Java List of Strings: Creation, Manipulation, and More
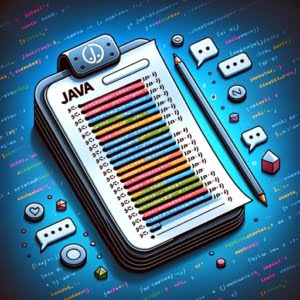
Are you finding it challenging to manage lists of strings in Java? You’re not alone. Many developers find themselves in a maze when it comes to handling lists of strings in Java, but we’re here to help.
Think of Java’s list of strings as a well-organized library – allowing us to store and manipulate data efficiently, providing a versatile and handy tool for various tasks.
This guide will walk you through everything you need to know about creating, manipulating, and using lists of strings in Java. We’ll cover everything from the basics of list creation to more advanced techniques, as well as alternative approaches.
Let’s get started and master lists of strings in Java!
TL;DR: How Do I Create and Use a List of Strings in Java?
In Java, you can create and use a list of strings using the
ArrayList
class. This class is part of the Java Collections Framework and is used for storing dynamic data.
Here’s a simple example:
List<String> list = new ArrayList<String>();
list.add("Hello");
list.add("World");
System.out.println(list);
# Output:
# [Hello, World]
In this example, we’ve created an ArrayList
named list
and added two strings, “Hello” and “World”. The add
method is used to append elements to the list. When we print the list, it outputs the elements enclosed in square brackets, separated by commas.
This is a basic way to create and use a list of strings in Java, but there’s much more to learn about manipulating and managing lists of strings. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
Creating a List of Strings in Java
One of the most basic and commonly used classes for handling lists in Java is the ArrayList
class. It’s part of the Java Collections Framework and is widely used because of its flexibility and functionality.
An ArrayList
is a resizable array that grows automatically when more elements are added to it. It also shrinks when elements are removed.
Here is a simple example of how to create a list of strings using the ArrayList
class:
List<String> fruits = new ArrayList<String>();
fruits.add("Apple");
fruits.add("Banana");
fruits.add("Cherry");
System.out.println(fruits);
# Output:
# [Apple, Banana, Cherry]
In this code block, we’ve created an ArrayList
named fruits
and added three strings to it. By using the add
method, we’re able to append elements to the end of the list. The System.out.println(fruits);
line prints out the entire list.
One of the advantages of using the ArrayList
class is its dynamic nature. It can grow and shrink at runtime, providing flexibility. However, it’s worth noting that ArrayList
is not synchronized, which means it’s not suitable for thread-safe operations without explicit synchronization.
In the next sections, we’ll dive deeper into more complex operations and alternative approaches for managing lists of strings in Java.
Advanced Operations on Lists of Strings
As you become more comfortable with handling lists of strings in Java, you’ll discover that there’s a lot more you can do beyond simply creating a list and adding elements to it. More complex operations such as sorting, filtering, and transforming become important tools in your Java toolkit.
Sorting a List of Strings
Sorting is a common operation performed on lists. In Java, we can use the Collections.sort()
method to sort a list of strings in alphabetical order. Let’s sort our fruits
list:
Collections.sort(fruits);
System.out.println(fruits);
# Output:
# [Apple, Banana, Cherry]
In this example, the Collections.sort()
method sorts the fruits
list in alphabetical order. The resulting output confirms the list’s new order.
Filtering a List of Strings
Filtering is another useful operation. Suppose we want to find all fruits in our list that start with the letter ‘A’. We can use Java 8’s Stream API to achieve this:
List<String> filteredFruits = fruits.stream()
.filter(fruit -> fruit.startsWith("A"))
.collect(Collectors.toList());
System.out.println(filteredFruits);
# Output:
# [Apple]
In this code block, we’re creating a new list filteredFruits
and using the stream().filter().collect()
methods to add only those fruits that start with ‘A’.
