Python Dataclass | Funadmentals, Usage, and Examples
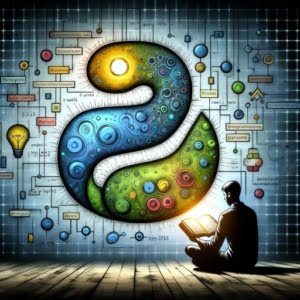
As a type of class specifically designed for storing data, Python dataclasses are a hidden gem in Python’s toolbox that can make your life as a programmer much easier.
This comprehensive guide aims to equip you with the knowledge and skills to effectively use Python dataclasses in your projects.
By the end of this post, you’ll gain a solid understanding of Python dataclasses, their purpose, benefits, and how to use them to write cleaner, more efficient code. So, let’s dive in and uncover the power of Python dataclasses!
TL;DR: What are Python dataclasses?
Python dataclasses are a type of class used for storing data. They automatically generate special methods like
__init__()
and__repr__()
that make managing and manipulating data easier. They are part of Python’s standard library since Python 3.7. For more advanced methods, background, tips and tricks, continue reading the article.
Example:
from dataclasses import dataclass
@dataclass
class Example:
field1: int
field2: str
example = Example(1, 'example')
print(example)
# Output:
# Example(field1=1, field2='example')
Table of Contents
Understanding Python Dataclasses
A Python dataclass, in essence, is a class specifically designed for storing data. They are part of the dataclasses
module in Python 3.7 and above. The main principle behind a dataclass is to minimize the amount of boilerplate code required to create classes. This is achieved with the help of a decorator called @dataclass
.
The @dataclass
decorator automatically adds special methods to your classes, such as __init__()
and __repr__()
, which are usually manually defined in traditional Python classes. These methods are used to initialize objects and represent them as strings for debugging purposes, respectively.
Why would you want to use dataclasses over traditional classes? The primary advantage is that dataclasses reduce the amount of code you have to write, making your code more readable and easier to understand.
Let’s take a look at a simple example of a Python dataclass:
from dataclasses import dataclass
@dataclass
class Book:
title: str
author: str
pages: int
price: float
book1 = Book("Python Basics", "John Doe", 200, 39.99)
print(book1)
# Output:
# Book(title='Python Basics', author='John Doe', pages=200, price=39.99)
In this example, Book
is a Python dataclass with four fields: title
, author
, pages
, and price
. The @dataclass
decorator automatically generates an __init__()
method to initialize these fields and a __repr__()
method to represent the Book
object as a string.
Therefore, when we create a Book
object and print it, Python automatically calls the __repr__()
method to display the object.
The @dataclass
decorator is a powerful tool that can make your code cleaner and more efficient. By reducing the amount of boilerplate code you have to write, dataclasses allow you to focus on the logic of your program rather than the implementation details of your classes. This can result in code that is easier to read, write, and maintain, making dataclasses a valuable tool for any Python developer.
Advanced Usage of Python Dataclasses
Python dataclasses are not just about reducing boilerplate code. They come with a plethora of advanced features that can make your programming life even easier. In this section, we’ll delve deeper into these features, such as default values and type hints, and see them in action.
Default Values
One of the advanced features of Python dataclasses is the ability to provide default values for the fields. This can be done using the familiar syntax used in function arguments. Here’s how you can do it:
from dataclasses import dataclass
@dataclass
class Book:
title: str = 'Unknown Title'
author: str = 'Unknown Author'
pages: int = 0
price: float = 0.0
book1 = Book()
print(book1)
# Output:
# Book(title='Unknown Title', author='Unknown Author', pages=0, price=0.0)
In this example, if we create a Book
object without providing any arguments, Python will use the default values specified in the dataclass.
Example of creating a Book
object with some arguments:
book2 = Book('Python Advanced', 'Jane Doe')
print(book2)
# Output:
# Book(title='Python Advanced', author='Jane Doe', pages=0, price=0.0)
In this example, Python uses the provided arguments for title
and author
, and the default values for pages
and price
.
Type Hints
Python dataclasses also support type hints. Type hints are a way of indicating the expected type of a variable or a function return. This can make your code more readable and self-documenting.
In the previous examples, we have already seen type hints in action. They are the str
, int
, and float
keywords that follow the colon after the field names.
Comparing Dataclasses with Other Python Structures
Understanding how Python dataclasses compare with other Python structures is key to knowing when to use them. Let’s take a closer look at how they stack up against traditional classes, tuples, and dictionaries.
Dataclasses vs Traditional Classes
In traditional classes, you have to manually define special methods like __init__()
and __repr__()
. With dataclasses, these methods are automatically generated, saving you the trouble of writing them yourself.
This makes your code cleaner and more efficient. However, traditional classes offer more flexibility as you can customize these methods to suit your needs.
