NPM Install Specific Version | Step-by-Step Guide
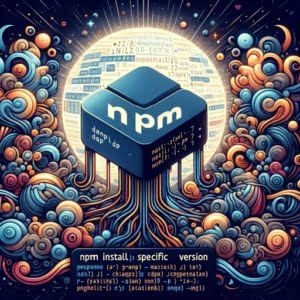
At IOFLOOD, we’ve often found ourselves needing to install specific versions of npm packages to ensure compatibility with our projects. To address this, we’ve crafted a guide detailing the steps to install a specific version of a package using npm. By following our simple instructions, you’ll gain the ability to precisely control the versions of packages in your projects, avoiding compatibility issues.
Like a meticulous librarian who organizes books by editions, npm (Node Package Manager) empowers you to install specific versions of packages with unparalleled precision. This guide will walk you through the steps to specify package versions using npm, ensuring your projects remain consistent and stable. By mastering npm’s versioning capabilities, you can prevent unforeseen conflicts and maintain the integrity of your projects.
Let’s simplify the process of installing specific package versions together and enhance the stability of our projects!
TL;DR: How Do I Install a Specific Version of a Package Using npm?
To install a specific version of a package using npm, you can use the command
npm install @
. For example, to install version 1.2.3 of lodash, you would execute:
npm install [email protected]
# Output:
# + [email protected]
In this example, the npm install
command is used to specify the version 1.2.3 of the lodash package. This ensures that your project uses the exact version required, maintaining consistency and preventing potential conflicts with other versions.
Dive deeper into this guide for more details and advanced scenarios for managing package versions with npm.
Table of Contents
Basic Use: NPM Version Control
When you’re starting with Node.js, understanding how to manage package versions with npm can feel like learning a new language. But it’s a critical skill that ensures your project remains stable and consistent. Let’s dive into the basic use of the npm install @
command with a straightforward example.
Installing a Specific Package Version
Imagine you’re working on a project that requires version 2.0.0 of the express
package. To install this specific version, you would run the following command:
npm install [email protected]
# Output:
# + [email protected]
In this code block, we’ve specified the version 2.0.0 of the express
package directly in the command. This action tells npm to fetch and install exactly this version, ensuring your project uses the version it was designed to work with. This precision is crucial for maintaining project consistency across different development environments and among team members.
The Importance of Specific Versions
Specifying package versions is like ensuring all team members are reading from the same book edition. It prevents the ‘it works on my machine’ syndrome by standardizing the development environment. Precise version control is vital for project stability, especially when working on larger projects or in teams.
Pros and Cons of Precise Version Control
Pros:
– Ensures consistency across development environments.
– Prevents compatibility issues between different package versions.
– Facilitates easier debugging and maintenance.
Cons:
– Requires regular updates to keep packages in sync with the latest versions.
– May miss out on important security patches or features introduced in newer versions.
Understanding the balance between maintaining project stability and keeping packages up-to-date is essential. While it’s important to specify versions for critical dependencies, it’s also beneficial to review and update these dependencies regularly to take advantage of improvements and security patches.
Advanced npm Versioning Techniques
As you become more comfortable with npm and package management, you’ll discover that specifying exact versions is just the beginning. npm offers powerful features for managing dependency versions more flexibly, such as version ranges, and the use of tilde (~) and caret (^) prefixes. These tools allow you to balance the need for stability with the desire for easy updates.
Utilizing Version Ranges
Version ranges let you specify a range of versions that you’re willing to use, rather than a single, specific version. This can be particularly useful for libraries that follow semantic versioning (SemVer) and are diligent about not introducing breaking changes in minor or patch versions.
For example, if you want to install any version of the moment
package that is compatible with version 2.8.x, you might use:
npm install moment@"2.8.*"
# Output:
# + [email protected]
This command tells npm to install the latest patch version of moment
that matches the 2.8 minor version. It’s a way to ensure you get bug fixes and minor improvements without risking major changes that could break your project.
The Tilde (~) and Caret (^) Prefixes
The tilde (~) and caret (^) are shorthand for expressing version ranges in npm. The tilde (~) prefix is used to allow changes that do not modify the leftmost non-zero digit in the version, typically used for allowing patch-level changes. The caret (^), however, allows changes that do not modify the leftmost non-zero digit, up to minor level changes.
For instance, using the caret (^) to install a package might look like this:
npm install lodash@"^4.17.0"
# Output:
# + [email protected]
By using ^4.17.0
, you’re indicating that you’re open to installing any 4.x.x version of lodash that is later than or equal to 4.17.0, but not a major version change that could introduce breaking changes. This approach offers a balance between avoiding potential bugs in new major versions and still benefiting from new features and bug fixes in minor or patch versions.
Balancing Flexibility and Stability
Choosing between specifying exact versions, version ranges, or using tilde (~) and caret (^) prefixes depends on your project’s needs for stability and flexibility. While exact versions offer the most stability, they can also make updates more cumbersome. Version ranges and prefixes, on the other hand, provide a middle ground, allowing for easier updates while still avoiding major changes that could disrupt your project.
