How to Trim Whitespace In Bash Shell Scripts
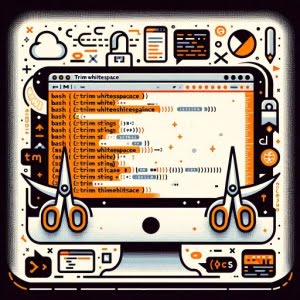
Are you finding it challenging to remove unnecessary spaces in your Bash scripts? You’re not alone. Many developers grapple with this task, but Bash can make this process a breeze.
Like a skilled barber, Bash can trim off the excess whitespace from your strings. These trimmed strings can run more efficiently, making your scripts cleaner and more effective.
This guide will walk you through the process of trimming whitespace in Bash. We’ll explore Bash’s core functionality, delve into its advanced features, and even discuss common issues and their solutions.
So, let’s dive in and start mastering whitespace trimming in Bash!
TL;DR: How Do I Trim Whitespace in Bash?
Trimming whitespace in Bash is a common task that can be accomplished using the
tr
command. For instance, you can usetrimmed_string=$(echo $string | tr -d ' ')
to remove all spaces in a string.
Here’s a simple example:
string=' Hello, World! '
trimmed_string=$(echo $string | tr -d ' ')
echo $trimmed_string
# Output:
# 'Hello,World!'
In this example, we have a string with leading, trailing, and internal spaces. We use the tr
command with the -d
option (which stands for delete) to remove all spaces from the string. The echo
command is used to print the original string, and the output is piped into the tr
command. The result is a new string with all spaces removed.
This is a basic way to trim whitespace in Bash, but there’s much more to learn about string manipulation in Bash. Continue reading for more detailed explanations and advanced usage scenarios.
Table of Contents
- Understanding the tr Command: A Beginner’s Guide
- Advanced Whitespace Trimming in Bash
- Exploring Alternative Whitespace Trimming Methods
- Troubleshooting Whitespace Trimming in Bash
- Bash’s String Manipulation Capabilities
- The Bigger Picture: Why Trimming Whitespace Matters
- Wrapping Up: Mastering Whitespace Trimming in Bash
Understanding the tr
Command: A Beginner’s Guide
The tr
command, short for translate, is a powerful tool in Bash that allows you to transform or delete characters from a string. When it comes to trimming whitespace, the tr
command becomes an invaluable asset.
Let’s take a look at a basic use case:
string=' Bash is awesome! '
trimmed_string=$(echo $string | tr -d ' ')
echo $trimmed_string
# Output:
# 'Bashisawesome!'
In this example, we have a string filled with excessive spaces. We use the tr
command with the -d
option, which stands for delete, to remove all the spaces from the string. The echo
command is used to print the original string, and the output is piped into the tr
command. The result is a new string with all spaces removed.
While this is a great way to remove all spaces in a string, it’s important to note that it removes all spaces, not just leading or trailing ones. This approach may not be suitable if you want to preserve spaces between words.
In the next section, we’ll delve into more advanced techniques to trim different types of whitespace in Bash.
Advanced Whitespace Trimming in Bash
In Bash, whitespace isn’t just about spaces – it also includes tabs and newlines. Trimming these types of whitespace requires a more advanced use of the tr
command.
Trimming Spaces and Tabs
Let’s first look at how to trim spaces and tabs from a string. We’ll use the -d
option with tr
command and include both space and tab characters in quotes.
string=$' Bash is awesome! '
trimmed_string=$(echo $string | tr -d '\t')
echo $trimmed_string
# Output:
# 'Bashisawesome!'
In this example, we use the tr
command to remove both spaces and tabs (\t
) from the string. The $'...'
format is used to allow the interpretation of escape sequences like \t
for tabs.
Trimming Newlines
Now, let’s see how to remove newlines (\n
) from a string:
string=$'Bash
is
awesome!'
trimmed_string=$(echo -n $string | tr -d '\n')
echo $trimmed_string
# Output:
# 'Bashisawesome!'
In this case, we use echo -n
to prevent adding a newline at the end of the output, and the tr
command removes all newline characters from the string.
These examples illustrate how to trim different types of whitespace in Bash. The key is to understand the type of whitespace you’re dealing with and use the tr
command accordingly. In the next section, we’ll explore alternative approaches for trimming whitespace in Bash.
Exploring Alternative Whitespace Trimming Methods
While the tr
command is a powerful tool for trimming whitespace, Bash also offers other commands like sed
and awk
that can perform similar tasks. Let’s explore these alternatives.
Using sed
to Trim Whitespace
sed
is a stream editor for filtering and transforming text. It can be used to trim leading and trailing whitespace from a string. Here’s how:
string=' Bash is awesome! '
trimmed_string=$(echo $string | sed 's/^[ ]*//;s/[ ]*$//')
echo $trimmed_string
# Output:
# 'Bash is awesome!'
In this example, sed
uses a regular expression to match leading (^[ ]*
) and trailing ([ ]*$
) whitespace and replace them with nothing, effectively trimming them.
Using awk
to Trim Whitespace
awk
is a programming language designed for text processing and typically used as a data extraction and reporting tool. It can also be used to trim whitespace:
string=' Bash is awesome! '
trimmed_string=$(echo $string | awk '{$1=$1};1')
echo $trimmed_string
# Output:
# 'Bash is awesome!'
In this case, awk
redefines the string by splitting and concatenating the fields, which removes leading and trailing whitespace.
While sed
and awk
provide more flexibility than tr
, they can be slower and more complex. However, for complex string manipulation tasks, they can be incredibly useful. The key is to choose the tool that best fits your needs.
