Convert a String to a Float in Python: 3 Easy Methods
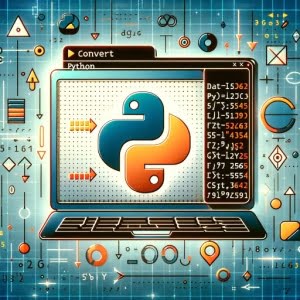
Are you wrestling with the task of converting strings to floats in Python? Python, akin to a proficient mathematician, is fully equipped to convert strings into floating-point numbers effortlessly.
This comprehensive guide will walk you through the process, introducing you to both basic and advanced techniques. By the end of this article, you’ll be able to handle this common Python task with confidence and ease.
So, let’s dive into to String conversion in Python!
TL;DR: How Do I Convert a Python String to Float?
You can convert a string to a float in Python using the
float()
function and the syntax,number = float(data)
.
Here’s a quick example to illustrate this:
data = '123.45'
number = float(data)
print(number)
# Output:
# 123.45
In this example, we have a string '123.45'
that we want to convert into a float. By passing this string into the float()
function, Python returns the floating-point number 123.45
. We then print the result to confirm our conversion.
If you’re interested in diving deeper into the topic, understanding potential pitfalls and learning about more advanced scenarios, we encourage you to continue reading.
Table of Contents
The Basics: Strings to Floats
The float()
function in Python is a built-in function that converts a number or a string to a floating-point number. Let’s break down how it works with strings.
data = '456.78'
number = float(data)
print(number)
# Output:
# 456.78
In the above example, the string '456.78'
is passed into the float()
function. Python then converts this string into the floating-point number 456.78
. The result is printed to confirm our conversion.
The float()
function is straightforward and easy to use, making it the go-to method for converting strings to floats in Python. However, it’s important to be aware of its limitations.
If you try to convert a string that doesn’t represent a valid floating-point number, Python will throw a
ValueError
.
Example:
data = 'Hello, World!'
number = float(data)
# Output:
# ValueError: could not convert string to float: 'Hello, World!'
In this case, the string 'Hello, World!'
is not a valid floating-point number, causing Python to raise a ValueError
.
When using the
float()
function, it’s crucial to ensure that the string you’re trying to convert is a valid representation of a floating-point number.
Handling Exceptions with Conversion
As we progress in Python, we often encounter strings that may not directly convert into floats using the float()
function. This is where error handling comes into play.
Try-Except
We can use a try-except
block to handle potential ValueError
exceptions that might arise when a string cannot be converted to a float.
data = 'Hello, World!'
try:
number = float(data)
except ValueError:
print('Cannot convert string to float')
# Output:
# Cannot convert string to float
In this example, we attempt to convert the string 'Hello, World!'
to a float. Since this string does not represent a valid floating-point number, a ValueError
is raised. However, our try-except
block catches this error and prints a custom error message instead.
Localized Formats
In addition to handling exceptions, it’s also important to consider different formats when converting strings to floats.
For instance, some locales use a comma as a decimal separator instead of a period. In such cases, we can replace the comma with a period before converting the string.
data = '123,45'
data = data.replace(',', '.')
number = float(data)
print(number)
# Output:
# 123.45
In this example, we replace the comma in the string '123,45'
with a period, resulting in the string '123.45'
. We can then convert this string to a float using the float()
function.
By understanding how to handle exceptions and different formats, you can convert strings to floats in Python more effectively and avoid common pitfalls.
Alternative Conversion Methods
While the float()
function is the most straightforward way to convert strings to floats in Python, there are alternative methods that you can use, especially when dealing with more complex scenarios or larger datasets.
Let’s explore a few of these alternatives.
Using Pandas Library
The Pandas library is a powerful tool for data manipulation in Python and can be used to convert strings to floats across an entire DataFrame or Series.
import pandas as pd
data = pd.Series(['1.23', '4.56', '7.89'])
numbers = pd.to_numeric(data)
print(numbers)
# Output:
# 0 1.23
# 1 4.56
# 2 7.89
# dtype: float64
In this example, we create a Pandas Series with strings representing floating-point numbers. We then use the pd.to_numeric()
function to convert these strings to floats.
This function is particularly useful when dealing with large datasets, as it can convert all strings to floats in one go.
The downside of using Pandas is that it’s a heavy library and might be overkill for simple tasks. It also requires you to handle NaN values and decide how to deal with strings that cannot be converted to floats.
Using Numpy Library
Numpy is another popular library in Python that can be used for numerical operations. The numpy.float()
function can be used to convert strings to floats.
import numpy as np
data = '123.45'
number = np.float(data)
print(number)
# Output:
# 123.45
In this example, we use the numpy.float()
function to convert the string '123.45'
to a float.
Numpy is a lightweight library compared to Pandas and is a good choice for numerical computations. However, like the float()
function, it will raise a ValueError
if the string cannot be converted to a float.
While the
float()
function is the most direct way to convert strings to floats in Python, libraries like Pandas and Numpy offer alternative methods that might be more suitable depending on your specific needs and the complexity of your data.
Solving Errors within Conversions
When converting strings to floats in Python, you might encounter a few common issues. Let’s discuss these problems and their solutions, and delve into some practical examples.
