Python Requests Library for ‘POST’ Requests
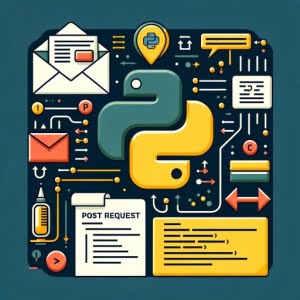
Are you finding it challenging to make POST requests in Python? You’re not alone. Many developers grapple with this task, but there’s a tool that can make this process a breeze.
Like a digital courier, Python’s requests library can deliver data to web servers with ease. These POST requests are a fundamental part of interacting with web servers.
This guide will walk you through the process of making POST requests in Python, from the basics to more advanced techniques, allowing you to send data to a server and receive a response.
So, let’s dive in and start mastering Python POST requests!
TL;DR: How Do I Make a POST Request in Python?
To make a POST request in Python, you can use the
requests.post()
function in Python’s requests library. This function allows you to send data to a server and receive a response.
Here’s a simple example:
import requests
response = requests.post('https://httpbin.org/post', data = {'key':'value'})
print(response.text)
# Output:
# The server's response to your POST request
In this example, we import the requests library and use the requests.post()
function to make a POST request to the server at ‘https://httpbin.org/post’. We send data in the form of a dictionary, with ‘key’ and ‘value’ as its elements. The server’s response to our POST request is then printed out.
This is a basic way to make a POST request in Python, but there’s much more to learn about this topic. Continue reading for a more detailed guide on making POST requests in Python, including more complex uses and troubleshooting tips.
Table of Contents
- Understanding the Basics of requests.post()
- Delving Deeper: Advanced POST Requests
- Exploring Alternatives: urllib and http.client
- Troubleshooting Common Issues
- HTTP Requests and Python’s Requests Library
- The Power of POST: Web Scraping, API Interaction, and Data Submission
- Wrapping Up: Mastering POST Requests in Python
Understanding the Basics of requests.post()
Let’s start by understanding the requests.post()
function. This function is part of the requests library in Python, a powerful tool for making HTTP requests. It allows us to send HTTP POST requests to a server, which is essentially like submitting a form on a webpage.
Here’s a basic example of how to use it:
import requests
url = 'https://httpbin.org/post'
data = {'Key1': 'Value1', 'Key2': 'Value2'}
response = requests.post(url, data=data)
print(response.text)
# Output:
# The server's response to your POST request
In this example, we first import the requests library. We then define the URL we want to send our POST request to and the data we want to send. This data is in the form of a dictionary. We then use the requests.post()
function to send our POST request, passing in our URL and data as parameters. The server’s response is stored in the response
variable, which we print out.
One of the advantages of using the requests.post()
function is its simplicity and readability. It abstracts the complexities of making HTTP requests, allowing you to send POST requests with just a few lines of code.
However, it’s important to be aware of potential pitfalls. For instance, the server you’re sending your request to needs to be able to handle POST requests. If it doesn’t, you may encounter errors. Also, the data you’re sending needs to be in a format the server can understand, usually a dictionary or a list of tuples, or you may run into issues.
Delving Deeper: Advanced POST Requests
Once you’ve mastered the basics of making POST requests with Python’s requests library, you can move on to more advanced techniques. These include sending JSON data, handling response status codes, and dealing with cookies and headers.
Sending JSON Data
In many cases, you might need to send JSON data in your POST request. This is especially common when interacting with APIs. Here’s how you can do it:
import requests
import json
url = 'https://httpbin.org/post'
data = {'Key1': 'Value1', 'Key2': 'Value2'}
response = requests.post(url, data=json.dumps(data))
print(response.text)
# Output:
# The server's response to your POST request
In this example, we’re using the json.dumps()
function to convert our dictionary into a JSON-formatted string before sending it in our POST request.
Handling Response Status Codes
When you make a POST request, the server responds with a status code. This code tells you whether your request was successful and, if not, why it failed. Here’s how you can check the status code of a response:
import requests
url = 'https://httpbin.org/post'
data = {'Key1': 'Value1', 'Key2': 'Value2'}
response = requests.post(url, data=data)
print(response.status_code)
# Output:
# 200
In this example, we’re printing out the status code of the response. A status code of 200 means the request was successful. Other common status codes include 404 (Not Found) and 500 (Internal Server Error).
Dealing with Cookies and Headers
Sometimes, you might need to send or receive cookies in your POST request, or you might need to set custom headers. Here’s how you can do it:
import requests
url = 'https://httpbin.org/post'
data = {'Key1': 'Value1', 'Key2': 'Value2'}
cookies = {'CookieName': 'CookieValue'}
headers = {'Custom-Header': 'CustomValue'}
response = requests.post(url, data=data, cookies=cookies, headers=headers)
print(response.text)
# Output:
# The server's response to your POST request
In this example, we’re sending a cookie and a custom header in our POST request. We’re using the cookies
and headers
parameters of the requests.post()
function to do this.
