Python Array Usage Guide with Examples
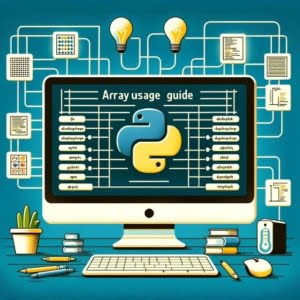
Arrays are a cornerstone of Python programming, playing a pivotal role in data management, analysis, and machine learning. Whether you’re a Python novice or a seasoned coder seeking to dust off your skills, mastering arrays is a non-negotiable.
Our mission in this comprehensive guide is to help you conquer the use of arrays in Python. We’ll plunge into the creation and manipulation of arrays, investigate their practical applications, and even skim over some advanced techniques.
By the end of this guide, you’ll not only understand arrays in Python but also possess the skills to wield them effectively in your programming endeavours. So, buckle up and let’s embark on this exciting journey into the realm of Python arrays!
TL;DR: What are arrays in Python?
Arrays in Python are a data structure that holds an ordered collection of items, allowing you to store a sequence of items in a single variable. They are particularly useful in handling large datasets and performing mathematical operations on data. For a more in-depth exploration of arrays, including their creation, manipulation, and practical uses, continue reading this article.
import array as arr
a = arr.array('d', [1.1, 3.5, 4.5])
print(a) # Output: array('d', [1.1, 3.5, 4.5])
Table of Contents
Unraveling the Mystery of Python Arrays
Before we plunge into the intricacies of using arrays, it’s crucial to first comprehend what an array is. In the realm of Python, an array is a data structure that houses an ordered collection of items.
This means you can store a sequence of items in a single array. The items are stored consecutively in memory, akin to a train where each car (element) is connected and in order, and you can access any car directly if you know its position (index).
One of the key advantages of arrays is their efficiency. When dealing with large data sets, arrays are far more efficient than other data structures, like lists. This is because arrays are stored in a contiguous block of memory, so accessing elements is quicker and more efficient.
Crafting Arrays in Python
Constructing arrays in Python is a breeze. You can create an array using the array
module. Let’s look at a simple example:
import array as arr
a = arr.array('d', [1.1, 3.5, 4.5])
print(a)
In this example, d
is a type code. This type code is the blueprint that determines the type of the array during creation.
Navigating Array Elements
Navigating elements in a Python array is straightforward. You can access an individual item in an array using its index. Python arrays are zero-indexed, meaning the first element sits at index 0. Here’s how you can navigate the elements within an array:
import array as arr
a = arr.array('d', [1.1, 3.5, 4.5])
print(a[0])
print(a[2])
Mastering the Art of Python Array Manipulation
Having gained a basic grasp of arrays and their creation, let’s dive into the deep end and explore how we can manipulate arrays in Python. Manipulation of arrays encompasses updating and deleting elements, slicing and iterating through arrays, and sorting and searching arrays.
Updating and Deleting Elements
Updating an element in a Python array is as simple as pointing to the index of the item you want to update. Let’s take a look at an example:
import array as arr
a = arr.array('d', [1.1, 3.5, 4.5])
a[0] = 2.1
print(a)
Deleting an element is equally straightforward. You can employ the del
statement to eradicate the element at a specific index:
import array as arr
a = arr.array('d', [1.1, 3.5, 4.5])
del a[1]
print(a)
Slicing and Iterating Through Arrays: A Piece of Cake
Slicing is your ticket to accessing a subset of an array’s elements. You can slice an array in Python in a similar fashion to how you would slice a list. Here’s a quick example:
import array as arr
a = arr.array('d', [1.1, 3.5, 4.5, 2.6, 7.8])
print(a[1:4])
Iterating through an array can be accomplished using a simple for loop:
import array as arr
a = arr.array('d', [1.1, 3.5, 4.5, 2.6, 7.8])
for i in a:
print(i)
Sorting and Searching Arrays: Easier Than You Think
Python equips you with built-in methods for sorting and searching arrays. You can utilize the sorted
function to arrange an array:
import array as arr
a = arr.array('d', [1.1, 3.5, 4.5, 2.6, 7.8])
b = arr.array(a.typecode, sorted(a))
print(b)
And you can use the index
method to hunt for the first occurrence of a value in an array:
import array as arr
a = arr.array('d', [1.1, 3.5, 4.5, 2.6, 7.8])
print(a.index(4.5))
Advanced Array Techniques in Python
Let’s dive deeper and unlock some advanced techniques for manipulating arrays in Python. Harnessing these techniques can significantly amplify your Python programming skills and pave the way for new avenues in data analysis and manipulation.
Python offers several advanced techniques that you can employ to manipulate arrays. For instance, list comprehension, a compact way of creating and manipulating arrays in a single line of code.
