Bash ‘Exec’ Command Guide: Scripting Syntax and Uses
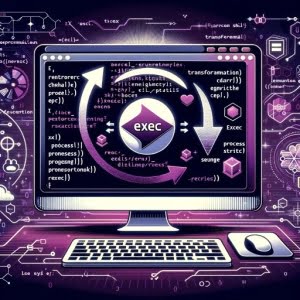
Are you finding the ‘exec’ command in Bash a bit puzzling? You’re not alone. Many developers find themselves in a maze when it comes to using the ‘exec’ command in Bash. Think of ‘exec’ as a chameleon, seamlessly replacing the current shell process with a new one, adding a new layer of versatility to your scripting.
The ‘exec’ command is a powerful tool in Bash scripting, allowing you to replace the current shell process and execute commands, making it an essential tool for any Bash user.
In this guide, we will help you understand and master the ‘exec’ command in Bash, from its basic usage to advanced techniques. We’ll cover everything from how to use ‘exec’ in simple scenarios, to complex uses such as using it with file descriptors or in scripts. We’ll also delve into common issues and their solutions.
So, let’s dive in and start mastering the ‘exec’ command in Bash!
TL;DR: How Do I Use the ‘exec’ Command in Bash?
The
'exec'
command in Bash is used to replace the current shell process with a new one. It is used with the syntax,exec [command]
. It’s like swapping out the engine of a running car with a new one, without having to stop the car.
Here’s a simple example:
exec ls
# Output:
# [Expected output from the 'ls' command]
In this example, we use the ‘exec’ command to replace the current shell with the ‘ls’ command. The ‘ls’ command lists the contents of the current directory, so the output will be the list of files and directories in the current directory.
This is a basic way to use the ‘exec’ command in Bash, but there’s much more to learn about this powerful command. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Understanding the Basics of Bash ‘exec’ Command
- Diving Deeper: Advanced Use of ‘exec’
- Exploring Alternatives to ‘exec’
- Troubleshooting Common ‘exec’ Issues
- Understanding Process Replacement in Unix-like Systems
- Expanding Horizons: ‘exec’ in Larger Scripts and Projects
- Wrapping Up: Mastering Bash ‘exec’ Command
Understanding the Basics of Bash ‘exec’ Command
The ‘exec’ command in Bash is a built-in utility that allows you to replace the current shell process with a new one. The new process could be any command that you want to execute. Once the command finishes executing, the shell process ends.
Let’s look at a simple example to understand how this works:
exec echo 'Hello, World!'
# Output:
# 'Hello, World!'
In this example, we use the ‘exec’ command to replace the current shell with the ‘echo’ command, which prints ‘Hello, World!’ to the terminal. Once the ‘echo’ command finishes executing, the shell process ends.
The ‘exec’ command is a powerful tool, but it can be a double-edged sword. The key advantage of using ‘exec’ is that it allows you to replace the current shell without creating a new one, which can be more efficient in terms of system resources. However, the potential pitfall is that once the ‘exec’ command is executed, the current shell ends. This means that any commands following the ‘exec’ command in a script would not be executed.
In the next section, we’ll delve into more advanced uses of the ‘exec’ command.
Diving Deeper: Advanced Use of ‘exec’
The ‘exec’ command in Bash can be used in more complex scenarios beyond simply replacing the current shell process. Let’s explore how to use ‘exec’ with file descriptors and in scripts.
Exec with File Descriptors
In Bash, ‘exec’ can also be used to manipulate file descriptors. For instance, you can use ‘exec’ to redirect output to a file. Here’s an example:
exec 3>&1
echo 'Hello, World!' >&3
# Output:
# 'Hello, World!'
In this example, we use ‘exec’ to create a new file descriptor (3) that points to the same location as the standard output (1). Then, we use ‘echo’ to write ‘Hello, World!’ to this new file descriptor, which effectively prints the message to the terminal.
Exec in Scripts
‘exec’ can also be used in scripts to replace the script’s process with another command. This can be useful in scenarios where you want the script to end immediately after executing a certain command. However, remember that using ‘exec’ in this way means that any commands following ‘exec’ in the script will not be executed, as the script’s process ends when ‘exec’ is called.
