Is Python Case Sensitive? A Comprehensive Guide
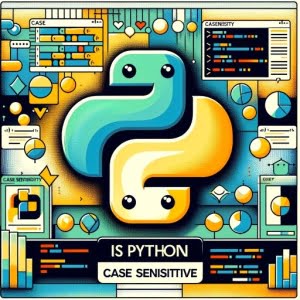
Have you ever been puzzled by Python’s behavior when it comes to the case of your variables, functions, or classes? You’re not alone. Many developers find themselves scratching their heads when they encounter unexpected results due to Python’s case sensitivity.
Think of Python’s case sensitivity as a strict grammar teacher who pays close attention to the case of your letters. This attribute of Python can be a powerful tool when used correctly, but it can also lead to confusing errors if overlooked.
In this guide, we’ll delve into the case sensitivity rules in Python and how they impact your coding. We’ll cover everything from the basics of case sensitivity, to Python’s syntax rules related to case, and even best practices to avoid common pitfalls.
So, let’s dive in and start mastering Python’s case sensitivity!
TL;DR: Is Python Case Sensitive?
Yes, Python is case sensitive. In Python, ‘Variable’ and ‘variable’ are treated as two distinct entities. Here’s a simple illustration:
Variable = 10
variable = 20
print(Variable, variable)
# Output:
# 10 20
In this example, we have two variables: ‘Variable’ and ‘variable’. Despite having similar names, Python treats them as separate variables due to the difference in case. As a result, each variable holds a different value, and the output reflects this.
This is just a basic demonstration of Python’s case sensitivity. To gain a deeper understanding of how case sensitivity affects variables, functions, and classes in Python, keep reading for more detailed explanations and advanced examples.
Table of Contents
- Exploring Case Sensitivity in Python
- Python Syntax and Case Sensitivity
- Best Practices for Case Usage in Python
- Troubleshooting Case Sensitivity in Python
- Python’s Design Philosophy and Case Sensitivity
- Case Sensitivity in Other Languages
- Diving Deeper: Python Naming Conventions and Style Guides
- Wrapping Up: Understanding Case Sensitivity in Python
Exploring Case Sensitivity in Python
Python, like many other programming languages, is case sensitive. This means that it treats the same sequence of characters as different entities if they have different cases. Let’s understand this with some examples.
Variables and Case Sensitivity
In Python, variables with the same name but different cases are treated as different variables. For instance:
myVariable = 'Hello'
myvariable = 'World'
print(myVariable, myvariable)
# Output:
# 'Hello World'
In this example, ‘myVariable’ and ‘myvariable’ are considered as two separate variables. The first one holds the string ‘Hello’, and the second one holds ‘World’. When we print them, we get two different words.
Functions and Case Sensitivity
Just like variables, Python also treats functions with different cases as separate functions. Here’s an example:
def myFunction():
return 'Hello'
def myfunction():
return 'World'
print(myFunction(), myfunction())
# Output:
# 'Hello World'
In this code, we have two functions: ‘myFunction’ and ‘myfunction’. Despite having similar names, Python treats them as separate functions due to the difference in case.
Classes and Case Sensitivity
Python extends its case sensitivity rules to classes as well. Consider the following example:
class MyClass:
def say_hello(self):
return 'Hello'
class Myclass:
def say_world(self):
return 'World'
obj1 = MyClass()
obj2 = Myclass()
print(obj1.say_hello(), obj2.say_world())
# Output:
# 'Hello World'
Here, ‘MyClass’ and ‘MyClass’ are treated as two separate classes. Each class has a different method, and when we create objects of these classes and call their methods, we get different outputs.
As you can see, Python’s case sensitivity plays a crucial role when dealing with variables, functions, and classes. Understanding this concept is essential for writing clear and error-free Python code.
Python Syntax and Case Sensitivity
Understanding Python’s case sensitivity is crucial, especially when it comes to syntax rules. Let’s delve into how Python’s syntax adheres to case sensitivity.
Python Identifiers and Case Sensitivity
Python identifiers, such as variable names, function names, and class names, are case sensitive. This means ‘myVariable’, ‘myvariable’, and ‘MYVARIABLE’ are all different identifiers in Python.
myVariable = 'Hello'
myvariable = 'Hi'
MYVARIABLE = 'Hey'
print(myVariable, myvariable, MYVARIABLE)
# Output:
# 'Hello Hi Hey'
In this example, the three variables hold different string values. Therefore, the output displays three different greetings.
