.Length() Java: A Guide for Beginners to Experts
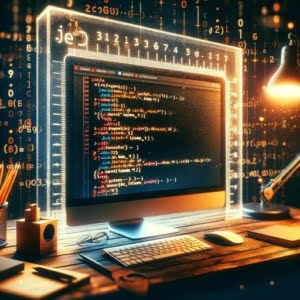
Are you finding it challenging to understand the .length property in Java? You’re not alone. Many developers, especially beginners, struggle with this concept. But think of .length in Java as a measuring tape, a tool that can help you determine the size of arrays and strings in Java.
Just like a tailor uses a measuring tape to measure the length of a cloth, a programmer uses .length to measure the size of arrays and strings. It’s a simple yet powerful tool that can make your programming tasks a lot easier.
This guide will walk you through the basics to advanced usage of .length in Java. We’ll explore how to use .length with arrays and strings, delve into its usage with multidimensional arrays and complex data structures, and even discuss common issues and their solutions.
So, let’s dive in and start mastering .length in Java!
TL;DR: How Do I Use .length in Java?
In Java,
.length
is used to get the length of an array or a string, with the syntaxint length = str.length();
. It’s a property that helps you determine the size of your arrays and strings, making it a handy tool in your Java programming toolkit.
Here’s a simple example:
String str = 'Hello';
int length = str.length();
System.out.println(length);
# Output:
# 5
In this example, we’ve created a string named str
with the value ‘Hello’. We then use the .length property to get the length of str
, which is 5. The length is stored in the length
variable, and we print it out, resulting in the output ‘5’.
This is a basic way to use .length in Java, but there’s much more to learn about handling arrays and strings in Java. Continue reading for more detailed explanations and advanced usage scenarios.
Table of Contents
- Using .length with Arrays and Strings in Java
- Delving Deeper: .length with Multidimensional Arrays
- Exploring Alternatives: Beyond .length in Java
- Navigating Potential Pitfalls: Troubleshooting .length in Java
- Understanding Arrays and Strings in Java
- Why is .length Important?
- Real-World Applications: The Relevance of .length in Java
- Wrapping Up: Mastering .length in Java
Using .length with Arrays and Strings in Java
In Java, the .length property is primarily used to determine the size of arrays and strings. It’s a simple and straightforward way to know how many elements an array has or how many characters a string contains.
Let’s start with a basic example of how to use .length with strings:
String str = 'Hello, World!';
int length = str.length();
System.out.println(length);
# Output:
# 13
In this example, we’ve created a string str
with the value ‘Hello, World!’. The string length is calculated by the .length() method, and we store the result in the length
variable. When we print length
, we get ’13’, which is the number of characters in our string (including spaces and punctuation).
Now, let’s see how we can use .length with arrays:
int[] numbers = {1, 2, 3, 4, 5};
int length = numbers.length;
System.out.println(length);
# Output:
# 5
Here, we’ve created an array numbers
with five elements. We then use the .length property to get the length of the array, which is ‘5’. This tells us that our array contains five elements.
Understanding .length: Advantages and Pitfalls
The .length property is a powerful tool in Java, but it’s essential to understand its advantages and potential pitfalls.
- Advantages: The .length property is straightforward and easy to use. It provides a quick way to determine the size of arrays and strings, which is often necessary in programming tasks.
Potential Pitfalls: However, it’s important to note that .length counts all characters in a string, including spaces and punctuation. Similarly, for arrays, .length will count all elements, regardless of their value. Therefore, it’s crucial to ensure your arrays and strings are correctly formatted to get accurate results.
Delving Deeper: .length with Multidimensional Arrays
As you become more comfortable with Java, you’ll find yourself working with more complex data structures, such as multidimensional arrays. The .length property continues to be a reliable tool in these situations.
Consider a two-dimensional array, which is essentially an ‘array of arrays’. Here’s how you can use .length with it:
int[][] numbers = {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}};
int length = numbers.length;
System.out.println(length);
# Output:
# 3
In this example, numbers
is a two-dimensional array containing three arrays. The .length property tells us that numbers
contains three elements, each of which is an array.
