Python round() | Function Guide (With Examples)
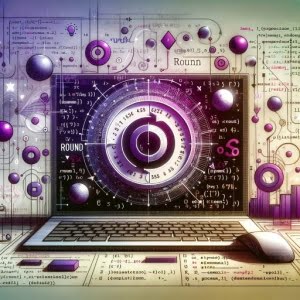
Ever felt like you’re wrestling with Python’s round function? Don’t worry, you’re not alone. Once you get the hang of it, you’ll see it as nothing less than a mathematical magician, adept at rounding numbers to your desired precision.
In this comprehensive guide, we’ll start from the ground up, explaining the basics of the round function in Python. Then, we’ll gradually venture into its more advanced applications.
Whether you’re a beginner trying to get your head around Python rounding, or an intermediate user looking to level up your skills, this guide is for you.
Let’s dive into the world of Python’s round function!
TL;DR: How Do I Use the Round Function in Python?
The round function in Python is a built-in function that rounds a number to the nearest even number. Here’s a quick example:
num = 3.14159
rounded_num = round(num, 2)
print(rounded_num)
# Output:
# 3.14
In this example, we have a number num
with the value of 3.14159. We use the round
function with two arguments: the number we want to round (num
), and the number of decimal places (2
). The round
function returns the rounded number, which we store in the variable rounded_num
. When we print rounded_num
, we get 3.14
as the output.
For a more in-depth understanding of how Python’s round function works, including its quirks and advanced applications, keep reading.
Table of Contents
- Understanding Python’s Round Function: A Beginner’s Perspective
- Rounding to Different Decimal Places
- Rounding Negative Numbers
- Exploring Alternatives to Python’s Round Function
- Navigating Through Common Python Rounding Issues
- Python Number Data Types and Rounding: The Foundations
- The Impact of Rounding in Data Analysis and Scientific Computing
- Further Resources for Python Functions
- Wrapping Up: Python’s Round Function Demystified
Understanding Python’s Round Function: A Beginner’s Perspective
Python’s built-in round
function is a powerful tool used to round a number to a specified number of decimal places. The function takes two arguments: the number you want to round and the number of decimal places to which you want to round the number. Here’s a basic example of how it works:
num = 3.653
rounded_num = round(num, 2)
print(rounded_num)
# Output:
# 3.65
In this example, num
is the number we want to round, and 2
is the number of decimal places. The round
function returns 3.65
, which is num
rounded to two decimal places.
One of the advantages of Python’s round
function is its simplicity and ease of use. However, it’s important to understand that Python rounds to the nearest even number when the number to be rounded is exactly halfway between two others. This is known as ’round half to even’ or ‘bankers’ rounding’. It’s a strategy used to prevent bias in rounding.
For example:
print(round(0.5))
print(round(1.5))
# Output:
# 0
# 2
In this case, 0.5
is exactly halfway between 0
and 1
, so Python rounds to the nearest even number, which is 0
. Similarly, 1.5
is halfway between 1
and 2
, so Python rounds to 2
, the nearest even number. This might be unexpected if you’re accustomed to traditional rounding methods where 0.5
would round up to 1
.
Rounding to Different Decimal Places
Python’s round
function allows you to round to any number of decimal places you need. This is achieved by specifying the second argument in the round
function. Let’s look at an example:
num = 3.14159
print(round(num))
print(round(num, 1))
print(round(num, 3))
print(round(num, 5))
# Output:
# 3
# 3.1
# 3.142
# 3.14159
In this example, we’re rounding the number 3.14159
to different decimal places. When no second argument is provided, Python rounds the number to the nearest integer. As we specify more decimal places, the rounding becomes more precise.
Rounding Negative Numbers
Python’s round
function also allows rounding of negative numbers. The function behaves in the same way as with positive numbers. Here’s a quick example:
num = -3.14159
print(round(num))
print(round(num, 1))
print(round(num, 3))
print(round(num, 5))
# Output:
# -3
# -3.1
# -3.142
# -3.14159
In this example, we’re rounding the negative number -3.14159
to different decimal places. The round
function treats negative numbers in the same way as positive numbers, rounding to the nearest even number in case of a tie.
As a best practice, always specify the number of decimal places when using the round
function to ensure the precision level you need. Also, be aware of the ’round half to even’ strategy Python uses to avoid bias in rounding.
Exploring Alternatives to Python’s Round Function
While Python’s built-in round
function is quite handy, it’s not the only way to round numbers in Python. There are alternative methods, like using the math
library, that can also be effective. Let’s explore these alternatives.
Rounding using the Math Library
Python’s math
library provides two functions – math.floor
and math.ceil
– that can be used for rounding numbers. math.floor
rounds a number down to the nearest integer, while math.ceil
rounds a number up to the nearest integer. Here’s how you can use these functions:
import math
num = 3.14159
print(math.floor(num))
print(math.ceil(num))
# Output:
# 3
# 4
In this example, math.floor(num)
returns 3
, which is the largest integer less than or equal to num
. On the other hand, math.ceil(num)
returns 4
, which is the smallest integer greater than or equal to num
.
These methods can be particularly useful when you want to round a number up or down to the nearest integer, regardless of its decimal part.
However, one limitation of math.floor
and math.ceil
is that they don’t allow you to specify the number of decimal places for rounding. They only round to the nearest integer.
In conclusion, while Python’s built-in round
function is a versatile tool for rounding numbers to a specified number of decimal places, alternative methods like math.floor
and math.ceil
can be more suitable for certain scenarios. As always, the best method depends on your specific needs and the nature of your data.
While Python’s round
function is generally straightforward, there are certain scenarios that might yield unexpected results. Understanding these can help you troubleshoot and find effective workarounds.
