Python Web Scraping: Complete Guide
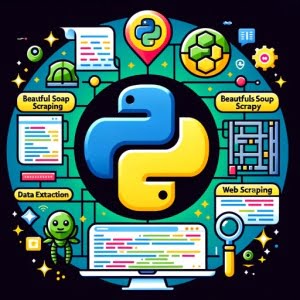
Ever wondered how to extract data from websites using Python? Like a digital miner, Python can dig up valuable data from the web. It’s a skill that’s not only fascinating but also highly useful in the era of data-driven decisions.
This guide will walk you through the process of web scraping in Python, from basic to advanced techniques. We’ll cover everything from setting up your Python environment for web scraping, using libraries like BeautifulSoup and Selenium, to handling dynamic content and even troubleshooting common issues.
Let’s dive in and start mastering Python web scraping!
TL;DR: How Do I Perform Web Scraping in Python?
To perform web scraping in Python, you can use libraries like BeautifulSoup. This library allows you to extract data from websites by parsing HTML and XML documents.
Here’s a simple example:
from bs4 import BeautifulSoup
import requests
url = 'http://example.com'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
print(soup.prettify())
# Output:
# <!DOCTYPE html>
# <html>
# <head>
# <title>
# Example Domain
# </title>
# ...
# </body>
# </html>
In this example, we first import the necessary libraries: BeautifulSoup for parsing the HTML content and requests for making HTTP requests. We then define the URL of the webpage we want to scrape. Using the requests.get(url)
function, we send a GET request to the URL and receive the response. The response text, which is the HTML content of the webpage, is then parsed using BeautifulSoup. Finally, we print the parsed HTML content in a more readable format using the prettify()
method.
This is a basic way to perform web scraping in Python, but there’s much more to learn about handling dynamic content, using other libraries like Selenium and Scrapy, and troubleshooting common issues. Continue reading for a more detailed guide on web scraping in Python.
Table of Contents
- Basic Python Web Scraping: BeautifulSoup
- Handling Dynamic Content: Selenium
- Alternative Python Web Scraping Techniques: Scrapy and APIs
- Troubleshooting Python Web Scraping Issues
- Understanding Web Scraping Fundamentals
- Beyond Basic Python Web Scraping: Data Analysis, Machine Learning, and More
- Wrapping Up: Mastering Python Web Scraping
Basic Python Web Scraping: BeautifulSoup
BeautifulSoup is a Python library that is used for web scraping purposes to pull the data out of HTML and XML files. It creates a parse tree from page source code that can be used to extract data in a hierarchical and more readable manner.
Let’s see how to use BeautifulSoup to scrape a simple static website.
First, we install BeautifulSoup using pip:
pip install beautifulsoup4
Then, we use it in our Python script. Let’s say we want to extract all the links from a webpage. Here is a simple example:
from bs4 import BeautifulSoup
import requests
url = 'http://example.com'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
for link in soup.find_all('a'):
print(link.get('href'))
# Output:
# http://www.iana.org/domains/example
In the above example, first we send a GET request to the URL using requests.get(url)
. The response, which is the HTML content of the webpage, is then parsed using BeautifulSoup. We then find all the ‘a’ tags (which define hyperlinks in HTML) using soup.find_all('a')
and print the URLs using link.get('href')
.
This is a basic example of how you can use BeautifulSoup to scrape static websites. This library is very powerful and flexible, and you can use it to extract all kinds of data from web pages. However, it’s important to note that BeautifulSoup cannot handle dynamic content (content that is loaded using JavaScript). For that, we would need to use other tools, which we will discuss in the next section.
Handling Dynamic Content: Selenium
While BeautifulSoup is a powerful tool for scraping static websites, it falls short when it comes to handling dynamic content. Dynamic content refers to parts of a website that are loaded or changed using JavaScript after the initial page load. For this, we need to use tools like Selenium.
Selenium is a web testing library used to automate browser activities. It can interact with all kinds of web elements, making it a good choice for scraping dynamic content.
First, we need to install Selenium. We also need to install a WebDriver, which is a tool that Selenium uses to interact with the browser. In this example, we’ll use the Chrome WebDriver.
pip install selenium
You can download the Chrome WebDriver from the Chrome WebDriver website. Make sure to download the version that matches the version of Chrome installed on your system.
Once you have downloaded the WebDriver, you need to add it to your system path. On Windows, you can do this by adding the path to the WebDriver to your PATH environment variable. On macOS and Linux, you can move the WebDriver to /usr/local/bin
, which is a directory that is included in the system path by default.
Now that Selenium and the WebDriver are installed, let’s see how we can use them to scrape a dynamic website. In this example, we’ll scrape a website that uses JavaScript to load content:
from selenium import webdriver
url = 'http://example.com'
# Initialize the WebDriver and open the URL
driver = webdriver.Chrome()
driver.get(url)
# Wait for the JavaScript to load
driver.implicitly_wait(10)
# Extract the data
data = driver.page_source
print(data)
# Close the WebDriver
driver.quit()
# Output:
# <!DOCTYPE html>
# <html>
# <head>
# <title>
# Example Domain
# </title>
# ...
# </body>
# </html>
In the above example, we first initialize the WebDriver and open the URL using driver.get(url)
. We then wait for the JavaScript to load using driver.implicitly_wait(10)
, which tells the WebDriver to wait up to 10 seconds for the JavaScript to load. After that, we extract the data from the page using driver.page_source
and print it. Finally, we close the WebDriver using driver.quit()
.