Understanding how to use these advanced features of npm effectively can help you manage your project’s dependencies more efficiently, ensuring that your project remains both stable and up-to-date.
Alternative Package Management
While npm install @
is a powerful command for managing package versions, the Node.js ecosystem offers alternative tools and approaches that cater to different project needs. Understanding these alternatives can enhance your version management strategy.
npm-shrinkwrap vs package-lock.json
npm-shrinkwrap.json
and package-lock.json
are two files that can help you lock down your project’s dependencies to specific versions, but they serve slightly different purposes.
npm-shrinkwrap.json
is created using thenpm shrinkwrap
command. It’s intended for production builds, ensuring that not only your direct dependencies but also the sub-dependencies are locked to specific versions.
npm shrinkwrap
# Output:
# Created npm-shrinkwrap.json
This command generates an npm-shrinkwrap.json
file, similar to package-lock.json
, but it is intended for sharing in different environments to ensure consistency.
package-lock.json
is automatically generated when installing packages with npm for the first time in a project. It aims to lock dependency versions for development purposes, ensuring that all developers working on the project have the same versions of dependencies.
Yarn: An Alternative to npm
Yarn is another popular package manager that can be used as an alternative to npm. It offers faster dependency resolution and more reliable installations through a detailed yarn.lock
file, which locks down versions of packages and their dependencies.
yarn add [email protected]
# Output:
# success Saved 1 new dependency.
# info Direct dependencies
# └─ [email protected]
# info All dependencies
# └─ [email protected]
In this example, using Yarn to add lodash version 4.17.15 to a project, the yarn.lock
file is updated to reflect this specific version, ensuring that all project contributors install the exact same version.
Comparing Approaches
Each of these tools and approaches offers benefits and drawbacks:
- npm-shrinkwrap and package-lock.json provide a high level of precision in version control, making them ideal for projects where consistency across environments is critical. However, managing these files can become cumbersome in large projects with many dependencies.
Yarn is praised for its speed and reliability, with the
yarn.lock
file offering a detailed snapshot of your project’s dependencies. While it’s a robust alternative to npm, it requires team members to adopt a new tool, which might not always be feasible.
Understanding these alternatives allows you to choose the best tool for your project’s needs, whether you prioritize speed, reliability, or consistency across development environments.
Even with a solid understanding of npm and its versioning capabilities, you might encounter issues such as installing incompatible package versions or resolving dependency conflicts. Let’s explore some common challenges and how to address them.
Incompatible Package Versions
One of the most common issues is attempting to install a package version that’s incompatible with your project’s current setup. This can lead to runtime errors or failed installations. To illustrate, let’s try installing a version of a package that requires a different Node.js version:
npm install some-package@latest
# Output:
# npm ERR! code ENOTSUP
# npm ERR! notsup Unsupported engine for [email protected]: wanted: {"node":">=14.0.0"} (current: {"node":"10.0.0"})
# npm ERR! notsup Not compatible with your version of node/npm: [email protected]
This error message indicates that the version of some-package
we’re trying to install requires Node.js version 14.0.0 or higher, but our current version is 10.0.0. To resolve this, you would need to update your Node.js to a compatible version or choose a different package version that matches your current environment.
Resolving Dependency Conflicts
Dependency conflicts can occur when two or more packages require different versions of the same dependency. npm tries to resolve these automatically, but sometimes manual intervention is needed. For example, if you have two dependencies that require different versions of a third dependency, you might use the npm ls
command to identify the conflict:
npm ls conflicted-package
# Output:
# project-name@version
# ├─┬ [email protected]
# │ └── [email protected]
# └─┬ [email protected]
# └── [email protected] incompatible
The output shows which versions of conflicted-package
are installed by each dependency, highlighting the incompatibility. To resolve this, you might need to upgrade one of the conflicting dependencies to a version that relies on a compatible version of conflicted-package
, or explore alternative packages.
Major Version Changes Impact
Major version changes in packages often include breaking changes. When a package you depend on releases a new major version, it’s crucial to review the release notes and test the update in a separate branch or environment before updating the dependency in your main project. This precaution helps prevent unexpected issues from arising due to breaking changes.
Understanding how to troubleshoot these common npm versioning issues can save you time and frustration. By applying best practices such as keeping your Node.js and npm versions up to date, regularly reviewing your project’s dependencies, and testing major updates in isolation, you can maintain a stable and efficient development environment.
Utilizing Semantic Versioning (SemVer)
At the heart of npm’s package management capabilities lies Semantic Versioning or SemVer. It’s a set of rules that software publishers follow to communicate the nature of changes in their releases. SemVer is formatted as MAJOR.MINOR.PATCH
, where:
MAJOR
version changes involve incompatible API changes,MINOR
version changes add functionality in a backwards-compatible manner, andPATCH
version changes are for backwards-compatible bug fixes.