Here’s an example where we use list comprehension to create an array of the first ten even numbers:
import array as arr
a = arr.array('d', [i * 2 for i in range(10)])
print(a)
Array Modules and Functions: Your Secret Toolkit
Python arms you with several modules and functions that you can use to work with arrays. For instance, the array
module provides the array()
function for creating arrays, and several other functions for manipulating arrays, such as append()
, insert()
, pop()
, and remove()
.
append()
append()
is a function that adds a single element to the end of the array.
import array as arr
a = arr.array('i', [1, 2, 3])
a.append(4) # appending 4 to the array
print(a)
Output:
array('i', [1, 2, 3, 4])
insert()
insert()
is a function that adds a single element to the array at the specified position.
import array as arr
a = arr.array('i', [1, 2, 3])
a.insert(1, 4) # inserting 4 at position 1
print(a)
Output:
array('i', [1, 4, 2, 3])
pop()
pop()
is a function that removes an element from the array, and returns it. The default is to remove the last element, but you can also specify the index of the element to remove.
import array as arr
a = arr.array('i', [1, 2, 3])
a.pop() # without index, it will remove last element
print(a)
a.pop(0) # removing element at position 0
print(a)
Output:
array('i', [1, 2])
array('i', [2])
remove()
remove()
is a function that removes the first occurrence of a specified element from the array.
import array as arr
a = arr.array('i', [1, 2, 3, 2])
a.remove(2) # removing first occurrence of 2
print(a)
Output:
array('i', [1, 3, 2])
Please note that the remove()
function throws a ValueError if the specified value is not found.
Handling Multi-Dimensional Arrays: The Next Level
So far, we’ve only explored one-dimensional arrays. However, Python also supports multi-dimensional arrays, which are arrays that contain other arrays. These are particularly useful in fields like data analysis and machine learning, where you often need to grapple with multi-dimensional data.
You can create a multi-dimensional array in Python using a nested list comprehension. Here’s an example of a two-dimensional array:
import array as arr
a = arr.array('d', [i * j for i in range(5) for j in range(5)])
print(a)
This creates a two-dimensional array where each element is the product of its two indices.
Arrays play a pivotal role in advanced Python programming. They provide an efficient and versatile data structure for storing and manipulating data, and they are a fundamental part of many advanced programming techniques, such as data analysis and machine learning.
Mastering advanced array techniques can significantly elevate your Python programming skills. Whether you’re a Python novice looking to broaden your knowledge, or an experienced programmer aiming to take your skills to the next level, understanding how to work with arrays is a crucial step in your journey to become a proficient Python programmer.
Practical Uses of Python Arrays
Arrays in Python are not just versatile, they’re like a Swiss Army knife, ready to be used in a plethora of applications. Let’s walk through some practical examples that spotlight how arrays can be leveraged in real-world Python programming.
Arrays and Mathematical Operations: A Match Made in Heaven
Arrays shine especially bright when used in mathematical operations. For instance, you can effortlessly perform operations on each element of an array without having to loop through each element individually.
Here’s an example where we multiply each element of an array by 2:
import array as arr
a = arr.array('d', [1.1, 3.5, 4.5, 2.6, 7.8])
b = arr.array('d', [i * 2 for i in a])
print(b)
Arrays in Data Analysis: The Secret Weapon
Arrays also play a pivotal role in data analysis. They provide an efficient way to store and manipulate large volumes of data. For instance, you can use arrays to compute the mean of a large dataset, as shown in this example:
import array as arr
a = arr.array('d', [1.1, 3.5, 4.5, 2.6, 7.8])
mean = sum(a) / len(a)
print(mean)
Arrays in Machine Learning and AI: The Power Players
In the realms of machine learning and AI, arrays are used to store and manipulate vast datasets. They are often used in tandem with libraries like NumPy and Pandas, which provide additional functionality for working with arrays.
Here’s an example of how this can be done:
# Required Libraries
import numpy as np
import pandas as pd
# Creating a numpy array of random numbers
numpy_array = np.random.rand(3,4)
print("Numpy Array:\n", numpy_array)
# Adding an extra dimension to an numpy array
print("\nAdding extra dimension to numpy array:\n", numpy_array[:, np.newaxis])
# Create DataFrame from Numpy Array
pandas_df = pd.DataFrame(numpy_array, columns=['A','B','C','D'])
print("\nPandas DataFrame:\n", pandas_df)
# Access a column from DataFrame
print("\nAccess single column from Pandas DataFrame:\n", pandas_df['A'])
# Slicing a DataFrame
print("\nSlicing the DataFrame:\n", pandas_df.iloc[0:2, 1:3])
Output:
Numpy Array:
[[0.59284679 0.99214359 0.70142422 0.8743428 ]
[0.55222637 0.32278367 0.63796683 0.91984956]
[0.92876088 0.29736746 0.54170962 0.63246541]]
Adding extra dimension to numpy array:
[[[0.59284679 0.99214359 0.70142422 0.8743428 ]]
[[0.55222637 0.32278367 0.63796683 0.91984956]]
[[0.92876088 0.29736746 0.54170962 0.63246541]]]
Pandas DataFrame:
A B C D
0 0.592847 0.992144 0.701424 0.874343
1 0.552226 0.322784 0.637967 0.919850
2 0.928761 0.297367 0.541710 0.632465
Access single column from Pandas DataFrame:
0 0.592847
1 0.552226
2 0.928761
Name: A, dtype: float64
Slicing the DataFrame:
B C
0 0.992144 0.701424
1 0.322784 0.637967
This python example does the following major operations :
- Generating a 2D numpy array of random numbers
- Adding an extra dimension to numpy array
- Creating a Pandas DataFrame from the numpy array.
