Selenium Guide: Learn Python Web Automation
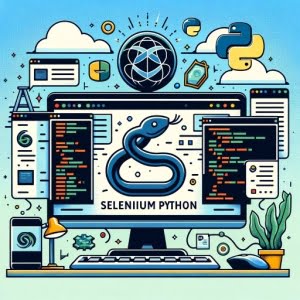
Are you finding it challenging to automate your web browser tasks using Selenium in Python? You’re not alone. Many developers face hurdles when first diving into web automation. But don’t worry, we’re here to help.
This guide is designed to be your personal assistant in this journey, turning Selenium into your own web surfing robot. It’s capable of interacting with web pages just like a human would, and we’re going to show you how.
Whether you’re just starting out or looking to enhance your skills, this guide will walk you through from the basics to advanced techniques of using Selenium with Python.
TL;DR: How Do I Use Selenium with Python?
To use Selenium with Python, you first need
pip install selenium
. Then, your import the webdriver:from selenium import webdriver
. Then you can start automating your browser tasks.
Here’s a simple example:
from selenium import webdriver
driver = webdriver.Firefox()
driver.get('http://www.google.com')
# Output:
# Firefox opens and navigates to Google's homepage.
In this example, we import the webdriver from the selenium module, initialize a new Firefox browser instance, and navigate to Google’s homepage.
This is a basic way to use Selenium with Python, but there’s so much more you can do with it. Continue reading for a more detailed guide on using Selenium with Python, from basic to advanced techniques.
Table of Contents
Getting Started with Selenium Python
The first step to using Selenium with Python is installing the selenium package. You can do this via pip, Python’s package installer. Here’s how:
pip install selenium
With Selenium installed, we can now set up a webdriver. The webdriver is a crucial component of Selenium as it interfaces with the chosen browser. Here’s how to set up a webdriver for Firefox:
from selenium import webdriver
driver = webdriver.Firefox()
In this code, we import the webdriver from the selenium module and create a new instance of the Firefox browser.
Navigating to a Webpage
To navigate to a webpage, we use the get
method of the driver object. For example, to navigate to Google’s homepage, we’d do:
driver.get('http://www.google.com')
# Output:
# Firefox opens and navigates to Google's homepage.
Finding Elements
Selenium allows us to find elements on the webpage using methods like find_element_by_name
, find_element_by_id
, and find_element_by_xpath
. Here’s an example of how to find a search box on a webpage:
search_box = driver.find_element_by_name('q')
In the above code, we’re finding the search box element by its name, which is ‘q’ in this case.
Clicking Buttons
To simulate a mouse click on an element, we use the click
method. Here’s how to click a ‘submit’ button:
submit_button = driver.find_element_by_name('btnK')
submit_button.click()
Filling Out Forms
To fill out forms, we use the send_keys
method. Here’s how to enter text into the previously found search box:
search_box.send_keys('Python')
This will type ‘Python’ into the search box.
By mastering these basic tasks, you’ll be well on your way to automating your browser with Selenium Python. In the next section, we’ll delve into more advanced techniques.
Intermediate Selenium Python Techniques
After familiarizing yourself with the basics, it’s time to dive into more complex tasks. These include handling alerts, switching between windows or frames, and dealing with dropdowns.
Handling Alerts
Alerts are pop-up boxes that appear on your browser, usually to get some kind of input from you. Selenium can interact with these alerts. Let’s say an alert pops up on your browser, you can handle it like this:
alert = driver.switch_to.alert
alert.accept()
# Output:
# The alert box is accepted and dismissed.
In the above code, we first switch to the alert using driver.switch_to.alert
, then we accept the alert using alert.accept()
.
Switching Between Windows or Frames
While working with Selenium, you might encounter situations where you need to switch between different windows or frames. Here’s how to do it:
# Switch to the new window
driver.switch_to.window(driver.window_handles[-1])
# Output:
# The driver switches to the last opened window.
In this code, driver.window_handles[-1]
gives us the handle of the last window. We then switch to this window using driver.switch_to.window()
.
Dealing with Dropdowns
Dropdowns are another common element you’ll encounter on web pages. Selenium can select options from dropdowns using the Select class. Here’s how:
from selenium.webdriver.support.ui import Select
select = Select(driver.find_element_by_name('dropdown'))
select.select_by_visible_text('Option1')
# Output:
# 'Option1' is selected from the dropdown.
In this example, we first import the Select class, then we find the dropdown element, and finally, we select an option using select_by_visible_text()
.
By mastering these intermediate techniques, you’ll be able to handle more complex web automation tasks with Selenium Python. In the next section, we’ll explore alternative approaches and when to use them.
