Python Wait: Pausing with time.sleep() and more
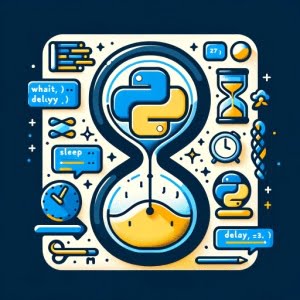
Ever found yourself in a situation where you needed your Python program to take a breather, or wait for a specific duration? You’re not alone.
Many developers find themselves in need of this feature, but Python, like a well-timed comedian, has the ability to pause its execution – using the wait function.
In this guide, we’ll walk you through the process of using the Python wait function, from the basics to more advanced techniques. We’ll cover everything from the time.sleep()
function, to more complex uses such as using it in loops or with threading, and even alternative approaches.
Let’s get started!
TL;DR: How Do I Make Python Wait or Pause in My Code?
To pause or delay execution in Python, you can use the
sleep()
function from Python’stime
module:time.sleep(5)
. This function makes Python wait for a specified number of seconds.
Here’s a simple example:
import time
time.sleep(5) # Makes Python wait for 5 seconds
# Output:
# (After a 5 second delay, the program continues...)
In this example, we import the time
module and use the sleep()
function to make Python pause for 5 seconds. The argument to sleep()
is the number of seconds that Python should wait.
This is a basic way to make Python wait, but there’s much more to learn about controlling the flow of your Python programs. Continue reading for a more detailed understanding and advanced usage scenarios.
Table of Contents
- Python Wait 101: The time.sleep() Function
- Python Wait in Loops and Threading
- Exploring Asynchronous Waiting in Python
- Troubleshooting Python Wait: Common Issues and Solutions
- Understanding Python’s time Module
- The Concept of Pausing Execution
- Real-World Applications of Python Wait Function
- Exploring Related Concepts
- Wrapping Up: Mastering the Python Wait Function
Python Wait 101: The time.sleep() Function
At the heart of making Python wait is the time.sleep()
function. This function is part of Python’s time
module and is your go-to tool for pausing the execution of your Python scripts.
The time.sleep()
function takes one argument: the number of seconds that you want Python to wait. This argument can be an integer or a float, which means you can make Python wait for a precise amount of time, right down to the millisecond.
Here’s a simple example:
import time
time.sleep(3.5) # Makes Python wait for 3.5 seconds
print('Hello, World!')
# Output:
# (After a 3.5 second delay, the program prints...)
# 'Hello, World!'
In this example, we first import the time
module. Then, we use the time.sleep()
function to make Python wait for 3.5 seconds. After the wait, Python prints ‘Hello, World!’.
The time.sleep()
function is straightforward and easy to use, which makes it perfect for beginners. However, it’s important to note that while Python is sleeping, it’s not doing anything else. This means that if you have a multi-threaded program and one thread goes to sleep, the other threads continue executing. But if your Python program is single-threaded, it will be completely unresponsive while it’s sleeping.
This is a key point to keep in mind when using time.sleep()
. While it’s a powerful tool for controlling the flow of your program, it should be used wisely to avoid creating programs that are unresponsive or slow.
Python Wait in Loops and Threading
As we delve deeper into the Python wait function, we uncover its potential in more complex scenarios. Two such scenarios involve using the wait function in loops and alongside threading.
Python Wait in Loops
In some cases, you might want your Python program to wait for a certain duration in each iteration of a loop. This can be achieved by placing the time.sleep()
function inside the loop. Here’s an example:
import time
for i in range(5):
print(i)
time.sleep(2) # Makes Python wait for 2 seconds in each iteration
# Output:
# 0
# (After a 2 second delay, the program prints...)
# 1
# (After a 2 second delay, the program prints...)
# 2
# (After a 2 second delay, the program prints...)
# 3
# (After a 2 second delay, the program prints...)
# 4
In this example, we have a loop that iterates five times. In each iteration, it prints the current iteration number and then waits for 2 seconds before proceeding to the next iteration. This way, we manage to introduce a delay in each iteration.