Python Keywords and Case Sensitivity
Python’s keywords are strictly lowercase. Trying to use an uppercase or capitalized version of a Python keyword will result in a syntax error.
For instance, ‘for’, ‘if’, ‘and’, ‘not’, ‘in’ are all Python keywords. Attempting to use ‘For’, ‘IF’, ‘AND’, ‘NOT’, ‘IN’ instead will not work.
For i in range(5): # This will throw a syntax error
print(i)
In this code, ‘For’ is not recognized as a keyword because Python expects ‘for’ in lowercase. Hence, the code will not execute and will throw a syntax error.
Python Built-in Functions and Case Sensitivity
Python’s built-in functions are also case sensitive. For instance, ‘print()’, ‘len()’, ‘type()’, ‘id()’, etc., should be used in lowercase. Using them in any other case will not work as expected.
Print('Hello') # This will throw a NameError
In this example, ‘Print’ is not recognized as a built-in function because Python expects ‘print’ in lowercase. Therefore, the code will throw a NameError.
In conclusion, Python’s syntax rules strictly adhere to case sensitivity, and understanding this is crucial for writing correct and efficient Python code.
Best Practices for Case Usage in Python
Understanding Python’s case sensitivity is only the first step. To write clear, efficient, and error-free Python code, it’s essential to follow some best practices related to case usage.
Consistent Case Usage
In Python, it’s crucial to be consistent with your case usage. This means if you’ve defined a variable, function, or class in a certain case, you should use the same case whenever you refer to it later in your code.
def myFunction():
return 'Hello World'
print(MyFunction()) # This will throw a NameError
In this example, we’ve defined a function ‘myFunction’ but tried to call it as ‘MyFunction’. Python treats them as two different functions due to the difference in case, and since ‘MyFunction’ is not defined, it throws a NameError.
Avoiding Inconsistent Case Usage
Inconsistent case usage can lead to errors and confusion. It’s a common mistake to define a variable in one case and then try to use it in a different case.
myVariable = 'Hello World'
print(myvariable) # This will throw a NameError
In this code, ‘myVariable’ is defined, but when we try to print ‘myvariable’, Python throws a NameError because ‘myvariable’ is not defined.
Following Python Naming Conventions
Python has specific naming conventions for variables, functions, and classes. Variables and functions should be in lowercase with words separated by underscores (snake_case), while class names should be in CamelCase.
# Good practice
my_variable = 'Hello'
def my_function():
return 'World'
class MyClass:
pass
# Bad practice
myVariable = 'Hello'
def myFunction():
return 'World'
class my_class:
pass
Following these best practices for case usage in Python will help you write cleaner, more readable code and avoid common pitfalls related to Python’s case sensitivity.
Troubleshooting Case Sensitivity in Python
Understanding Python’s case sensitivity can help avoid many common errors. Let’s explore some of these errors and how to resolve them.
Unintentional Multiple Variables
One of the common mistakes is unintentionally creating multiple variables due to inconsistent case usage. This can lead to unexpected results.
myVariable = 'Hello'
myvariable = 'World'
print(myVariable, myvariable)
# Output:
# 'Hello World'
In this example, we intended to print ‘Hello Hello’, but due to the difference in case, Python treats ‘myVariable’ and ‘myvariable’ as two separate variables. Hence, the output is ‘Hello World’.
Undefined Name Error
Another common error is the ‘NameError’, which occurs when we try to use a variable or function that has not been defined.
def myFunction():
return 'Hello World'
print(MyFunction()) # This will throw a NameError
In this code, we’ve defined a function ‘myFunction’ but tried to call it as ‘MyFunction’. Python treats them as two different functions due to the difference in case, and since ‘MyFunction’ is not defined, it throws a NameError.
Syntax Error with Keywords
Python’s keywords are strictly lowercase. Trying to use an uppercase or capitalized version of a Python keyword will result in a syntax error.
For i in range(5): # This will throw a syntax error
print(i)
In this example, ‘For’ is not recognized as a keyword because Python expects ‘for’ in lowercase. Hence, the code will not execute and will throw a syntax error.
Remember, being mindful of Python’s case sensitivity and following best practices can help you write error-free code.
