Java List vs ArrayList: Detailed Differences and Uses
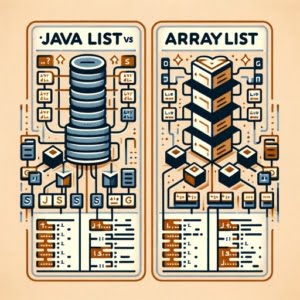
Are you finding it challenging to understand the difference between List and ArrayList in Java? You’re not alone. Many developers find themselves puzzled when it comes to these two data structures, but we’re here to help.
Think of Java’s List and ArrayList as two different tools in your programming toolbox – each with their own strengths and weaknesses, and each suited to different tasks.
In this guide, we’ll walk you through the differences between List and ArrayList in Java, from their basic usage to more advanced techniques. We’ll cover everything from how to declare and use these data structures, to more complex uses and even alternative approaches.
Let’s dive in and start mastering the use of List and ArrayList in Java!
TL;DR: What’s the Difference Between List and ArrayList in Java?
The primary difference between
List
andArrayList
in Java is that List is an interface, while ArrayList is a class that implements this interface. ArrayList offers more functionality and flexibility, but using List can help you create a more generalized program.
Here’s a simple example of using both:
List<String> list = new ArrayList<>();
list.add('Hello');
System.out.println(list.get(0));
// Output:
// 'Hello'
In this example, we’ve created a List object using the ArrayList class. We then added the string ‘Hello’ to the list and printed the first item in the list, which outputs ‘Hello’.
This is just a basic introduction to the differences between List and ArrayList in Java. There’s much more to learn about these two data structures, their uses, and how to choose between them. Continue reading for a more detailed comparison and advanced usage scenarios.
Table of Contents
- Basic Usage of List and ArrayList in Java
- Advanced Usage: List vs ArrayList in Java
- Exploring Alternatives: LinkedList and Vector in Java
- Troubleshooting List and ArrayList in Java
- Understanding Interfaces and Classes in Java
- Utilizing List and ArrayList in Larger Java Projects
- Wrapping Up: Deciphering Java List vs ArrayList
Basic Usage of List and ArrayList in Java
When starting with Java, two of the most common data structures you’ll encounter are List and ArrayList. Let’s break down how to declare and use both of them.
Declaring a List and an ArrayList
A List in Java is declared as follows:
List<String> myList = new ArrayList<>();
In this example, we’ve declared a List named ‘myList’. The “ indicates that this List will hold elements of type String. We’ve used new ArrayList()
to instantiate the List using the ArrayList class.
An ArrayList, on the other hand, is declared slightly differently:
ArrayList<String> myArrayList = new ArrayList<>();
Here, we’ve declared an ArrayList named ‘myArrayList’. Similar to the List, this ArrayList will hold elements of type String. However, notice that we’ve used ArrayList
on the left side of the declaration. This allows us to directly access the additional methods provided by the ArrayList class.
Using a List and an ArrayList
Once declared, using a List or an ArrayList is quite straightforward. For instance, to add elements to the List or ArrayList, you can use the add
method:
myList.add('Hello');
myArrayList.add('Hello');
Both of these lines will add the string ‘Hello’ to the respective data structure. To retrieve an element, you can use the get
method:
String myListElement = myList.get(0);
String myArrayListElement = myArrayList.get(0);
// Output:
// myListElement: 'Hello'
// myArrayListElement: 'Hello'
Both of these lines will retrieve the first element (at index 0) from the respective data structure and store it in a variable.
Differences in Functionality and Flexibility
While List and ArrayList can be used in similar ways, they offer different levels of functionality and flexibility.
A List in Java is an interface, meaning it’s a blueprint for how a data structure should behave. It provides a set of methods that any class implementing the List interface must have. However, it doesn’t provide any implementation for these methods.
On the other hand, ArrayList is a class that implements the List interface. It provides an implementation for all the methods required by the List interface, plus some additional methods for added functionality. This makes ArrayList more flexible than List, as it offers more ways to manipulate data.
In the next section, we’ll delve deeper into these differences and explore more advanced uses of List and ArrayList in Java.
Advanced Usage: List vs ArrayList in Java
As you become more comfortable with Java, you’ll start to discover the more advanced features of List and ArrayList. Let’s explore some of these complex uses and discuss the different methods provided by the List interface and the ArrayList class.
Advanced List Methods
The List interface provides a set of methods for manipulating elements in the list. For instance, the set
method allows you to replace an element at a specific index:
List<String> myList = new ArrayList<>();
myList.add('Hello');
myList.set(0, 'Goodbye');
System.out.println(myList.get(0));
// Output:
// 'Goodbye'
In this example, we’ve replaced the first element (at index 0) of the list with the string ‘Goodbye’. The set
method is part of the List interface, so it can be used with any class that implements this interface, including ArrayList.
