Numpy reshape() Function: Python Array Reshaping Guide
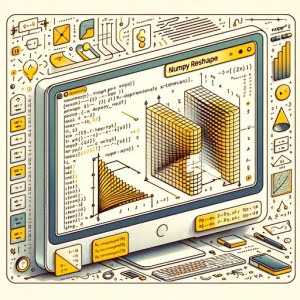
Struggling with reshaping arrays in Python? Just like a skilled potter, Numpy’s reshape() function allows you to mold your arrays into any shape you desire. Whether you’re a beginner or a seasoned Python developer, understanding how to reshape arrays can significantly streamline your data manipulation tasks.
In this comprehensive guide, we will explore the ins and outs of the numpy reshape function. From basic to advanced usage, you’ll learn how to manipulate your arrays effectively, saving you time and making your code more efficient.
So, if you’re ready to master the art of array reshaping in Python using numpy reshape, let’s dive in!
TL;DR: How Do I Reshape Arrays in Python Using Numpy?
The numpy reshape() function allows you to change the shape of an array without altering its data. It’s a powerful tool in Python for array manipulation. Here’s a simple example:
import numpy as np
arr = np.array([1, 2, 3, 4, 5, 6])
reshaped_arr = arr.reshape(2, 3)
print(reshaped_arr)
# Output:
# [[1 2 3]
# [4 5 6]]
In this example, we’ve reshaped a one-dimensional array with six elements into a two-dimensional array with two rows and three columns using numpy’s reshape function. The original data remains intact, but the structure of the array has changed.
Interested in learning more? Continue reading to delve deeper into the numpy reshape function and its advanced uses.
Table of Contents
- Harnessing Numpy Reshape: Basic Use
- Numpy Reshape: Advanced Techniques
- Exploring Alternatives to Numpy Reshape
- Troubleshooting Numpy Reshape: Common Issues and Solutions
- Python Arrays and Numpy: A Primer
- Numpy Reshape: Beyond Basic Array Manipulation
- Further Learning Resources
- Numpy Reshape: A Comprehensive Recap
Harnessing Numpy Reshape: Basic Use
Numpy reshape is a versatile function in Python’s Numpy library that allows you to change the dimensions of your array without affecting the data it contains. Let’s dive into its basic usage and understand how it works.
Understanding Numpy Reshape
The numpy reshape function takes two arguments: the array you want to reshape and a tuple that defines the new shape. The new shape should be compatible with the number of elements in the original array. Here’s a simple example:
import numpy as np
arr = np.array([1, 2, 3, 4, 5, 6])
reshaped_arr = arr.reshape(2, 3)
print(reshaped_arr)
# Output:
# [[1 2 3]
# [4 5 6]]
In this example, we’ve reshaped a one-dimensional array with six elements into a two-dimensional array with two rows and three columns.
Advantages of Numpy Reshape
Numpy reshape is a powerful tool for data manipulation in Python. It allows you to restructure your data to fit the requirements of various algorithms and functions. Additionally, it’s fast and efficient, making it an excellent choice for large datasets.
Pitfalls and Considerations
While numpy reshape is incredibly useful, it’s important to remember that the new shape must be compatible with the number of elements in the original array. If it’s not, you’ll encounter a ValueError. Always ensure that the product of the dimensions of the new shape equals the number of elements in the original array.
In the next section, we’ll explore more advanced uses of the numpy reshape function.
Numpy Reshape: Advanced Techniques
Now that we’ve covered the basics, let’s delve into the more sophisticated applications of the numpy reshape function. We’ll explore reshaping multidimensional arrays and using the ‘-1’ argument.
Reshaping Multidimensional Arrays
Numpy reshape isn’t limited to one-dimensional arrays. You can reshape multidimensional arrays as well. Let’s reshape a two-dimensional array into a three-dimensional array.
import numpy as np
arr = np.array([[1, 2, 3], [4, 5, 6]])
reshaped_arr = arr.reshape(2, 1, 3)
print(reshaped_arr)
# Output:
# [[[1 2 3]]
# [[4 5 6]]]
In this example, we’ve reshaped a two-dimensional array with two rows and three columns into a three-dimensional array with two blocks, one row in each block, and three columns in each row.
