Java JFrame Class: Syntax Guide and Code Examples
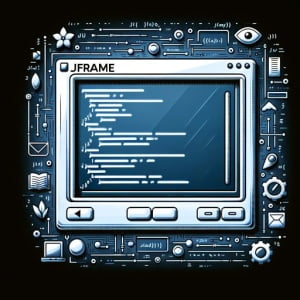
Are you grappling with usin the Java Swing JFrame class? You’re not alone. Many developers find themselves in a maze when it comes to building graphical user interfaces in Java, but we’re here to help.
Think of JFrame as a master architect’s blueprint – it’s a foundation upon which we can construct intuitive and interactive GUIs in Java. It’s a part of the Swing library, which is built atop Java’s original abstract window toolkit (AWT).
This guide will walk you through the process of using JFrame to create GUIs in Java. We’ll cover everything from the basics of JFrame, such as setting up your first window, to more advanced techniques like handling events and creating complex layouts.
So, let’s roll up our sleeves and start mastering JFrame in Java!
TL;DR: How Do I Utilize the JFrame Class in Java?
To use the
JFrame
class, you start by creating an instance ofJFrame
withJFrame frame = new JFrame();
, setting its size and visibility, such asframe.setSize(400, 400);
andframe.setVisible(true);
, and then adding components to it.
Here’s a simple example:
JFrame frame = new JFrame();
frame.setSize(400, 400);
frame.setVisible(true);
// Output:
// This will create a simple window.
In this example, we first create an instance of JFrame. We then set its size to 400×400 pixels using the setSize
method. Finally, we make the JFrame visible by calling the setVisible
method with true
as the argument.
This is a basic way to create a GUI using JFrame in Java, but there’s much more to learn about creating and manipulating GUIs with JFrame. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
Building Your First JFrame
Creating a JFrame is the first step towards building a GUI in Java. Let’s walk through this process together, focusing on the basic properties like size, title, and visibility. We’ll also add some simple components like buttons and labels to our JFrame.
Creating a JFrame
To create a JFrame, you simply need to create an instance of the JFrame class. Here’s how you do it:
JFrame frame = new JFrame();
This line of code creates a new instance of JFrame and assigns it to the variable frame
.
Setting JFrame Properties
Once you’ve created a JFrame, you can set its properties. Let’s start by setting the size and title of our JFrame:
frame.setSize(500, 500);
frame.setTitle("My First JFrame");
In the first line, we’re setting the size of the JFrame to be 500×500 pixels. In the second line, we’re setting the title of the JFrame to be ‘My First JFrame’.
Making JFrame Visible
By default, the JFrame is not visible. To make it visible, you need to call the setVisible
method and pass true
as an argument:
frame.setVisible(true);
This line of code makes the JFrame visible.
Adding Components to JFrame
Now, let’s add a button and a label to our JFrame. Here’s how you do it:
JButton button = new JButton("Click Me!");
JLabel label = new JLabel("Hello, JFrame!");
frame.add(button);
frame.add(label);
In this block of code, we first create a button with the text ‘Click Me!’ and a label with the text ‘Hello, JFrame!’. We then add these components to the JFrame using the add
method.
And that’s it! You’ve just created your first JFrame in Java and added some components to it. Remember, the key to mastering JFrame (and GUI creation in general) is practice. So, don’t hesitate to experiment with different settings and components.
Exploring Alternative GUI Creation Techniques
While JFrame is a powerful tool for creating GUIs in Java, it’s not the only option. Other methods, such as using JavaFX or AWT, can also be used to create GUIs. Let’s explore these alternatives.
JavaFX: The Modern Approach
JavaFX is a modern GUI toolkit introduced in Java 7 to replace Swing. It’s designed to provide a lightweight, hardware-accelerated Java UI platform.
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.stage.Stage;
public class HelloWorld extends Application {
public static void main(String[] args) {
launch(args);
}
@Override
public void start(Stage primaryStage) {
Button btn = new Button();
btn.setText("Say 'Hello World'");
btn.setOnAction(event -> System.out.println("Hello World!"));
Scene scene = new Scene(btn, 300, 250);
primaryStage.setTitle("Hello World!");
primaryStage.setScene(scene);
primaryStage.show();
}
}
// Output:
// When the button is clicked, "Hello World!" is printed to the console.