These advanced techniques allow you to handle a wide range of scenarios when making POST requests. However, they also introduce more complexity and potential sources of errors, so be sure to understand them thoroughly before using them.
Exploring Alternatives: urllib and http.client
While the requests library is a popular choice for making POST requests in Python, it’s not the only option. Let’s explore two alternative methods: urllib and http.client.
Making POST Requests with urllib
urllib
is a built-in Python module for handling URLs and making HTTP requests. Here’s how you can make a POST request with it:
import urllib.request
import urllib.parse
url = 'https://httpbin.org/post'
data = urllib.parse.urlencode({'Key1': 'Value1', 'Key2': 'Value2'}).encode()
req = urllib.request.Request(url, data=data)
response = urllib.request.urlopen(req)
print(response.read().decode())
# Output:
# The server's response to your POST request
In this example, we’re using the urllib.request.Request()
function to create a POST request, and the urllib.request.urlopen()
function to send it. We’re also using the urllib.parse.urlencode()
function to encode our data into a format suitable for a POST request.
Making POST Requests with http.client
http.client
is another built-in Python module for making HTTP requests. Here’s how you can make a POST request with it:
import http.client
conn = http.client.HTTPSConnection('httpbin.org')
headers = {'Content-type': 'application/x-www-form-urlencoded'}
data = 'Key1=Value1&Key2=Value2'
conn.request('POST', '/post', data, headers)
response = conn.getresponse()
print(response.read().decode())
# Output:
# The server's response to your POST request
In this example, we’re using the http.client.HTTPSConnection()
function to establish a connection to the server, and the request()
method to send a POST request.
Both urllib and http.client provide more control over your HTTP requests than the requests library, which can be useful in certain situations. However, they also have a steeper learning curve and require more code to accomplish the same tasks. For most use cases, the simplicity and convenience of the requests library make it the better choice.
Troubleshooting Common Issues
While making POST requests in Python is generally straightforward, you might encounter some issues. Let’s discuss some common challenges, their solutions, and some helpful tips.
Handling Different Response Status Codes
One common issue is dealing with different response status codes. As we discussed earlier, the status code of a response can tell you a lot about what happened with your request. Here’s how you can handle different status codes:
import requests
url = 'https://httpbin.org/post'
data = {'Key1': 'Value1', 'Key2': 'Value2'}
response = requests.post(url, data=data)
if response.status_code == 200:
print('Request was successful.')
elif response.status_code == 404:
print('The requested resource could not be found.')
else:
print(f'Received status code {response.status_code}')
# Output:
# 'Request was successful.'
In this example, we’re checking the status code of the response and printing out a different message depending on the code.
Dealing with Timeouts
Another common issue is dealing with timeouts. If a server takes too long to respond to your request, it might time out. Here’s how you can handle timeouts:
import requests
url = 'https://httpbin.org/post'
data = {'Key1': 'Value1', 'Key2': 'Value2'}
try:
response = requests.post(url, data=data, timeout=5)
print(response.text)
except requests.exceptions.Timeout:
print('The request timed out')
# Output:
# 'The request timed out'
In this example, we’re using the timeout
parameter of the requests.post()
function to set a timeout of 5 seconds. If the server doesn’t respond within this time, a requests.exceptions.Timeout
exception is raised, which we catch and handle by printing out a message.
Managing SSL Certificates
If you’re making a POST request to a server over HTTPS, you might need to deal with SSL certificates. Here’s how you can handle them:
import requests
url = 'https://httpbin.org/post'
data = {'Key1': 'Value1', 'Key2': 'Value2'}
response = requests.post(url, data=data, verify='/path/to/certfile')
print(response.text)
# Output:
# The server's response to your POST request
In this example, we’re using the verify
parameter of the requests.post()
function to specify the path to a CA_BUNDLE file or directory with certificates of trusted CAs. This tells requests to verify the SSL certificate of the server.
These are just a few examples of the issues you might encounter when making POST requests in Python. Remember, the key to effective troubleshooting is understanding the problem and knowing where to look for solutions.
HTTP Requests and Python’s Requests Library
To fully grasp the concept of making POST requests in Python, it’s essential to understand the basics of HTTP requests and the structure of a POST request.
HTTP Requests: GET vs POST
HTTP, or Hypertext Transfer Protocol, is the foundation of any data exchange on the Web. HTTP requests are messages sent by the client to initiate an action on the server. There are several types of HTTP requests, but the two most common are GET and POST.
