Java String Replace(): Mastering the Method
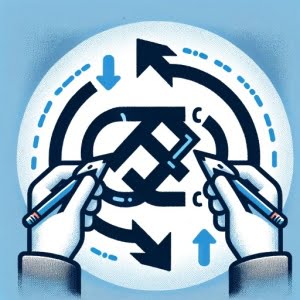
Ever found yourself wrestling with replacing parts of a string in Java? You’re not alone. Many developers find it a bit challenging to replace strings in Java, but we’re here to help.
Think of Java’s String class as a skilled surgeon, capable of precise replacements within your text. It’s a powerful tool that can help you manipulate and handle text data efficiently in your Java programs.
In this guide, we’ll walk you through the process of replacing strings in Java, from the basic usage of the replace() method to more advanced techniques. We’ll cover everything from making simple replacements to handling complex scenarios using regular expressions and alternative approaches.
So, let’s dive in and start mastering Java string replace!
TL;DR: How Do I Replace a String in Java?
In Java, you can use the replace() method of the String class to replace characters or substrings, like so:
String newText = oldText.replace('World', 'Java');
This method is a part of the String class and is used to create a new string by replacing specific parts of the original string.
Here’s a simple example:
String text = 'Hello, World!';
String newText = text.replace('World', 'Java');
System.out.println(newText);
# Output:
# 'Hello, Java!'
In this example, we have a string ‘Hello, World!’. We use the replace() method to replace the substring ‘World’ with ‘Java’. The result is a new string ‘Hello, Java!’.
This is a basic way to replace a string in Java, but there’s much more to learn about string manipulation in Java. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Understanding Java String Replace: The Basics
- Delving Deeper: Java String Replace with Regular Expressions
- Exploring Alternatives: StringBuilder and Third-Party Libraries
- Troubleshooting Java String Replace: Common Issues and Solutions
- Understanding the Fundamentals: Java’s String Class, Immutability and Regular Expressions
- The Bigger Picture: Relevance of String Replacement
- Wrapping Up: Mastering Java String Replace
Understanding Java String Replace: The Basics
The replace() method is a part of the String class in Java. It’s used to replace each occurrence of a specified character or substring with a new character or substring.
The method comes in two forms:
- replace(char oldChar, char newChar)
- replace(CharSequence target, CharSequence replacement)
The first form replaces all occurrences of a specified ‘oldChar’ with a new ‘newChar’. The second form replaces a sequence of characters ‘target’ with another sequence ‘replacement’.
Let’s look at a simple example:
String str = 'Hello, World!';
String newStr = str.replace('World', 'Java');
System.out.println(newStr);
# Output:
# 'Hello, Java!'
In this code, we’ve replaced ‘World’ with ‘Java’ in the original string ‘Hello, World!’. The replace() method returns a new string ‘Hello, Java!’, leaving the original string unchanged.
Note: Strings in Java are immutable, meaning they cannot be changed once created. So, the replace() method doesn’t modify the original string but creates a new one.
The replace() method is straightforward and easy to use, making it a go-to choice for simple string replacements. However, it might not be the best choice for complex replacements or when performance is a concern, as creating new strings can be costly in terms of memory and processing time.
Delving Deeper: Java String Replace with Regular Expressions
As you gain more experience with Java, you’ll often encounter situations where simple string replacements aren’t enough. This is where the replaceAll() method comes in handy.
The replaceAll() method works similarly to the replace() method but with a significant difference: it uses regular expressions (regex), allowing you to perform more complex replacements.
Here’s a basic example of using replaceAll() with regular expressions:
String str = 'Hello, World! World, meet Java.';
String newStr = str.replaceAll('World', 'Java');
System.out.println(newStr);
# Output:
# 'Hello, Java! Java, meet Java.'
In this example, replaceAll() replaces all occurrences of ‘World’ with ‘Java’. But the real power of replaceAll() emerges when you use it with regular expressions. For instance, you can use it to replace all digits in a string with X’s, like so:
String str = 'My phone number is 123-456-7890.';
String newStr = str.replaceAll('\d', 'X');
System.out.println(newStr);
# Output:
# 'My phone number is XXX-XXX-XXXX.'
In this code, ‘\d’ is a regular expression that matches any digit. The replaceAll() method replaces each digit in the string with ‘X’.