To find the length of the individual arrays, you can use .length like this:
int lengthFirstArray = numbers[0].length;
System.out.println(lengthFirstArray);
# Output:
# 3
Here, numbers[0].length
gives the length of the first array within numbers
, which is ‘3’.
Best Practices and Potential Issues
While .length is highly useful, it’s important to use it correctly to avoid potential issues. Here are a few best practices and pitfalls to keep in mind:
- Best Practice: Always check if your array is not null before calling .length to avoid NullPointerException.
Potential Issue: If you try to access an index that is equal to or larger than the array’s length, you will encounter an ArrayIndexOutOfBoundsException. Always ensure your index is less than the array’s length.
Best Practice: When dealing with multidimensional arrays, remember that .length will give you the length of the outermost array. To get the length of the inner arrays, you need to specify the index of the inner array.
Exploring Alternatives: Beyond .length in Java
While .length is a powerful tool for arrays and strings in Java, it’s not the only way to determine the length or size. As you become more proficient in Java, you’ll encounter alternative methods, especially when working with collections.
Using size() with Collections
In Java, collections are a more advanced data structure than arrays, and they come with their own method for determining size: the size() method. Here’s how you can use it with an ArrayList:
import java.util.ArrayList;
ArrayList<Integer> numbers = new ArrayList<Integer>();
numbers.add(1);
numbers.add(2);
numbers.add(3);
int size = numbers.size();
System.out.println(size);
# Output:
# 3
In this example, we’ve created an ArrayList numbers
and added three elements to it. The size() method tells us that numbers
contains three elements.
Advantages and Disadvantages
The size() method and collections offer several advantages over .length and arrays:
- Flexibility: Collections like ArrayList can grow and shrink dynamically, which is not possible with arrays.
Methods: Collections come with a rich set of methods for sorting, searching, and manipulating data.
However, these advantages come with some trade-offs:
- Performance: Arrays can be faster to access and use less memory than collections.
Complexity: Collections are more complex than arrays, which can make them harder to learn for beginners.
Recommendations
While .length is a great tool for beginners and for situations where you’re working with simple arrays and strings, collections and the size() method are powerful alternatives for more complex and dynamic data structures. As an expert Java programmer, it’s important to be familiar with both and to use the right tool for the job.
As with any tool in programming, using .length in Java can sometimes lead to unexpected issues. Two of the most common exceptions you might encounter are NullPointerExceptions and ArrayIndexOutOfBoundsExceptions. Let’s discuss these issues, their solutions, and some best practices to avoid them.
NullPointerExceptions with .length
A NullPointerException can occur if you try to use .length on a null object. Here’s an example:
String str = null;
int length = str.length();
# Output:
# Exception in thread 'main' java.lang.NullPointerException
In this scenario, we’ve declared a string str
but haven’t assigned any value to it. When we try to use .length on str
, Java throws a NullPointerException because str
is null.
Solution: Always ensure the object you’re calling .length on is not null. You can do this with a simple conditional check:
if (str != null) {
int length = str.length();
}
ArrayIndexOutOfBoundsExceptions with .length
An ArrayIndexOutOfBoundsException can occur if you try to access an array element at an index that’s equal to or larger than the array’s length. Here’s an example:
int[] numbers = {1, 2, 3};
int number = numbers[3];
# Output:
# Exception in thread 'main' java.lang.ArrayIndexOutOfBoundsException: Index 3 out of bounds for length 3
In this example, we’re trying to access numbers[3]
, but numbers
only has three elements, with indices 0, 1, and 2. There’s no element at index 3, so Java throws an ArrayIndexOutOfBoundsException.
Solution: Always ensure your index is less than the array’s length. You can do this with a simple conditional check:
if (index < numbers.length) {
int number = numbers[index];
}
Best Practices for Using .length
These examples illustrate the importance of using .length carefully. Here are some best practices to avoid common issues:
- Always check if your array or string is not null before calling .length.
Always ensure your index is less than the array’s length before accessing an element.
