Python Infinity: Syntax, Uses, and Examples
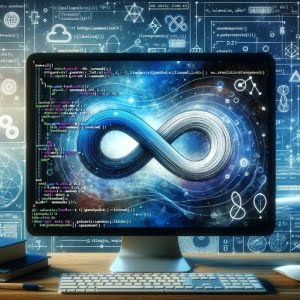
If you’ve found yourself asking, ‘How do I represent infinity in Python?’, you’re in the right place. Much like the boundless cosmos, Python houses a mechanism to depict the notion of infinity.
This article is your comprehensive guide to unravel and employ the concept of infinity in Python, spanning from the rudimentary usage to intricate techniques. Let’s dive into the limitless world of Python infinity, shall we?
TL;DR: How Do I Represent Infinity in Python?
Python provides a straightforward way to represent positive and negative infinity. You can express positive infinity with
float('inf')
and negative infinity withfloat('-inf')
.
Here’s a simple example:
positive_infinity = float('inf')
negative_infinity = float('-inf')
print(positive_infinity > 1000000) # True
print(negative_infinity < -1000000) # True
In this example, we assign positive and negative infinity to the variables positive_infinity
and negative_infinity
respectively. Then, we print out the results of comparing these variables with large numbers. As expected, positive_infinity
is greater than any large number, and negative_infinity
is less than any large negative number.
Intriguing, isn’t it? Keep reading for a more detailed exploration and advanced usage scenarios of infinity in Python.
Table of Contents
Python Infinity Basic Usage
Python, like many programming languages, uses the concept of ‘floating point’ to represent real numbers. This includes a special value called ‘infinity’. You can create positive and negative infinity in Python using float('inf')
and float('-inf')
, respectively. These representations of infinity behave as you would expect in mathematical operations and comparisons.
Let’s see this in action with a simple code example:
positive_infinity = float('inf')
negative_infinity = float('-inf')
# Basic mathematical operations
print(positive_infinity + 100) # inf
print(negative_infinity + 100) # -inf
# Comparisons
print(positive_infinity > 1000000) # True
print(negative_infinity < -1000000) # True
In this code, we first assign positive and negative infinity to the variables positive_infinity
and negative_infinity
. We then perform basic mathematical operations and comparisons.
As you can see, adding any number to positive_infinity
still results in positive_infinity
, and similarly for negative_infinity
. When compared with any finite number, positive_infinity
is greater and negative_infinity
is less.
This behavior of infinity in Python can be extremely useful in a variety of scenarios, such as initializing variables in algorithms where you need a value that’s guaranteed to be greater or less than any other number.
Infinity Squared (Advanced Usage)
While the basic usage of infinity in Python can be quite straightforward, the concept becomes more nuanced when applied to more complex scenarios such as algorithms and data structures. Let’s delve into some of these advanced use cases.
Infinity in Algorithms
Infinity can be used as an initial value in certain algorithms, where you need a value that is guaranteed to be larger or smaller than any other number.
For instance, in the Dijkstra’s shortest path algorithm, we initialize the distance to all nodes as infinity until we find a path.
Here’s a simplified example of how this might look in code:
# Initialize distances to all nodes as infinity
distances = {node: float('inf') for node in nodes}
# The distance to the start node is 0
distances[start_node] = 0
print(distances) # {'A': 0, 'B': inf, 'C': inf, 'D': inf}
In this example, we initialize the distances to all nodes as infinity. The distance to the start node is then set to 0. When we print the distances, we see that the start node ‘A’ has a distance of 0, while all other nodes have a distance of infinity.
Infinity in Data Structures
Infinity can also be used in data structures, such as in a priority queue where you want to initialize the priority of certain elements as the lowest possible.
Here’s a simple code snippet demonstrating this:
# Initialize priorities as negative infinity
priorities = {element: float('-inf') for element in elements}
print(priorities) # {'E1': -inf, 'E2': -inf, 'E3': -inf}
In this code, we initialize the priorities of all elements as negative infinity. This ensures that any new element added with a finite priority will have a higher priority.
These are just a few examples of the advanced uses of infinity in Python. As you delve deeper into Python programming, you’ll find more complex scenarios where understanding and using infinity effectively can be crucial.
Alternative Ways to Represent Infinity in Python
While using float('inf')
and float('-inf')
is the standard way to represent infinity in Python, there are alternative approaches that can be used to represent unbounded values. Let’s delve into some of these methods.
Using Large Numbers
One simple approach is to use an arbitrarily large (or small) number to represent infinity. The choice of this number will depend on the context of your program and the range of values you’re working with.
Here’s an example:
# Using a large number to represent infinity
positive_infinity = 1e300
print(positive_infinity > 1000000) # True
In this example, we’re using 1e300
(1 followed by 300 zeros) as a stand-in for positive infinity. This number is so large that for most practical purposes, it behaves like infinity.