Python Wait with Threading
In a multi-threaded program, you might want one thread to wait while the other threads continue their execution. This can be achieved using the time.sleep()
function in the relevant thread. Here’s an example:
import time
import threading
def print_nums():
for i in range(5):
print(i)
time.sleep(1)
def print_letters():
for letter in 'abcde':
print(letter)
time.sleep(1)
threading.Thread(target=print_nums).start()
threading.Thread(target=print_letters).start()
# Output:
# 0
# a
# (After a 1 second delay, the program prints...)
# 1
# b
# (After a 1 second delay, the program prints...)
# 2
# c
# (After a 1 second delay, the program prints...)
# 3
# d
# (After a 1 second delay, the program prints...)
# 4
# e
In this example, we have two threads that run concurrently. Each thread prints and then waits for 1 second before printing again. Notice how the output alternates between numbers and letters, showing that the threads are running concurrently and each waiting for its own time.sleep()
to finish.
These are just two of the many ways you can use the Python wait function in more complex scenarios. By understanding and mastering these techniques, you can create more efficient and effective Python programs.
Exploring Asynchronous Waiting in Python
While time.sleep()
is a powerful tool for controlling the flow of your Python programs, there are other techniques to pause execution in Python, especially when dealing with asynchronous programming. One such alternative is asyncio.sleep()
, a function provided by Python’s asyncio
module.
The asyncio.sleep() Function
The asyncio.sleep()
function is an asynchronous version of time.sleep()
. Instead of blocking the execution of your program, asyncio.sleep()
allows other tasks to run during the wait time. This function is especially useful in asynchronous programs where you want to avoid blocking the entire program when one task is waiting.
Here’s an example of how to use asyncio.sleep()
:
import asyncio
async def main():
print('Hello,')
await asyncio.sleep(1)
print('World!')
asyncio.run(main())
# Output:
# 'Hello,'
# (After a 1 second delay, the program prints...)
# 'World!'
In this example, we define an asynchronous function main()
that prints ‘Hello,’, waits for 1 second using asyncio.sleep()
, and then prints ‘World!’. Despite the wait, the asyncio.sleep()
function doesn’t block the entire program, and other tasks (if any) could run during the wait time.
Comparing time.sleep() and asyncio.sleep()
While both time.sleep()
and asyncio.sleep()
can be used to pause execution in Python, they are used in different contexts and have different impacts on your program:
time.sleep()
: This function is simple and easy to use, making it perfect for beginners and simple programs. However, it blocks the execution of your program during the wait time, which can be a downside if you need your program to remain responsive.asyncio.sleep()
: This function is more complex and requires understanding of asynchronous programming. It doesn’t block your program during the wait time, allowing other tasks to run, which can be a big advantage in more complex, asynchronous programs.
Choosing between time.sleep()
and asyncio.sleep()
depends on your specific needs and the complexity of your program. If you’re just starting out or working on a simple, single-threaded program, time.sleep()
is likely the best choice. But if you’re working on a more complex, asynchronous program and need to maintain responsiveness during wait times, asyncio.sleep()
might be the better option.
Troubleshooting Python Wait: Common Issues and Solutions
While the Python wait function is a powerful tool, it’s not without its quirks. Here, we’ll discuss some common issues you might encounter when using time.sleep()
or asyncio.sleep()
, and provide solutions and workarounds.
Interruptions During Sleep
One common issue is the interruption of time.sleep()
. If a signal is received during the sleep, the function may wake up earlier than expected. Here’s an example:
import time
import signal
def signal_handler(signum, frame):
print('Signal received!')
# Set the signal handler
signal.signal(signal.SIGINT, signal_handler)
print('Going to sleep...')
try:
time.sleep(5)
except Exception as e:
print(f'Woke up due to: {e}')
print('Awake!')
# Output:
# 'Going to sleep...'
# (If an interrupt signal (like Ctrl+C) is sent during the sleep...)
# 'Signal received!'
# 'Woke up due to: interrupted sleep'
# 'Awake!'
In this example, if an interrupt signal (like Ctrl+C) is sent during the sleep, the time.sleep()
function is interrupted, and the program immediately proceeds to the next line of code.
Blocking Issues with time.sleep()
As mentioned earlier, time.sleep()
blocks the execution of your program. This means that if you have a single-threaded program, it will be completely unresponsive during the sleep. If you need your program to remain responsive, consider using asyncio.sleep()
instead, or restructuring your program to use multiple threads.
Asynchronous Waiting with asyncio.sleep()
While asyncio.sleep()
doesn’t block your program, it requires a more complex setup and an understanding of asynchronous programming. Make sure to thoroughly test your program to ensure that all tasks are running as expected during the wait time.
In conclusion, while the Python wait function is a powerful tool, it’s important to understand its quirks and potential issues. By being aware of these, you can write more robust and efficient Python programs.