Advanced ArrayList Methods
The ArrayList class provides additional methods not found in the List interface. For instance, the trimToSize
method allows you to minimize the storage of an ArrayList by removing any unused capacity:
ArrayList<String> myArrayList = new ArrayList<>(10);
myArrayList.add('Hello');
myArrayList.trimToSize();
In this example, we’ve created an ArrayList with an initial capacity of 10. After adding only one element, we’ve used trimToSize
to remove the unused capacity, thereby optimizing memory usage. This method is specific to the ArrayList class and cannot be used with a List.
Analyzing the Differences
The List interface and the ArrayList class each have their own set of methods, providing different levels of functionality. While the methods provided by the List interface are more basic and generalized, the methods provided by the ArrayList class offer more advanced and specialized functionality.
Choosing between List and ArrayList depends on your specific needs. If you only need basic functionality and want to keep your options open for using different types of lists, you might prefer to use List. However, if you need more advanced functionality or want to optimize memory usage, you might find ArrayList more suitable.
In the next section, we’ll explore alternative data structures in Java and how they compare to List and ArrayList.
Exploring Alternatives: LinkedList and Vector in Java
While List and ArrayList are widely used data structures in Java, they are not the only ones. Two other notable data structures are LinkedList and Vector. Let’s dive into these alternatives and compare them to List and ArrayList.
LinkedList: A List Alternative
A LinkedList in Java is similar to an ArrayList, but with a different underlying data structure. While ArrayList uses a dynamic array, LinkedList uses a doubly-linked list.
Here’s an example of declaring and using a LinkedList:
LinkedList<String> myLinkedList = new LinkedList<>();
myLinkedList.add('Hello');
System.out.println(myLinkedList.get(0));
// Output:
// 'Hello'
In this example, we’ve created a LinkedList, added the string ‘Hello’, and printed the first item, which outputs ‘Hello’.
LinkedList provides similar functionality to ArrayList, but with some differences. For instance, adding or removing elements in the middle of a LinkedList is generally faster than in an ArrayList. However, accessing elements by index is slower in a LinkedList.
Vector: An ArrayList Alternative
A Vector in Java is similar to an ArrayList, but with synchronization. This means that Vector is thread-safe, making it a good choice for multi-threaded environments.
Here’s an example of declaring and using a Vector:
Vector<String> myVector = new Vector<>();
myVector.add('Hello');
System.out.println(myVector.get(0));
// Output:
// 'Hello'
In this example, we’ve created a Vector, added the string ‘Hello’, and printed the first item, which outputs ‘Hello’.
While Vector provides similar functionality to ArrayList, its synchronization feature makes it slower. However, if thread safety is a concern, Vector can be a suitable choice.
Comparing LinkedList and Vector to List and ArrayList
Each of these data structures has its own advantages and disadvantages. LinkedList provides faster insertions and deletions in the middle of the list, but slower access by index. Vector provides thread safety, but at the cost of slower performance. On the other hand, List and ArrayList provide a balance of functionality and performance, making them suitable for a wide range of tasks.
In the end, the choice between these data structures depends on your specific needs and the characteristics of your Java application.
Troubleshooting List and ArrayList in Java
As with any tool, using List and ArrayList in Java can sometimes lead to unexpected issues. Let’s discuss some common problems you might encounter, such as type safety issues and performance considerations, and provide solutions for each.
Type Safety Issues
One common issue in Java is type safety. When declaring a List or an ArrayList, you specify the type of elements it will hold. If you try to add an element of a different type, you’ll encounter a compile-time error:
List<String> myList = new ArrayList<>();
myList.add(123);
// Compile-time error: incompatible types
In this example, we’ve declared a List of Strings and tried to add an integer. This results in a compile-time error because the types are incompatible.
To avoid this issue, always ensure that the elements you’re adding to the List or ArrayList are of the correct type.
Performance Considerations
Another issue to consider is performance. While ArrayList provides a lot of flexibility, it can be slower than other data structures for certain operations. For example, adding or removing elements in the middle of an ArrayList is slower than at the end because all subsequent elements need to be shifted.
If performance is a concern, consider using a LinkedList for operations that involve adding or removing elements in the middle of the list. As we discussed earlier, LinkedList provides faster insertions and deletions in the middle of the list compared to ArrayList.
In conclusion, while List and ArrayList are powerful tools in Java, they come with their own set of issues. By understanding these issues and knowing how to troubleshoot them, you can use List and ArrayList more effectively in your Java applications.