Handling ‘ValueError’
One of the most common errors you might come across is the ValueError
. This error is raised when you try to convert a string that doesn’t represent a valid floating-point number.
data = 'Hello, Python!'
try:
number = float(data)
except ValueError:
print('Cannot convert string to float')
# Output:
# Cannot convert string to float
In this example, we tried to convert the string 'Hello, Python!'
to a float, which resulted in a ValueError
. To handle this error, we used a try-except
block to catch the ValueError
and print a custom error message.
Dealing with Different Formats
Another issue you might face is dealing with strings in different formats. For instance, some locales use a comma as a decimal separator instead of a period. In such cases, you can replace the comma with a period before converting the string.
data = '123,45'
data = data.replace(',', '.')
number = float(data)
print(number)
# Output:
# 123.45
In this example, we replaced the comma in the string '123,45'
with a period, resulting in the string '123.45'
. We could then convert this string to a float using the float()
function.
By understanding these common issues and their solutions, you can effectively convert strings to floats in Python and avoid potential pitfalls.
Explained: String and Float Data Types
To fully grasp the process of converting strings to floats in Python, it’s essential to understand the fundamental concepts of strings and floats.
Python Strings
In Python, a string is a sequence of characters. It is an immutable data type, which means that once a string is created, it cannot be changed.
Strings in Python can be created by enclosing characters in quotes. Python treats single quotes the same as double quotes.
data = '123.45'
print(type(data))
# Output:
# <class 'str'>
In the above code, '123.45'
is a string. We can confirm this by using the type()
function, which returns <class 'str'>
, indicating that the data type is a string.
Python Floats
A float, or floating-point number, is a number with a decimal point. Floats are used in Python to represent numbers that aren’t integers. The float()
function in Python converts a specified value into a floating-point number.
number = float('123.45')
print(type(number))
# Output:
# <class 'float'>
In this example, we converted the string '123.45'
to a float using the float()
function. We can confirm the conversion by using the type()
function, which returns <class 'float'>
, indicating that the data type is a float.
Practical Uses: Strings to Floats
The ability to convert strings to floats in Python extends beyond simple scripts or exercises. It plays a significant role in real-world applications, particularly in data processing and machine learning.
In data processing, datasets often contain numerical values represented as strings. Converting these strings to floats is a crucial step in preparing your data for analysis.
Similarly, in machine learning, you might need to convert strings to floats to feed your data into a model, as many machine learning algorithms require numerical input.
# A simple example in data processing
import pandas as pd
data = pd.DataFrame({'values': ['1.23', '4.56', '7.89']})
data['values'] = pd.to_numeric(data['values'])
print(data)
# Output:
# values
# 0 1.23
# 1 4.56
# 2 7.89
In this example, we have a DataFrame with a column of strings representing floating-point numbers. Using the pd.to_numeric()
function, we convert these strings to floats to prepare our data for analysis.
Further Resources for Python Strings
While this guide focused on converting strings to floats, Python offers a wide range of data type conversions. You might find it useful to explore related concepts such as converting strings to integers or lists to tuples. Additionally, understanding error handling in Python can help you write more robust code.
If you’re interested in learning more, here are a few resources that you might find helpful:
- Python Strings: Tips for Efficient Text Handling: Gain insights into efficient text handling with Python strings, learning tips and methods to improve your code’s performance.
Guide on Concatenating Strings in Python: This guide by IOFlood explores various techniques for concatenating strings in Python, including using the
+
operator, thestr.join()
method, and f-strings.Tutorial on Python Docstrings: This IOFlood tutorial focuses on Python docstrings, explaining what they are and how to use them effectively for documenting.
Python Tutorials: W3Schools provides beginner-friendly tutorials for learning Python.
Python Official Documentation: The official documentation is a comprehensive resource that covers all aspects of the Python programming language.
Python Convert String to Float: A Practical Guide: This practical guide on DigitalOcean explains how to convert a string to a float in Python, covering various scenarios and providing examples to illustrate the conversion process.
Recap: Convert Python Strings to Float
In this in-depth guide, we’ve explored the conversion of strings to floats in Python, a task that might seem simple at first but can present challenges in different contexts.
We’ve seen how the float()
function can be used to convert strings to floats and discussed potential pitfalls, such as the ValueError
that arises when trying to convert a string that doesn’t represent a valid floating-point number.
Beyond the float()
function, we’ve also discussed alternative approaches, such as using the Pandas and Numpy libraries. While these libraries can be more suitable for complex scenarios or large datasets, they come with their own considerations, such as handling NaN values in Pandas or the lightweight nature of Numpy.
Here’s a comparison of the methods we’ve discussed:
Method | Use Case | Considerations |
---|---|---|
float() | Simple conversions | Raises ValueError for invalid strings |
Pandas to_numeric() | Large datasets | Requires handling of NaN values |
Numpy float() | Numerical computations | Lightweight but raises ValueError for invalid strings |
The ability to convert strings to floats in Python is a fundamental skill that plays a significant role in data processing and machine learning. By understanding the basics, handling common issues, and exploring alternative approaches, you can effectively convert strings to floats in Python and apply this knowledge in your coding journey.
Remember to keep exploring, keep practicing, and keep coding!