Python API Integration: Comprehensive Guide
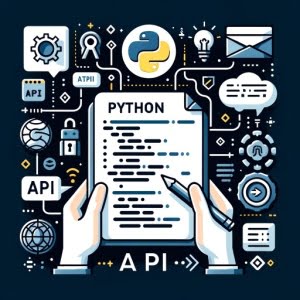
Ever felt like you’re wrestling with using APIs from Python? You’re not alone. Many developers find Python API access a bit daunting. Think of Python’s API access as a bridge – a bridge that connects your Python code to external services or applications.
APIs are a powerful way to extend the functionality of your Python scripts, making APIs extremely popular for accessing external services or data sources.
In this guide, we’ll walk you through the process of using Python for APIs, from the basics to more advanced techniques. We’ll cover everything from making simple API calls using the requests library, handling different types of API calls (GET, POST, PUT, DELETE), to dealing with API responses and even troubleshooting common issues.
Let’s get started!
TL;DR: How Do I Use Python to make API calls?
To use Python to make API calls, you typically import that API’s library and make API calls using provided functions. An alternative is to use the
requests
module to connect to API’s with direct GET or POST requests, after reviewing that API’s documented endpoints. APIs are fundamental in Python programming and allows your code to interact with external services or applications.
Here’s a simple example using the requests library to make a GET request:
import requests
response = requests.get('https://api.example.com')
print(response.json())
# Output:
# {'key': 'value'}
In this example, we import the requests library, which is a popular Python library for making HTTP requests. We then make a GET request to ‘https://api.example.com’. The response from the server is converted into a Python dictionary using the .json()
method and then printed out.
This is just the tip of the iceberg when it comes to using Python API. Keep reading for more detailed examples and advanced usage scenarios.
Table of Contents
- Diving into Python API: Basic Use
- Deep Dive into Python API: Advanced Use
- Exploring Alternative Approaches in Python API
- Troubleshooting Python API: Common Issues and Solutions
- Understanding APIs and HTTP Protocol
- Python API: The Gateway to Web Scraping, Data Analysis, and More
- Wrapping Up: Mastering Python API
Diving into Python API: Basic Use
Python is known for its simplicity and readability, and this extends to its approach to APIs. At the most basic level, interacting with APIs in Python involves making HTTP requests to a server somewhere on the internet. The requests
library is the de facto standard for making these HTTP requests.
Making Your First API Call
Let’s start with a simple example of how to make an API call using the requests
library:
import requests
url = 'https://api.example.com'
response = requests.get(url)
print(response.json())
# Output:
# {'key': 'value'}
In this example, we’re making a GET request to ‘https://api.example.com’. The requests.get(url)
function sends a GET request to the specified URL and returns a response object. The .json()
method is then used to convert the response into a Python dictionary, which is then printed out.
Understanding the Function and Its Advantages
The requests
library is incredibly user-friendly and flexible. It abstracts the complexities of making requests behind a simple API, allowing you to send HTTP/1.1 requests with various methods like GET, POST, and others. With it, you can send HTTP requests in Python in just a few lines of code.
Potential Pitfalls
While requests
is powerful and easy to use, it’s important to handle potential errors and exceptions that may arise during the execution of your program. For instance, if the server is down or the URL is incorrect, requests.get(url)
will throw an error. Therefore, it’s good practice to use try/except
blocks to catch and handle these exceptions.
import requests
url = 'https://api.example.com'
try:
response = requests.get(url)
print(response.json())
except requests.exceptions.RequestException as e:
print(f'Request failed: {e}')
# Output:
# Request failed: HTTPConnectionPool(host='api.example.com', port=80): Max retries exceeded with url: / (Caused by NewConnectionError('<urllib3.connection.HTTPConnection object at 0x7f3b279fc810>: Failed to establish a new connection: [Errno -2] Name or service not known'))
This way, even if the request fails, your program will not crash and can continue executing subsequent code.
Deep Dive into Python API: Advanced Use
As you become more comfortable with Python APIs, you’ll need to understand different types of API calls and how to handle their responses. This is crucial as different API endpoints might require different types of requests, and the responses can vary greatly.
