String Manipulation in Bash Script: A Linux Shell Guide
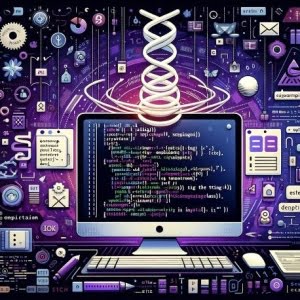
Are you finding it challenging to manipulate strings in Bash? You’re not alone. Many developers grapple with this task, but there are various tools that can make this process a breeze.
Bash offers a toolbox of features to shape and mold strings to your needs. These features can be used to perform various tasks, such as substring extraction, pattern replacement, and string length calculation.
This guide will walk you through the essentials of string manipulation in Bash, from basic techniques to advanced features. We’ll explore Bash’s core functionality, delve into its advanced features, and even discuss common issues and their solutions.
So, let’s dive in and start mastering string manipulation in Bash!
TL;DR: How Do I Manipulate Strings in Bash?
Bash provides several built-in features for string manipulation, such as pattern replacement, string length calculation, and
substring
extraction.substring
extraction can be used with the syntax,echo ${string:index:length}
Here’s a simple example of substring extraction:
string='Hello, World!'
echo ${string:7:5}
# Output:
# 'World'
In this example, we’ve used Bash’s substring extraction feature to extract a part of the string. The numbers inside the curly braces following the variable name ‘string’ represent the starting index and the length of the substring. In this case, ‘7’ is the starting index and ‘5’ is the length, so it extracts ‘World’ from ‘Hello, World!’.
This is just a basic way to manipulate strings in Bash, but there’s much more to learn about Bash’s string manipulation capabilities. Continue reading for more detailed explanations and advanced techniques.
Table of Contents
- Bash String Manipulation: The Basics
- Bash String Manipulation: Advanced Techniques
- Alternative Methods for Bash String Manipulation
- Troubleshooting Bash String Manipulation
- Bash: A Powerful Tool for String Manipulation
- Exploring the Relevance of Bash String Manipulation
- Wrapping Up: Mastering Bash String Manipulation
Bash String Manipulation: The Basics
Let’s start with the basics of string manipulation in Bash. We’ll cover string concatenation, substring extraction, and string length calculation. These are the fundamental techniques you’ll use frequently when working with strings in Bash.
String Concatenation in Bash
Concatenation is the process of joining two or more strings together. In Bash, you can concatenate strings without any special operators.
string1='Hello,'
string2=' World!'
concatenated=$string1$string2
echo $concatenated
# Output:
# 'Hello, World!'
In this example, we’ve concatenated ‘Hello,’ and ‘ World!’ to produce ‘Hello, World!’.
Substring Extraction in Bash
Bash allows you to extract a substring from a string. This can be useful when you need to parse a string to get specific information.
string='Hello, World!'
substring=${string:7:5}
echo $substring
# Output:
# 'World'
In this example, we’ve extracted ‘World’ from ‘Hello, World!’. The numbers inside the curly braces represent the starting index and the length of the substring.
String Length Calculation in Bash
You can calculate the length of a string using the ‘#’ operator in Bash.
string='Hello, World!'
length=${#string}
echo $length
# Output:
# 13
In this example, we’ve calculated the length of ‘Hello, World!’, which is 13 characters long.
These basic techniques provide a solid foundation for string manipulation in Bash. However, they have their limitations, and it’s important to be aware of potential pitfalls. For example, if a string contains whitespace, it can cause unexpected results. Therefore, always ensure to handle strings carefully, especially when they contain special characters or whitespace.
Bash String Manipulation: Advanced Techniques
Now that we’ve covered the basics, let’s delve into some more complex string manipulation techniques in Bash, including pattern matching and replacement, case conversion, and string splitting. These techniques can provide more control and flexibility when working with strings in Bash.
Pattern Matching and Replacement in Bash
Bash allows you to match a pattern within a string and replace it with another string. This can be useful when you need to modify the content of a string.
string='The quick brown fox jumps over the lazy dog.'
replaced_string=${string//quick/slow}
echo $replaced_string
# Output:
# 'The slow brown fox jumps over the lazy dog.'
In this example, we’ve replaced ‘quick’ with ‘slow’ in the string. The ‘//’ operator is used to replace all occurrences of a pattern within a string.
