List in Java: Your Ultimate Usage Guide
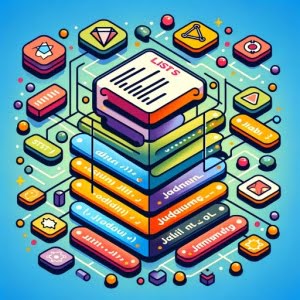
Are you finding it challenging to work with lists in Java? You’re not alone. Many developers find themselves puzzled when it comes to handling lists in Java, but we’re here to help.
Think of Java’s lists as a versatile toolbox – they offer a dynamic way to store and manipulate data, providing a flexible and handy tool for various tasks.
This guide will walk you through the ins and outs of using lists in Java, from their creation, manipulation, and usage. We’ll cover everything from the basics of using lists to more advanced techniques, as well as alternative approaches.
Let’s get started!
TL;DR: How Do I Use a List in Java?
In Java, you can create and use a list using the
ArrayList
class and the syntax,List<String> list = new ArrayList<>();
. This class provides dynamic arrays in Java, which can be handy when working with an unknown number of objects.
Here’s a simple example:
List<String> list = new ArrayList<>();
list.add('Hello');
System.out.println(list.get(0));
// Output:
// 'Hello'
In this example, we create a new ArrayList
object, add a string ‘Hello’ to it, and then print the first element of the list. The ArrayList
class in Java is a resizable array, which can be found in the java.util
package. It is like an array, but there is no size limit. We can add or remove elements anytime. So it is more flexible than the traditional array.
But there’s much more to learn about lists in Java. Continue reading for a deeper understanding and more advanced usage scenarios.
Table of Contents
Getting Started with Java Lists
Java lists are a part of the Java Collections Framework, and they are used to store an ordered collection of objects. The most commonly used type of List is the ArrayList
. Here’s how you can create, add elements to, and retrieve elements from a list in Java:
// Import the ArrayList class
import java.util.ArrayList;
// Create an ArrayList object
ArrayList<String> cars = new ArrayList<String>();
// Add items to the ArrayList
cars.add('Volvo');
cars.add('BMW');
cars.add('Ford');
cars.add('Mazda');
// Access an item in the ArrayList
System.out.println(cars.get(0));
// Output:
// 'Volvo'
In the above code, we first import the ArrayList
class. Then, we create an ArrayList
object named cars
that will store strings. We add four car names to cars
using the add()
method, and then we print out the first item.
Advantages of Using Java Lists
Java lists, especially ArrayLists
, are extremely flexible. They can grow and shrink dynamically as needed and allow you to add, remove, or get items by index. Additionally, they can store different types of objects, including user-defined objects.
Potential Pitfalls of Using Java Lists
However, while Java lists are flexible and easy to use, they do have some potential pitfalls. For one, you can’t use primitive types like int
or char
with lists – you have to use their wrapper classes like Integer
or Character
. Also, if you try to access an index that doesn’t exist, you’ll get an IndexOutOfBoundsException
.
Advanced List Operations in Java
As you become more comfortable with Java lists, you’ll find that they offer a range of more complex operations that can be incredibly useful.
Sorting Lists
Java provides built-in methods to sort lists. Let’s take a look at an example:
// Import necessary classes
import java.util.ArrayList;
import java.util.Collections;
// Create an ArrayList object
ArrayList<String> cars = new ArrayList<String>();
// Add items to the ArrayList
cars.add('Volvo');
cars.add('BMW');
cars.add('Ford');
cars.add('Mazda');
// Sort the ArrayList
Collections.sort(cars);
// Print the sorted ArrayList
for (String car : cars) {
System.out.println(car);
}
/* Output:
BMW
Ford
Mazda
Volvo
*/
In this example, we use the Collections.sort()
method to sort our list of cars in alphabetical order. We then print out the sorted list using a for-each loop.
Searching Lists
You can search for an element in a list using the indexOf()
method, which returns the index of the first occurrence of the specified element, or -1 if the list does not contain the element.
int index = cars.indexOf('Ford');
System.out.println(index);
// Output:
// 1
Here, we’re searching for ‘Ford’ in our list and printing its index.
