Java List Methods Explained: From Basics to Advanced
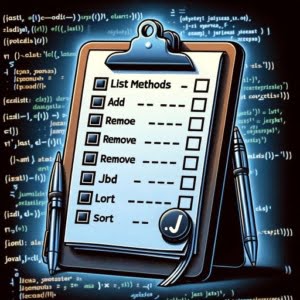
Are you finding it challenging to work with Java List methods? You’re not alone. Many developers, especially those new to Java, often find themselves grappling with these methods.
Think of Java List methods as a Swiss Army knife – they offer a variety of functions to manipulate lists, making them an extremely versatile tool in a developer’s toolkit.
This guide will walk you through the key methods available in Java for handling lists, from basic use to advanced techniques. We’ll cover everything from adding and retrieving elements from a list to more complex operations like sorting and replacing elements.
So, let’s dive in and start mastering Java List methods!
TL;DR: What Are the Main Methods for Java Lists?
Java Lists offer a variety of methods such as
add()
,get()
,set()
,remove()
,size()
, and more. These methods allow you to manipulate and interact with lists in Java in a variety of ways.
Here’s a basic example of using some of these methods:
List<String> list = new ArrayList<>();
list.add('Hello');
String item = list.get(0);
// Output:
// 'Hello'
In this example, we create a new ArrayList and add the string ‘Hello’ to it using the add()
method. We then retrieve the first item in the list using the get()
method, which returns ‘Hello’.
This is just a basic introduction to Java List methods, but there’s much more to learn about these versatile tools. Continue reading for a more detailed understanding and advanced usage scenarios.
Table of Contents
Getting Started with Java List Methods
When you’re just starting out with Java, there are a few basic List methods you’ll use quite often. Let’s go through these methods one by one, with examples to illustrate their use.
Adding Elements: The add()
Method
The add()
method allows you to add elements to your list. Here’s a simple example:
List<String> list = new ArrayList<>();
list.add('Java');
list.add('Python');
list.add('JavaScript');
// Output:
// [Java, Python, JavaScript]
In this example, we create a new list and add three elements to it using the add()
method. The elements are added in the order they were inserted.
Accessing Elements: The get()
Method
The get()
method allows you to access elements in your list based on their index. Let’s use the list we created above:
String item = list.get(1);
// Output:
// Python
In this example, we use the get()
method to access the second element in the list (remember, list indices start at 0 in Java).
Modifying Elements: The set()
Method
The set()
method allows you to replace an element at a specific position in your list. Here’s how you can use it:
list.set(0, 'C++');
// Output:
// [C++, Python, JavaScript]
In this example, we replace the first element in the list (‘Java’) with ‘C++’ using the set()
method.
Removing Elements: The remove()
Method
The remove()
method allows you to remove elements from your list based on their index. Let’s remove the second element from our list:
list.remove(1);
// Output:
// [C++, JavaScript]
In this example, we remove the second element in the list (‘Python’) using the remove()
method.
Determining List Size: The size()
Method
The size()
method allows you to determine the number of elements in your list. Let’s find out how many elements are in our list now:
int size = list.size();
// Output:
// 2
In this example, we use the size()
method to determine that there are two elements in our list.
These are the basic Java List methods that you’ll use quite often. They’re simple to use, but very powerful. In the next section, we’ll explore some more advanced List methods.
Delving Deeper: Advanced Java List Methods
Once you’ve mastered the basic Java List methods, it’s time to explore some more advanced methods. These methods allow you to perform more complex operations on your lists. Let’s dive in.
Iterating Over Lists: The iterator()
Method
The iterator()
method returns an iterator over the elements in the list. Here’s how you can use it:
Iterator<String> iterator = list.iterator();
while (iterator.hasNext()) {
System.out.println(iterator.next());
}
// Output:
// C++
// JavaScript
In this example, we create an Iterator object and use it to iterate over the elements in the list. The hasNext()
method returns true if there are more elements to iterate over, and the next()
method returns the next element.
List Iteration with More Control: The listIterator()
Method
The listIterator()
method returns a list iterator over the elements in the list. Unlike the iterator()
method, the list iterator allows you to iterate in either direction, modify the list during iteration, and obtain the iterator’s current position. Here’s an example:
ListIterator<String> listIterator = list.listIterator();
while (listIterator.hasNext()) {
System.out.println(listIterator.nextIndex() + ": " + listIterator.next());
}
// Output:
// 0: C++
// 1: JavaScript
In this example, we create a ListIterator object and use it to iterate over the elements in the list. The nextIndex()
method returns the index of the element that would be returned by a subsequent call to next()
.
