Python Get Current Time: Guide (With Examples)
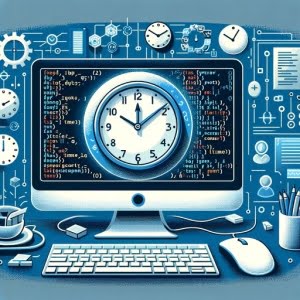
Ever wondered how to get the current time in Python? Time is an essential part of many coding projects, whether you’re logging events, scheduling tasks or simply displaying the current time on a user interface.
In this comprehensive guide, we’ll walk you through how to fetch and format the current time in Python. We’ll start with the basics and gradually delve into more advanced techniques. So, let’s dive in and explore how to get the current time in Python, down to the millisecond!
TL;DR: How Do I Get the Current Time in Python?
You can get the current time in Python by using the datetime module. Here’s a quick example:
from datetime import datetime
now = datetime.now()
current_time = now.strftime('%H:%M:%S')
print('Current Time:', current_time)
# Output:
# 'Current Time: 14:30:15'
In this example, we’re importing the datetime module and using its now
function to get the current time. We then format this time into hours, minutes, and seconds (HH:MM:SS) using the strftime
function. The result is printed out, showing the current time when the script is run.
Interested in learning more? Keep reading for a more detailed explanation and advanced usage scenarios!
Table of Contents
Python’s Datetime Module
Python’s built-in datetime
module is a powerful tool for working with dates and times. At its most basic, it can provide you with the current date and time, which is our focus in this section. Let’s explore this with a simple code example:
from datetime import datetime
current_time = datetime.now()
print(current_time)
# Output:
# 2022-03-01 14:30:15.123456
Here, we’re importing the datetime
class from the datetime
module. We then call the now()
function, which returns the current date and time down to the microsecond. The result is then printed out.
This is a simple yet powerful way to get the current time in Python.
It’s worth noting that the output is in the format YYYY-MM-DD HH:MM:SS.ssssss, which might not be ideal for all use cases.
In the next section, we’ll look at how to format this output to better suit your needs.
Tailoring Time with strftime and Microsecond Precision
As we’ve seen, the datetime.now()
function provides us with the current date and time down to the microsecond. But what if we want to format this output to better suit our needs? This is where strftime
, a method of the datetime
class, comes in handy.
Formatting Time with strftime
strftime
allows us to format date and time outputs in any way we want. Let’s see it in action:
from datetime import datetime
current_time = datetime.now()
formatted_time = current_time.strftime('%H:%M:%S')
print('Current Time:', formatted_time)
# Output:
# 'Current Time: 14:30:15'
In this example, we’re using strftime
with the format code %H:%M:%S
, which corresponds to hours, minutes, and seconds. This gives us the current time in the format we want.
Microsecond Precision
Python’s datetime
module can get the current time down to the microsecond. Here’s how you can do it:
from datetime import datetime
current_time = datetime.now()
microsecond_time = current_time.strftime('%H:%M:%S.%f')
print('Current Time:', microsecond_time)
# Output:
# 'Current Time: 14:30:15.123456'
In this example, we’ve added . %f
to our format string in strftime
. This fetches the current time down to the microsecond.
By mastering strftime
and understanding its format codes, you can tailor Python’s current time output to your exact needs.
Exploring Alternatives: The Time Module
While the datetime
module is incredibly versatile, Python offers other ways to fetch the current time. One such alternative is the time
module.
Time Module in Action
The time
module’s time
function returns the current time in seconds since the Epoch (January 1, 1970). Here’s how you can use it:
import time
current_time_seconds = time.time()
print('Current Time:', current_time_seconds)
# Output:
# 'Current Time: 1646149815.123456'
This output is a floating-point number representing the current time in seconds since the Epoch. While this might not seem as readable as our previous examples, it’s a commonly used time format in many computing systems and languages.
Converting Time Since the Epoch to Readable Format
You can convert the time since the Epoch to a more readable format using the time
module’s ctime
function:
import time
current_time_seconds = time.time()
readable_time = time.ctime(current_time_seconds)
print('Current Time:', readable_time)
# Output:
# 'Current Time: Tue Mar 1 14:30:15 2022'
Here, we’re passing the output of time.time()
to time.ctime()
, which converts it to a string representing local time.
Advantages and Disadvantages
The time
module’s approach to fetching the current time is simple and straightforward. However, it might not be as flexible as datetime
when it comes to formatting the output. That said, it’s a handy tool to have in your Python time mastery toolkit, especially when dealing with systems or languages that use time since the Epoch.
Method | Use Case | Flexibility | Precision |
---|---|---|---|
datetime.now() | Fetching the current date and time | High | Microsecond |
time.time() | Getting the time since the Epoch | Low | Second |
In the end, the method you choose to fetch the current time in Python depends on your specific needs and the requirements of your project. Both datetime
and time
have their strengths and can be used effectively in different scenarios.
While Python makes it relatively straightforward to fetch the current time, you might encounter some challenges when dealing with time zones and daylight saving time. Here, we’ll discuss these issues and provide solutions and workarounds.