Mastering Different API Calls
In addition to GET requests, there are several other types of HTTP requests that you can make with the requests
library. Here’s a brief overview of each:
- GET: Fetches data from a server. Data is passed in the URL of the request.
POST: Sends data to a server to create a new resource. Data is passed in the body of the request.
PUT: Updates an existing resource on a server. Like POST, data is passed in the body of the request.
DELETE: Deletes an existing resource on a server.
Let’s see how to make these different types of requests in Python:
import requests
# GET
response = requests.get('https://api.example.com/data')
print(response.json())
# POST
response = requests.post('https://api.example.com/data', data={'key': 'value'})
print(response.json())
# PUT
response = requests.put('https://api.example.com/data/1', data={'key': 'new-value'})
print(response.json())
# DELETE
response = requests.delete('https://api.example.com/data/1')
print(response.status_code)
# Output:
# {'key': 'value'}
# {'id': 1, 'key': 'value'}
# {'id': 1, 'key': 'new-value'}
# 204
In this example, we’re making GET, POST, PUT, and DELETE requests to ‘https://api.example.com/data’. The requests.post()
and requests.put()
methods take an additional data
parameter where you can pass the data you want to send to the server.
Analyzing API Responses
Understanding the response from an API call is just as important as making the call. The response
object returned by all requests
functions contains the server’s response to your HTTP request. This includes the status code, headers, and the response body.
The status code tells you whether the request was successful, and if not, why. For example, a status code of 200 means ‘OK’, while a status code of 404 means ‘Not Found’. The headers can give you useful information like the content type of the response body. The response body is where the data you requested is stored.
Here’s how you can access this information:
import requests
response = requests.get('https://api.example.com/data')
print('Status Code:', response.status_code)
print('Headers:', response.headers)
print('Body:', response.json())
# Output:
# Status Code: 200
# Headers: {'Content-Type': 'application/json', 'Date': 'Tue, 14 Dec 2021 13:36:35 GMT', 'Server': 'api.example.com'}
# Body: {'key': 'value'}
In this example, we’re printing the status code, headers, and body of the response from a GET request to ‘https://api.example.com/data’.
Exploring Alternative Approaches in Python API
While the requests
library is a popular choice for interacting with APIs in Python, it’s not the only option. Python’s standard library includes modules like urllib
and httplib
that provide lower-level interfaces for making HTTP requests. These can offer more control and flexibility in certain situations.
Interacting with APIs using urllib
The urllib
module in Python’s standard library provides functions for making HTTP requests. This can be a good option if you want to avoid external dependencies, but it can be a bit more complex to use than requests
.
Here’s an example of how to make a GET request using urllib
:
import urllib.request
import json
url = 'https://api.example.com/data'
with urllib.request.urlopen(url) as response:
data = json.loads(response.read().decode())
print(data)
# Output:
# {'key': 'value'}
In this example, we’re using the urlopen
function from urllib.request
to make a GET request to ‘https://api.example.com/data’. The response is read and decoded into a Python string, which is then converted into a dictionary using json.loads()
.
Making HTTP Requests with httplib
The httplib
module provides a low-level interface for making HTTP and HTTPS requests. It can be more verbose and complex to use than requests
or urllib
, but it can also offer more control and flexibility.
Here’s an example of a GET request using httplib
:
import http.client
import json
conn = http.client.HTTPSConnection('api.example.com')
conn.request('GET', '/data')
response = conn.getresponse()
data = json.loads(response.read().decode())
print(data)
# Output:
# {'key': 'value'}
In this example, we’re creating a connection to ‘api.example.com’ using http.client.HTTPSConnection
, and then making a GET request to ‘/data’. The response is read and decoded into a Python string, which is then converted into a dictionary using json.loads()
.
Choosing the Right Tool for the Job
While requests
is generally the easiest and most intuitive library for making HTTP requests in Python, urllib
and httplib
can be useful alternatives in certain situations. If you need more control over your HTTP requests, or if you want to avoid external dependencies, these modules from Python’s standard library can be good options.
However, they can also be more complex and verbose to use than requests
. If you’re just getting started with Python APIs, we recommend sticking with requests
until you’re more comfortable.