Sorting Lists: The sort()
Method
The sort()
method sorts the elements of the list according to their natural ordering, or by a Comparator provided at the time of invocation. Here’s how to use it:
list.add('Ruby');
list.sort(null);
// Output:
// [C++, JavaScript, Ruby]
In this example, we add a new element to the list and then sort the list. The list is sorted in ascending order according to the natural ordering of its elements.
Replacing All Elements: The replaceAll()
Method
The replaceAll()
method replaces each element of the list with the result of applying the operator to that element. Here’s an example:
list.replaceAll(s -> s.toUpperCase());
// Output:
// [C++, JAVASCRIPT, RUBY]
In this example, we replace each element in the list with its uppercase equivalent using the replaceAll()
method.
These advanced Java List methods provide you with more power and flexibility when working with lists. They may be a bit more complex than the basic methods, but they’re well worth learning.
Exploring Alternatives: LinkedList, Vector, and Stack
Java offers a variety of classes and interfaces that can be used instead of the standard ArrayList for manipulating lists. In this section, we’ll explore three such alternatives: LinkedList
, Vector
, and Stack
.
LinkedList: A List with More Flexibility
The LinkedList
class is an implementation of the List and Deque interfaces. It provides a linked-list data structure. Let’s see an example:
List<String> linkedList = new LinkedList<>();
linkedList.add('Java');
linkedList.add('Python');
linkedList.add('JavaScript');
// Output:
// [Java, Python, JavaScript]
In this example, we create a LinkedList and add elements to it. LinkedList offers methods like addFirst()
, addLast()
, removeFirst()
, and removeLast()
, providing more flexibility in adding or removing elements.
Vector: A Thread-Safe Alternative
The Vector
class is similar to ArrayList, but it’s thread-safe. Every method in Vector is synchronized, making it a good choice for multi-threaded programs. Here’s how you can use it:
List<String> vector = new Vector<>();
vector.add('Java');
vector.add('Python');
vector.add('JavaScript');
// Output:
// [Java, Python, JavaScript]
In this example, we create a Vector and add elements to it. Vector’s methods work the same way as ArrayList’s, but with the added benefit of thread safety.
Stack: A List with LIFO Access
The Stack
class is a subclass of Vector that implements a stack data structure. It provides methods like push()
, pop()
, peek()
, and search()
. Here’s an example:
Stack<String> stack = new Stack<>();
stack.push('Java');
stack.push('Python');
stack.push('JavaScript');
// Output:
// [Java, Python, JavaScript]
In this example, we create a Stack and push elements onto it. The pop()
method would remove the last element added (‘JavaScript’), and peek()
would return it without removing it.
These alternative classes and interfaces offer different ways to work with lists in Java. Depending on your specific needs, one of these might be a better fit than the standard ArrayList.
Troubleshooting Java List Methods
While working with Java List methods, you might encounter some common errors or obstacles. Let’s go through some of these issues and discuss how to solve them.
IndexOutOfBoundsException
One of the most common errors you might encounter is the IndexOutOfBoundsException
. This error occurs when you try to access or remove an element at an index that does not exist in the list.
try {
list.get(5);
} catch (IndexOutOfBoundsException e) {
e.printStackTrace();
}
// Output:
// java.lang.IndexOutOfBoundsException: Index 5 out of bounds for length 3
In this example, we try to access the element at index 5, but our list only has three elements. This results in an IndexOutOfBoundsException
. To avoid this error, always ensure that the index you’re trying to access or remove is within the range of the list size.
ConcurrentModificationException
Another common error is the ConcurrentModificationException
. This error occurs when you try to modify the list while iterating over it using an Iterator.
try {
Iterator<String> iterator = list.iterator();
while (iterator.hasNext()) {
String item = iterator.next();
if (item.equals('Python')) {
list.remove(item);
}
}
} catch (ConcurrentModificationException e) {
e.printStackTrace();
}
// Output:
// java.util.ConcurrentModificationException
In this example, we try to remove an element from the list while iterating over it. This results in a ConcurrentModificationException
. To avoid this error, use the remove()
method of the Iterator instead of the List to remove elements during iteration.
Performance Considerations
When working with large lists, performance can become an issue. Some methods, like add()
and get()
, have a constant time complexity for ArrayLists, but methods like add()
and remove()
can have linear time complexity for LinkedLists if the index is near the end of the list. Therefore, choose your List implementation carefully based on the operations you’ll be performing most often.
In summary, while Java List methods are powerful tools, they can sometimes be tricky to use correctly. By understanding the common errors and their solutions, as well as performance considerations, you can use these methods more effectively and efficiently.