This is a basic example of how you can use Selenium to scrape dynamic websites. Selenium is a very powerful tool that can handle all kinds of web elements and interactions, making it a great choice for scraping complex, dynamic websites.
Alternative Python Web Scraping Techniques: Scrapy and APIs
While BeautifulSoup and Selenium are powerful tools for web scraping, there are other libraries and techniques that you might find useful, depending on your needs. Let’s explore a couple of these alternatives: Scrapy and APIs.
Scrapy: A Powerful Web Scraping Framework
Scrapy is an open-source Python framework for large scale web scraping. It provides all the tools you need to extract data from websites, process it, and store it in your preferred format. Scrapy is a versatile framework that can handle a wide range of scraping tasks.
Here is a simple example of how to use Scrapy to scrape a website:
pip install scrapy
import scrapy
from scrapy.crawler import CrawlerProcess
class MySpider(scrapy.Spider):
name = 'example_spider'
start_urls = ['http://example.com']
def parse(self, response):
self.log('Visited %s' % response.url)
process = CrawlerProcess({
'USER_AGENT': 'Mozilla/4.0 (compatible; MSIE 7.0; Windows NT 5.1)'
})
process.crawl(MySpider)
process.start()
# Output:
# 2022-02-20 16:20:10 [example_spider] INFO: Visited http://example.com
In this example, we define a Scrapy Spider, which is a class that defines how to follow links on the website and extract data. The parse
method is where we define how to process the webpage and extract data.
APIs: A Clean and Respectful Approach
If the website you’re trying to scrape provides an API, it’s almost always a better idea to use the API rather than scraping the website directly. APIs provide a clean and respectful way to extract data from a website. They are designed to be used by programs and are usually much more stable and faster than scraping the website directly.
Here is a simple example of how to use the requests library to call an API:
import requests
response = requests.get('http://api.example.com/data')
data = response.json()
print(data)
# Output:
# {'key': 'value', 'key2': 'value2'}
In this example, we use the requests.get()
function to send a GET request to the API endpoint, and then we use the response.json()
method to parse the JSON response from the API.
These are just a couple of the alternative approaches to web scraping in Python. Depending on your needs, you might find one of these methods to be more suitable than BeautifulSoup or Selenium. As always, it’s important to respect the website’s terms of service and to scrape responsibly.
Troubleshooting Python Web Scraping Issues
While Python’s web scraping capabilities are powerful and flexible, you might still run into some common issues. Let’s discuss how to handle different website structures, deal with ‘robots.txt’ files, and find solutions to these problems.
Handling Different Website Structures
Different websites have different structures, which can make it challenging to write a universal web scraping script. When you encounter a website with a different structure, you might need to adjust your script to handle the new structure.
For example, if a website uses a different tag for its links, you would need to adjust your BeautifulSoup or Scrapy script to look for that tag. Similarly, if a website loads its content dynamically, you might need to use Selenium to interact with the JavaScript on the page.
Dealing with ‘robots.txt’ Files
The ‘robots.txt’ file is a file that webmasters use to give instructions about their site to web robots. This file is usually located at the root of the website (e.g., http://example.com/robots.txt).
Some websites use the ‘robots.txt’ file to ask web scrapers not to scrape certain parts of the site. While this file is not legally binding, it’s considered good web scraping etiquette to respect the wishes of the webmaster as expressed in the ‘robots.txt’ file.
Here’s how you can check the ‘robots.txt’ file of a website using Python:
import requests
response = requests.get('http://example.com/robots.txt')
print(response.text)
# Output:
# User-agent: *
# Disallow: /private
In this example, the ‘robots.txt’ file is asking all web scrapers (denoted by the User-agent: *
) not to scrape the ‘/private’ part of the site.
Solutions for Common Problems
When you encounter a problem while web scraping, the first step is to understand the problem. This might involve reading the error message, checking the website’s structure, or looking at the ‘robots.txt’ file.
Once you understand the problem, you can start looking for a solution. This might involve adjusting your script to handle a different website structure, using a different library to handle dynamic content, or respecting the wishes of the webmaster as expressed in the ‘robots.txt’ file.
Remember, web scraping is a powerful tool, but with great power comes great responsibility. Always respect the website’s terms of service and scrape responsibly.
Understanding Web Scraping Fundamentals
To master Python web scraping, it’s essential to understand the fundamentals of how web scraping works. Let’s delve into the core concepts, including HTTP requests, HTML parsing, and the Document Object Model (DOM).