Transforming a List of Strings
Transformation is about changing the form of the elements in the list. For instance, we can transform our list of fruits to contain only the first letter of each fruit:
List<String> transformedFruits = fruits.stream()
.map(fruit -> fruit.substring(0, 1))
.collect(Collectors.toList());
System.out.println(transformedFruits);
# Output:
# [A, B, C]
Here, we’re using the stream().map().collect()
methods to create a new list transformedFruits
that contains only the first letter of each fruit in the fruits
list.
These are just a few examples of the more advanced operations you can perform on lists of strings in Java. As you continue to explore, you’ll discover many more possibilities.
Exploring Alternative Approaches
While ArrayList
is a popular choice for managing lists of strings in Java, it’s not the only option. Other classes and techniques offer unique benefits and may be more suitable for certain scenarios. Let’s explore some of these alternatives.
Using LinkedList Class
The LinkedList
class is another implementation of the List
interface that can be used to manage a list of strings. Unlike ArrayList
, LinkedList
is a doubly-linked list, which means it maintains a link to both the next element and the previous one.
Here’s how to create a list of strings using LinkedList
:
List<String> animals = new LinkedList<String>();
animals.add("Dog");
animals.add("Cat");
animals.add("Bird");
System.out.println(animals);
# Output:
# [Dog, Cat, Bird]
The LinkedList
class is particularly efficient when it comes to adding or removing elements from the list’s beginning or end. However, it can be slower than ArrayList
for operations that require accessing elements in the middle of the list.
Leveraging Java 8 Streams
Java 8 introduced the Stream API, which provides a new abstraction for working with sequences of objects in a functional programming style. Streams can be used to perform complex operations on lists of strings in a more readable and concise way.
For example, here’s how to filter and transform a list of strings using streams:
List<String> filteredAndTransformedAnimals = animals.stream()
.filter(animal -> animal.startsWith("C"))
.map(animal -> animal.toUpperCase())
.collect(Collectors.toList());
System.out.println(filteredAndTransformedAnimals);
# Output:
# [CAT]
In this example, we’re creating a new list filteredAndTransformedAnimals
that contains only those animals that start with ‘C’, transformed to uppercase.
Java 8 streams can make your code more readable and easier to understand, especially for complex operations. However, they can also be less efficient than traditional loops for simple operations or very large lists.
Choosing between ArrayList
, LinkedList
, and Java 8 streams depends on your specific use case. Consider the size of your list, the types of operations you need to perform, and the readability and maintainability of your code when making your decision.
Working with lists of strings in Java is not without its challenges. You may encounter some common issues and exceptions. Let’s discuss a few and explore solutions and workarounds.
Dealing with NullPointerException
A NullPointerException
can occur when you try to invoke a method or access a property of an object that is null. Here’s an example:
List<String> list = null;
list.add("Hello");
# Output:
# Exception in thread "main" java.lang.NullPointerException
In this example, we’ve declared a list but haven’t instantiated it. When we try to add an element to the list, Java throws a NullPointerException
.
To avoid this, always ensure your list is properly instantiated before using it:
List<String> list = new ArrayList<String>();
list.add("Hello");
System.out.println(list);
# Output:
# [Hello]
Handling IndexOutOfBoundsException
An IndexOutOfBoundsException
is thrown when you try to access an index that is out of the range of existing indices in the list. Here’s an example:
List<String> list = new ArrayList<String>();
list.add("Hello");
System.out.println(list.get(1));
# Output:
# Exception in thread "main" java.lang.IndexOutOfBoundsException: Index 1 out of bounds for length 1
In this example, we’ve added one element to the list, but we’re trying to access the second element (index 1), which doesn’t exist. To avoid this exception, always check if the index you’re trying to access is within the bounds of the list.
These are just a couple of the common issues you may encounter when working with lists of strings in Java. Being aware of these potential pitfalls can save you a lot of debugging time and help you write more robust code.
Understanding Java’s List Interface and String Class
Before we delve deeper into the manipulation of lists of strings in Java, it’s crucial to understand the basic building blocks: the List
interface, the String
class, and the concept of generics in Java.