Using the ‘-1’ Argument in Numpy Reshape
The ‘-1’ argument in numpy reshape is a powerful feature that automatically calculates the size of a dimension. This is useful when you know the shape of some dimensions and want numpy to figure out the rest. Let’s see it in action:
import numpy as np
arr = np.array([1, 2, 3, 4, 5, 6])
reshaped_arr = arr.reshape(2, -1)
print(reshaped_arr)
# Output:
# [[1 2 3]
# [4 5 6]]
In this example, we’ve told numpy to reshape the array into two rows and let it calculate the number of columns. Numpy determined that three columns would maintain the same number of elements in the array.
Mastering these advanced techniques will allow you to manipulate arrays in Python more effectively, enhancing your data analysis capabilities.
Exploring Alternatives to Numpy Reshape
While numpy reshape is a powerful tool for manipulating arrays in Python, it’s not the only one. In this section, we’ll introduce other numpy functions that can be used to manipulate arrays, such as resize, ravel, and flatten. We’ll provide code examples and compare these functions with reshape.
Numpy Resize: A Flexible Reshaper
Unlike reshape, the numpy resize function can change the total size of the array. If the new size is greater than the original, the array is filled with repeated copies of itself. Let’s see it in action:
import numpy as np
arr = np.array([1, 2, 3])
resized_arr = np.resize(arr, (2, 3))
print(resized_arr)
# Output:
# [[1 2 3]
# [1 2 3]]
In this example, we’ve resized a one-dimensional array with three elements into a two-dimensional array with two rows and three columns. The original data is repeated to fill the new size.
Numpy Ravel and Flatten: Making Arrays One-Dimensional
Numpy ravel and flatten functions are used to convert multidimensional arrays into one-dimensional arrays. The main difference between them is that flatten always returns a copy of the original array, while ravel returns a view whenever possible, making it more memory efficient.
Here’s an example of ravel:
import numpy as np
arr = np.array([[1, 2, 3], [4, 5, 6]])
raveled_arr = arr.ravel()
print(raveled_arr)
# Output:
# [1 2 3 4 5 6]
In this example, we’ve converted a two-dimensional array into a one-dimensional array using numpy’s ravel function.
Each of these functions has its own use cases and advantages. Understanding when to use reshape, resize, ravel, or flatten can help you manipulate arrays more effectively in Python.
Troubleshooting Numpy Reshape: Common Issues and Solutions
While numpy reshape is a powerful tool, it’s not without its quirks. Let’s discuss some common issues you may encounter when using the function and how to resolve them.
ValueError: Incompatible Shapes
One of the most common errors when using numpy reshape is ValueError due to incompatible shapes. This error occurs when the new shape isn’t compatible with the number of elements in the original array. Here’s an example that produces this error:
import numpy as np
arr = np.array([1, 2, 3, 4, 5, 6])
try:
reshaped_arr = arr.reshape(2, 4)
except ValueError as e:
print(e)
# Output:
# cannot reshape array of size 6 into shape (2,4)
In this example, we tried to reshape a one-dimensional array with six elements into a two-dimensional array with two rows and four columns, which would require eight elements. This mismatch in size caused numpy to raise a ValueError.
Solutions and Workarounds
To avoid this error, ensure that the new shape is compatible with the number of elements in the original array. The product of the dimensions of the new shape should equal the number of elements in the original array.
If you’re unsure about the size of one dimension, you can use the ‘-1’ argument in numpy reshape. Numpy will automatically calculate the size of the dimension for you. Here’s the corrected code:
import numpy as np
arr = np.array([1, 2, 3, 4, 5, 6])
reshaped_arr = arr.reshape(2, -1)
print(reshaped_arr)
# Output:
# [[1 2 3]
# [4 5 6]]
In this example, we’ve told numpy to reshape the array into two rows and let it calculate the number of columns. Numpy determined that three columns would maintain the same number of elements in the array.
Python Arrays and Numpy: A Primer
Before we delve deeper into the numpy reshape function, let’s take a moment to understand the fundamental concepts of arrays in Python and the numpy library.
