Python Timer Usage Guide
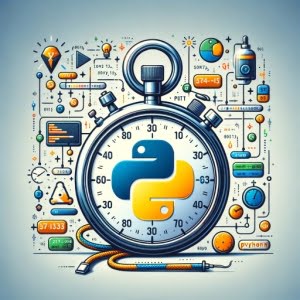
Ever felt like you’re racing against time while coding in Python? You’re not alone. Many developers find themselves in a time crunch when it comes to optimizing their code. But there’s a tool in Python that can help you keep track of time – Python’s timer functions.
Think of Python’s timer functions as a stopwatch – a tool that helps you measure the time taken by different parts of your code, thereby helping you optimize it better. These timer functions are versatile and can be used in various scenarios, from simple code blocks to complex multi-threaded applications.
This guide will walk you through the basics to more advanced techniques of using timers in Python. We’ll explore Python’s built-in timer functions, delve into their advanced features, and even discuss common issues and their solutions.
So, let’s dive in and start mastering Python timers!
TL;DR: How Do I Use a Timer in Python?
Python’s built-in
time
module provides a functiontime()
that returns the current system time. You can use this function to create a simple timer.
Here’s a basic example:
import time
start_time = time.time()
# Your code here
end_time = time.time()
elapsed_time = end_time - start_time
print(f'Time elapsed: {elapsed_time} seconds')
# Output:
# 'Time elapsed: X seconds'
In this example, we import the time
module and use the time()
function to get the current system time before and after the code block we want to time. The difference between the end time and the start time gives us the elapsed time, which is the time taken by our code block.
This is a basic way to use a timer in Python, but there’s much more to learn about timing and optimizing your code. Continue reading for more detailed explanations and advanced usage scenarios.
Table of Contents
- Using Python’s time() Function: A Beginner’s Guide
- Benchmarking with Python’s timeit Module
- Exploring Alternative Timer Methods in Python
- Resolving Common Python Timer Issues
- Understanding Python’s Time and Datetime Modules
- The Concept of Time in Computing
- Benchmarking and Performance Testing
- Beyond Basic Timing: Performance Optimization and Multi-threading
- Wrapping Up: Mastering Python Timers
Using Python’s time() Function: A Beginner’s Guide
Python’s built-in time
module provides a function called time()
. This function returns the current system time in seconds since the epoch (the point where the time starts), which is system-defined. In Unix systems, for example, the epoch is January 1, 1970, 00:00:00 (UTC). So, time()
gives you the number of seconds passed since this point in time.
Let’s see how to use this function to create a simple timer.
import time
start_time = time.time()
# Let's simulate a delay with sleep function
time.sleep(5)
end_time = time.time()
elapsed_time = end_time - start_time
print(f'Time elapsed: {elapsed_time} seconds')
# Output:
# 'Time elapsed: 5.0 seconds'
In the above code, we first record the start time before the delay (simulated by the sleep()
function), and then record the end time after the delay. The difference between the end time and the start time gives us the elapsed time, which is approximately 5 seconds.
This is a simple, yet effective way to measure the time taken by a code block. However, keep in mind that the precision of time()
can be affected by system load, which means the result can slightly vary each time you run the code. This is one of the potential pitfalls when using time()
for timing.
Benchmarking with Python’s timeit Module
As you start working on larger projects, you’ll quickly realize that precision matters when measuring time. This is where Python’s timeit
module comes into play. The timeit
module provides a simple way to time small bits of Python code. It has both a command-line interface and a callable one. It avoids a number of common traps for measuring execution times.
Let’s see an example of how to use timeit
.
import timeit
# Let's time the execution of a list comprehension
start_time = timeit.default_timer()
# List comprehension
numbers = [i for i in range(1000000)]
end_time = timeit.default_timer()
elapsed_time = end_time - start_time
print(f'Time elapsed: {elapsed_time} seconds')
# Output:
# 'Time elapsed: X seconds'
In this example, we’re using timeit.default_timer()
, which is the most precise timer available on our platform. We use it to time the execution of a list comprehension. The timeit
module provides more precision and is more reliable for benchmarking code performance compared to the time()
function.
Keep in mind that timeit
disables the garbage collector by default (which can slow down your code), giving you the best possible time. However, if your code uses resources that need to be cleaned up by the garbage collector, you might get skewed results. You can enable the garbage collector using gc.enable()
if necessary.
