Read a File Line by Line: Bash Shell Script File Handling
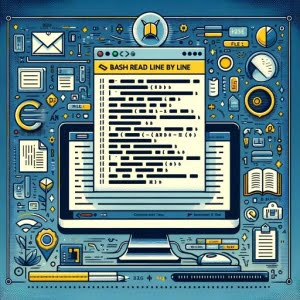
Are you struggling with reading a file line by line in Bash? You’re not alone. Many developers find this task a bit tricky, however Bash can handle files with finesse, reading through each line, making it an invaluable tool for many scripting tasks.
In this guide, we’ll walk you through the process of reading a file line by line in Bash, from the basics to more advanced techniques. We’ll cover everything from simple while loops for reading files, handling different types of file content, to troubleshooting common issues.
So, let’s dive in and start mastering Bash file reading!
TL;DR: How Do I Read a File Line by Line in Bash?
In Bash, you can use a
while
loop to read a file line by line. The syntax used for this is,while IFS= read -r line; do COMMAND; done < file.txt
. TheIFS
(Internal Field Separator) is set to nothing to prevent leading/trailing whitespace from being trimmed.
Here’s a simple example:
while IFS= read -r line
do
echo "$line"
done < file.txt
# Output:
# [Expected output from command]
In this example, we use a while loop to read a file named ‘file.txt’. The IFS
is set to nothing and -r
prevents backslash escapes from being interpreted. Each line is read into the variable line
, which is then echoed (printed) out.
This is a basic way to read a file line by line in Bash, but there’s much more to learn about file handling and processing in Bash. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
Bash Basics: Reading a File Line by Line
Let’s start with the basics. In Bash, one of the simplest ways to read a file line by line is by using a while loop. The while loop is a fundamental construct in Bash scripting, allowing for repetitive tasks to be performed.
Consider the following code block:
while IFS= read -r line
do
echo "Line: $line"
done < basic.txt
# Output:
# Line: [Each line from basic.txt will be printed here]
In this example, ‘basic.txt’ is our file. The IFS= read -r line
command reads each line of the file. The IFS=
part prevents leading/trailing whitespace from being trimmed, and -r
prevents backslash escapes from being interpreted. Each line is then stored in the line
variable and printed out prefixed with ‘Line: ‘.
This basic use of a while loop is straightforward, but it’s also powerful. It allows us to process each line individually, which is useful in many scripting scenarios.
However, it’s important to note that this method can be slow for large files, as it reads the file one line at a time. It also doesn’t handle unusual line endings or special characters well. In the next sections, we’ll discuss more advanced usage scenarios and alternative methods to handle these potential pitfalls.
Advanced File Reading in Bash
As you become more familiar with Bash, you’ll find that there are many ways to enhance the line by line reading process. One such method involves processing each line while reading it, allowing for more complex operations.
Let’s consider a situation where we want to number each line in a file. Here’s how we can accomplish this:
line_number=1
while IFS= read -r line
do
echo "Line $line_number: $line"
((line_number++))
done < numbered.txt
# Output:
# Line 1: [First line of numbered.txt]
# Line 2: [Second line of numbered.txt]
# ...
In this example, we’ve introduced a line_number
variable that increases with each line read. This allows us to print out the line number along with the content of each line. It’s a simple addition but demonstrates how we can start processing data as we read it.
This method still reads the file line by line, but now we’re adding more functionality to our while loop. It’s a step up from the basic use, but it also introduces more complexity.
Remember, as with the basic use, this method can be slow with large files and might not handle unusual line endings or special characters well. As you advance in your Bash scripting journey, you’ll learn more about these potential issues and how to handle them, which we’ll cover in the ‘Troubleshooting and Considerations’ section.
Exploring Alternative Methods in Bash
As you delve deeper into Bash scripting, you’ll discover alternative commands for reading a file line by line. Two such commands are readarray
and mapfile
. These commands can read a file into an array, which can then be processed in various ways.
Utilizing readarray
The readarray
command, also known as mapfile
, reads lines from the standard input into an array variable.
Here’s an example of how to use readarray
to read a file line by line:
readarray -t lines < readarray.txt
for line in "${lines[@]}"; do
echo "$line"
done
# Output:
# [Each line from readarray.txt will be printed here]
In this code block, readarray -t lines < readarray.txt
reads the file ‘readarray.txt’ into the array lines
. The -t
option removes the trailing newline from each line read. We then loop through the lines
array and print each line.
Leveraging mapfile
The mapfile
command is identical to readarray
. It’s simply an alternative name for the same command. Here’s the previous example using mapfile
instead:
mapfile -t lines < mapfile.txt
for line in "${lines[@]}"; do
echo "$line"
done
# Output:
# [Each line from mapfile.txt will be printed here]
The readarray
and mapfile
commands provide a more efficient way to read large files compared to a while loop, as they read the file in chunks rather than line by line. However, they store the entire file in memory, which can be a problem with very large files. They also don’t handle unusual line endings or special characters any better than a while loop.
As with any tool, it’s essential to understand these commands’ strengths and limitations and choose the right tool for the job.
Addressing Common Issues in Bash File Reading
While reading a file line by line in Bash is a straightforward process, you may encounter some common issues. These issues often involve handling empty lines or special characters. Let’s discuss these problems and provide solutions and workarounds.
Handling Empty Lines
When reading a file, you might come across empty lines. By default, the read
command in Bash skips these lines. If you want to preserve these empty lines, you can use the -t
option with readarray
or mapfile
.