Note: In Java, backslashes in strings need to be escaped with another backslash, hence ‘\d’ instead of ‘\d’.
While replaceAll() offers more flexibility, it can be slower than replace() due to the overhead of processing regular expressions. Therefore, it’s best to use replace() for simple replacements and save replaceAll() for when you need the extra power of regular expressions.
Exploring Alternatives: StringBuilder and Third-Party Libraries
While Java’s String class provides robust methods for string replacement, there are alternative approaches that can offer more efficiency or flexibility, especially in complex scenarios or large-scale applications. Two such alternatives are using the StringBuilder class and incorporating third-party libraries.
Harnessing StringBuilder for String Replacement
StringBuilder is a mutable sequence of characters, meaning it can be modified after it’s created. This mutable nature makes StringBuilder a more performance-friendly alternative for string replacement in certain scenarios.
Here’s an example of using StringBuilder to replace a character in a string:
StringBuilder str = new StringBuilder('Hello, World!');
int index = str.indexOf('World');
if (index != -1) {
str.replace(index, index + 'World'.length(), 'Java');
}
System.out.println(str);
# Output:
# 'Hello, Java!'
In this code, we first find the index of ‘World’ in the string. If ‘World’ is found (i.e., index != -1), we replace it with ‘Java’ using the replace() method of StringBuilder.
Leveraging Third-Party Libraries for Advanced Replacements
Third-party libraries like Apache Commons Lang and Google’s Guava provide powerful utilities for string manipulation, including string replacement. These libraries can handle complex replacements and offer additional features not available in the standard Java String class.
Here’s an example of using the StringUtils class from Apache Commons Lang to replace a string:
import org.apache.commons.lang3.StringUtils;
String str = 'Hello, World!';
String newStr = StringUtils.replace(str, 'World', 'Java');
System.out.println(newStr);
# Output:
# 'Hello, Java!'
In this example, StringUtils.replace() works similarly to String.replace(), but it’s part of a larger suite of string utilities provided by Apache Commons Lang.
While these alternatives can be powerful, they also add complexity and dependencies to your project. Therefore, it’s recommended to stick with the standard String methods for simple replacements and only resort to these alternatives when necessary.
Troubleshooting Java String Replace: Common Issues and Solutions
While Java’s string replace methods are powerful, they can sometimes lead to unexpected results. Let’s discuss some common issues you might encounter and how to solve them.
Dealing with Special Characters in Regular Expressions
One common issue when using the replaceAll() method involves special characters in regular expressions. For instance, if you try to replace ‘.’ (which is a special character in regex matching any character), you might get unexpected results.
String str = 'Hello, World.';
String newStr = str.replaceAll('.', '!');
System.out.println(newStr);
# Output:
# '!!!!!!!!!!!!!!'
In this example, instead of replacing the period at the end of the string, replaceAll() replaces every character with ‘!’. This is because ‘.’ is a special character in regular expressions.
To solve this, you can escape special characters using ‘\’.
String str = 'Hello, World.';
String newStr = str.replaceAll('\\.', '!');
System.out.println(newStr);
# Output:
# 'Hello, World!'
In this corrected code, replaceAll() correctly replaces the period at the end of the string with ‘!’.
Using replace() with Null Values
Another common issue involves using the replace() method with null values. If you try to replace a substring with null, replace() throws a NullPointerException.
String str = 'Hello, World!';
String newStr = str.replace('World', null);
System.out.println(newStr);
# Output:
# Exception in thread 'main' java.lang.NullPointerException
In this example, trying to replace ‘World’ with null results in a NullPointerException. To avoid this, always ensure the replacement value is not null before using the replace() method.
These are just a couple of examples of issues you might encounter when replacing strings in Java. Always remember to test your code thoroughly and understand the nuances of the methods you’re using.
Understanding the Fundamentals: Java’s String Class, Immutability and Regular Expressions
Before we delve further into string replacements in Java, it’s essential to understand some fundamental concepts: Java’s String class, the immutability of strings, and regular expressions.
Java’s String Class
The String class in Java is used to create and manipulate strings. It provides several methods for string manipulation, including replace(), replaceAll(), and replaceFirst() for replacing characters or substrings.
String str = 'Hello, World!';
System.out.println(str);
# Output:
# 'Hello, World!'
In this example, we create a string using the String class and print it to the console.