The timeit
module is a powerful tool for benchmarking, but like any tool, it’s important to use it correctly. Make sure to understand its ins and outs to get the most accurate results.
Exploring Alternative Timer Methods in Python
While Python’s built-in time
and timeit
modules are quite effective for most use-cases, there are other methods and libraries to create timers, especially when you need more advanced features. Let’s explore some of these alternatives.
Using Python’s datetime Module
Python’s datetime
module can also be used to measure elapsed time. Here’s an example:
from datetime import datetime
start_time = datetime.now()
# Let's simulate a delay with sleep function
time.sleep(5)
end_time = datetime.now()
elapsed_time = end_time - start_time
print(f'Time elapsed: {elapsed_time.seconds} seconds')
# Output:
# 'Time elapsed: 5 seconds'
In this example, we use datetime.now()
to get the current date and time. The difference between the end time and the start time gives us a timedelta
object, from which we can extract the elapsed seconds.
Using Third-party Libraries: pytest
pytest
is a testing framework that allows you to effortlessly create small, simple tests, yet scales to support complex functional testing. It includes a time
fixture for benchmarking.
Here’s an example of how to use pytest
to time a function:
import pytest
# Function to test
def my_function():
time.sleep(5)
def test_my_function(benchmark):
result = benchmark(my_function)
# Run the test
pytest.main(['-v'])
# Output:
# 'test_my_function PASSED (5.00 seconds)'
In this example, we use pytest
‘s benchmark
fixture to time the execution of my_function()
. The test will pass if the function works correctly, and the output will show the time taken by the function.
Each of these methods has its own advantages and disadvantages. For example, timeit
is great for precision, but it disables the garbage collector by default, which might not be ideal for all scenarios. On the other hand, datetime
is less precise but more versatile. pytest
provides a great way to time functions during testing, but it’s a third-party library and may be overkill for simple tasks.
Method | Precision | Garbage Collector | Use Case |
---|---|---|---|
time | Low | Enabled | Simple tasks |
timeit | High | Disabled | Benchmarking |
datetime | Medium | Enabled | Versatile |
pytest | High | Depends on settings | Testing |
Choosing the right method depends on your specific needs. If you’re just starting out or working on a simple project, Python’s built-in time
module might be enough. But as you work on larger, more complex projects, you might need to consider other options.
Resolving Common Python Timer Issues
While Python timers are incredibly useful, they are not without their quirks. Here are a few common issues you might encounter and some tips to resolve them.
Time Precision and Resolution
The precision of your timer can be influenced by several factors. For instance, the time()
function from the time
module measures time in seconds since the epoch. However, it only provides precision up to microseconds. If you need more precision, you might want to consider using a different method.
The timeit
module, for example, provides a higher precision timer. It measures time in fractions of a second, making it a better choice for benchmarking or timing short code snippets.
Here’s an example of how you might use timeit
for high precision timing:
import timeit
start_time = timeit.default_timer()
# Your code here
time.sleep(0.123)
end_time = timeit.default_timer()
elapsed_time = end_time - start_time
print(f'Time elapsed: {elapsed_time} seconds')
# Output:
# 'Time elapsed: 0.123 seconds'
System-Dependent Limitations
Python’s time functions are system-dependent. This means that they rely on the underlying system’s capabilities to measure time. As a result, the precision and maximum measurable time can vary between different systems.
If you’re encountering limitations with Python’s built-in time functions, you might want to consider using a third-party library. Libraries like pytest
offer more advanced timing features and can provide higher precision and longer maximum measurable times.
Remember, the right tool for the job depends on your specific needs. Understanding the strengths and limitations of each method will help you choose the best one for your project.
Understanding Python’s Time and Datetime Modules
Python provides several modules for dealing with time, the most commonly used ones being time
and datetime
. Both modules provide functions for working with dates and times, but they serve different purposes.
The time
module is primarily for working with Unix timestamps, which are floating-point numbers representing seconds since the epoch (January 1, 1970). It provides functions to parse these timestamps into more human-readable formats, and to create timers.
Here’s an example of using the time
module to get the current Unix timestamp and convert it into a more readable format:
import time
timestamp = time.time()
print(f'Unix timestamp: {timestamp}')
readable_time = time.ctime(timestamp)
print(f'Readable time: {readable_time}')
# Output:
# 'Unix timestamp: X.XXX'
# 'Readable time: Wed Dec 1 10:20:30 2022'
On the other hand, the datetime
module is more high-level and provides easier ways to manipulate dates and times. It provides the datetime
class which combines date and time information, and provides methods to manipulate and format such data.