Case Conversion in Bash
Bash provides features to convert the case of a string. This can be handy when you need to normalize the case of a string.
string='Hello, World!'
lowercase_string=${string,,}
echo $lowercase_string
# Output:
# 'hello, world!'
In this example, we’ve converted ‘Hello, World!’ to lowercase. The ‘,,’ operator is used to convert all characters in a string to lowercase.
String Splitting in Bash
Bash allows you to split a string into an array based on a delimiter. This can be useful when you need to parse a string into multiple parts.
string='apple,banana,carrot'
IFS=',' read -ra array <<< "$string"
echo ${array[1]}
# Output:
# 'banana'
In this example, we’ve split ‘apple,banana,carrot’ into an array using ‘,’ as the delimiter, and then printed the second element of the array.
These advanced techniques offer more capabilities for string manipulation in Bash, but they also come with potential pitfalls. For example, pattern matching and replacement can cause unexpected results if the pattern contains special characters. Therefore, always ensure to handle patterns and strings carefully.
Alternative Methods for Bash String Manipulation
While Bash provides a variety of built-in features for string manipulation, there are also alternative methods that can be used. Tools like awk, sed, and Perl one-liners offer different approaches to string manipulation. Let’s explore these alternatives and how they can be used in Bash.
String Manipulation with Awk
Awk is a powerful text processing tool that can be used to manipulate strings in Bash.
string='Hello, World!'
echo $string | awk '{gsub(/World/, "Universe"); print}'
# Output:
# 'Hello, Universe!'
In this example, we’ve used awk’s ‘gsub’ function to replace ‘World’ with ‘Universe’ in the string.
String Manipulation with Sed
Sed, short for stream editor, is another tool that can be used for string manipulation in Bash.
string='Hello, World!'
echo $string | sed 's/World/Universe/g'
# Output:
# 'Hello, Universe!'
In this example, we’ve used sed’s ‘s’ command to replace ‘World’ with ‘Universe’ in the string.
String Manipulation with Perl One-Liners
Perl one-liners can be a powerful tool for string manipulation in Bash.
string='Hello, World!'
echo $string | perl -pe 's/World/Universe/g'
# Output:
# 'Hello, Universe!'
In this example, we’ve used a Perl one-liner to replace ‘World’ with ‘Universe’ in the string.
These alternative methods offer additional flexibility and capabilities for string manipulation in Bash. However, they also come with their own set of considerations. For example, using these tools requires additional knowledge of their syntax and features. Therefore, the decision to use these alternatives should be made based on the specific requirements of your string manipulation tasks.
Troubleshooting Bash String Manipulation
While Bash provides powerful features for string manipulation, there can be situations where you encounter unexpected results. These can be due to factors such as whitespace, special characters, or variable scoping. Let’s discuss these common issues and provide solutions and workarounds for each.
Dealing with Whitespace in Bash Strings
Whitespace can cause unexpected results when manipulating strings in Bash. For example, a string with leading or trailing spaces can lead to inaccurate string length calculations.
string=' Hello, World! '
length=${#string}
echo $length
# Output:
# 18
In this example, the length of the string is 18, which includes the leading and trailing spaces. To avoid this, you can trim the whitespace before calculating the length.
Handling Special Characters in Bash Strings
Special characters can also cause unexpected results in Bash string manipulation. For example, the ‘&’ character has a special meaning in Bash and can lead to unexpected results when used in pattern replacement.
string='Hello, & World!'
replaced_string=${string//&/and}
echo $replaced_string
# Output:
# 'Hello, and World!'
In this example, the ‘&’ character is replaced with ‘and’ in the string. To avoid issues with special characters, always ensure to escape them or handle them carefully.
Understanding Variable Scoping in Bash
Variable scoping can also affect string manipulation in Bash. For example, a variable defined inside a function is not accessible outside that function.
function example_function {
local string='Hello, World!'
}
example_function
echo $string
# Output:
# ''
In this example, the ‘string’ variable is defined inside the ‘example_function’ function and is not accessible outside that function, leading to an empty output. To avoid this, always ensure to understand and handle variable scoping correctly in Bash.
Bash: A Powerful Tool for String Manipulation
To fully grasp the power of Bash string manipulation, it’s important to understand the fundamental concepts that it’s built upon. Let’s discuss these underlying concepts, such as character encoding, pattern matching, and regular expressions.