This system is crucial for maintaining project stability as it helps developers understand the impact of updating a package without needing to dive into the code.
npm and SemVer
npm heavily relies on SemVer to manage package versions. When you run an npm install
command without specifying a version, npm installs the latest version that matches the version range specified in your package.json
file. This behavior is governed by SemVer rules. For example, if your project depends on a package foo
with the version specified as ^1.0.0
, npm will install the latest 1.x.x
version available but will not upgrade to 2.0.0
because that could contain breaking changes.
npm install foo@^1.0.0
# Output:
# + [email protected]
In this code block, npm resolved the caret (^) version range ^1.0.0
to the latest minor version 1.8.2
that’s compatible with 1.0.0
, adhering to the SemVer rules. This ensures that your project benefits from the latest features and bug fixes within the 1.x.x
range without risking major changes that could break your application.
The Importance of Specific Versioning
Specifying versions when installing npm packages allows developers to control the exact versions used in a project, ensuring consistency across development environments and among team members. This practice prevents the ‘it works on my machine’ problem and is fundamental for continuous integration/continuous deployment (CI/CD) pipelines, where automated processes require predictable outcomes.
The npm versioning philosophy emphasizes the balance between flexibility in updating packages and the stability required for reliable software development. By understanding and utilizing SemVer and npm’s versioning tools, developers can maintain this balance, ensuring that their projects are both stable and up-to-date.
Automation & CI/CD with NPM
The ability to install specific versions of npm packages isn’t just a convenience—it’s a cornerstone of modern software development practices, particularly in Continuous Integration/Continuous Deployment (CI/CD) pipelines and Docker containers. These environments thrive on predictability and consistency, necessitating precise control over the versions of packages being used.
CI/CD Pipelines and Specific Versioning
In CI/CD pipelines, every code commit triggers an automated process that builds, tests, and deploys your application. Specifying exact versions of npm packages ensures that these processes are repeatable and consistent across different stages of deployment, from development to production. For example, specifying a package version in your package.json
can prevent unexpected updates from causing issues in your build:
"dependencies": {
"react": "16.13.1"
}
# Output:
# Ensures the CI/CD pipeline uses React version 16.13.1 consistently
This code snippet from a package.json
file illustrates how specifying the version of React as 16.13.1
guarantees that this exact version is used throughout the pipeline, eliminating the risk of discrepancies between environments.
Docker Containers and Version Control
Docker containers encapsulate your application and its environment. Using npm to install specific versions of packages when building your Docker images can ensure that the container runs the same way, every time, regardless of where it’s deployed. For instance, installing a specific version of a package in a Dockerfile ensures consistency:
RUN npm install [email protected]
# Output:
# Installs Express version 4.17.1 in the Docker container
This Dockerfile command explicitly installs Express version 4.17.1, ensuring that all instances of the container use the same version of Express, thereby enhancing reliability and predictability in automated environments.
Further Resources for NPM Version Mastery
To deepen your understanding of npm and specific version installation, here are three excellent resources:
- NPM Documentation – Official guide on npm commands, including version management.
Node.js Best Practices – Collection of best practices for Node.js development, including npm package management.
Understanding Semantic Versioning – An in-depth look at Semantic Versioning and its significance in software development.
These resources provide valuable insights into npm and its ecosystem, equipping you with the knowledge to manage package versions effectively in any project or automated environment.
Recap: Install Specific Version w/ npm
In this comprehensive guide, we’ve delved into the specifics of using npm to install particular versions of packages, a vital skill for maintaining the consistency and stability of your Node.js projects. Like a librarian carefully selecting editions, npm offers precision in package management that can significantly impact project success.
We began with the essentials, demonstrating how to use the npm install @
command to specify the exact version of a package your project requires. This foundational knowledge is crucial for every developer to ensure that everyone on the team is working with the same set of tools.
Moving to more advanced territory, we explored npm’s features like version ranges, and the significance of tilde (~) and caret (^) prefixes in managing dependency versions more flexibly. These features allow developers to balance the need for stability with the benefits of receiving critical updates.
We also discussed alternative tools and approaches for version management, such as npm-shrinkwrap, package-lock.json, and yarn. Each offers distinct advantages and challenges, enabling teams to choose the best fit for their project’s needs.
Approach | Flexibility | Stability | Complexity |
---|---|---|---|
npm install | Low | High | Low |
Version Ranges (~, ^) | Moderate | Moderate | Moderate |
npm-shrinkwrap/package-lock.json | High | High | High |
Yarn | Moderate | High | Moderate |
As we wrap up, it’s clear that mastering npm and understanding how to specify package versions are essential skills for modern developers. These capabilities ensure that your projects are reliable, consistent, and up-to-date, laying the groundwork for successful development and deployment.
Whether you’re just starting out or looking to refine your npm skills, this guide serves as a valuable resource. We encourage you to continue exploring npm and package management through further reading and practice. With a solid grasp of these concepts, you’re well-equipped to tackle any project with confidence.