HTTP Requests: The Foundation of Web Scraping
HTTP stands for Hypertext Transfer Protocol, and it’s the protocol used for transferring data over the internet. When you visit a website, your browser sends an HTTP request to the server where the website is hosted. The server then responds with the data that makes up the website, which your browser renders as the web page you see.
In Python web scraping, we use libraries like requests
to send HTTP requests and receive the server’s response. Here’s a basic example:
import requests
response = requests.get('http://example.com')
print(response.text)
# Output:
# <!DOCTYPE html>
# <html>
# <head>
# <title>
# Example Domain
# </title>
# ...
# </body>
# </html>
In this example, we send a GET request to ‘http://example.com’ and print the response text, which is the HTML content of the website.
HTML Parsing: Extracting Data from the Response
HTML stands for Hypertext Markup Language, and it’s the standard markup language for documents designed to be displayed in a web browser. The data we want to scrape from a website is contained in the HTML of the web page.
In Python web scraping, we use libraries like BeautifulSoup to parse the HTML content and extract the data we need. The parsing process involves traversing the HTML tree structure and finding the tags that contain our desired data.
Document Object Model (DOM): Navigating the HTML Tree
The Document Object Model (DOM) is a programming interface for HTML documents. It represents the structure of a web page as a tree, where each node is an object representing a part of the page. This tree-like structure makes it easy to navigate the HTML document and find the data we need.
In Python web scraping, we use the DOM to navigate the HTML content and find the tags that contain our desired data. Libraries like BeautifulSoup and Selenium provide methods for navigating the DOM and finding specific elements.
Understanding these fundamentals is key to mastering Python web scraping. Once you understand how HTTP requests work, how to parse HTML content, and how to navigate the DOM, you’ll be well-equipped to handle any web scraping task.
Beyond Basic Python Web Scraping: Data Analysis, Machine Learning, and More
Python web scraping is a powerful tool that goes beyond simply extracting data from websites. It opens up a world of possibilities in data analysis, machine learning, web automation, and more. Let’s explore some of these broader applications.
Data Analysis: Making Sense of the Data
Once you’ve scraped data from a website, you can use Python’s data analysis libraries to analyze the data and gain insights. For example, you could use pandas to clean and analyze the data, and matplotlib to visualize the results.
Here’s a simple example of how you could use pandas to analyze scraped data:
import pandas as pd
data = {'Name': ['Alice', 'Bob', 'Charlie'], 'Age': [25, 30, 35]}
df = pd.DataFrame(data)
print(df.describe())
# Output:
# Age
# count 3.000000
# mean 30.000000
# std 5.000000
# min 25.000000
# 25% 27.500000
# 50% 30.000000
# 75% 32.500000
# max 35.000000
In this example, we create a pandas DataFrame from our scraped data and use the describe()
method to generate descriptive statistics of the data.
Machine Learning: Predicting the Future
With enough data, you can use Python’s machine learning libraries to create models that predict future outcomes. For example, you could use scikit-learn to create a regression model that predicts house prices based on scraped real estate data.
Web Automation: Doing More with Less
Python web scraping can also be used for web automation. For example, you could write a script that automatically logs into a website, navigates to a specific page, fills out a form, or performs other actions. Selenium, which we discussed earlier, is a great tool for this.
Further Resources for Python Web Scraping Mastery
- Beautiful Soup in Python: Web Scraping Simplified – Learn how to parse and scrape web pages using Python’s Beautiful Soup library.
Beautiful Soup Documentation – A comprehensive guide to using Beautiful Soup for web scraping in Python.
Selenium with Python – An in-depth guide to using Selenium with Python for web scraping dynamic websites.
Scrapy Tutorial – A step-by-step guide to using Scrapy, a powerful Python framework for large scale web scraping.
These resources provide a wealth of information that can help you deepen your understanding of Python web scraping and explore its broader applications. Happy scraping!
Wrapping Up: Mastering Python Web Scraping
In this comprehensive guide, we’ve delved into the world of Python web scraping, from basic to advanced techniques. We’ve explored how Python can be used as a powerful tool to extract data from websites, a skill that is becoming increasingly important in our data-driven world.
We began with the basics, learning how to use BeautifulSoup for scraping static websites. We then navigated through handling dynamic content using Selenium, and discussed alternative approaches like Scrapy and APIs. Along the way, we tackled common challenges you might face when web scraping, such as handling different website structures and dealing with ‘robots.txt’ files, providing you with solutions for each issue.
We also looked at the broader applications of Python web scraping, from data analysis and machine learning to web automation. Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
BeautifulSoup | Easy to use, great for static websites | Cannot handle dynamic content |
Selenium | Can handle dynamic content, interacts with JavaScript | More complex than BeautifulSoup |
Scrapy | Powerful, great for large scale scraping | Requires learning a new framework |
APIs | Clean and respectful, usually faster and more stable | Not always available |
Whether you’re a beginner just starting out with Python web scraping or an experienced developer looking to level up your web scraping skills, we hope this guide has given you a deeper understanding of how to extract data from websites using Python and the power of this tool. Happy scraping!