Understanding the Java List Interface
Before delving deeper into Java List methods, it’s crucial to understand the List interface’s fundamentals in Java and how it fits into the language’s overall structure.
The Role of the List Interface
In Java, a List is an ordered collection, also known as a sequence. The List interface is part of Java’s Collection Framework and extends the Collection interface. It provides a way to store the ordered collection, meaning it maintains the insertion order, which is the order in which elements were inserted into the List.
List<String> list = new ArrayList<>();
list.add('Java');
list.add('Python');
list.add('JavaScript');
// Output:
// [Java, Python, JavaScript]
In this example, we add three elements to the list. The list maintains the order of these elements as they were inserted.
Polymorphism in Java Lists
One of Java’s core concepts is polymorphism, which allows an object to take on many forms. Most commonly, this occurs when a parent class reference is used to refer to a child class object.
In the context of Java Lists, this means we can use the List reference to create an ArrayList, LinkedList, Vector, or Stack object. This is possible because all these classes implement the List interface.
List<String> arrayList = new ArrayList<>();
List<String> linkedList = new LinkedList<>();
List<String> vector = new Vector<>();
List<String> stack = new Stack<>();
In this example, we create four different types of lists using a List reference. This is polymorphism in action. The type of list we can create is not determined until runtime.
Understanding these fundamental concepts is key to mastering Java List methods and Java as a whole. With this knowledge, you can leverage the power of the List interface and polymorphism to write more flexible and reusable code.
Leveraging Java List Methods in Real-World Applications
Java List methods are not just theoretical concepts, but practical tools that you’ll use in real-world applications. They play a crucial role in larger projects and algorithms, where managing and manipulating data is often required.
Java List Methods in Larger Projects
In larger projects, you might need to manage large amounts of data, which could involve storing, retrieving, and manipulating data. Java List methods can help you handle these tasks efficiently.
For instance, you might need to sort a list of users based on their last login time, remove inactive users, or find users with specific attributes. Java List methods like sort()
, removeIf()
, and stream().filter()
can make these tasks much easier.
Java List Methods in Algorithms
Java List methods are also commonly used in algorithms. For instance, in sorting algorithms like bubble sort or quicksort, you might need to swap elements in a list. The set()
method can be used for this purpose.
In graph algorithms, you might need to maintain a list of nodes to visit. The add()
, get()
, and remove()
methods can help manage this list.
Related Topics to Explore
While Java List methods are a significant aspect of Java, they often accompany other topics in typical use cases. These can include Java Stream API for more advanced data manipulation, Java Collections Framework for a broader range of data structures, and Java Generics for type-safe data structures.
Further Resources for Mastering Java List Methods
To help you dive deeper into Java List methods and related topics, here are some resources that you might find useful:
- Mastering Lists in Java: Essential Techniques – Learn how to use List to manage collections of elements in Java.
Sorting List in Java – Learn how to sort Lists in Java using built-in methods like Collections.sort().
Understanding List vs ArrayList in Java – Learn about the flexibility and the specific features of Lists and ArrayList.
Oracle’s official Java tutorials covers the Java List interface and other parts of the Collections Framework.
Baeldung’s guides on Java offers a variety of in-depth guides on Java List methods and related topics.
Java Code Geeks offers a variety of articles and tutorials on Java.
Remember, mastering Java List methods takes practice. Don’t be afraid to explore, experiment, and learn from your mistakes.
Wrapping Up: Java List Methods
In this comprehensive guide, we’ve delved deep into the world of Java List methods, a powerful toolset for manipulating lists in Java.
We began with the basics, learning how to use fundamental Java List methods like add()
, get()
, set()
, remove()
, and size()
. We then ventured into more advanced territory, exploring complex methods like iterator()
, listIterator()
, sort()
, and replaceAll()
. Along the way, we also tackled common challenges you might face when using Java List methods, providing you with solutions and workarounds for each issue.
We also explored alternative approaches to list manipulation in Java, comparing ArrayList with other List implementations like LinkedList, Vector, and Stack. Here’s a quick comparison of these List types:
List Type | Flexibility | Thread Safety | LIFO Access |
---|---|---|---|
ArrayList | Moderate | No | No |
LinkedList | High | No | No |
Vector | Moderate | Yes | No |
Stack | Low | Yes | Yes |
Whether you’re just starting out with Java or you’re looking to level up your list manipulation skills, we hope this guide has given you a deeper understanding of Java List methods and their capabilities.
With their balance of flexibility, thread safety, and ease of use, Java List methods are a powerful tool for data manipulation in Java. Now, you’re well equipped to use these methods in your next Java project. Happy coding!