However, this approach has its limitations. The chosen ‘large number’ may not be large enough in some contexts, and there’s always a risk of overflow when performing operations with these numbers.
Using None
In some cases, you might find it useful to use None
to represent an undefined or infinite value.
Here’s an example:
# Using None to represent infinity
positive_infinity = None
print(positive_infinity is None) # True
In this code, we’re using None
to represent an undefined or infinite value. This can be useful in certain scenarios, but it requires careful handling. Since None
is not a number, trying to perform mathematical operations with None
will result in a TypeError
.
While
float('inf')
andfloat('-inf')
are the standard ways to represent infinity in Python, alternatives like using large numbers orNone
can also be used depending on the context. However, these alternatives come with their own set of challenges and require careful handling.
Troubleshooting Common Issues and Solutions
While using infinity in Python can be straightforward in many cases, there are some potential pitfalls that you should be aware of. Let’s explore some common issues and their solutions.
Unexpected Results in Mathematical Operations
One common issue arises when performing mathematical operations involving infinity. For instance, the result of adding positive and negative infinity is not a number (nan
), which might not be the expected result.
positive_infinity = float('inf')
negative_infinity = float('-inf')
print(positive_infinity + negative_infinity) # nan
In this example, adding positive_infinity
and negative_infinity
results in nan
(Not a Number). This is because the sum of positive and negative infinity is an undefined operation in mathematics.
Comparisons Involving Infinity
Another potential issue arises when comparing infinity with other values. For instance, comparing positive infinity with None
does not result in an error, but always returns False
.
positive_infinity = float('inf')
print(positive_infinity > None) # False
In this example, comparing positive_infinity
with None
returns False
. This might not be the expected result, as one might expect an error or positive_infinity
to be greater than None
.
Tips for Handling Infinity
To avoid these issues, it’s important to handle infinity carefully in your code. Here are a few tips:
- Be aware of the potential issues when performing mathematical operations involving infinity, and check for
nan
values in your results. Avoid comparing infinity with
None
or other non-numeric values. Instead, check forNone
or other special values explicitly in your code.Use
math.isinf()
to check if a value is infinity. This function returnsTrue
if the value is positive or negative infinity, andFalse
otherwise.
import math
positive_infinity = float('inf')
print(math.isinf(positive_infinity)) # True
In this code, we use the math.isinf()
function to check if positive_infinity
is indeed infinity, and it returns True
.
By understanding these common issues and their solutions, you can use infinity in Python more effectively and avoid potential pitfalls in your code.
Related Concepts
Understanding infinity in Python paves the way to explore related concepts. The representation of infinity is closely tied to the concept of floating-point representation in computer systems.
It’s worthwhile to delve into how Python handles floating-point numbers, the precision limitations, and the concept of ‘NaN’ (Not a Number).
Another linked concept is that of ‘limits’. In calculus, limits involving infinity are common, and understanding these can provide a deeper insight into why certain operations with infinity yield the results they do.
Further Resources
For those keen on diving deeper into these topics, we have provided some online resources:
- IOFlood’s Python Math Guide ecplores Python’s math module and its wide range of mathematical functions.
Python Exponent: Raising Numbers to Powers – Dive into Python’s exponentiation operator for raising numbers to a power.
Equals Plus or Minus Symbol: Quick Guide on the usage of the equals plus or minus symbol in mathematical expressions.
Achieving Limitless Math in Python – Learn strategies for performing expansive mathematical operations in Python.
Official Python Math Library Documentation – Access information about Python’s math module.
Discussion on Endless Range Functionality in Python – Conversations about implementing endless ranges in Python.
By extending your understanding of infinity in Python and related math topics, you can leverage this powerful concept to solve complex problems and write more efficient, robust code.
An Infinite Recap
In this article, we’ve journeyed through the limitless world of Python infinity. We started with the basics, understanding how to represent positive and negative infinity in Python using float('inf')
and float('-inf')
respectively.
positive_infinity = float('inf')
negative_infinity = float('-inf')
print(positive_infinity > 1000000) # True
print(negative_infinity < -1000000) # True
We then delved into advanced usage scenarios, exploring how infinity finds its place in complex algorithms and data structures. We also discussed alternative approaches to represent unbounded values, such as using large numbers or None
.
We addressed common issues and their solutions, providing tips for handling infinity effectively in your code. We also touched upon the fundamentals of infinity in mathematics and computer science, and how these concepts translate into Python.
Finally, we discussed the practical applications of infinity in real-world Python programs and suggested further resources for deepening your understanding.
By understanding and leveraging Python’s representation of infinity, you can solve complex problems and write more efficient, robust code. Remember, the possibilities with Python infinity are indeed infinite!