Here’s an example of how ‘exec’ can be used in a script:
#!/bin/bash
echo 'This will run.'
exec echo 'This will also run, and then the script ends.'
echo 'This will not run.'
# Output:
# 'This will run.'
# 'This will also run, and then the script ends.'
In this script, the ‘exec’ command replaces the script’s process with the ‘echo’ command. As a result, the final ‘echo’ command does not run.
Using ‘exec’ in this way can be a powerful tool, but it should be used with caution. On the one hand, it can make your scripts more efficient by avoiding the creation of additional subshells. On the other hand, it can lead to unexpected behavior if you’re not careful, as any commands following ‘exec’ will not be executed.
Exploring Alternatives to ‘exec’
While ‘exec’ is a powerful tool in Bash, there are alternative methods to accomplish similar tasks. Let’s delve into some of these alternatives and understand their benefits and drawbacks.
Using Subshells
One alternative to using ‘exec’ to replace the current shell process is to use subshells. A subshell is a child process launched by a shell (or shell script). Here’s an example of how you can use a subshell to run a command:
(echo 'Hello, World!')
# Output:
# 'Hello, World!'
In this example, we use a subshell to run the ‘echo’ command. Unlike ‘exec’, this does not replace the current shell process, so any commands following the subshell in a script would still be executed.
The benefit of using subshells is that they allow you to execute commands without terminating the current shell process. However, they do create an additional process, which can be less efficient than using ‘exec’.
Using ‘nohup’ and ‘&’ for Background Execution
If you want to execute a command but keep your current script running, you can use the ‘nohup’ command or the ‘&’ operator. Here’s an example:
nohup echo 'Hello, World!' &
# Output:
# 'nohup: ignoring input and appending output to ‘nohup.out’'
In this example, we use ‘nohup’ and ‘&’ to run the ‘echo’ command in the background. This allows the current script to continue running, even if the command takes a long time to complete.
The benefit of this approach is that it allows you to execute commands without interrupting the flow of your script. However, it can be more complex to manage background processes, especially in larger scripts.
In conclusion, while ‘exec’ is a powerful tool, there are alternative approaches that can be used depending on your specific needs. The key is to understand the trade-offs and choose the approach that best suits your situation.
Troubleshooting Common ‘exec’ Issues
Like any command, using ‘exec’ in Bash can sometimes lead to errors or unexpected behavior. Let’s explore some common obstacles you might encounter when using ‘exec’ and discuss how to overcome them.
Command Not Found Error
A common error you might encounter when using ‘exec’ is the ‘command not found’ error. This typically happens when you try to ‘exec’ a command that doesn’t exist or isn’t in your PATH.
exec nonexistentcommand
# Output:
# 'bash: exec: nonexistentcommand: not found'
In this example, we try to ‘exec’ a command that doesn’t exist, leading to a ‘command not found’ error. To solve this issue, make sure the command you’re trying to ‘exec’ is installed and in your PATH.
Ignoring Commands After ‘exec’
Another issue you might encounter when using ‘exec’ is that any commands after ‘exec’ in a script are ignored. This is because ‘exec’ replaces the current shell process, so any subsequent commands in the same shell or script won’t be executed.
exec echo 'Hello, World!'
echo 'This will not run.'
# Output:
# 'Hello, World!'
In this example, the second ‘echo’ command is ignored because the ‘exec’ command replaces the current shell process.
To avoid this issue, only use ‘exec’ when you want to end the current shell process. If you need to continue executing commands in the same shell or script, consider using a subshell or background process instead.
Best Practices and Optimization
When using ‘exec’, there are a few best practices to keep in mind. First, remember that ‘exec’ replaces the current shell process, so use it sparingly and only when necessary. Second, always check the command you’re trying to ‘exec’ is valid and in your PATH to avoid ‘command not found’ errors. Finally, consider using alternatives like subshells or background processes if you need to continue executing commands after ‘exec’.
Understanding Process Replacement in Unix-like Systems
To fully grasp the ‘exec’ command in Bash, it’s crucial to understand the concept of process replacement in Unix-like operating systems. This foundational concept is the bedrock upon which ‘exec’ operates.