Modifying Elements
You can modify an element of a list using the set()
method. Here’s how:
cars.set(0, 'Opel');
System.out.println(cars.get(0));
// Output:
// 'Opel'
In this code, we’re replacing the first element (‘BMW’) of our list with ‘Opel’.
Exploring Other Types of Lists in Java
While ArrayList
is the most commonly used list in Java, there are other types of lists that you might find useful depending on your specific needs. Two of these are LinkedList
and Vector
.
Understanding LinkedList
A LinkedList
is a linear data structure where each element is a separate object. Each of these elements (nodes) contains data and a link to the next node in the sequence.
// Import the LinkedList class
import java.util.LinkedList;
// Create a LinkedList object
LinkedList<String> cars = new LinkedList<String>();
// Add items to the LinkedList
cars.add('Volvo');
cars.add('BMW');
cars.add('Ford');
cars.add('Mazda');
// Print the LinkedList
System.out.println(cars);
// Output:
// [Volvo, BMW, Ford, Mazda]
Here, we create a LinkedList
, add some elements to it, and print the list. LinkedLists
are best used when you expect to perform more add/remove operations and fewer get/set operations.
Getting to Know Vector
Vector
is a legacy class from the earliest versions of Java. It’s similar to ArrayList
, but it’s synchronized, meaning it’s thread-safe.
// Import the Vector class
import java.util.Vector;
// Create a Vector object
Vector<String> cars = new Vector<String>();
// Add items to the Vector
cars.add('Volvo');
cars.add('BMW');
cars.add('Ford');
cars.add('Mazda');
// Print the Vector
System.out.println(cars);
// Output:
// [Volvo, BMW, Ford, Mazda]
In this example, we create a Vector
, add some elements to it, and print the list. Vectors
are best used in multithreaded environments where you need to ensure data consistency.
Making the Right Choice
The choice between ArrayList
, LinkedList
, and Vector
depends on your specific use-case. If you need to frequently access elements, ArrayList
is a good choice. If you need to frequently add or remove elements, LinkedList
might be more suitable. And if you’re working in a multithreaded environment, Vector
could be the right choice.
Troubleshooting Common Issues with Java Lists
Working with lists in Java is not without its challenges. It’s common to encounter a few issues, especially when you’re just starting out. Let’s discuss some of these problems and how to resolve them.
Handling IndexOutOfBoundsException
An IndexOutOfBoundsException
occurs when you try to access an index that doesn’t exist in the list. For example:
ArrayList<String> list = new ArrayList<String>();
list.add('Hello');
System.out.println(list.get(1));
/* Output:
Exception in thread 'main' java.lang.IndexOutOfBoundsException: Index: 1, Size: 1
*/
In the code above, we’re trying to access the second element (index 1) in a list that only contains one element. This throws an IndexOutOfBoundsException
.
To avoid this, always ensure that the index you’re trying to access exists. You can check the size of the list using the size()
method:
if (list.size() > 1) {
System.out.println(list.get(1));
} else {
System.out.println('Index does not exist');
}
// Output:
// 'Index does not exist'
Dealing with ConcurrentModificationException
A ConcurrentModificationException
occurs when you try to modify a list while iterating over it. For example:
ArrayList<String> list = new ArrayList<String>();
list.add('Hello');
list.add('World');
for (String item : list) {
if (item.equals('Hello')) {
list.remove(item);
}
}
/* Output:
Exception in thread 'main' java.util.ConcurrentModificationException
*/
In this code, we’re trying to remove an item from the list while we’re iterating over it, which throws a ConcurrentModificationException
.
To avoid this, you can use an Iterator
to safely remove items from a list while iterating over it:
import java.util.Iterator;
Iterator<String> iterator = list.iterator();
while (iterator.hasNext()) {
String item = iterator.next();
if (item.equals('Hello')) {
iterator.remove();
}
}
// This code will successfully remove 'Hello' from the list
In this revised code, we’re using an Iterator
to iterate over the list and safely remove the item ‘Hello’.
Understanding the Java List Interface
To fully grasp how lists work in Java, it’s crucial to understand the List
interface and the concept of generics.