This JavaFX example creates a simple ‘Hello World’ application with a clickable button. When the button is clicked, “Hello World!” is printed to the console.
JavaFX provides a clean and modern API, but requires more boilerplate code than Swing. It’s a good choice for complex, large-scale GUI applications.
AWT: The Original GUI Toolkit
AWT (Abstract Window Toolkit) is Java’s original platform-dependent GUI toolkit, preceding Swing and JavaFX. It provides a set of components that are rendered using each platform’s native GUI toolkit, ensuring a consistent look and feel across all platforms.
import java.awt.*;
import java.awt.event.*;
public class AWTCounter extends Frame {
private Label lblCount;
private TextField tfCount;
private Button btnCount;
private int count = 0;
public AWTCounter () {
setLayout(new FlowLayout());
lblCount = new Label("Counter");
add(lblCount);
tfCount = new TextField(count + "", 10);
tfCount.setEditable(false);
add(tfCount);
btnCount = new Button("Count");
add(btnCount);
BtnCountListener listener = new BtnCountListener();
btnCount.addActionListener(listener);
setTitle("AWT Counter");
setSize(250, 100);
setVisible(true);
}
public static void main(String[] args) {
AWTCounter app = new AWTCounter();
}
private class BtnCountListener implements ActionListener {
public void actionPerformed(ActionEvent evt) {
++count;
tfCount.setText(count + "");
}
}
}
// Output:
// When the button is clicked, the counter increases.
This AWT example creates a simple counter application. When the ‘Count’ button is clicked, the counter increases.
AWT is simple and easy to use, but lacks the sophisticated features and flexibility of Swing and JavaFX. It’s a good choice for simple, small-scale GUI applications.
When choosing between JFrame, JavaFX, and AWT, consider your application’s requirements, your team’s expertise, and the specific features and drawbacks of each toolkit.
Troubleshooting Common JFrame Issues
While JFrame is a powerful tool for creating GUIs in Java, like any tool, it can sometimes be tricky to use. Let’s explore some common issues that developers often encounter when using JFrame, along with their solutions.
Handling Window Closing Events
One common issue when working with JFrame is handling window closing events. By default, clicking the close button on a JFrame does not actually terminate the Java program. Here’s how you can make it do so:
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
This line of code sets the default close operation for the JFrame. When the JFrame is closed, it will terminate the Java program.
Dealing with Thread Issues
Swing, and by extension JFrame, is not thread-safe. This means that you should only modify Swing components (like JFrame) on the Event Dispatch Thread. Here’s how you can ensure that your JFrame code executes on the Event Dispatch Thread:
SwingUtilities.invokeLater(new Runnable() {
public void run() {
// Your JFrame code here
}
});
This code schedules the enclosed Runnable to be executed on the Event Dispatch Thread. This ensures that your JFrame code is executed in a thread-safe manner.
Resolving Resizing Issues
Another common issue with JFrame is dealing with resizing issues. By default, a JFrame can be resized by the user. If you want to prevent the user from resizing the JFrame, you can disable resizing:
frame.setResizable(false);
This line of code makes the JFrame non-resizable.
These are just a few of the common issues that you might encounter when using JFrame. Remember, every problem has a solution, so don’t be discouraged if you encounter issues. Happy coding!
The Fundamentals of GUI Creation in Java
Java, being a versatile and widely-used language, provides several ways to create GUIs. Let’s delve into the fundamentals of GUI creation in Java and the role JFrame plays in it.
GUI Creation in Java: The Basics
The process of creating a GUI (Graphical User Interface) in Java involves creating a container (like a JFrame), adding components (like buttons and text fields) to the container, and handling events (like button clicks).
JFrame frame = new JFrame("My GUI");
JButton button = new JButton("Click me");
frame.add(button);
frame.setSize(300, 300);
frame.setVisible(true);
In this simple example, we first create a JFrame with the title ‘My GUI’. We then create a button with the text ‘Click me’ and add it to the JFrame. Finally, we set the size of the JFrame and make it visible.
Understanding the Swing Library
Swing is a GUI widget toolkit for Java. It’s part of Oracle’s Java Foundation Classes (JFC) — an API for providing a graphical user interface for Java programs. Swing was developed to provide a more sophisticated set of GUI components than the earlier Abstract Window Toolkit (AWT).