Troubleshooting Python API: Common Issues and Solutions
While working with Python API, you might encounter certain issues. These can range from handling errors and timeouts to dealing with rate limits. It’s important to know how to troubleshoot these issues to ensure your program runs smoothly.
Handling Errors
When making API calls, there’s always a chance that something might go wrong. The server might be down, the URL might be incorrect, or there might be a problem with the network. It’s crucial to handle these errors correctly in your code to avoid crashes.
Here’s an example of how to handle errors using try/except
:
import requests
url = 'https://api.example.com'
try:
response = requests.get(url)
response.raise_for_status()
except requests.exceptions.HTTPError as err:
print(f'HTTP error occurred: {err}')
except requests.exceptions.RequestException as err:
print(f'There was a problem with your request: {err}')
# Output:
# HTTP error occurred: 404 Client Error: Not Found for url: https://api.example.com/
In this example, we’re using the raise_for_status()
method, which will raise a HTTPError
if the response was unsuccessful. This allows us to catch and handle HTTP errors separately from other types of errors.
Dealing with Timeouts
API calls can sometimes take longer than expected. This could be due to network latency, server problems, or a host of other issues. To prevent your program from hanging indefinitely, you can use the timeout
parameter in requests
:
import requests
url = 'https://api.example.com'
try:
response = requests.get(url, timeout=5)
print(response.json())
except requests.exceptions.Timeout:
print('The request timed out')
except requests.exceptions.RequestException as err:
print(f'There was a problem with your request: {err}')
# Output:
# The request timed out
In this example, if the response is not received within 5 seconds, a Timeout
exception is raised.
Managing Rate Limits
Many APIs impose a limit on the number of requests you can make in a certain period of time. If you exceed this limit, the server might respond with a 429 Too Many Requests
status code.
You can handle this in your code by catching the HTTPError
and checking if the status code is 429:
import requests
import time
url = 'https://api.example.com'
while True:
try:
response = requests.get(url)
response.raise_for_status()
break
except requests.exceptions.HTTPError as err:
if response.status_code == 429:
time.sleep(1) # wait for 1 second before trying again
else:
raise err
print(response.json())
# Output:
# {'key': 'value'}
In this example, if a 429 Too Many Requests
status code is received, the program waits for 1 second before trying again.
Understanding APIs and HTTP Protocol
Before we dive deeper into Python APIs, it’s essential to have a firm grasp of what APIs are and how they work, as well as an understanding of the HTTP protocol that underpins them.
What are APIs?
APIs, or Application Programming Interfaces, are sets of rules and protocols that allow different software applications to communicate with each other. They define the methods and data formats that a program can use to communicate with the software component, be it an operating system, service, or another application.
In the context of web development, APIs are often built as HTTP-based web services that can be accessed via HTTP protocol. They allow your application to interact with external services and data via endpoints, which are essentially specific URLs where your API can access the resources it needs.
How Do APIs Work?
APIs work as a contract between different software applications. When an application wants to access a particular resource, it sends a request to the API with a specific set of instructions. The API processes the request, interacts with the necessary services or databases, and then returns the requested data to the application.
Here’s a simple analogy: think of an API as a restaurant menu. The menu provides a list of dishes you can order along with a description of each dish. When you specify what menu items you want, the restaurant’s kitchen does the work and provides you with some finished dishes. You don’t know exactly how the kitchen prepares those dishes, and you don’t really need to. Similarly, an API lists a bunch of operations that a developer can use, along with a description of what they do. The developer doesn’t need to know how, for example, an operating system builds and manages a file. They just need to know that there’s an API function with a particular name that can be used to create a file, and what parameters to pass to it.
Understanding the HTTP Protocol
HTTP stands for Hypertext Transfer Protocol, and it’s the foundation of any data exchange on the Web. HTTP is a protocol that is used to send and receive data over the internet. When you type a URL into your browser, you are actually sending an HTTP request to a server for the webpage associated with that URL. The server then sends back an HTTP response with the webpage’s content.
HTTP is a stateless protocol, which means each request is processed independently, without any knowledge of the requests that came before it. This is where HTTP methods come in. They indicate the desired action to be performed on the identified resource.
