Python Requests Module Usage Guide (With Examples)
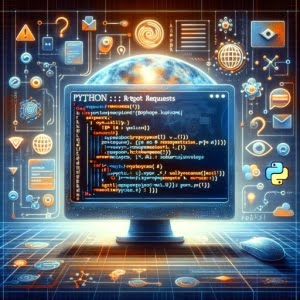
Are you grappling with HTTP requests in Python? Imagine having a seasoned negotiator to help you converse with the world wide web. That’s exactly what the Python requests library does for you.
This comprehensive guide is designed to usher you into the world of making HTTP requests in Python using the requests library. We’ll start from the rudimentary basics and gradually delve into more sophisticated techniques.
By the end of this guide, you’ll be able to wield Python requests like a pro, whether you’re fetching data from an API or automating tasks that involve web communication.
TL;DR: How Can I Use the Python Requests Library?
To use the Python requests library, you need to import it first, and then use its methods to make HTTP requests. Here’s a basic example of making a GET request:
import requests
response = requests.get('http://example.com')
print(response.text)
# Output:
# '<!doctype html>...</html>'
In this example, the requests.get()
method is used to send a GET request to ‘http://example.com’. The server’s response is stored in the response
object. Printing response.text
displays the HTML content of the webpage.
This is the crux of using the Python requests library, but there’s so much more you can do with it. Keep reading for a more in-depth explanation and to explore advanced usage scenarios.
Table of Contents
- Making GET and POST Requests with Python
- Advanced Python Requests: Cookies, Headers, and Timeouts
- Exploring Alternatives: urllib and httplib
- Troubleshooting Common Issues with Python Requests
- Understanding HTTP Requests
- Leveraging Python Requests for Larger Projects
- Harnessing the Power of Python Requests
Making GET and POST Requests with Python
The Python requests library is primarily used to send all types of HTTP requests. However, GET and POST requests are the most common. Let’s break down how to make these requests using Python requests.
Sending a GET Request
A GET request is used to retrieve data from a server. Here’s how you can send a GET request using Python requests:
import requests
response = requests.get('http://example.com')
print(response.text)
# Output:
# '<!doctype html>...</html>'
In this example, requests.get('http://example.com')
sends a GET request to the specified URL. The server’s response is stored in the response
object. response.text
contains the server’s response as a string.
Sending a POST Request
A POST request is used to send data to a server. Here’s an example of how to send a POST request:
import requests
data = {'key': 'value'}
response = requests.post('http://example.com', data = data)
print(response.text)
# Output:
# 'Response from server'
In this example, requests.post('http://example.com', data = data)
sends a POST request to the specified URL. The data
parameter contains the data you want to send. The server’s response is stored in the response
object.
These are the basics of using Python requests for HTTP communication. Once you’ve mastered these, you can start exploring more advanced features of the library.
Advanced Python Requests: Cookies, Headers, and Timeouts
The Python requests library is more than just GET and POST requests. It has several advanced features that allow you to fine-tune your HTTP requests. Let’s dive into some of these features.
Handling Cookies
Cookies are small pieces of data stored in the user’s browser. They are used for session management, tracking user activity, and storing user preferences. Here’s how you can handle cookies using Python requests:
import requests
response = requests.get('http://example.com')
# Print the cookies
print(response.cookies)
# Output:
# <RequestsCookieJar[Cookie(version=0, name='cookie_name', value='cookie_value', port=None, port_specified=False, domain='', domain_specified=False, domain_initial_dot=False, path='/', path_specified=True, secure=False, expires=None, discard=True, comment=None, comment_url=None, rest={'HttpOnly': None}, rfc2109=False)]>
In this example, response.cookies
contains the cookies sent by the server.
Sending Headers
Headers provide additional parameters for HTTP requests. Here’s how you can send headers using Python requests:
import requests
headers = {'User-Agent': 'my-app/0.0.1'}
response = requests.get('http://example.com', headers=headers)
print(response.text)
# Output:
# 'Response from server'
In this example, requests.get('http://example.com', headers=headers)
sends a GET request with the specified headers.