The List Interface
In Java, List
is an interface of the Java Collection framework. It provides a way to store the ordered collection. It is an ordered collection of objects in which duplicate values can be stored. Since List
preserves the insertion order, it allows positional access and insertion of elements.
The String Class
The String
class represents character strings. All string literals in Java programs, such as “abc”, are implemented as instances of this class. Strings are constant, their values cannot be changed after they are created.
Generics in Java
Generics are a paradigm that allows types (classes and interfaces) to be parameters when defining classes, interfaces, and methods. In the context of lists of strings, List
is a generic type that represents a List
of String
objects. The “ part is the type parameter, and it tells the Java compiler that this list will only hold String
objects.
Here’s a simple example of a list of strings:
List<String> names = new ArrayList<String>();
names.add("John");
names.add("Jane");
System.out.println(names);
# Output:
# [John, Jane]
In this example, we’ve created a list of strings named names
and added two strings to it. The add
method appends elements to the end of the list. When we print the list, it displays all the elements in the order they were added.
Understanding these fundamental concepts is key to effectively working with lists of strings in Java. With this foundation, you can better understand how to create, manipulate, and manage lists of strings in your Java programs.
The Power of Lists of Strings in Java Applications
Working with lists of strings is a common task in Java programming. It’s a fundamental skill that can be applied in various real-world scenarios, such as data processing, file I/O, and more.
Data Processing
In data processing, you often need to handle and manipulate large sets of text data. Lists of strings are an essential tool for this task. They allow you to store, sort, filter, and transform text data in a structured and efficient way.
File I/O
When reading or writing text files in Java, you often deal with lines of text, which can be naturally represented as lists of strings. You can read a text file line by line into a list of strings, manipulate it as needed, and then write it back to a file.
Exploring Related Concepts
Once you’ve mastered lists of strings, you may want to explore related concepts in the Java Collections Framework, such as sets, maps, and queues. You may also want to delve deeper into Java 8 streams, which provide a powerful and expressive tool for manipulating collections of data.
Further Resources for Mastering Java Lists
To continue your journey in mastering lists in Java, here are some additional resources:
- Java List: A Quick Overview – Understand List’s memory management and performance considerations in Java.
Java List Sorting Guide – Explore sorting techniques for custom object lists and natural ordering of elements.
Adding Elements to List in Java – Learn various ways to add elements to Lists in Java, including add(), addAll(), and insert().
Oracle’s Java Collections Tutorial covers the Java Collections Framework, including lists, sets, maps, and more.
This Java 8 Stream Tutorial by Baeldung provides a comprehensive introduction to Java 8 streams and aggregating data.
Java List Collection Tutorial by CodeJava is complete with practical examples on Java List collection.
Wrapping Up: Lists of Strings in Java
In this comprehensive guide, we’ve explored the ins and outs of creating, manipulating, and using lists of strings in Java. From the basics of ArrayList
to advanced operations and alternative approaches, we’ve covered a wide range of techniques to help you handle lists effectively in your Java programs.
We began with the basics, learning how to create a list of strings using the ArrayList
class. We then delved into more advanced topics, such as sorting, filtering, and transforming lists. Along the way, we tackled common issues like NullPointerException
and IndexOutOfBoundsException
, equipping you with the knowledge to troubleshoot these problems.
We also explored alternative approaches for managing lists of strings, such as using the LinkedList
class and Java 8 streams. These alternatives offer unique benefits and may be more suitable for certain scenarios, depending on the size of your list and the types of operations you need to perform.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
ArrayList | Dynamic, flexible | Not thread-safe without explicit synchronization |
LinkedList | Efficient for adding/removing at start/end | Slower for accessing elements in the middle |
Java 8 Streams | More readable, expressive for complex operations | Less efficient for simple operations or large lists |
Whether you’re a beginner just starting out with Java, or an experienced developer looking to brush up on your skills, we hope this guide has provided you with a deeper understanding of how to work with lists of strings in Java.
Mastering lists of strings is a key skill in Java programming. With this knowledge, you’re well-equipped to handle a wide range of programming tasks. Happy coding!