we’ve used ‘var’ with a stream to convert a list of strings to uppercase. The ‘map’ method is used to apply a function to each element of the stream, in this case the ‘toUpperCase’ method of the String class. The result is collected into a new list.
These are just a couple of examples of how you can use ‘var’ in more advanced ways in Java. As you continue to learn and practice, you’ll discover even more ways to use ‘var’ to improve your code.
Alternative Approaches to ‘var’
While ‘var’ is a powerful keyword in Java, it’s not always the best choice. There are situations where it’s better to specify the type of a variable explicitly, and there are also common errors and best practices to be aware of when using ‘var’.
When Not to Use ‘var’
One situation where you might not want to use ‘var’ is when the type of a variable is not clear from its initial value. For example:
var number = getNumber();
In this example, it’s not clear what type ‘number’ is without looking at the implementation of the ‘getNumber’ method. In these cases, it might be better to specify the type explicitly for the sake of readability.
Common Errors
A common error when using ‘var’ is trying to use it with null values. For example:
var name = null; // Compilation error
This will result in a compilation error because the type of ‘name’ cannot be inferred from a null value.
Best Practices
When using ‘var’, it’s a good practice to always initialize your variables with a value that makes the type clear. It’s also a good practice to use ‘var’ sparingly and only when it improves the readability of your code.
Another best practice is to avoid using ‘var’ with variables that have a wide scope. The wider the scope of a variable, the harder it can be to infer its type at a glance, which can make your code harder to read.
Ultimately, the decision to use ‘var’ should be based on whether it makes your code more readable and maintainable. While ‘var’ can make your code more concise, it’s not always the best choice, and it’s important to consider the trade-offs.
Troubleshooting ‘var’ in Java
Like any feature, using ‘var’ in Java can sometimes lead to unexpected issues. It’s important to know how to troubleshoot these problems and understand the considerations when using ‘var’.
Type Inference Errors
One common issue is type inference errors. These occur when the Java compiler cannot infer the type of a variable declared with ‘var’. For instance, if you try to declare a variable with ‘var’ without initializing it, you’ll get a compilation error.
var name; // Compilation error
In this case, the compiler doesn’t have enough information to infer the type of the ‘name’ variable, resulting in an error.
Null Values
Another common issue is using ‘var’ with null values. The ‘var’ keyword requires an initial value to infer the variable type. If you try to initialize a ‘var’ variable with a null value, you’ll get a compilation error.
var name = null; // Compilation error
This is because the compiler cannot infer the type from a null value.
Solutions and Tips
To avoid these issues, always initialize your ‘var’ variables with a value that makes the type clear. If the type cannot be clearly inferred from the initial value, consider specifying the type explicitly.
String name = getName(); // Better than var name = getName();
In this example, specifying the type explicitly with ‘String’ makes the code clearer and avoids potential type inference issues.
Remember, ‘var’ is a powerful tool, but it’s not always the best choice. Always consider the readability and clarity of your code when deciding whether to use ‘var’.
Understanding Type Inference in Java
Type inference is a feature in Java that allows the Java compiler to automatically determine the data type of expressions. It’s a fundamental concept that’s closely related to the ‘var’ keyword.
How Does Type Inference Work?
In Java, type inference is the process by which the Java compiler automatically determines the data type of an expression. This is done based on the context in which the expression is used.
For instance, when you declare a variable with ‘var’ and initialize it with a value, the Java compiler can infer the type of the variable from its initial value.
var name = 'John'; // The type of 'name' is inferred to be String
In this example, the Java compiler infers that the type of the ‘name’ variable is String because it’s initialized with a string value.
How Does ‘var’ Relate to Type Inference?
The ‘var’ keyword in Java leverages the power of type inference. When you declare a variable with ‘var’, you don’t need to specify its type. Instead, the Java compiler infers the type from the variable’s initial value.
This can make your code more concise and easier to read, especially when dealing with complex types.
var list = new ArrayList<String>(); // The type of 'list' is inferred to be ArrayList<String>
In this example, the Java compiler infers that the type of the ‘list’ variable is ArrayList based on its initial value.
Understanding type inference is key to mastering the use of ‘var’ in Java. By leveraging type inference, ‘var’ can help you write cleaner, more readable code.
The Relevance of ‘var’ in Larger Java Projects
The ‘var’ keyword in Java is not just a tool for simplifying variable declarations in small programs or scripts. It has significant relevance in larger Java projects as well.
In large-scale projects, the use of ‘var’ can greatly enhance code readability and maintainability. It makes the code cleaner and less cluttered, especially when dealing with complex types. This can be particularly beneficial in projects with numerous developers, where code readability is crucial.
Moreover, ‘var’ can be a valuable tool when working with modern Java features like lambda expressions and streams. Using ‘var’ in conjunction with these features can result in more concise and expressive code.
Exploring Related Concepts
If you’ve mastered the use of ‘var’ in Java, you might want to explore related concepts to broaden your Java knowledge. Lambda expressions and streams are two such concepts that are worth exploring. They are powerful features in Java that can greatly enhance your code, especially when used in conjunction with ‘var’.
Lambda expressions allow you to represent anonymous functions in a concise way, while streams provide a functional programming approach to handle sequences of elements. Understanding these concepts can take your Java skills to the next level.
Further Resources for ‘var’
To deepen your understanding of ‘var’ and related concepts in Java, you might find the following resources helpful:
- IOFlood’s Article on Variables in Java provides insights on Java variables.
The Java Protected Keyword – Explore Java’s protected keyword for encapsulation in your code.
Java .length Property Explained – The .length property in Java for determining the length of arrays and strings.
Oracle’s Java Tutorials cover a wide range of Java topics, including ‘var’, lambda expressions, and streams.
Baeldung’s Guide to ‘var’ in Java provides a detailed explanation of ‘var’ in Java, complete with examples.
GeeksforGeeks’ Understanding “var” keyword in Java explains the concept and usage of “var” keyword in Java language.
Wrapping Up: ‘var’ Keyword in Java
In this comprehensive guide, we’ve delved into the depths of the ‘var’ keyword in Java, exploring its usage, advantages, common issues, and their solutions.
We began with the basics, understanding how ‘var’ works and its role in type inference. We then moved on to more advanced usage of ‘var’, including its application in lambda expressions and streams. Along the way, we tackled common challenges you might face when using ‘var’, such as type inference errors and issues with null values, providing you with solutions and tips for each problem.
We also discussed alternative approaches to ‘var’, highlighting situations where explicit type declaration might be more beneficial. Throughout, we emphasized the importance of readability and clarity in code when deciding whether to use ‘var’.
Here’s a quick comparison of ‘var’ and traditional variable declaration:
Method | Flexibility | Readability | Error Handling |
---|---|---|---|
‘var’ | High | High (with clear initial values) | Type inference errors can occur |
Traditional Declaration | Low | High (always clear type) | No type inference errors |
Whether you’re just starting out with ‘var’ in Java or looking to enhance your skills, we hope this guide has given you a deeper understanding of ‘var’ and its role in Java programming.
With ‘var’, you have a powerful tool for making your code more concise and readable. It’s another step towards mastering Java. Happy coding!