GET, POST, PUT, and DELETE are some of the most common HTTP methods. Each has a specific purpose:
- GET: Retrieves information from the server.
- POST: Sends new information to the server.
- PUT: Updates existing information on the server.
- DELETE: Removes existing information from the server.
When we talk about using Python to make API calls, we’re essentially using Python to send HTTP requests to a server somewhere on the internet.
Python API: The Gateway to Web Scraping, Data Analysis, and More
Python APIs are not just about fetching data from a server or interacting with a database. They open up a whole new world of possibilities for Python developers. From web scraping to data analysis, automation, and beyond, Python APIs are a powerful tool in a developer’s arsenal.
Python API for Web Scraping
Web scraping is a technique used to extract large amounts of data from websites. Python APIs play a critical role in this process. They allow your program to interact with the website’s server, send HTTP requests, and receive server responses.
Here’s a simple example of how you might use the requests
library to scrape data from a website:
import requests
url = 'https://api.example.com/data'
response = requests.get(url)
data = response.json()
print(data)
# Output:
# {'key': 'value'}
In this example, we’re sending a GET request to the server and receiving a JSON response, which we then print out.
Python API in Data Analysis
Python APIs are also a crucial tool in data analysis. They allow you to fetch data from various sources, which you can then analyze using Python’s powerful data analysis libraries like pandas and NumPy.
For example, you might use the requests
library to fetch data from a REST API, convert the JSON response into a pandas DataFrame, and then perform your analysis.
Automating Tasks with Python API
Python APIs are a great tool for automation. They allow your Python programs to interact with other software or services, automating tasks that would be time-consuming to perform manually.
For example, you might write a Python script that uses the GitHub API to automatically create issues or pull requests, or a script that uses the Google Sheets API to update a spreadsheet.
Exploring Related Concepts: JSON, OAuth, and More
As you delve deeper into Python APIs, you’ll encounter a host of related concepts and technologies. JSON, or JavaScript Object Notation, is a popular data format for APIs. OAuth is a standard for authorizing access to APIs. Understanding these concepts will greatly enhance your ability to work with Python APIs.
Further Resources for Python API Mastery
Ready to take your Python API skills to the next level? Here are some resources that can help:
- Python Web Scraping Essentials: Quick Reference – Discover how to extract structured information from web pages using Python.
Python requests Library: HTTP Requests – Discover the Python “requests” library for making HTTP requests and working with APIs.
Web Scraping with Beautiful Soup in Python – Dive into web scraping techniques for extracting data from HTML and XML documents.
Python API Tutorial: Getting Started with APIs provides an introduction to APIs in Python, including how to make API requests and handle API responses.
Python Requests Library Tutorial focuses on the
requests
library, a powerful tool for making HTTP requests in Python.Working with JSON Data in Python provides an in-depth look at JSON and how to work with JSON data in Python.
Wrapping Up: Mastering Python API
In this comprehensive guide, we’ve delved into the world of Python API, a powerful tool for interacting with external services and applications.
We began with the basics, understanding how to use Python API and making API calls using the requests library. We then moved onto more advanced usage, exploring different types of API calls such as GET, POST, PUT, and DELETE, and how to handle their responses. We also discussed how to use alternative libraries like urllib and httplib for making HTTP requests.
Along the way, we tackled common challenges one might face when using Python API, such as handling errors, timeouts, and rate limits, providing solutions and workarounds for each issue. We also provided a deep dive into what APIs are, how they work, and the importance of the HTTP protocol in API calls.
Finally, we looked at the broader impact of Python API, discussing its relevance in web scraping, data analysis, automation, and more. We also suggested related concepts to explore for deeper understanding, like JSON and OAuth.
Here’s a quick comparison of the libraries we’ve discussed:
Library | Simplicity | Flexibility | Control |
---|---|---|---|
requests | High | Moderate | Moderate |
urllib | Moderate | High | High |
httplib | Low | High | High |
Whether you’re a beginner just starting out with Python API or an experienced developer looking to level up your skills, we hope this guide has given you a deeper understanding of Python API and its capabilities.
With its balance of simplicity, flexibility, and control, Python API is a powerful tool for any developer. Happy coding!