- Accessing a single column from the pandas dataframe.
- Slicing certain columns and rows from the pandas dataframe.
Lists in Python: The Jack of All Trades
While arrays are a formidable tool in Python, they are not the only data structure in the game. Python also boasts lists, which are incredibly versatile and can accommodate different types of data. But how do arrays and lists square off, and when should you favor one over the other?
In Python, a list is a built-in data structure capable of holding a collection of items. These items can be of any type, and a single list can even hold items of different types. Here’s a quick snapshot of a list in Python:
list_example = [1, 'two', 3.0, 'four']
print(list_example)
Arrays vs. Lists: The Key Differences
The main divergences between arrays and lists in Python lie in their functionality and usage. This table breaks down the key differences, and then we’ll go over each one:
Arrays | Lists | |
---|---|---|
Data Type | Homogeneous i.e., can hold data of a single type | Heterogeneous i.e., can hold different types of data |
Memory Efficiency | More efficient in handling large data volume due to contiguous memory storage | Less efficient for large data volume |
Operations | Mathematical operations can be performed easily | Cannot perform mathematical operations directly |
Usage | Usually used when performing mathematical operations on data | Used for general-purpose data storage |
Flexibility | Less flexible as it can store single type of data | More flexible as it can store multiple types of data |
Arrays come into play when you need to perform mathematical operations on a homogeneous set of data, while lists come to the rescue when you need a general-purpose data structure that can hold different types of data.
Another critical difference lies in how they manage memory. Arrays outperform lists when dealing with large volumes of homogeneous data. This is because arrays are stored in a contiguous block of memory, facilitating faster access and manipulation of data.
The choice between an array or a list largely depends on the task at hand. If you’re wrestling with large volumes of data that are all of the same type, and you need to perform mathematical operations on that data, an array is likely your best bet due to its efficiency.
On the flip side, if you need a data structure to hold a collection of different types of data, a list would be more fitting. Lists are more flexible than arrays and are a good choice for general-purpose programming.
Efficiency Showdown: Arrays vs. Lists in Python
As touched upon earlier, arrays are more efficient than lists when dealing with large volumes of homogeneous data. This is owing to the way arrays are stored in memory, which enables faster access and manipulation of data.
However, for smaller volumes of data, or for collections of heterogeneous data, the difference in efficiency between arrays and lists is negligible.
Property | Array | List |
---|---|---|
Data Types | Homogeneous (same type) | Heterogeneous (any type) |
Memory | Stored in a contiguous block of memory | Not stored in a contiguous block of memory |
Use Case | Mathematical operations, large volumes of data | General-purpose, collections of different types of data |
Efficiency | More efficient with large volumes of data | Less efficient with large volumes of data |
Choosing the right data structure is a pivotal decision in Python programming. Gaining a clear understanding of the differences between arrays and lists, and knowing when to employ each, can help you write more efficient and effective code.
Further Resources for Python Arrays
To enhance your expertise in Python arrays, refer to the following resources that could be immensely beneficial:
- Python Data Types Explained: Core Concepts explores the versatility of sequence data types for various data tasks.
Adding Elements to a Set in Python – Explore how to add elements to sets in Python for efficient data management.
Exploring Dataclasses in Python – Master Python dataclass features and understand their advantages.
W3Schools’ Tutorial on Creating Numpy Arrays teaches how to create Numpy arrays in Python.
Real Python’s Guide on Python Array covers array handling in Python.
W3Schools’ Python List Reference is a straightforward reference for Python’s list functions.
By incorporating a deep understanding of Python arrays into your development toolbox and delving into relevant topics, you can ascend to new heights as a proficient Python programmer.
Final Takeaways
In this comprehensive guide, we’ve journeyed through the fascinating world of Python arrays. We’ve demystified what arrays are, learned how to create and manipulate them, and discovered their myriad practical uses. We also took a leap into some advanced techniques that can take your array manipulation skills to new heights.
But our exploration didn’t stop at the basics. We plunged into the deep end with advanced array techniques, from leveraging array modules and functions to handling multi-dimensional arrays. Mastering these techniques can significantly enhance your Python programming skills, opening up new possibilities for data analysis and manipulation.
Whether you’re a Python novice or an experienced programmer, the benefits of understanding and mastering arrays are everywhere. So keep practicing, keep exploring, and soon you’ll find yourself adept at Python arrays, ready to tackle any programming challenge that comes your way!