Troubleshooting Whitespace Trimming in Bash
While trimming whitespace in Bash is straightforward in most cases, there are certain situations that might pose challenges. Let’s explore some common issues and their solutions.
Handling Leading and Trailing Whitespace
One common issue is the need to preserve internal spaces while removing leading and trailing whitespace. The tr
command removes all instances of the specified character, which might not be the desired outcome. In such cases, the sed
command comes in handy.
string=' Bash is awesome! '
trimmed_string=$(echo "$string" | sed -e 's/^[[:space:]]*//' -e 's/[[:space:]]*$//')
echo "$trimmed_string"
# Output:
# 'Bash is awesome!'
In this example, sed
uses two expressions to remove leading and trailing spaces, while preserving the internal spaces between words.
Dealing with Multi-Line Strings
Another challenge arises when dealing with multi-line strings. The tr
command treats the entire input as a single line, which can lead to unexpected results. Here, the awk
command can be a better fit.
string=$' Bash
is
awesome! '
trimmed_string=$(echo "$string" | awk '{$1=$1};1')
echo "$trimmed_string"
# Output:
# 'Bash'
# 'is'
# 'awesome!'
In this example, awk
effectively trims leading and trailing whitespace from each line, preserving the multi-line structure of the string.
These examples illustrate how to handle common issues in whitespace trimming. By understanding these challenges and their solutions, you can more effectively manipulate strings in Bash.
Bash’s String Manipulation Capabilities
Bash, as a command language, excels in manipulating strings. It offers a variety of built-in commands and features that make string operations efficient and straightforward. Understanding these capabilities is fundamental to mastering whitespace trimming.
The Power of tr
The tr
command, short for translate, is a Unix utility that translates or deletes characters from standard input, writing to standard output. It’s a simple, yet powerful tool for single-character transformations.
string='HELLO'
lowercase_string=$(echo $string | tr 'A-Z' 'a-z')
echo $lowercase_string
# Output:
# 'hello'
In this example, we use tr
to convert a string to lowercase. This demonstrates how tr
can manipulate characters in a string.
The Flexibility of sed
sed
, short for stream editor, is a powerful and flexible tool that manipulates text in a stream or file. It’s commonly used for text substitutions and deletions.
string='Hello, World!'
replaced_string=$(echo $string | sed 's/World/Planet/')
echo $replaced_string
# Output:
# 'Hello, Planet!'
In this example, we use sed
to replace ‘World’ with ‘Planet’ in a string, demonstrating its ability to perform complex text transformations.
The Utility of awk
awk
is a versatile programming language designed for text processing. It’s particularly useful for data extraction and reporting.
string='Hello, World!'
word_count=$(echo $string | awk '{print NF}')
echo $word_count
# Output:
# '2'
In this example, we use awk
to count the number of words in a string, showcasing its utility in text processing tasks.
Understanding these commands and how they manipulate strings is crucial to mastering whitespace trimming in Bash. In the next section, we’ll discuss the relevance of whitespace trimming in script writing and data processing.
The Bigger Picture: Why Trimming Whitespace Matters
Trimming whitespace in Bash isn’t just about aesthetics or preference. It has practical implications in script writing and data processing.
Excessive or inconsistent whitespace can interrupt the flow of data, cause errors in scripts, or lead to incorrect results in data processing. By mastering whitespace trimming, you can ensure that your scripts and data are clean, efficient, and error-free.
Exploring Related Concepts
While trimming whitespace is a valuable skill, it’s just one aspect of string manipulation in Bash. To truly master Bash scripting, you might want to explore related concepts like regular expressions and file handling in Bash.
Regular expressions allow you to match and manipulate strings based on patterns, making them a powerful tool for complex string operations. File handling, on the other hand, involves reading from and writing to files, which is a common requirement in many Bash scripts.
Further Resources for Mastering Bash
To deepen your understanding of Bash and its capabilities, consider exploring these resources:
- GNU Bash Manual: The official manual for Bash, providing comprehensive documentation on its features and usage.
Bash Guide for Beginners: A beginner-friendly guide to Bash scripting, covering basic to intermediate concepts.
Advanced Bash-Scripting Guide: A detailed guide on advanced Bash scripting concepts, suitable for more experienced users.
Remember, mastering Bash takes time and practice. Don’t be afraid to experiment, make mistakes, and learn from them. Happy scripting!
Wrapping Up: Mastering Whitespace Trimming in Bash
In this comprehensive guide, we’ve explored the ins and outs of trimming whitespace in Bash. We’ve covered the various commands and techniques you can utilize to manipulate strings effectively and efficiently in Bash.
We began with the basics, examining the tr
command and its ability to delete specific characters, such as spaces, from a string. We then delved deeper into the nuances of whitespace, discussing how to handle different types of whitespace including spaces, tabs, and newlines.
As we advanced, we explored alternative methods for trimming whitespace, introducing the sed
and awk
commands as powerful tools for complex string manipulations. We also tackled common challenges that you might encounter when trimming whitespace and provided practical solutions for each.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
tr | Simple, fast | Only handles single characters |
sed | Handles regular expressions | Slower, more complex |
awk | Handles multi-line strings | Slower, more complex |
Whether you’re just starting out with Bash or looking to refine your scripting skills, we hope this guide has given you a deeper understanding of how to trim whitespace in Bash. Mastering these techniques will not only make your scripts cleaner and more efficient, but also open up new possibilities for data processing and manipulation.
Remember, Bash is a powerful tool for text processing and automation. With this guide, you’re well on your way to becoming a Bash scripting expert. Happy scripting!