Understanding Python’s time Module
To truly master the Python wait function, it’s important to understand the fundamentals. At the heart of Python’s ability to pause execution is the time
module, a built-in Python module for handling time-related tasks.
The time
module provides a range of functions for working with dates and times, but for our purposes, the most important function is time.sleep()
. As we’ve seen, this function makes Python wait for a specified number of seconds.
Here’s a simple example:
import time
print('Start')
time.sleep(2)
print('End')
# Output:
# 'Start'
# (After a 2 second delay, the program prints...)
# 'End'
In this example, time.sleep(2)
makes Python wait for 2 seconds between printing ‘Start’ and ‘End’.
The Concept of Pausing Execution
Pausing execution, or making a program wait, is a fundamental concept in programming. It allows you to control the flow of your program and coordinate actions. For example, you might want to wait for a user to enter input, for a file to be written, or for a network request to complete.
In Python, pausing execution is achieved using the time.sleep()
function or other similar functions. While the program is waiting, it’s not doing anything else. This means that if you have a single-threaded program, it will be completely unresponsive during the wait.
On the other hand, in a multi-threaded program, other threads can continue executing while one thread is waiting. This is where more advanced techniques, like threading and asynchronous programming, come into play.
In conclusion, understanding Python’s time
module and the concept of pausing execution is key to mastering the Python wait function. With these fundamentals in hand, you can create Python programs that are more efficient and responsive.
Real-World Applications of Python Wait Function
The Python wait function is not just a theoretical concept; it has numerous practical applications in real-world scenarios. From web scraping to automation tasks, the ability to pause execution can be a powerful tool in your Python programming arsenal.
Python Wait in Web Scraping
In web scraping, Python’s wait function can be used to avoid overloading the server with too many requests in a short period. By introducing a delay between each request, you can ensure that your web scraping program behaves in a polite and responsible manner.
Python Wait in Automation Tasks
In automation tasks, the wait function can be used to coordinate different actions. For example, you might need to wait for a file to be written before reading it, or wait for a user to enter input before proceeding.
Exploring Related Concepts
To take your Python programming skills to the next level, consider exploring related concepts like multithreading and asynchronous programming. These advanced topics can provide you with more control over the flow of your program and can help you create more efficient and responsive Python applications.
Further Resources for Mastering Python Wait
To deepen your understanding of the Python wait function and related concepts, consider exploring the following resources:
- IOFlood’s Guide to Python Time can help gain insight on Python’s time module and date and time manipulations.
Getting Current Time in Python – Explore techniques for getting the current time in Python applications seamlessly.
Monitoring Execution Time in Python with Timer – Explore Python’s timer functionality for time tracking in your scripts.
Python’s official
time
module documentation is the definitive resource on thetime
module, including thesleep()
function.Python’s official documentation on the
asyncio
module provides in-depth information on asynchronous programming in Python, including theasyncio.sleep()
function.Real Python’s guide to threading in Python provides a practical introduction to multithreading in Python.
By understanding the Python wait function and its practical applications, and by exploring related concepts, you can create Python programs that are more efficient, responsive, and powerful.
Wrapping Up: Mastering the Python Wait Function
In this comprehensive guide, we’ve delved into the depths of the Python wait function, a powerful tool for controlling the flow of your Python programs. From the basic usage of time.sleep()
to more advanced techniques involving loops, threading, and asynchronous programming, we’ve explored the many ways you can make Python wait.
We began with the basics, learning how to use time.sleep()
to pause execution for a specified number of seconds. We then ventured into more advanced territory, exploring how to use the wait function in loops and with threading.
Along the way, we tackled common issues you might encounter when using the wait function, such as interruptions and blocking, providing you with solutions and workarounds for each issue.
We also looked at alternative approaches to pausing execution in Python, introducing you to asyncio.sleep()
, an asynchronous version of time.sleep()
. Here’s a quick comparison of these methods:
Method | Pros | Cons |
---|---|---|
time.sleep() | Simple and easy to use | Blocks the execution of the program during the wait time |
asyncio.sleep() | Doesn’t block the program during the wait time, allowing other tasks to run | Requires a more complex setup and an understanding of asynchronous programming |
Whether you’re a beginner just starting out with Python or an experienced developer looking to level up your skills, we hope this guide has given you a deeper understanding of the Python wait function and its practical applications. Happy coding!