Dealing with Timeouts
Timeouts are used to set a limit on the amount of time you’re willing to wait for a response. Here’s how you can handle timeouts using Python requests:
import requests
try:
response = requests.get('http://example.com', timeout=1)
print(response.text)
except requests.exceptions.Timeout:
print('The request timed out')
# Output:
# 'The request timed out'
In this example, requests.get('http://example.com', timeout=1)
sends a GET request and waits for a response for a maximum of 1 second. If the server doesn’t respond within 1 second, a requests.exceptions.Timeout
exception is raised.
These are just a few of the advanced features of the Python requests library. By mastering these, you can make your HTTP communication more precise and efficient.
Exploring Alternatives: urllib and httplib
While the Python requests library is a powerful tool for making HTTP requests, it’s not the only option. Python also provides other libraries such as urllib and httplib. Let’s compare these libraries to the requests library and discuss their pros and cons.
Using urllib for HTTP Requests
urllib is a Python module for fetching URLs. Here’s an example of how to make a GET request using urllib:
import urllib.request
response = urllib.request.urlopen('http://example.com')
html = response.read()
print(html)
# Output:
# b'<!doctype html>...</html>'
In this example, urllib.request.urlopen('http://example.com')
sends a GET request to the specified URL. The server’s response is stored in the response
object. response.read()
returns the server’s response as bytes.
One advantage of urllib is that it’s part of the Python Standard Library, so you don’t need to install anything to use it. However, urllib’s API is less user-friendly compared to requests. For example, to send a POST request with urllib, you need to encode your data as bytes and create a urllib.request.Request
object.
Using httplib for HTTP Requests
httplib is another Python module for making HTTP requests. Here’s an example of how to make a GET request using httplib:
import http.client
conn = http.client.HTTPConnection('www.example.com')
conn.request('GET', '/')
response = conn.getresponse()
print(response.read())
# Output:
# b'<!doctype html>...</html>'
In this example, http.client.HTTPConnection('www.example.com')
creates a connection to the specified server. conn.request('GET', '/')
sends a GET request to the server. The server’s response is stored in the response
object. response.read()
returns the server’s response as bytes.
Like urllib, httplib is part of the Python Standard Library. However, httplib’s API is even more low-level than urllib’s. For example, httplib doesn’t automatically handle redirects or cookies. This makes httplib more flexible than urllib or requests, but also more difficult to use.
In conclusion, while urllib and httplib offer alternative ways to make HTTP requests in Python, the requests library provides a more user-friendly and high-level API. Depending on your needs, you might prefer one over the others.
Troubleshooting Common Issues with Python Requests
While the Python requests library simplifies HTTP communication, you might still encounter some issues. Let’s discuss some common problems and their solutions.
Handling Errors and Exceptions
Python requests raises exceptions for network errors, timeouts, and invalid URLs. Here’s how you can handle these exceptions:
import requests
try:
response = requests.get('http://example.com')
except requests.exceptions.RequestException as error:
print(f'An error occurred: {error}')
# Output:
# 'An error occurred: HTTPConnectionPool(host='example.com', port=80): Max retries exceeded with url: / (Caused by NewConnectionError('<urllib3.connection.HTTPConnection object at 0x7f8c0a3e3c50>: Failed to establish a new connection: [Errno -2] Name or service not known'))'
In this example, requests.get('http://example.com')
is wrapped in a try-except block. If a requests.exceptions.RequestException
is raised, the error message is printed.
Best Practices to Avoid Issues
Here are some best practices to avoid issues when using Python requests:
- Always use a timeout. This prevents your program from hanging if the server doesn’t respond.
- Handle exceptions. This allows your program to fail gracefully in case of errors.
- Use sessions for multiple requests to the same server. This can improve performance by reusing the same TCP connection.
- Close the response stream when you’re done with it. This can prevent resource leaks.
By following these best practices, you can avoid many common issues when using the Python requests library.
Understanding HTTP Requests
HTTP stands for Hypertext Transfer Protocol. It’s the foundation of any data exchange on the Web, and it’s a protocol used for transmitting hypertext requests and information between server and browser. Python requests library leverages this protocol to allow HTTP requests to be sent using python.