In Unix-like systems, every running program is a process. Each process has a unique identifier known as the Process ID (PID). When you execute a command or script in Bash, a new process is created for that command or script. This new process runs alongside the original shell process.
However, there are situations where you might want to replace the current shell process with a new one, rather than creating a new process alongside it. This is where ‘exec’ comes in.
The ‘exec’ command replaces the current shell process with a new one. The new process uses the same PID as the original process, because it’s not a new process running alongside the original, but a replacement of the original.
Here’s a simple example to illustrate this concept:
echo $$
exec bash
echo $$
# Output:
# 12345
# 12345
In this example, the echo $$
command prints the PID of the current shell process. When we execute exec bash
, the current shell process is replaced with a new Bash process. However, when we print the PID again, it’s still the same as before, because the original process was replaced, not supplemented.
Understanding this concept of process replacement is key to mastering the ‘exec’ command. It’s the underlying principle that allows ‘exec’ to work as it does, and it’s a fundamental part of how Unix-like operating systems manage processes.
Expanding Horizons: ‘exec’ in Larger Scripts and Projects
The ‘exec’ command isn’t just for small, isolated tasks. It can play a significant role in larger scripts or projects. Its ability to replace the current shell process with a new one can be leveraged in various ways to optimize the performance of your scripts.
For example, you might use ‘exec’ in a script that needs to execute a command and then end immediately, without any further processing. This can reduce the overhead of creating a new shell process and make your script more efficient.
Complementary Commands and Functions
The ‘exec’ command often doesn’t work alone. There are several other commands and functions in Bash that often accompany ‘exec’ in typical use cases.
One such command is ‘trap’. The ‘trap’ command allows you to specify commands that will be executed when a signal is received. This can be useful in conjunction with ‘exec’, as you can use ‘trap’ to clean up before the ‘exec’ command replaces the current shell process.
Here’s an example of how ‘trap’ can be used with ‘exec’:
trap 'rm -f /tmp/tempfile' EXIT
exec somecommand
# Output:
# [Expected output from 'somecommand']
In this example, the ‘trap’ command sets up a command to remove a temporary file when the shell exits. Then, the ‘exec’ command replaces the current shell process with ‘somecommand’. When ‘somecommand’ finishes executing and the shell exits, the ‘trap’ command is triggered, and the temporary file is removed.
Further Resources for Mastering ‘exec’
To delve deeper into the ‘exec’ command and related topics, you might find the following resources helpful:
- Bash Reference Manual: The official Bash Reference Manual provides a comprehensive guide to all the built-in commands in Bash, including ‘exec’.
The Linux Command Line: This book by William Shotts provides a thorough introduction to the command line in Linux, including a detailed discussion of shell processes and how commands like ‘exec’ work.
Advanced Bash-Scripting Guide: This guide provides an in-depth look at scripting in Bash, including advanced topics like process management and file descriptors.
Wrapping Up: Mastering Bash ‘exec’ Command
In this comprehensive guide, we’ve explored the ‘exec’ command in Bash, a potent tool that allows you to replace the current shell process with a new one.
We began with the basics, understanding how to use ‘exec’ in simple scenarios, and then ventured into more advanced territory, exploring the use of ‘exec’ with file descriptors and in scripts. We also delved into common issues that you might encounter when using ‘exec’ and provided solutions to help you troubleshoot these problems.
We also took a detour to explore alternative approaches to process replacement, such as using subshells or background processes, giving you a sense of the broader landscape of tools available in Bash.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
exec | Efficient, replaces the current shell process | Ends the current shell process |
Subshells | Allows execution of commands without terminating the current shell process | Creates an additional process |
Background processes | Allows execution of commands without interrupting the flow of the script | More complex to manage |
Whether you’re just starting out with ‘exec’ or you’re looking to deepen your understanding, we hope this guide has given you a comprehensive understanding of the ‘exec’ command in Bash.
The ‘exec’ command is a powerful tool in Bash, offering a level of control and efficiency that can be crucial in larger scripts or projects. Now, you’re well equipped to harness the power of ‘exec’. Happy scripting!