Exploring Alternative Web Automation Tools
While Selenium is a powerful tool for web automation, it’s not the only one available. Other Python libraries, such as BeautifulSoup and Scrapy, can also be used for web automation tasks. Let’s briefly explore these alternatives.
BeautifulSoup: Simple Web Scraping
BeautifulSoup is a Python library used for parsing HTML and XML documents. It’s often used for web scraping, which is the process of extracting data from websites. Here’s a simple example of how to use BeautifulSoup to scrape a webpage:
from bs4 import BeautifulSoup
import requests
response = requests.get('http://www.example.com')
soup = BeautifulSoup(response.text, 'html.parser')
title = soup.find('title')
print(title.string)
# Output:
# 'Example Domain'
In this example, we first send a GET request to the webpage using the requests module. We then parse the response text using BeautifulSoup and find the title element. The title string is then printed.
While BeautifulSoup is great for simple web scraping tasks, it doesn’t interact with JavaScript and can’t simulate user interactions like Selenium can.
Scrapy: Powerful Web Crawling
Scrapy is another Python library used for web scraping and crawling, but it’s more powerful and flexible than BeautifulSoup. It’s designed for large-scale web scraping tasks. Here’s a simple example of how to use Scrapy:
import scrapy
class ExampleSpider(scrapy.Spider):
name = 'example'
start_urls = ['http://www.example.com']
def parse(self, response):
title = response.css('title::text').get()
print(title)
# Output:
# 'Example Domain'
In this example, we define a Scrapy Spider to crawl the webpage and extract the title. Note that Scrapy is asynchronous and can handle multiple requests at once, making it faster than Selenium for large-scale scraping tasks.
While Scrapy is powerful, it has a steeper learning curve than Selenium and BeautifulSoup, and it’s overkill for simple web automation tasks.
In conclusion, while Selenium is excellent for simulating user interactions and automating browser tasks, BeautifulSoup and Scrapy are better suited for web scraping tasks. Choose the tool that best fits your needs.
Troubleshooting Common Selenium Python Issues
While Selenium is a powerful tool for web automation, it’s not without its quirks and challenges. In this section, we’ll discuss common issues you might encounter when using Selenium Python, such as dealing with dynamic content, handling timeouts, and dealing with CAPTCHAs. For each issue, we’ll provide solutions and workarounds, along with code examples.
Dealing with Dynamic Content
Dynamic content is content that changes without the page reloading, usually as a result of JavaScript. Selenium can handle dynamic content, but it requires explicit waits. Here’s an example:
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
driver = webdriver.Firefox()
driver.get('http://www.example.com')
try:
element = WebDriverWait(driver, 10).until(
EC.presence_of_element_located((By.ID, 'dynamicContent'))
)
finally:
driver.quit()
# Output:
# The driver waits up to 10 seconds for the dynamic content to load.
In this code, we use WebDriverWait
along with expected_conditions
to wait until the dynamic content is loaded.
Handling Timeouts
Timeouts can occur when a certain operation doesn’t complete within a specified time. To handle timeouts, you can use implicit waits. Here’s how:
driver = webdriver.Firefox()
driver.implicitly_wait(10) # seconds
driver.get('http://www.example.com')
element = driver.find_element_by_id('myDynamicElement')
# Output:
# The driver waits up to 10 seconds before throwing a NoSuchElementException.
In this code, we use driver.implicitly_wait()
to wait up to 10 seconds before throwing a NoSuchElementException.
Dealing with CAPTCHAs
CAPTCHAs are designed to prevent bots from interacting with web pages, and they’re a common hurdle when automating web browsers. While there’s no perfect solution for dealing with CAPTCHAs using Selenium, third-party services like 2Captcha can solve CAPTCHAs for a fee.
By understanding these common issues and their solutions, you’ll be better equipped to use Selenium Python for your web automation tasks. In the next section, we’ll delve into the fundamentals of Selenium and how it works.
Understanding Selenium’s Inner Workings
To effectively use Selenium Python for web automation, it’s beneficial to understand how Selenium works, its architecture, and how it interacts with different webdrivers.
Selenium’s Architecture
At its core, Selenium is a framework for automating browsers. It provides a way for developers to write scripts in several popular programming languages, including Python. These scripts then communicate with a browser through a driver, such as ChromeDriver for Google Chrome or GeckoDriver for Firefox. The driver receives commands from the Selenium script and executes them on the browser, returning any results.
Here’s a simple illustration of this process:
from selenium import webdriver
driver = webdriver.Firefox()
driver.get('http://www.example.com')
# Output:
# Firefox opens and navigates to 'http://www.example.com'.
In this code, we create a new instance of the Firefox webdriver, which opens a new Firefox window. We then send a command to navigate to 'http://www.example.com'
. The driver executes this command on the browser and returns any results.