The Anatomy of HTTP Requests
An HTTP request consists of two parts: the request line and the headers. The request line contains the HTTP method (GET, POST, etc.), the request URL, and the HTTP version. The headers provide additional information about the request or the client, such as the user agent or the accepted content types.
Here’s an example of an HTTP request:
GET / HTTP/1.1
Host: www.example.com
User-Agent: python-requests/2.25.1
Accept-Encoding: gzip, deflate
Accept: */*
Connection: keep-alive
In this example, GET / HTTP/1.1
is the request line. It indicates that the client wants to retrieve (GET) the root page (/) using HTTP version 1.1. The rest of the lines are the headers.
Types of HTTP Requests
There are several types of HTTP requests, also known as ‘HTTP methods’. Here are the most commonly used ones:
- GET: Retrieves information from the server.
- POST: Sends new information to the server.
- PUT: Updates existing information on the server.
- DELETE: Removes existing information from the server.
Each of these methods corresponds to a specific action on the server. The Python requests library provides a method for each of these HTTP methods, which makes it very easy to send HTTP requests.
Leveraging Python Requests for Larger Projects
The Python requests library isn’t just for making simple HTTP requests. It’s a versatile tool that can be used in larger projects such as web scraping or API integration. Let’s explore these use cases in more detail.
Web Scraping with Python Requests
Web scraping is the process of extracting data from websites. Python requests can be used to retrieve the HTML content of a webpage, which can then be parsed to extract useful information.
import requests
from bs4 import BeautifulSoup
response = requests.get('http://example.com')
soup = BeautifulSoup(response.text, 'html.parser')
# Print the title of the webpage
print(soup.title.string)
# Output:
# 'Example Domain'
In this example, requests.get('http://example.com')
retrieves the HTML content of the webpage. BeautifulSoup(response.text, 'html.parser')
parses the HTML content. soup.title.string
extracts the title of the webpage.
API Integration with Python Requests
APIs (Application Programming Interfaces) are used to interact with external services. Python requests can be used to send API requests and handle the responses.
import requests
response = requests.get('https://api.example.com/data')
data = response.json()
# Print the data
print(data)
# Output:
# {'key': 'value'}
In this example, requests.get('https://api.example.com/data')
sends a GET request to the API. response.json()
parses the JSON response from the API.
Further Reading
If you want to dive deeper into Python requests, here are some related topics you might find interesting:
- Python Web Scraping Use Cases Explored – Master data extraction from tables and structured content in Python.
Simplifying Web Connectivity with Python curl – Dive into making HTTP requests and interacting with APIs using “curl”.
Integrating APIs in Python: A Quick Guide – Dive into API endpoints, authentication, and data retrieval with Python.
Python Guides on Web Scraping – Peruse this Python guide offering an overview of web scraping scenarios and practices.
Udacity’s Tutorial on Web Scraping with Python Requests explains how to perform web scraping with Python Requests.
DataCamp’s Tutorial on Web Scraping Using Python can enhance your skill set by learning web scraping with Python.
Harnessing the Power of Python Requests
Throughout this guide, we’ve explored the extensive capabilities of the Python requests library for making HTTP requests. From basic GET and POST requests to handling cookies, headers, and timeouts, we’ve seen that Python requests is a versatile tool for web communication.
We’ve also addressed common issues you might encounter when using Python requests, such as handling errors and exceptions. Remember, applying best practices like using timeouts, handling exceptions, and closing response streams can help avoid these issues.
In our quest for mastery, we ventured into alternative libraries like urllib and httplib. While these offer different ways to make HTTP requests, Python requests provides a more user-friendly and high-level API, making it a preferred choice for many.
To wrap up, let’s look at a comparison of the discussed methods:
Method | Pros | Cons |
---|---|---|
Python Requests | User-friendly, High-level API, Advanced features | External library |
urllib | Part of Standard Library | Less user-friendly API |
httplib | Part of Standard Library, More flexible | Low-level API |
This table summarizes the pros and cons of Python requests, urllib, and httplib. Depending on your needs, you might prefer one over the others.
Remember, the Python requests library isn’t just for simple HTTP requests. It’s a powerful tool that can be leveraged for larger projects like web scraping or API integration. So, the next time you’re dealing with HTTP requests in Python, you know which library to turn to!