Understanding Character Encoding in Bash
Character encoding is a key concept in string manipulation. It’s the system that computers use to represent and manipulate text.
string='Hello, World!'
byte=${string:7:1}
echo $byte
# Output:
# 'W'
In this example, we’ve extracted the byte at index 7 from the string, which is ‘W’. This is based on the ASCII character encoding, where each character is represented by a byte.
Pattern Matching in Bash
Pattern matching is another fundamental concept in Bash string manipulation. It allows you to find and manipulate parts of a string based on a pattern.
string='The quick brown fox jumps over the lazy dog.'
if [[ $string =~ quick ]]; then
echo 'Match found!'
else
echo 'Match not found!'
fi
# Output:
# 'Match found!'
In this example, we’ve used the ‘=~’ operator to check if the string contains the pattern ‘quick’.
Regular Expressions in Bash
Regular expressions, or regex, are a powerful tool for pattern matching in Bash. They allow you to define complex patterns for string manipulation.
string='The quick brown fox jumps over the lazy dog.'
if [[ $string =~ ^The quick ]]; then
echo 'Match found!'
else
echo 'Match not found!'
fi
# Output:
# 'Match found!'
In this example, we’ve used a regular expression ‘^The\ quick’ to check if the string starts with ‘The quick’. The ‘^’ character is a regex metacharacter that matches the start of a line.
Understanding these fundamental concepts can greatly enhance your ability to manipulate strings in Bash. They form the foundation upon which all Bash string manipulation features are built.
Exploring the Relevance of Bash String Manipulation
Bash string manipulation is not just a standalone skill but a key component in various tasks related to Bash scripting, text processing, and system administration. Understanding how to effectively manipulate strings in Bash can significantly enhance your ability to handle these tasks.
Bash String Manipulation in Scripting
In Bash scripting, string manipulation is often used to process input, parse output, and control the flow of the script.
# Bash script to greet user
username=$(whoami)
greeting="Hello, $username!"
echo $greeting
# Output:
# 'Hello, username!'
In this script, we’ve used string manipulation to create a personalized greeting for the user.
Bash String Manipulation in Text Processing
In text processing, Bash string manipulation can be used to parse and transform text data.
# Bash command to count words in a text file
text=$(cat file.txt)
words=($text)
word_count=${#words[@]}
echo $word_count
# Output:
# 'Number of words in file.txt'
In this command, we’ve used string manipulation to count the number of words in a text file.
Bash String Manipulation in System Administration
In system administration, Bash string manipulation can be used to parse and analyze system logs, configuration files, and command output.
# Bash command to get system uptime
uptime=$(uptime -p)
uptime=${uptime//up /}
echo $uptime
# Output:
# 'System uptime'
In this command, we’ve used string manipulation to get the system uptime in a clean format.
Further Resources for Bash String Manipulation Mastery
Here are some resources that can help you further explore and master Bash string manipulation:
- GNU Bash Manual: The official manual for Bash, covering all its features in detail.
- Advanced Bash-Scripting Guide: A comprehensive guide to scripting in Bash, including string manipulation.
- Bash Academy: An interactive platform to learn Bash scripting, with exercises and quizzes.
Wrapping Up: Mastering Bash String Manipulation
In this comprehensive guide, we’ve journeyed through the vast landscape of string manipulation in Bash. We’ve explored the basic techniques, delved into advanced features, and even ventured into alternative methods, equipping you with a robust set of tools for any string manipulation task you might encounter.
We began with the basics, learning how to concatenate strings, extract substrings, and calculate string lengths in Bash. We then ventured into advanced territory, mastering pattern matching and replacement, case conversion, and string splitting. Along this journey, we also encountered common issues such as dealing with whitespace and special characters, and learned how to troubleshoot these challenges.
We didn’t stop there. We also explored alternative methods for string manipulation, using tools like awk, sed, and Perl one-liners. These tools provide additional capabilities and flexibility, but also require a deeper understanding of their syntax and features.
Here’s a quick comparison of the methods we’ve discussed:
Method | Flexibility | Complexity |
---|---|---|
Bash Built-in | Moderate | Low |
awk | High | Moderate |
sed | High | High |
Perl One-Liners | Very High | High |
Whether you’re just starting out with Bash or you’re looking to level up your string manipulation skills, we hope this guide has given you a deeper understanding of Bash string manipulation and its capabilities.
With its balance of flexibility, power, and ease of use, Bash string manipulation is a vital skill for any developer or system administrator. Happy scripting!