Python Subprocess Module Guide (With Examples)
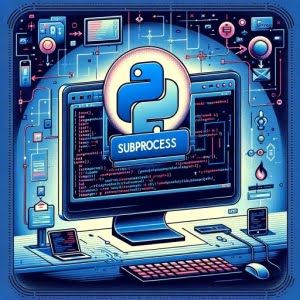
Ever felt like you’re wrestling with your Python script just to run shell commands? You’re not alone. Python’s subprocess module, akin to a skilled conductor, is here to orchestrate your system’s processes and command lines directly from your Python code.
This guide will walk you through the ins and outs of using the subprocess module, transforming you from a novice to a pro. We’ll explore everything from basic usage to advanced techniques, ensuring you have a comprehensive understanding of this powerful tool.
So, let’s dive in and start taming those shell commands with Python subprocess.
TL;DR: How do I use the Python subprocess module?
The Python subprocess module is a powerful tool that allows you to spawn new processes, connect to their input/output/error pipes, and obtain their return codes. Here’s a straightforward example:
import subprocess
subprocess.run(['ls', '-l'])
# Output:
# total 0
# drwxr-xr-x 2 anton staff 64 Dec 12 12:34 Documents
# drwxr-xr-x 5 anton staff 160 Dec 12 12:34 Downloads
This example runs the ‘ls -l’ command and prints the output, listing the files in the current directory along with their details. It’s a simple yet powerful illustration of what the Python subprocess module can do. But don’t stop here – there’s so much more to learn! Continue reading for a deep dive into the subprocess module, complete with more detailed information and advanced usage examples.
Table of Contents
- Unleashing Python Subprocess for Beginners
- Mastering Input/Output with Python Subprocess
- Exploring Alternative Ways to Run Shell Commands
- Navigating Common Pitfalls in Python Subprocess
- Understanding Subprocess: Processes, Threads, and Shell Scripting
- Python Subprocess in Real-World Applications
- Further Resources for Python Modules
- Python Subprocess: A Recap
Unleashing Python Subprocess for Beginners
Subprocess module’s run()
function is your starting point in the journey of executing shell commands from Python. It’s simple to use and incredibly powerful. Let’s see it in action with a basic example:
import subprocess
subprocess.run(['echo', 'Hello, World!'])
# Output:
# Hello, World!
In this example, we’re using the run()
function to execute the ‘echo’ command, which simply prints ‘Hello, World!’ to the console. The command and its arguments are passed as a list to run()
. The function then spawns a new process to execute this command and waits for it to finish. The output of the command is printed directly to the console.
The run()
function is a high-level function recommended for basic use due to its simplicity. However, it’s worth noting that it doesn’t give you the output of the command. For that, we’ll need to dive a bit deeper into the subprocess module.
While run()
is a powerful ally, it’s not without its pitfalls. For one, it can raise a CalledProcessError
if the command it’s trying to execute fails. It’s important to be aware of this and handle such exceptions in your code to prevent your Python script from crashing.
In the upcoming sections, we’ll delve deeper into the subprocess module and explore more complex usage scenarios. So, stay tuned!
Mastering Input/Output with Python Subprocess
As you grow more comfortable with the basics of the subprocess module, you’ll soon find yourself craving more control over the processes you spawn. Specifically, you’ll want to handle their input and output directly from your Python script. This is where the Popen
class comes into play.
Consider the following example:
import subprocess
process = subprocess.Popen(['echo', 'Hello, World!'], stdout=subprocess.PIPE)
output, error = process.communicate()
print(output.decode())
# Output:
# Hello, World!
In this code, we’re using the Popen
class instead of the run()
function. Popen
gives us much more control over the process we’re spawning. In this case, we’re redirecting the process’s output to a pipe, which we can then read from our Python script.
The communicate()
method is used to send and receive data to/from the process. It returns a tuple containing the process’s output and error messages, if any. Here, we’re only interested in the output, which we then print to the console.
The Popen
class also allows you to manage the lifecycle of the process, giving you the ability to terminate it or wait for it to finish.
While Popen
provides more control, it also comes with its own set of challenges. For example, if you’re not careful, you can run into deadlocks when using pipes. In the next section, we’ll discuss some common issues you might encounter and how to handle them.
Exploring Alternative Ways to Run Shell Commands
While the subprocess module is a powerful tool, Python provides other ways to run shell commands. Let’s explore two of them: os.system()
and os.popen()
.
Using os.system()
First up is os.system()
. Here’s a basic usage example:
import os
os.system('echo Hello, World!')
# Output:
# Hello, World!
The os.system()
function takes a string containing the command you want to run and executes it. It’s simple to use, but it has its limitations. For instance, it doesn’t allow you to capture the command’s output. Instead, it returns the command’s exit status.
Using os.popen()
Next, we have os.popen()
. Here’s how you can use it:
import os
output = os.popen('echo Hello, World!').read()
print(output)
# Output:
# Hello, World!
The os.popen()
function also takes a string containing the command to run. However, it provides a file-like object from which you can read the command’s output. This makes it more flexible than os.system()
.
While these alternatives can be useful in certain situations, they are generally considered less powerful and less flexible than the subprocess module. For example, they don’t allow you to handle input and output as efficiently as subprocess does, and they don’t provide as much control over the spawned processes.
When deciding which tool to use, consider the complexity of the task at hand. For simple tasks, os.system()
or os.popen()
might suffice. But for more complex tasks, especially those requiring tight control over input and output, the subprocess module is usually the way to go.
Like any powerful tool, the Python subprocess module comes with its own set of challenges. Being aware of these common issues can save you a lot of headaches down the road.