Understanding Interfaces and Classes in Java
Before we delve into the specifics of List and ArrayList, it’s crucial to grasp the concepts of interfaces and classes in Java, as they form the backbone of these two data structures.
What are Interfaces in Java?
In Java, an interface is a reference type, similar to a class, that can contain only constants, method signatures, default methods, static methods, and nested types. It provides a way to ensure that a class adheres to a certain contract, without concerning itself with how the class achieves this.
For instance, the List interface in Java specifies a set of methods that a class must have to be considered a List. However, it doesn’t provide any implementation for these methods.
What are Classes in Java?
A class in Java is a blueprint for creating objects. It includes fields for storing state and methods for performing actions. Classes can implement one or more interfaces, providing an implementation for all the methods required by the interface.
For example, ArrayList is a class in Java that implements the List interface. This means that ArrayList provides an implementation for all the methods specified by the List interface, and may include additional methods for added functionality.
List<String> myList = new ArrayList<>();
myList.add('Hello');
System.out.println(myList.get(0));
// Output:
// 'Hello'
In this code snippet, we create an object of the ArrayList class and use it as a List. The ArrayList class provides implementations for the add
and get
methods, among others.
The Role of Data Structures in Java
In Java, data structures like List and ArrayList play a crucial role in organizing and storing data. They provide a way to manage and access data efficiently, making it easier to build complex applications.
Understanding the differences between List and ArrayList, and the role of interfaces and classes in Java, can help you choose the right data structure for your needs and use it effectively.
Utilizing List and ArrayList in Larger Java Projects
As you dive deeper into Java development, you’ll find that understanding and effectively using data structures like List and ArrayList becomes increasingly important. The choice between these two can significantly impact the design, performance, and scalability of your project.
Impact on Design
The choice between List and ArrayList can influence the design of your Java project. For example, using List in your code promotes the use of interfaces, which makes your code more flexible and adaptable to changes. You can easily switch between different List implementations (like LinkedList or Vector) without changing the rest of your code.
On the other hand, using ArrayList directly in your code can make it more specific and less flexible, but it allows you to leverage the additional methods provided by the ArrayList class.
Impact on Performance
The performance of your Java project can also be affected by your choice between List and ArrayList. For operations like adding or removing elements, ArrayList is faster when these operations are performed at the end of the list, while LinkedList is faster when these operations are performed in the middle of the list. Therefore, choosing the right data structure based on your specific needs can optimize the performance of your project.
Further Resources for Mastering Java Data Structures
To further deepen your understanding of List, ArrayList, and other data structures in Java, here are some useful resources:
- Getting Started with Java List Tutorial – Understand the differences between List and other collection types in Java.
Java List Add Operation – Explore appending elements to Lists dynamically to accommodate changing data requirements.
Overview of List Methods in Java – Master using List methods to perform operations on lists of elements in Java.
Oracle Java Documentation provides in-depth explanations of Java data structures, including List and ArrayList.
GeeksforGeeks’ Java Data Structures offers detailed articles and code examples on data structures in Java.
Java Programming Masterclass – This online course covers all aspects of Java data structures like List and ArrayList.
Remember, mastering data structures in Java is a journey. With practice and continuous learning, you’ll become more proficient and confident in using List, ArrayList, and other data structures in your Java projects.
Wrapping Up: Deciphering Java List vs ArrayList
In this comprehensive guide, we’ve delved into the intricacies of List and ArrayList in Java, two vital data structures that every Java developer should be familiar with.
We kicked off with the basics, explaining how to declare and use both List and ArrayList while highlighting their differences in functionality and flexibility. As we advanced, we explored more complex uses of these data structures, discussing the additional methods provided by the List interface and the ArrayList class.
We didn’t stop there; we examined alternative data structures like LinkedList and Vector, comparing their strengths and weaknesses with List and ArrayList. We also addressed common issues you might encounter when using these data structures, such as type safety and performance considerations, equipping you with practical solutions.
Here’s a quick comparison of the data structures we’ve discussed:
Data Structure | Flexibility | Performance | Use Case |
---|---|---|---|
List | Moderate | High for general uses | When you need a generalized program |
ArrayList | High | High for adding/removing at the end | When you need more functionality |
LinkedList | High | High for adding/removing in the middle | When frequent insertions/deletions are required |
Vector | Moderate | Lower due to synchronization | In multi-threaded environments |
Whether you’re a Java beginner or an experienced developer looking to refresh your knowledge, we hope this guide has deepened your understanding of List and ArrayList, their differences, and how to effectively use them in your Java projects.
With this knowledge, you are better equipped to handle different programming scenarios and make optimal decisions based on your application’s specific needs. Happy coding!