GET requests are used to retrieve data from a server. When you type a URL into your browser’s address bar, you’re making a GET request to that URL.
POST requests, on the other hand, are used to send data to a server. For example, when you fill out a form on a website and click ‘submit’, you’re making a POST request with the form data.
Structure of a POST Request
A POST request is made up of several parts:
- The request line, which includes the request method (POST) and the URL
- The headers, which provide information about the request
- The body, which contains the data being sent to the server
Here’s an example of what a POST request might look like:
POST /post HTTP/1.1
Host: httpbin.org
Content-Type: application/x-www-form-urlencoded
Content-Length: 27
Key1=Value1&Key2=Value2
In this example, the request line is ‘POST /post HTTP/1.1’, the headers include ‘Host’, ‘Content-Type’, and ‘Content-Length’, and the body is ‘Key1=Value1&Key2=Value2’.
Python’s Requests Library
Python’s requests library is a built-in Python module for making HTTP requests. It abstracts the complexities of making requests behind a beautiful, simple API, allowing you to send HTTP/1.1 requests with various methods like GET, POST, and others. With it, you can add content like headers, form data, multipart files, and parameters to HTTP requests via simple Python libraries to HTTP requests.
In the context of making POST requests, the requests library provides the requests.post()
function. This function allows you to send HTTP POST requests to a specified URL, optionally passing data to send to the server in the body of the request.
The Power of POST: Web Scraping, API Interaction, and Data Submission
POST requests are a vital part of many areas in Python programming, including web scraping, API interaction, and data submission.
POST in Web Scraping
Web scraping is the process of extracting data from websites. While GET requests are commonly used in web scraping to retrieve the HTML of a webpage, POST requests can also be used when you need to send data to the server, such as form data or cookies, to get the information you need.
POST in API Interaction
APIs, or Application Programming Interfaces, allow different software applications to communicate with each other. Many APIs require you to make POST requests to send data to the server, such as creating a new resource or updating an existing one.
POST in Data Submission
When you submit data through a form on a website, you’re typically making a POST request. Python can automate this process, allowing you to submit data to websites programmatically.
Related Concepts: GET Requests, REST APIs, and JSON Handling
If you’re interested in learning more about making POST requests in Python, you might also want to explore related concepts like GET requests, REST APIs, and JSON handling.
GET requests, like POST requests, are a type of HTTP request. However, while POST requests are used to send data to a server, GET requests are used to retrieve data from a server.
REST APIs are a type of API that use HTTP requests to interact with data. They can use various types of HTTP requests, including both GET and POST.
JSON, or JavaScript Object Notation, is a common data format for sending data in HTTP requests, including POST requests. Python’s json module provides functions for working with JSON data.
Further Resources for Mastering Python POST Requests
To deepen your understanding of POST requests in Python, consider checking out the following resources:
- IOFlood’s Python Web Scraping Article – Explore web scraping ethics and best practices in Python development.
Automating Web Testing with Selenium in Python – Dive into browser automation and interaction for web applications.
URL Handling Made Easy with Python urllib – Dive into URL manipulation, retrieval, and content download using urllib.
Python’s Official Documentation on the Requests Library – Get comprehensive insights into Python’s Requests library.
Sending HTTP Requests with Python by Real Python presents a detailed tutorial on sending HTTP requests using Python for practical data fetching.
Mozilla’s Guide to HTTP Requests provides a guide on HTTP POST requests for enhanced web interactions.
Wrapping Up: Mastering POST Requests in Python
In this comprehensive guide, we’ve explored the ins and outs of making POST requests in Python using the requests library. We’ve journeyed through the basics of requests.post()
function, delved into more advanced usage, and even explored alternative methods.
We started off with the basics, learning how to use the requests.post()
function to send data to a server. We then ventured into more advanced territory, exploring how to send JSON data, handle response status codes, and deal with cookies and headers. We also discussed common issues you might encounter when making POST requests, such as handling different types of response status codes, dealing with timeouts, and managing SSL Certificates, providing you with solutions and workarounds for each issue.
We also looked at alternative approaches to making POST requests in Python, comparing the requests library with other built-in Python modules like urllib and http.client. Here’s a quick comparison of these methods:
Method | Pros | Cons |
---|---|---|
requests.post() | Simple, readable, abstracts complexities | Server must handle POST requests, data format must be acceptable |
urllib | More control, built-in module | More complex, requires more code |
http.client | More control, built-in module | More complex, requires more code |
Whether you’re a beginner just starting out with making POST requests in Python, or an experienced developer looking to level up your skills, we hope this guide has given you a deeper understanding of the process, its challenges, and how to overcome them.
With this knowledge, you’re well-equipped to make the most of POST requests in your Python projects. Happy coding!