Swing provides a native look and feel that emulates the look and feel of several platforms, and also supports a pluggable look and feel that allows applications to have a look and feel unrelated to the underlying platform.
The Role of JFrame in GUI Creation
In the Swing library, JFrame is used to create a window. This window serves as a top-level container that houses various GUI components like buttons, labels, text fields, etc.
JFrame frame = new JFrame("My JFrame");
frame.setSize(400, 400);
frame.setVisible(true);
In this code snippet, we create a JFrame with the title ‘My JFrame’, set its size to 400×400 pixels, and make it visible. This JFrame can now be used to hold various GUI components.
JFrame plays a crucial role in GUI creation in Java. Understanding how to use JFrame effectively is key to building robust and interactive GUIs in Java.
Expanding JFrame Usage in Larger Projects
As your skills in Java GUI creation grow, you’ll likely find yourself working on larger projects. In these scenarios, JFrame can be used to create complex applications with multiple windows and dialogs. This section will discuss these advanced applications and suggest related topics for further study.
Building Complex Applications with Multiple Windows
In larger applications, you might need to create multiple windows. In Java, you can create multiple instances of JFrame for this purpose. Each JFrame can act as an independent window, each with its own set of components and event handlers.
JFrame frame1 = new JFrame("Window 1");
frame1.setSize(300, 300);
frame1.setVisible(true);
JFrame frame2 = new JFrame("Window 2");
frame2.setSize(300, 300);
frame2.setVisible(true);
In this example, we create two JFrames, each representing a separate window. Each window can be manipulated independently of the other.
Creating Dialogs in JFrame
Dialogs are another important aspect of GUI applications. They’re typically used to capture user input or display messages. Here’s how you can create a simple dialog with JFrame:
JOptionPane.showMessageDialog(frame, "This is a dialog message");
This line of code creates a simple message dialog that displays the text ‘This is a dialog message’.
Further Topics for Exploration
As you continue to explore JFrame and Swing, there are several related topics that you might find interesting:
- Event handling: Learn how to handle various types of events, such as mouse clicks and keyboard input.
- Custom painting: Discover how to customize the appearance of your GUI components using custom painting.
- Multithreading in Swing: Understand how to create responsive GUIs by performing long-running tasks on a separate thread.
Further Resources for JFrame
If you’re interested in learning more about JFrame and Java GUI creation, here are a few resources that you might find helpful:
- Tutorial on Java Classes can help you by IOFlood is your roadmap to Java proficiency.
CompletableFuture in Java: A Complete Overview – Explore asynchronous programming and task handling in Java.
JList in Java: Exploring List Component – Understand displaying and selecting items in a list format with JList in Java.
Java Swing JFrame Tutorial by CodeJava explains creating windows in GUI applications with Java Swing JFrame.
JFrame Oracle Official Documentation by Oracle provides comprehensive information on using JFrame in Java Swing.
Java JFrame Tutorial by JavaTpoint covers creating frames, adding components, and customizing JFrame in Java Swing.
Wrapping Up: JFrame Usage GUide
In this comprehensive guide, we’ve delved deep into the world of JFrame, a powerful tool for creating graphical user interfaces (GUIs) in Java.
We began with the basics, learning how to create a JFrame, set its properties, and add components to it. We then ventured into more advanced territory, exploring how to use layout managers, handle events, and create menus in JFrame. We also tackled common challenges you might face when using JFrame, such as handling window closing events, dealing with thread issues, and resolving resizing issues, providing you with solutions for each problem.
We also looked at alternative approaches to GUI creation in Java, comparing JFrame with other methods like JavaFX and AWT. Here’s a quick comparison of these methods:
Method | Ease of Use | Flexibility | Suitability for Large Projects |
---|---|---|---|
JFrame | High | High | High |
JavaFX | Moderate | Very High | Very High |
AWT | High | Moderate | Low |
Whether you’re just starting out with JFrame or you’re looking to level up your GUI creation skills, we hope this guide has given you a deeper understanding of JFrame and its capabilities.
With its balance of ease of use, flexibility, and suitability for large projects, JFrame is a powerful tool for GUI creation in Java. Now, you’re well equipped to create intuitive and interactive GUIs for your Java applications. Happy coding!