Here’s an example of using the datetime
module to get the current date and time:
from datetime import datetime
current_datetime = datetime.now()
print(f'Current datetime: {current_datetime}')
# Output:
# 'Current datetime: 2022-12-01 10:20:30.123456'
The Concept of Time in Computing
In computing, time is a critical concept. It’s used for a variety of purposes, from timestamping events to measuring the performance of algorithms. Understanding how time works in computing can help you better understand and use Python’s timer functions.
Benchmarking and Performance Testing
Benchmarking is the process of measuring the performance of a system or a component of the system under certain conditions. In Python, we often use benchmarking to measure the performance of our code. This can help us identify bottlenecks and optimize our code for better performance.
Performance testing, on the other hand, is a broader term that includes testing the speed, responsiveness, and stability of a system under a workload. Python provides several tools for performance testing, including the timeit
module we discussed earlier.
Understanding these concepts can help you make the most out of Python’s timer functions, whether you’re timing a simple code block or benchmarking a complex algorithm.
Beyond Basic Timing: Performance Optimization and Multi-threading
Python timers are not just about measuring time. They play a crucial role in performance optimization and multi-threading, two advanced concepts in Python programming.
Performance Optimization with Timers
Performance optimization is all about making your code run faster or use less resources. Python timers can help you identify bottlenecks in your code, i.e., parts of your code that are taking too long to run. Once you’ve identified these bottlenecks, you can focus your optimization efforts on these areas.
Multi-threading and Timers
Multi-threading is a technique where multiple threads are spawned by the main program to execute tasks concurrently. Python’s threading
module provides a Timer
class that starts its work after a delay. This can be useful in multi-threaded programs where tasks need to be scheduled at specific intervals.
Here’s an example of using the Timer
class:
from threading import Timer
def hello():
print('Hello, World!')
t = Timer(5.0, hello)
t.start()
# Output (after 5 seconds):
# 'Hello, World!'
In this example, we create a Timer
object that waits for 5 seconds before calling the hello
function.
Asynchronous Programming in Python
Asynchronous programming is a form of parallel programming that allows a unit of work to run separately from the main application thread. When the work is complete, it notifies the main thread about completion or failure, so the main thread can then pick up the result and continue execution.
Python’s asyncio
module provides support for asynchronous I/O through coroutines and tasks, which can be timed using the time
module in a similar way as synchronous code.
Further Resources for Mastering Python Timers
If you’re interested in diving deeper into Python timers and related topics, here are a few resources to get you started:
- Python Time Tutorial: Getting Started – Discover Python’s time module for accurate time measurements and synchronization.
Simplifying Code Delays with Python Wait – Explore Python’s wait functionality for synchronizing tasks and managing delays.
Python Get Current Date: Accessing Today’s Date – Learn how to retrieve the current date in Python effortlessly.
Python’s Official Documentation on time – A comprehensive guide on Python’s
time
module.Python’s Official Documentation on datetime – A detailed guide on Python’s
datetime
module.Python’s Official Documentation on timeit – A guide on benchmarking and performance testing with
timeit
.
Remember, mastering Python timers is not just about learning the syntax. It’s about understanding the underlying concepts and knowing how and when to apply them. Happy learning!
Wrapping Up: Mastering Python Timers
In this comprehensive guide, we’ve delved into the world of Python timers, a crucial tool for optimizing code and measuring performance in Python.
We began with the basics, exploring how to create a simple timer using Python’s built-in time
module. We then delved into more advanced usage, learning how to use the timeit
module for precise benchmarking and performance testing.
We also discussed common issues you might face when using Python timers, such as time precision and system-dependent limitations, and provided solutions for each issue.
We didn’t stop there. We explored alternative methods to create timers, such as using Python’s datetime
module and third-party libraries like pytest
. Each method has its own strengths and weaknesses, and choosing the right one depends on your specific needs.
Here’s a quick comparison of these methods:
Method | Precision | Garbage Collector | Use Case |
---|---|---|---|
time | Low | Enabled | Simple tasks |
timeit | High | Disabled | Benchmarking |
datetime | Medium | Enabled | Versatile |
pytest | High | Depends on settings | Testing |
Python timers are not just about measuring time – they’re about optimizing your code, benchmarking your algorithms, and understanding the performance of your Python programs.
With the knowledge you’ve gained from this guide, you’re now well-equipped to master Python timers. Happy coding!