Handling Errors
One common issue is handling errors. When a subprocess call fails, it raises a CalledProcessError
. Here’s an example:
import subprocess
try:
subprocess.run(['ls', '/nonexistent_directory'], check=True)
except subprocess.CalledProcessError as e:
print(f'Error: {e}')
# Output:
# Error: Command '['ls', '/nonexistent_directory']' returned non-zero exit status 1.
In this code, we’re trying to list the contents of a directory that doesn’t exist. This causes the ls
command to fail, raising a CalledProcessError
. We catch this error and print a message to the console.
Dealing with Blocking I/O
Another common issue is dealing with blocking I/O. If a subprocess produces a lot of output, it can fill up the pipe and block until the program reads from it. To avoid this, you can use the Popen
class with the communicate()
method, as we discussed in the ‘Advanced Use’ section.
Managing Subprocesses on Different Platforms
Finally, remember that subprocess behavior can vary between different platforms. For instance, on Windows, you can’t use single quotes to enclose command-line arguments. Instead, you have to use double quotes. Be aware of these differences when writing cross-platform scripts.
Understanding these common issues and how to handle them will make you a more effective Python subprocess user. Remember, every challenge is an opportunity to learn and grow!
Understanding Subprocess: Processes, Threads, and Shell Scripting
To truly harness the power of the Python subprocess module, it’s important to understand the fundamentals it’s built upon: processes, threads, and shell scripting.
What are Processes and Threads?
In computing, a process is an instance of a computer program that is being executed. It contains the program code and its current activity. Each process usually has multiple ‘threads’ of execution, which are essentially smaller sequences of programmed instructions that can be managed independently by a scheduler.
What is Shell Scripting?
Shell scripting, on the other hand, is a way of giving commands directly to the operating system. In a shell script, you can run multiple commands in sequence and automate repetitive tasks.
Why Use Python Subprocess?
The Python subprocess module allows you to spawn new processes, connect to their input/output/error pipes, and obtain their return codes. This means you can run shell commands directly from your Python script and manage their input and output.
Let’s take a look at a simple example:
import subprocess
subprocess.run(['echo', 'Hello, Subprocess!'])
# Output:
# Hello, Subprocess!
In this code, we’re using the run()
function from the subprocess module to execute the echo
command, which prints ‘Hello, Subprocess!’ to the console.
But why use subprocess instead of other alternatives like os.system()
or os.popen()
? The subprocess module provides more flexibility and control over your shell commands. It allows you to handle input and output more efficiently, manage the lifecycle of your processes, and work with pipes and redirects.
In the end, the choice between subprocess and its alternatives depends on your specific needs. For simple tasks, os.system()
or os.popen()
might suffice. But for more complex tasks requiring fine-grained control over your shell commands, subprocess is the way to go.
Python Subprocess in Real-World Applications
The Python subprocess module isn’t just a fascinating subject for computer science enthusiasts. It has practical, real-world applications that make it an invaluable tool for many professionals.
Automation with Subprocess
Automation is one area where subprocess truly shines. Whether you’re automating data backups, system updates, or repetitive tasks, subprocess allows your Python script to interact with the system shell and automate commands.
import subprocess
subprocess.run(['tar', '-czf', 'backup.tar.gz', '/path/to/directory'])
# Output:
# No output on success
In this example, we’re using subprocess to automate a data backup task. The tar
command is used to create a compressed archive of a directory.
Data Processing and System Administration
Subprocess is also a powerful ally in data processing and system administration. It allows you to execute shell commands that manipulate data, manage system processes, and more.
import subprocess
output = subprocess.run(['ps', 'aux'], stdout=subprocess.PIPE).stdout.decode()
print(output)
# Output:
# USER PID %CPU %MEM VSZ RSS TTY STAT START TIME COMMAND
# root 1 0.0 0.2 225848 9440 ? Ss Feb24 0:06 /sbin/init
In this code, we’re using subprocess to run the ps aux
command, which lists currently running processes. This can be useful in system administration for monitoring system activity.
Further Resources for Python Modules
If you’re interested in diving deeper into this subject, here are several related topics that you might find interesting:
- Python Modules: Your Coding Allies – Discover modules for machine learning and artificial intelligence.
Python Random Number Generators – Learn about random number generation techniques in Python.
Simplifying UUID Generation in Python – Learn how to ensure uniqueness and identify objects with UUIDs in Python.
Execute System Commands with Python – DigitalOcean’s guide to executing system commands in Python using ‘os’ and ‘subprocess’.
Assigning Shell Command Output to Python Variable – Lean how to assign the output of a shell command to a Python variable in this Medium article.
Executing Shell Command in Python – JavaTpoint’s tutorial on how to execute shell command in Python.
Subprocess is a powerful, versatile module that can make your Python scripts more effective and efficient. So, why not start exploring what it can do for you?
Python Subprocess: A Recap
The Python subprocess module is a powerful tool for executing shell commands directly from your Python script. It provides methods like run()
and Popen()
to spawn new processes, connect to their input/output/error pipes, and obtain their return codes.
While run()
is simple and easy to use, Popen()
offers more control over the spawned processes. It allows you to manage their lifecycle, handle their input and output, and work with pipes and redirects.
Python also offers alternative methods for running shell commands, such as os.system()
and os.popen()
. However, these are generally considered less flexible and less powerful than subprocess.
Despite its power, subprocess comes with its own set of challenges. These include handling errors, dealing with blocking I/O, and managing subprocesses on different platforms. But with a good understanding of the module and its fundamentals, you can navigate these challenges and harness the power of subprocess in your Python scripts.
In conclusion, whether you’re automating tasks, processing data, or managing system processes, the Python subprocess module is a powerful ally. It’s a testament to the versatility and power of Python, and a tool every Pythonista should have in their toolbox.