Python and Web Automation
Python is a popular language for web automation due to its simplicity and the powerful libraries it offers, such as Selenium. With Python and Selenium, you can automate a variety of browser tasks, such as filling out forms, clicking buttons, and navigating between pages.
Here’s an example of how to fill out a form using Selenium Python:
from selenium import webdriver
driver = webdriver.Firefox()
driver.get('http://www.example.com')
form_field = driver.find_element_by_name('form_field')
form_field.send_keys('Hello, World!')
# Output:
# The form field with the name 'form_field' is filled with 'Hello, World!'.
In this code, we first navigate to 'http://www.example.com'
. We then find a form field by its name and fill it with the text 'Hello, World!'
.
By understanding the basics of Selenium and Python, you’ll be better equipped to use them for your web automation tasks. In the next section, we’ll explore how Selenium can be used beyond web automation.
Expanding Selenium’s Horizons
While Selenium is primarily known for web automation, its capabilities stretch far beyond that. Selenium is also a powerful tool for web scraping and testing web applications, making it a versatile tool in any developer’s toolkit.
Web Scraping with Selenium
Web scraping is the process of extracting data from websites. While there are many tools available for web scraping, Selenium stands out due to its ability to interact with JavaScript and simulate user interactions. Here’s a simple example of how to use Selenium for web scraping:
from selenium import webdriver
driver = webdriver.Firefox()
driver.get('http://www.example.com')
page_title = driver.title
print(page_title)
# Output:
# 'Example Domain'
In this code, we navigate to 'http://www.example.com'
and print the title of the webpage. Selenium’s ability to interact with JavaScript makes it possible to scrape websites that other tools may struggle with.
Testing Web Applications with Selenium
Selenium is also widely used for testing web applications. It can simulate user interactions, making it possible to automate tests that would otherwise be time-consuming to perform manually. Here’s a simple example of how to use Selenium to test a login form:
from selenium import webdriver
driver = webdriver.Firefox()
driver.get('http://www.example.com/login')
username_field = driver.find_element_by_name('username')
password_field = driver.find_element_by_name('password')
username_field.send_keys('testuser')
password_field.send_keys('testpassword')
submit_button = driver.find_element_by_name('submit')
submit_button.click()
# Output:
# The login form is filled and submitted.
In this code, we navigate to a login page, fill out the login form, and click the submit button. Selenium allows us to automate this process and check whether the login functionality is working as expected.
Further Resources for Selenium Python Mastery
To dive deeper into Selenium Python, check out these resources:
- Beginner’s Guide to Python Web Scraping – Master the techniques for navigating and parsing HTML with Python.
Python Web Scraping with Beautiful Soup explores how Beautiful Soup eases web scraping and data extraction in Python.
Python requests.post(): Sending HTTP POST Requests – Discover how to send HTTP POST requests using the “requests” library.
Selenium Python Bindings 2 Documentation – The official documentation for Selenium Python that covers all aspects of Selenium Python.
Practical Programming for Total Beginners provides an introduction to web scraping with Python.
Selenium WebDriver With Python Tutorial provides practical examples of how to use Selenium Python for web automation and testing.
By exploring these resources and experimenting with Selenium Python, you’ll be well on your way to mastering web automation.
Wrapping Up Selenium Python
In this guide you learned about the powerful Python library Selenium, which can be used to automate web browsing tasks from within Python.
We began with an exploration of Selenium Python
and its fundamental concepts. We learned how to install Selenium, set up a webdriver, and perform basic tasks like navigating to a webpage, finding elements, clicking buttons, and filling out forms. We then delved into more advanced techniques like handling alerts, switching between windows or frames, and dealing with dropdowns.
We also explored alternative approaches to web automation, comparing Selenium with other Python libraries like BeautifulSoup and Scrapy. While Selenium excels at simulating user interactions and automating browser tasks, we learned that BeautifulSoup and Scrapy are more suited for web scraping tasks.
We also discussed common issues and their solutions, such as dealing with dynamic content, handling timeouts, and dealing with CAPTCHAs. We learned that Selenium’s power comes with its quirks and challenges, but with the right knowledge and techniques, these can be effectively managed.
Here’s a comparison table of Selenium and other web automation libraries:
Library | Web Automation | Web Scraping | Learning Curve |
---|---|---|---|
Selenium | Excellent | Good | Moderate |
BeautifulSoup | Poor | Excellent | Easy |
Scrapy | Poor | Excellent | Hard |
In conclusion, Selenium Python is a powerful tool for web automation. Whether you’re a beginner just starting out or an expert looking to enhance your skills, this guide has provided you with the knowledge and techniques to master Selenium Python. Happy automating!