Time Zones: A Common Hurdle
Python’s datetime.now()
function returns the current time in the system’s local time zone. But what if you need the current time in a different time zone? The pytz
library comes to the rescue.
from datetime import datetime
import pytz
new_york_tz = pytz.timezone('America/New_York')
current_time = datetime.now(new_york_tz)
print('Current Time in New York:', current_time)
# Output:
# 'Current Time in New York: 2022-03-01 10:30:15.123456-05:00'
In this example, we’re using the pytz
library to get the timezone for New York. We then pass this timezone to datetime.now()
, which returns the current time in that timezone.
Dealing with Daylight Saving Time
Daylight saving time can also pose a challenge when working with time in Python. The pytz
library can help here too, as it takes daylight saving time into account when calculating time.
from datetime import datetime
import pytz
paris_tz = pytz.timezone('Europe/Paris')
current_time = datetime.now(paris_tz)
print('Current Time in Paris:', current_time)
# Output (depends on the date):
# 'Current Time in Paris: 2022-03-01 20:30:15.123456+01:00'
# or
# 'Current Time in Paris: 2022-07-01 20:30:15.123456+02:00'
In this example, the output will be different depending on the date, as Paris observes daylight saving time. From the last Sunday in March to the last Sunday in October, the time will be UTC+2. For the rest of the year, it will be UTC+1.
By understanding these considerations and knowing how to navigate them, you can ensure that your Python scripts always fetch the correct current time, no matter where and when.
Understanding Python’s Time and Date Handling
Before we can fully grasp how to fetch the current time in Python, it’s crucial to understand how Python handles time and dates. Python’s approach to time and date management is both powerful and flexible, allowing us to perform a wide range of operations.
Python’s Datetime Module: A Closer Look
At the heart of Python’s time and date handling is the datetime
module. This module provides classes for manipulating dates and times in both simple and complex ways.
from datetime import datetime
# Get the current date and time
current_datetime = datetime.now()
# Get the current date
current_date = current_datetime.date()
# Get the current time
current_time = current_datetime.time()
print('Current Date and Time:', current_datetime)
print('Current Date:', current_date)
print('Current Time:', current_time)
# Output:
# Current Date and Time: 2022-03-01 14:30:15.123456
# Current Date: 2022-03-01
# Current Time: 14:30:15.123456
In this example, we’re using the now()
function of the datetime
class to get the current date and time. We’re then using the date()
and time()
methods to extract the date and time, respectively.
Why Understanding Time and Date Handling Matters
Understanding how Python handles time and dates is crucial when working with time in your scripts. Whether you’re logging events, scheduling tasks, or simply displaying the current time, you’ll need to fetch, format, and sometimes manipulate time and dates. By mastering Python’s datetime
module and related functions, you can ensure that your scripts handle time accurately and efficiently.
Broad Applications of Python Time Fetching
Getting the current time in Python isn’t just a standalone operation. It plays a crucial role in larger scripts and projects, serving as a foundational skill in your Python programming journey.
Logging Events
In a logging system, each log entry typically includes a timestamp. This timestamp helps developers understand the sequence of events and troubleshoot issues. Here’s a simple example of how you might use Python’s datetime
module in a logging scenario:
from datetime import datetime
# An event to log
event = 'User logged in'
# Get the current time
current_time = datetime.now().strftime('%Y-%m-%d %H:%M:%S')
# Log the event
print(f'{current_time} - {event}')
# Output:
# '2022-03-01 14:30:15 - User logged in'
In this example, we’re fetching the current time and including it in a log entry. This provides a timestamp for the event ‘User logged in’.
Scheduling Tasks
Python’s time functions can also be used to schedule tasks. For instance, you might want a script to run at a specific time each day. By fetching the current time and comparing it to the desired time, you can determine when to run your script.
More Resources
To deepen your understanding of how Python handles time, consider exploring the following resources:
- IOFlood’s Article on Python Time explores Python’s time module for managing time-related tasks with ease and accuracy.
Code Benchmarking with Timeit in Python – Master timeit usage in Python for profiling code and optimizing performance.
Adding Delays to Python Programs with Wait – Master Python’s wait mechanisms for controlling script execution and timing.
Official Python Documentation for the datetime Module – Dive into Python’s built-in module for manipulating dates and times.
Official Python Documentation for the time Module – Learn about Python’s time module, used for handling time-related tasks.
Pytz Library Documentation – Explore Pytz, a Python library that enables precise and cross-platform timezone calculations.
By understanding how to fetch the current time in Python and apply it to real-world scenarios, you’ll be better equipped to tackle more complex Python projects.
Wrapping Up: Python Time Mastery
We’ve journeyed through the process of fetching the current time in Python, starting from the basics and venturing into more advanced techniques. Here’s a quick recap:
- We learned how to use Python’s
datetime
module to fetch the current date and time, as well as how to format the output usingstrftime
. - We explored the precision of Python’s time fetching, learning how to get the current time down to the microsecond.
- We examined alternative methods for fetching the current time, such as using the
time
module. - We navigated potential pitfalls, including dealing with time zones and daylight saving time.
- We delved into the fundamentals of how Python handles time and dates, and why understanding these concepts is crucial.
- Finally, we discussed broader applications of fetching the current time in Python, including its role in logging events and scheduling tasks.
Remember, the method you choose to fetch the current time in Python depends on your specific needs and the requirements of your project. Whether you’re using datetime
, time
, or another approach, Python provides the tools you need to handle time effectively and accurately.