Here’s an example:
mapfile -t lines < emptylines.txt
for line in "${lines[@]}"; do
echo "$line"
done
# Output:
# [Each line from emptylines.txt will be printed here, including empty lines]
In this code block, the -t
option removes the trailing newline from each line read, preserving the empty lines. We then loop through the lines
array and print each line, including the empty ones.
Dealing with Special Characters
Another common issue involves handling special characters, such as backslashes. By default, the read
command interprets backslashes. To prevent this, you can use the -r
option.
Here’s an example:
while IFS= read -r line
do
echo "$line"
done < specialchars.txt
# Output:
# [Each line from specialchars.txt will be printed here, with backslashes preserved]
In this code block, the -r
option prevents backslash escapes from being interpreted. Each line, including those with backslashes, is read into the variable line
and then printed out.
Remember, while these solutions and workarounds can handle common issues, they might not cover all scenarios. Always test your scripts thoroughly and understand the potential pitfalls when reading files in Bash.
Understanding File Handling in Bash
To fully grasp how Bash reads a file line by line, it’s crucial to understand the basics of file handling in Bash and its underlying concepts.
In Unix-like systems, like Linux, everything is treated as a file. This includes not only text files and executables but also directories and hardware devices. Bash, being a command-line shell for Unix-like systems, naturally has robust file handling capabilities.
Bash and File Descriptors
In Bash, files are managed through file descriptors. File descriptors are non-negative integers that act as handles for the files. When a file is opened, it’s associated with a file descriptor.
For instance, when Bash starts, it opens three file descriptors:
# File Descriptors in Bash
0 - Standard Input (stdin)
1 - Standard Output (stdout)
2 - Standard Error (stderr)
These file descriptors represent the standard input, output, and error streams, respectively.
Line by Line Reading: Why It’s Important
Reading a file line by line is a common operation in shell scripting. It’s used in various tasks like log file analysis, configuration file parsing, and data processing. Reading line by line allows Bash to process each line individually, enabling complex operations on each line.
Here’s a simple example of a script that reads a log file and prints only the error messages:
while IFS= read -r line
do
if [[ $line == *"ERROR"* ]]; then
echo "$line"
fi
done < logfile.txt
# Output:
# [Error lines from logfile.txt will be printed here]
In this code block, the script reads a file named ‘logfile.txt’ line by line. It checks each line for the string ‘ERROR’ and prints the line if it’s present. This is a basic example, but it illustrates the power of line by line reading in Bash.
Understanding these fundamental concepts is key to mastering file handling in Bash. As you continue your journey, you’ll uncover more advanced topics and techniques, enhancing your Bash scripting skills.
Beyond Basics: File Reading in Larger Bash Projects
Mastering the art of reading a file line by line in Bash is more than just a neat trick. It’s an essential skill that has significant implications in larger scripts or projects. Whether you’re creating a complex script to automate system tasks, or processing large amounts of data, Bash’s file handling capabilities are invaluable.
The Bigger Picture: File Manipulation and Text Processing
As you progress in your Bash scripting journey, you’ll encounter related topics such as file manipulation and text processing. These topics build upon the fundamentals of file reading, expanding your capabilities.
For instance, you might need to modify a file while reading it, like replacing certain text or deleting specific lines. Or you might need to process the text in a file, like extracting data or formatting the text.
Here’s an example of a script that reads a file and replaces certain text:
while IFS= read -r line
do
echo "${line//old/new}"
done < replace.txt
# Output:
# [Each line from replace.txt will be printed here, with 'old' replaced by 'new']
In this code block, the script reads a file named ‘replace.txt’ line by line. It replaces the text ‘old’ with ‘new’ in each line and prints the result. This is a basic example of file manipulation in Bash.
Further Resources for Bash Scripting Mastery
To deepen your understanding of Bash scripting and related topics, consider exploring the following resources:
- GNU Bash Manual: The official manual for Bash, providing comprehensive documentation.
- Bash Academy: An interactive platform to learn Bash scripting from scratch.
- Advanced Bash-Scripting Guide: A detailed guide covering advanced topics in Bash scripting.
These resources offer in-depth information on Bash scripting, from basic to advanced topics, helping you enhance your skills and knowledge.
In this comprehensive guide, we’ve delved into the world of Bash, focusing on reading a file line by line. We’ve covered everything from the basic usage of a while loop to more complex methods involving readarray
and mapfile
commands.
We began with the basics, learning how to use a while loop to read a file line by line in Bash. We then explored more advanced usage scenarios, such as numbering each line or processing each line while reading it. We also introduced alternative commands like readarray
and mapfile
, which offer more efficient ways to read large files.
Along the way, we addressed common issues that you might encounter when reading a file in Bash, such as handling empty lines or special characters, providing solutions and workarounds for each challenge.
Here’s a quick comparison of the methods we’ve discussed:
Method | Speed | Memory Efficiency | Complexity |
---|---|---|---|
While Loop | Slow | High | Low |
Readarray/Mapfile | Fast | Low | Moderate |
Whether you’re just starting out with Bash or you’re looking to enhance your scripting skills, we hope this guide has given you a deeper understanding of how to read a file line by line in Bash.
Reading a file line by line is a fundamental operation in Bash scripting, with applications in various tasks like log file analysis, configuration file parsing, and data processing. With the knowledge you’ve gained from this guide, you’re well-equipped to tackle these tasks. Happy scripting!