Rounding Halfway Numbers
One common issue arises from Python’s ’round half to even’ strategy, also known as ‘bankers’ rounding’. This strategy can produce results that are counterintuitive to those used to traditional rounding methods. Here’s an example:
print(round(2.5))
print(round(3.5))
# Output:
# 2
# 4
In this case, while 2.5
rounds down to 2
, 3.5
rounds up to 4
. This is because Python rounds to the nearest even number when a number is exactly halfway between two others. To mitigate this, you might choose to add a small bias to the number before rounding.
Rounding Very Large Numbers
Another issue is that Python’s round
function may not round very large numbers as expected. This is due to the limitations of floating point precision. For example:
num = 1e20 + 0.1
print(round(num) - 1e20)
# Output:
# 0.0
Here, 1e20 + 0.1
should be 1e20 + 0.1
, but due to the limitations of floating point precision, the 0.1
is lost when rounding. This is something to be aware of when dealing with very large numbers.
Rounding Negative Numbers
Finally, when rounding negative numbers, remember that Python’s round
function rounds towards the nearest even number, not towards zero. This means that round(-1.5)
will return -2
, not -1
as it might in other rounding methods.
print(round(-1.5))
# Output:
# -2
As a tip, always verify your results when dealing with negative numbers, especially when they’re halfway between two others.
In conclusion, while Python’s round
function is a powerful tool, it’s important to understand its nuances to avoid unexpected results.
Python Number Data Types and Rounding: The Foundations
To fully grasp how Python’s round
function works, it’s crucial to understand Python’s number data types and the concept of rounding.
Python has three numeric data types: integers (int
), floating point numbers (float
), and complex numbers (complex
). The round
function is typically used with float
numbers, which are numbers with a decimal point.
integer_num = 10
float_num = 10.0
complex_num = 10 + 0j
print(type(integer_num))
print(type(float_num))
print(type(complex_num))
# Output:
# <class 'int'>
# <class 'float'>
# <class 'complex'>
In this example, we have three variables, each representing one of Python’s numeric data types. The type
function returns the data type of each variable. As you can see, 10
is an integer (int
), 10.0
is a floating point number (float
), and 10 + 0j
is a complex number (complex
).
Now, let’s talk about rounding. Rounding is a process that reduces the number of digits in a number while keeping its value close to what it was. The result is a number that is easier to work with and present. For example, the number 3.14159
rounded to two decimal places would be 3.14
.
In Python, the round
function is used to round a number to the nearest value, based on the specified number of decimal places. This is particularly useful when you need to reduce the precision of a floating point number for easier readability or to meet certain data storage requirements.
Understanding these fundamental concepts will help you make the most of Python’s round
function and its applications.
The Impact of Rounding in Data Analysis and Scientific Computing
The round
function in Python isn’t just a neat tool for making numbers more manageable; it plays a significant role in fields like data analysis and scientific computing. In these domains, data is often represented as floating point numbers, and rounding can help manage this data more effectively.
For instance, in data analysis, you might need to round your data to a certain number of decimal places to make it easier to visualize or compare. Similarly, in scientific computing, rounding can help reduce the computational complexity of your calculations, making your programs run more efficiently.
Beyond the round
function, Python offers a wealth of mathematical functions and concepts that are worth exploring. For instance, understanding floating point precision can help you avoid common pitfalls when working with floating point numbers. Python’s math
library offers a wide array of mathematical functions that can assist in more complex calculations.
Here’s an example of how rounding can be used in data analysis. Suppose you have a list of floating point numbers representing some measurements, and you want to round these numbers to two decimal places for easier comparison:
measurements = [3.14159, 2.71828, 1.41421]
rounded_measurements = [round(num, 2) for num in measurements]
print(rounded_measurements)
# Output:
# [3.14, 2.72, 1.41]
In this example, we use a list comprehension to round each number in the measurements
list to two decimal places. The result is a new list, rounded_measurements
, which is easier to work with.
Further Resources for Python Functions
For those looking to delve deeper into Python’s mathematical capabilities and more, consider the following resources:
- Python Built-In Functions Efficiency Techniques – Learn about built-in functions that enhance your debugging and profiling capabilities.
Sequence Generation with range() in Python – Learn how to efficiently work with numeric ranges using Python’s “range.”
Simplifying Data Union with Python’s union() – Dive into set operations, deduplication, and merging in Python.
Essential Python Built-In Functions for Data Science – A comprehensive guide highlighting key built-in Python functions for data science.
Python’s Official Documentation on Math Module – Delve into the functionalities of Python’s math module.
Python’s Official Documentation on Built-In Functions – Understand Python’s built-in functions and their usages in depth.
Wrapping Up: Python’s Round Function Demystified
In this guide, we’ve journeyed through the intricacies of Python’s round
function, from its basic usage to more advanced applications. We’ve demystified how Python rounds numbers, shedding light on its ’round half to even’ strategy and how it handles large and negative numbers.
We’ve also explored alternative methods for rounding numbers in Python, such as using the math.floor
and math.ceil
functions from Python’s math
library. While these methods lack the precision of the round
function, they can be useful in scenarios where rounding up or down to the nearest integer is required.
Here’s a quick comparison of the methods we’ve discussed:
Method | Description | Precision |
---|---|---|
round | Python’s built-in function for rounding numbers. | Can specify number of decimal places. |
math.floor | Rounds a number down to the nearest integer. | Rounds to the nearest integer only. |
math.ceil | Rounds a number up to the nearest integer. | Rounds to the nearest integer only. |
Remember, the best method depends on your specific needs and the nature of your data. Always verify your results, especially when dealing with halfway numbers, very large numbers, or negative numbers.
With this knowledge, you’re now better equipped to handle rounding in Python effectively and efficiently. Happy coding!