Immutability of Strings
In Java, strings are immutable, meaning they cannot be changed once created. When you perform an operation on a string, like replacing a substring, Java doesn’t modify the original string. Instead, it creates a new string with the changes.
String str = 'Hello, World!';
String newStr = str.replace('World', 'Java');
System.out.println(str);
System.out.println(newStr);
# Output:
# 'Hello, World!'
# 'Hello, Java!'
In this code, even though we replace ‘World’ with ‘Java’, the original string ‘Hello, World!’ remains unchanged. The replace() method returns a new string ‘Hello, Java!’.
The Concept of Regular Expressions
Regular expressions (regex) are sequences of characters that form a search pattern. They’re used for pattern matching with strings, and string searching and replacing. In Java, you can use regular expressions with the replaceAll() method of the String class.
String str = 'Hello, World! World, meet Java.';
String newStr = str.replaceAll('World', 'Java');
System.out.println(newStr);
# Output:
# 'Hello, Java! Java, meet Java.'
In this example, replaceAll() uses the regular expression ‘World’ to replace all occurrences of ‘World’ with ‘Java’.
These fundamental concepts are key to understanding how string replacement works in Java. With this foundation, you can better understand and use Java’s string replace methods.
The Bigger Picture: Relevance of String Replacement
String replacement in Java isn’t just a programming exercise. It has significant practical applications in various areas, including text processing, data cleaning, and more. Understanding how to replace strings in Java can help you manipulate and clean data, find and replace patterns in text, and even build complex software applications.
String Replacement in Text Processing
Text processing is a common task in many areas of software development, from web development to data science. Whether you’re building a web scraper, analyzing text data, or developing a natural language processing (NLP) model, you’ll often need to replace certain strings in your text data. Java’s string replace methods can help you do this efficiently and effectively.
Data Cleaning with Java String Replace
Data cleaning is another area where string replacement comes in handy. In data science, you often work with large datasets that may contain errors, inconsistencies, or unwanted characters. Using Java’s string replace methods, you can clean your data by replacing these unwanted strings with appropriate values.
Exploring Related Concepts
While string replacement is a powerful tool, it’s just one part of the broader field of string manipulation in Java. To deepen your understanding, you might want to explore related concepts like string concatenation, string splitting, and string conversion. Regular expressions, which we touched on in this guide, are another powerful tool for string manipulation that you might want to delve deeper into.
Further Resources for Mastering Java String Manipulation
Here are some resources that can help you further explore and master string manipulation in Java:
- Beginner’s Guide to the String Class in Java – Learn Java String methods and operations.
Reverse String in Java: Techniques – Learn how to reverse strings in Java using different methods.
Exploring String Split in Java – Learn about specifying delimiters and limiting the number of splits.
Official Java Tutorials by Oracle provides a comprehensive guide on the String class and its methods.
Java replace() method by TutorialsPoint gives a clear and concise explanation of the .replace() method.
Java String replace() method by Programiz provides a simple and beginner-friendly explanation of the .replace() method in Java.
Wrapping Up: Mastering Java String Replace
In this comprehensive guide, we’ve delved deep into the process of replacing strings in Java. We’ve explored the various methods provided by Java’s String class, their usage, and their implications in different scenarios.
We began with the basics, understanding how to use the replace() method for simple string replacements. We then advanced to more complex replacements using the replaceAll() method with regular expressions, providing you with the ability to handle a variety of string replacement scenarios. Along the way, we’ve also discussed alternative approaches like using StringBuilder and third-party libraries for more efficient or complex replacements.
Throughout our journey, we’ve tackled common issues you might encounter when replacing strings in Java, such as dealing with special characters in regular expressions and handling null values. For each issue, we’ve provided solutions and tips to help you navigate these challenges.
Here’s a quick comparison of the methods we’ve discussed:
Method | Flexibility | Performance | Complexity |
---|---|---|---|
replace() | Moderate | High | Low |
replaceAll() | High | Moderate | Moderate |
StringBuilder | High | High | High |
Third-Party Libraries | High | Varies | High |
Whether you’re a beginner just starting out with Java or an experienced developer looking to level up your string manipulation skills, we hope this guide has given you a deep understanding of Java string replace and its various applications.
With this knowledge, you’re now equipped to handle a wide range of string replacement scenarios in your Java programs. Happy coding!