Here is an example code block comparing a traditional class with a data class:
Traditional class:
class TraditionalBook:
def __init__(self, title, author, pages, price):
self.title = title
self.author = author
self.pages = pages
self.price = price
# Define the __repr__ method here
def __repr__(self):
return f"TraditionalBook(title={self.title}, author={self.author}, pages={self.pages}, price={self.price})"
traditional_book = TraditionalBook("Python Basics", "John Doe", 200, 39.99)
print(traditional_book)
# Output: TraditionalBook(title=Python Basics, author=John Doe, pages=200, price=39.99)
Dataclass:
from dataclasses import dataclass
@dataclass
class DataClassBook:
title: str
author: str
pages: int
price: float
data_class_book = DataClassBook("Python Basics", "John Doe", 200, 39.99)
print(data_class_book)
# Output: DataClassBook(title=Python Basics, author=John Doe, pages=200, price=39.99)
As you can see, the data class version is much shorter and easier to read. The __init__()
and __repr__()
methods are automatically implemented for you.
Dataclasses vs Tuples
Both dataclasses and tuples are used to group related data. However, tuples are immutable and their elements are accessed using indices, which can be less readable when dealing with complex data.
On the other hand, dataclasses are mutable and their fields can be accessed by name, making your code more self-explanatory.
Here are some examples showcasing tuples vs dataclasses:
Tuples:
# Define a book as a tuple
book_tuple = ("Python Basics", "John Doe", 200, 39.99)
print(book_tuple)
# Output: ('Python Basics', 'John Doe', 200, 39.99)
# Access elements by index
print(book_tuple[0]) # Output: 'Python Basics'
print(book_tuple[1]) # Output: 'John Doe'
Here, unless you know by heart what each index means, it’s hard to understand what the data represents just by reading the code.
Dataclasses:
from dataclasses import dataclass
@dataclass
class Book:
title: str
author: str
pages: int
price: float
# Define a book as a Data Class
book_dataclass = Book("Python Basics", "John Doe", 200, 39.99)
print(book_dataclass)
# Output: Book(title='Python Basics', author='John Doe', pages=200, price=39.99)
# Access elements by name
print(book_dataclass.title) # Output: 'Python Basics'
print(book_dataclass.author) # Output: 'John Doe'
With dataclasses, the code has immediately become more readable because we can access the fields by their names and we know exactly what each field represents.
Dataclasses vs Dictionaries
Dictionaries, like dataclasses, allow access to their elements by name. But dictionaries are more flexible as they can hold any number of items and of any type. Dataclasses, however, have a fixed number of fields and each field has a specific type. This makes dataclasses more suitable when you have a fixed schema to follow.
Here are some examples showing the difference between dictionaries and dataclasses in Python:
Dictionaries:
# Define a book as a dictionary
book_dict = {"title": "Python Basics", "author": "John Doe", "pages": 200, "price": 39.99}
print(book_dict)
# Output: {'title': 'Python Basics', 'author': 'John Doe', 'pages': 200, 'price': 39.99}
# Add a new field
book_dict["ISBN"] = "123-456-789"
print(book_dict)
# Output: {'title': 'Python Basics', 'author': 'John Doe', 'pages': 200, 'price': 39.99, 'ISBN': '123-456-789'}
# Access elements by key
print(book_dict["title"]) # Output: 'Python Basics'
print(book_dict["author"]) # Output: 'John Doe'
Here, dictionaries can be easily modified (add or remove items) and do not require a fixed schema.
Dataclasses:
from dataclasses import dataclass
@dataclass
class Book:
title: str
author: str
pages: int
price: float
# Define a book as a Data Class
book_dataclass = Book("Python Basics", "John Doe", 200, 39.99)
print(book_dataclass)
# Output: Book(title='Python Basics', author='John Doe', pages=200, price=39.99)
# Trying to add a new field will raise an AttributeError
book_dataclass.ISBN = "123-456-789" # Raises AttributeError: 'Book' object has no attribute 'ISBN'
# Access elements by name
print(book_dataclass.title) # Output: 'Python Basics'
print(book_dataclass.author) # Output: 'John Doe'
In this case, the structure is fixed by the dataclass definition. It is not as flexible as a dictionary, but it provides a clear definition of the data structure we are using, which can be beneficial in many cases.
Summary of Dataclasses vs Other Structures
From the above examples, you can see that Dataclasses serve an important role in Python programming. However, there are certainly situations where other data structures are more appropriate.
Here is a table summarizing the key differences:
Dataclasses | Traditional Classes | Tuples | Dictionaries | |
---|---|---|---|---|
Automatically generates special methods | Yes | No | N/A | N/A |
Mutable | Yes | Yes | No | Yes |
Elements accessed by name | Yes | Yes | No | Yes |
Can hold any number of items of any type | No | Yes | Yes | Yes |
Suitable when you have a fixed schema | Yes | Yes | No | No |
If you need immutability and order, tuples are the way to go. If you need a flexible container that can hold any number of items of any type, dictionaries are your best bet. But if you’re dealing with complex data and want to make your code cleaner and more efficient, dataclasses are a perfect choice.