Python’s Design Philosophy and Case Sensitivity
Python’s case sensitivity is not a random decision, but a part of its design philosophy. The creators of Python aimed for a language that is easy to read, write, and understand. They believed that making Python case sensitive would help create more readable code.
Consider the following example:
# Two different variables due to case sensitivity
myVariable = 'Hello'
myvariable = 'World'
print(myVariable)
print(myvariable)
# Output:
# 'Hello'
# 'World'
In this example, the difference in case allows us to use what seems like the same name to represent two different things. This is a part of Python’s goal to make code more explicit and readable.
Case Sensitivity in Other Languages
Case sensitivity is not unique to Python. Many other programming languages, including C++, Java, and JavaScript, are also case sensitive. However, not all languages are case sensitive. For instance, SQL and Pascal are case insensitive languages.
The choice of case sensitivity in a language can significantly affect how you write code. In case sensitive languages like Python, ‘myVariable’, ‘myvariable’, and ‘MYVARIABLE’ are treated as different entities. In contrast, in case insensitive languages, these would all be treated as the same entity.
Understanding the case sensitivity of Python and other languages can help you write more accurate and efficient code.
Diving Deeper: Python Naming Conventions and Style Guides
Python’s case sensitivity is closely tied to its naming conventions and style guides. Understanding these conventions can help you write cleaner, more efficient, and more Pythonic code.
Python Naming Conventions
Python has specific naming conventions for variables, functions, and classes. Variables and functions should be in lowercase with words separated by underscores (snake_case), while class names should be in CamelCase.
# Good practice
my_variable = 'Hello'
def my_function():
return 'World'
class MyClass:
pass
# Bad practice
myVariable = 'Hello'
def myFunction():
return 'World'
class my_class:
pass
In the above example, the first set of variable, function, and class names follow Python’s naming conventions, while the second set does not.
Python Style Guides
Python has a style guide known as PEP 8, which provides guidelines for writing readable and consistent Python code. PEP 8 covers various aspects of coding style, including naming conventions, indentation, and spacing.
For instance, PEP 8 recommends using lowercase with underscores for function and variable names, and CamelCase for class names. These recommendations align with Python’s case sensitivity.
Further Resources for Python Case Sensitivity
To delve deeper into Python’s case sensitivity and related topics, here are some resources that can help:
- Python’s official documentation: Python’s official documentation is a comprehensive resource that covers all aspects of the language, including its case sensitivity.
PEP 8 — Style Guide for Python Code: PEP 8 is Python’s official style guide. It provides guidelines for writing readable and consistent Python code, including naming conventions.
Python Tutor: Python Tutor is an interactive tool that allows you to visualize Python code execution, which can be helpful for understanding case sensitivity and other concepts.
Wrapping Up: Understanding Case Sensitivity in Python
In this comprehensive guide, we’ve navigated the world of Python’s case sensitivity, a fundamental concept that plays a crucial role in writing clear and efficient Python code.
We started with the basics, exploring how Python treats variables, functions, and classes with different cases. We then delved into Python’s syntax rules related to case sensitivity, providing practical examples to illustrate these concepts. We also discussed common errors related to Python’s case sensitivity and provided solutions to help you avoid these pitfalls.
We ventured into more advanced territory by discussing best practices for case usage in Python. We emphasized the importance of consistent case usage and following Python’s naming conventions. We also looked at Python’s design philosophy and how case sensitivity fits into it.
Here’s a quick recap of the key points we’ve discussed:
Key Point | Description |
---|---|
Python’s Case Sensitivity | Python treats the same sequence of characters as different entities if they have different cases. |
Syntax Rules | Python’s syntax rules, including identifiers and keywords, strictly adhere to case sensitivity. |
Common Errors | Common errors related to Python’s case sensitivity include unintentional multiple variables, undefined name errors, and syntax errors with keywords. |
Best Practices | Consistent case usage and following Python’s naming conventions are crucial for writing clear and efficient Python code. |
Whether you’re a beginner just starting out with Python or an experienced developer looking to brush up on the fundamentals, we hope this guide has provided you with a deeper understanding of Python’s case sensitivity and its impact on your coding.
With this knowledge, you’re well-equipped to write Python code that is more accurate, efficient, and free of common errors related to case sensitivity. Happy coding!