Understanding Arrays in Python
In Python, an array is a data structure that stores a collection of items. Each item in the array holds a value. The beauty of an array lies in its ability to hold multiple items of the same type, allowing operations to be performed on them efficiently. However, Python doesn’t natively support arrays. Instead, it provides lists that are more general and can hold different types of items.
# Python list example
list_example = [1, 2, 3, 4, 5]
print(list_example)
# Output:
# [1, 2, 3, 4, 5]
In this example, we’ve created a list in Python. The list can hold items of any data type.
Introducing the Numpy Library
Numpy, which stands for ‘Numerical Python’, is a library in Python that supports large, multi-dimensional arrays and matrices. It provides a host of functions to perform mathematical operations on these arrays. Numpy arrays are densely packed arrays of a homogeneous type, which makes them more efficient for storing and manipulating data.
import numpy as np
# Numpy array example
numpy_array_example = np.array([1, 2, 3, 4, 5])
print(numpy_array_example)
# Output:
# [1 2 3 4 5]
In this example, we’ve created a numpy array. Unlike Python lists, numpy arrays can hold items of one data type, making them more efficient for numerical computations.
Understanding these basic concepts is essential for grasping the workings of the numpy reshape function, as we’ll see in the following sections.
Numpy Reshape: Beyond Basic Array Manipulation
The numpy reshape function is more than just a tool for array manipulation in Python. Its applications extend into various fields, including data analysis, machine learning, and more.
Array Reshaping in Data Analysis
In data analysis, reshaping arrays can be crucial. The shape of your data can significantly impact the results of your analysis. For instance, some algorithms require data to be in a specific shape. Numpy reshape allows you to meet these requirements efficiently.
Numpy Reshape and Machine Learning
Machine learning often involves working with large datasets that come in various shapes. Numpy reshape can be used to prepare your data for machine learning algorithms, which often require data to be in a specific format.
Exploring Related Numpy Functions and Concepts
While numpy reshape is a powerful tool, it’s just the tip of the iceberg when it comes to numpy’s capabilities. Other functions like numpy resize, ravel, and flatten also offer unique ways to manipulate arrays. Additionally, concepts like broadcasting and vectorization can help you write more efficient code.
Further Learning Resources
To deepen your understanding of numpy reshape and related concepts, we recommend exploring our article, An In-Depth Look at Numpy Arrays and Functions. It explores the role of NumPy in image processing, signal processing, and numerical simulations.
We have also gathered additional resources that you may find helpful:
- Combining Arrays with numpy.concatenate() – A Comprehensive Tutorial on the “numpy concatenate” function, to help you understand its role in combining arrays.
Data Manipulation with NumPy Transpose() – Master the art of flipping and rotating NumPy arrays for specialized calculations.
A Numpy Guide on the official Python Wiki page for Numpy that provides an overview of its features and functionalities.
Numpy Tutorial YouTube Video – A YouTube tutorial providing visual instruction for understanding and using Numpy.
Numpy Book PDF – A downloadable PDF from MIT offering comprehensive knowledge about Numpy.
Mastering numpy reshape and related functions can significantly enhance your data manipulation capabilities in Python, opening up new possibilities in your coding projects.
Numpy Reshape: A Comprehensive Recap
In this article, we’ve delved deep into the numpy reshape function, a powerful tool for array manipulation in Python. We’ve explored its basic and advanced usage, providing practical code examples along the way.
We’ve also discussed common issues that you might encounter when using numpy reshape, such as ValueError due to incompatible shapes, and provided solutions and workarounds for these issues.
Beyond the numpy reshape function, we’ve introduced alternative functions like resize, ravel, and flatten, that offer unique ways to manipulate arrays in Python. These functions, while having their own specific use cases, can be used in conjunction with numpy reshape to provide a comprehensive set of tools for array manipulation.
In summary, the numpy reshape function is a key tool in Python for anyone working with arrays, particularly in fields like data analysis and machine learning. By mastering numpy reshape and related functions, you can streamline your data manipulation tasks and make your Python code more efficient.