The List Interface
The List
interface is a part of the Java Collections Framework and extends the Collection
interface. It represents an ordered collection of objects, meaning you can access elements of a list in a specific order, and they are indexed.
The List
interface provides several methods for manipulating elements in the list. Some of the most commonly used methods include add()
, get()
, set()
, remove()
, and size()
.
// Import the ArrayList class
import java.util.ArrayList;
// Create an ArrayList object
ArrayList<String> list = new ArrayList<String>();
// Use the add() method
list.add('Hello');
// Use the get() method
System.out.println(list.get(0));
// Use the set() method
list.set(0, 'World');
// Use the size() method
System.out.println(list.size());
/* Output:
Hello
1
*/
In this example, we create a list, add an element to it, retrieve the element, change the element, and check the size of the list.
Generics in Java
Generics in Java is a feature that allows type (classes and interfaces) to be a parameter when defining classes, interfaces, and methods. Much like the actual parameters we pass to methods, we can also pass types to constructors and methods using generics.
When it comes to lists, generics let us ensure that our lists are type-safe, meaning we can define what kind of elements our list can contain.
// This list can only contain strings
List<String> list = new ArrayList<String>();
// This list can only contain integers
List<Integer> numbers = new ArrayList<Integer>();
In the code above, we define a list that can only contain strings and a list that can only contain integers. If we try to add an element of the wrong type to either of these lists, we’ll get a compile-time error.
Exploring the Relevance of Lists in Java
Java lists, as part of the broader Java Collections Framework, play an integral role in data structures and algorithms. They allow for dynamic data management, making them a go-to tool for many developers.
Lists in Data Structures and Algorithms
In data structures and algorithms, lists are often used to store and manipulate data. They allow for efficient insertion, deletion, and traversal of elements, making them ideal for various algorithmic solutions.
Lists in Larger Java Projects
In larger Java projects, lists are often used to handle dynamic data sets. They can store user input, database query results, and more. Their flexibility and ease of use make them a staple in many Java applications.
Related Topics to Explore
While lists are a powerful tool, they’re just one part of a larger picture. Other data structures such as arrays, sets, and maps also play a crucial role in Java programming. Understanding these tools and how they interact is key to mastering Java.
Arrays are a simpler form of data storage but lack the dynamic nature of lists. Sets, on the other hand, are used when you want to store a collection of unique elements. Maps, or key-value pairs, are used when you want to associate specific values with unique keys.
Further Resources for Mastering Java Lists
To deepen your understanding of Java lists and related topics, consider exploring these resources:
- Introduction to Java Collections – Master storage, retrieval, and manipulation of data with the usage of Java Collections.
Java List Methods Overview – Explore methods for manipulating Lists in Java, including add(), remove(), and get().
Oracle’s Java Tutorials offers comprehensive tutorials on Java lists and the Collections Framework.
Geeks for Geeks’ Java Library offers a wealth of information on Java’s data structures and lists.
Java Code Geeks offers a wide range of Java tutorials on data structures and algorithms.
Wrapping Up: List in Java
In this comprehensive guide, we’ve delved into the world of lists in Java, a fundamental concept in Java programming that allows for dynamic data storage and manipulation.
We started with the basics, learning how to create, add elements to, and retrieve elements from a list. We then explored more advanced operations such as sorting, searching, and modifying elements in a list. Along the way, we tackled common issues like IndexOutOfBoundsException
and ConcurrentModificationException
, providing solutions to help you overcome these hurdles.
We also introduced alternative types of lists like LinkedList
and Vector
, helping you understand when to use them. Here’s a quick comparison of these types of lists:
List Type | Pros | Cons |
---|---|---|
ArrayList | Dynamic size, easy to use | Not efficient for adding/removing in the middle |
LinkedList | Efficient add/remove at both ends | Not efficient for random access |
Vector | Thread-safe | Less performance-efficient due to synchronization |
Whether you’re a beginner just starting out with lists in Java, or an intermediate developer looking to brush up on your skills, we hope this guide has been a valuable resource. With a firm grasp of lists, you’re now better equipped to handle a wide range of programming tasks in Java.
Remember, practice is key when it comes to programming. So, keep experimenting with different list operations and scenarios. Happy coding!