Immutability and Dataclasses
Immutability is a property of an object whose state cannot be modified after it is created. In Python, tuples are an example of an immutable data structure. Dataclasses, by default, are mutable.
You can make Dataclasses immutable by setting the
frozen
parameter of the@dataclass
decorator toTrue
. This can be useful when you want to ensure that an object remains constant throughout its lifetime.
Example of making a dataclass immutable:
from dataclasses import dataclass
@dataclass(frozen=True)
class ImmutableBook:
title: str
author: str
pages: int
price: float
try:
book = ImmutableBook('Python Basics', 'John Doe', 200, 39.99)
book.title = 'Python Advanced' # This will raise an error
except Exception as e:
print(f"An error occurred: {e}")
In this example, trying to modify the
title
of theImmutableBook
object will raise anAttributeError
because the dataclass is immutable.
Python and Object-Oriented Programming
Python is an object-oriented programming (OOP) language, which means it uses objects and classes as its fundamental building blocks. In Python, everything is an object, and we can create our own objects using classes. OOP in Python provides a clear, intuitive way to structure code, making it more readable and maintainable.
Python’s approach to OOP is flexible and powerful. It supports multiple inheritance, where a class can inherit from multiple parent classes, and polymorphism, where a subclass can modify the behavior of a parent class. It also supports encapsulation, where data and methods can be bundled together into a single unit, or object.
Python dataclasses fit neatly into Python’s approach to OOP. A dataclass is essentially a class that’s been optimized for storing data. It automatically generates special methods that are commonly used in classes, such as __init__()
and __repr__()
. This saves you the trouble of writing these methods yourself and makes your classes more efficient and easier to work with.
By using dataclasses, you can take full advantage of Python’s OOP capabilities while keeping your code clean and efficient. Dataclasses are a perfect example of how Python’s flexible and powerful OOP features can be leveraged to make your life as a programmer easier.
Further Reading
For those who want to delve deeper into Python programming and its object-oriented features, there are many resources available online.
For example you can, Click Here for insights on the world of sequences in Python and learn how to manipulate them efficiently.
Additionaly, here are a few articles that provide a more in-depth look at Python and OOP in general:
- Exploring deque in Python – Master Python deque operations for building complex data processing pipelines.
Understanding Python’s Array Module – Explore Python array examples and use cases for various programming tasks.
Guide on Object-Oriented Programming – Comprehensive guide on Python’s object-oriented programming.
Python’s Official Data Model Documentation – Detailed insight into Python’s data model from the official docs.
Python Classes and OOP – Understand Python’s classes and object-oriented programming with Python Guru.
If you’re interested in learning more about Python dataclasses, there are many resources available online. Here are a few recommended ones:
- Official Python Documentation on Dataclasses – Explore Python’s dataclasses from the official Python documentation.
Real Python’s Guide on Python 3.7’s Dataclasses dives into Dataclasses in Python 3.7.
Corey Schafer’s Video Tutorial on Python Dataclasses helps you understand Python dataclasses.
Other Python Libraries, Functions, and Tools
While Python dataclasses are a powerful tool in their own right, there are other Python libraries and tools that can complement them and enhance your Python programming experience. Let’s take a look at a few of them:
attrs
attrs
is a Python library that, like dataclasses, simplifies writing classes. It offers more features than dataclasses and works with older versions of Python. However, it’s a third-party library and not part of Python’s standard library, unlike dataclasses. You can learn more about attrs
here.
typing
The typing
module in Python is used for type hints, a feature that we’ve seen in use with dataclasses. Type hints can make your code more readable and help you catch certain types of errors earlier. You can learn more about Python’s typing
module here.
pydantic
pydantic
is a data validation library that uses Python type annotations. It’s useful for parsing complex data, converting from one format to another, and for validation. It can work together with dataclasses to provide data validation. You can learn more about pydantic
here.
Wrapping Up:
Python dataclasses are a powerful tool that can significantly streamline your code, making it more efficient and easier to read. They serve as a type of class specifically designed for storing data, equipped with special methods like __init__()
and __repr__()
that are automatically generated. This frees you from the need to write these methods yourself, saving you time and reducing the chance of errors.
When compared with traditional classes, tuples, and dictionaries, Python dataclasses stand out for their efficiency and readability. They offer the flexibility of traditional classes, the order of tuples, and the named access of dictionaries, all while reducing boilerplate code. However, the choice of data structure always depends on the specific needs of your project.
In the broader context of Python programming, dataclasses fit neatly into Python’s approach to object-oriented programming. They leverage Python’s OOP capabilities to make your code cleaner and more efficient, demonstrating how Python’s flexible and powerful OOP features can simplify your life as a programmer.
Mastering Python dataclasses can be a valuable addition to your Python programming skillset. So, start using them in your projects and experience the difference they make. Remember, practice is key when mastering any programming concept. Happy coding!