Be mindful of off-by-one errors. Remember that array indices start at 0, not 1.
Understanding Arrays and Strings in Java
Before we delve deeper into the usage of .length, it’s crucial to have a solid understanding of arrays and strings in Java. These are fundamental data structures that you’ll encounter frequently in Java programming.
Arrays in Java
An array in Java is a static data structure that holds a fixed number of values of a single type. The length of an array is established when the array is created, and it cannot be changed.
int[] numbers = {1, 2, 3, 4, 5};
# Output:
# An array of integers with 5 elements
In this example, numbers
is an array that can hold five integers.
Strings in Java
A string in Java is an object that represents a sequence of characters. The length of a string is the number of characters it contains.
String str = 'Hello, World!';
# Output:
# A string of 13 characters, including spaces and punctuation
In this example, str
is a string that contains 13 characters, including spaces and punctuation.
Why is .length Important?
Knowing the length of arrays and strings is a fundamental aspect of programming. It allows you to control how you iterate through arrays and strings, helps prevent errors like ArrayIndexOutOfBoundsExceptions, and enables you to efficiently manipulate and process data.
Whether you’re sorting an array, reversing a string, or performing complex data processing tasks, understanding and correctly using .length in Java is a crucial skill.
Real-World Applications: The Relevance of .length in Java
While we’ve discussed .length in the context of arrays and strings, it’s important to understand its relevance in real-world programming and data processing tasks. When you’re working with large datasets or developing complex algorithms, knowing the size of your arrays and strings is crucial.
.length in Data Processing
In data processing, you often need to iterate through arrays or strings. Whether you’re cleaning data, transforming it, or analyzing it, the .length property is a fundamental tool. It allows you to control your loops and prevent errors like ArrayIndexOutOfBoundsExceptions.
.length in Algorithm Development
When developing algorithms, especially those dealing with data structures, the .length property is invaluable. It can help you sort arrays, search for elements, reverse strings, and more. Understanding and correctly using .length is a fundamental skill in algorithm development.
Exploring Related Concepts
As you continue your journey in Java programming, we recommend exploring related concepts like array manipulation and string handling. These topics will deepen your understanding of Java and strengthen your programming skills.
Further Resources for Mastering .length in Java
To help you further explore these concepts, here are some resources that you might find helpful:
- Java Variables Tutorial: Best Practices and Tips – Java variables explained in a comprehensive guide.
Mastering Final Usage in Java – Explore Java’s final keyword for enforcing immutability.
Var Keyword in Java Explained – Discover the var keyword in Java for type inference and variable declarations.
Oracle’s Java Tutorials includes detailed guides on arrays and strings.
GeeksforGeeks’ Java Programming Language Offers tutorials and exercises on Java data structures and algorithms.
Java Code Geeks provides a variety of articles on Java data processing and algorithm development.
Wrapping Up: Mastering .length in Java
In this comprehensive guide, we’ve explored how to use .length in Java to determine the size of arrays and strings. We’ve seen how .length can be a simple yet powerful tool in your Java programming toolkit, helping you efficiently manipulate and process data.
We began with the basics, learning how to use .length with arrays and strings. We then ventured into more advanced territory, exploring .length with multidimensional arrays and complex data structures. Along the way, we tackled common issues you might encounter when using .length, such as NullPointerExceptions and ArrayIndexOutOfBoundsExceptions, providing you with solutions and workarounds for each issue.
We also looked at alternative approaches to determining the length of arrays and strings in Java, such as using the size() method with collections. Here’s a quick comparison of these methods:
Method | Use Case | Pros | Cons |
---|---|---|---|
.length | Arrays and Strings | Straightforward and easy to use | Can lead to exceptions if not used carefully |
size() | Collections | Flexible, can be used with dynamic data structures | More complex than .length |
Whether you’re just starting out with Java or you’re looking to level up your programming skills, we hope this guide has given you a deeper understanding of .length and its capabilities.
With its balance of simplicity and power, .length is a fundamental tool for any Java programmer. Now, you’re well equipped to use .length in your Java programs. Happy coding!