Python String replace() Function (and Alternatives)
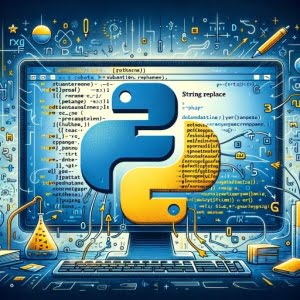
Have you ever grappled with the task of replacing substrings in Python? If so, you’re not alone. But fear not, Python’s replace()
method is here to make your life easier. Think of it as a skilled surgeon, capable of precisely replacing any substring within a string.
Whether you’re a beginner just starting out or an intermediate Pythonista looking to refine your skills, this comprehensive guide will walk you through the ins and outs of Python’s string replacement process.
So, let’s dive into the world of Python string replace and enhance your coding skills together.
TL;DR: How Do I Replace a Substring in Python?
The answer is simple: you use Python’s
replace()
method. Here’s a quick example to illustrate:
text = 'Hello, World!'
new_text = text.replace('World', 'Python')
print(new_text)
# Output:
# 'Hello, Python!'
In this example, we’ve taken the string ‘Hello, World!’, and used the replace()
method to replace the substring ‘World’ with ‘Python’. The result is a new string: ‘Hello, Python!’.
Intrigued? Keep reading for a more detailed exploration of Python’s string replace capabilities, from basic usage to advanced techniques.
Table of Contents
- Python’s replace() Method: A Beginner’s Guide
- Advanced String Replacement Techniques
- Exploring Alternative Substring Replacement Methods
- Troubleshooting Common String Replacement Issues
- Understanding Python Strings and Their Methods
- The Power of Python String Replacement
- Recap: Harnessing Python’s String Replace Method
Python’s replace()
Method: A Beginner’s Guide
Python’s replace()
is a built-in string method that allows you to replace all occurrences of a specified substring with another substring. This method follows the syntax: str.replace(old, new[, count])
, where ‘old’ is the substring you want to replace, ‘new’ is the replacement substring, and ‘count’ is an optional argument specifying the maximum number of replacements to be made.
Let’s illustrate this with a simple example:
# Original string
text = 'I love apples. apples are my favorite fruit.'
# Using replace() to change 'apples' to 'oranges'
new_text = text.replace('apples', 'oranges')
print(new_text)
# Output: 'I love oranges. oranges are my favorite fruit.'
In this example, we’ve replaced all instances of the substring ‘apples’ with ‘oranges’ in the string. The replace()
method is case-sensitive, meaning ‘Apples’ and ‘apples’ are treated as different substrings.
While the replace()
method is incredibly useful, it’s essential to be aware of its potential pitfalls. For instance, it will replace all instances of the specified substring, not just the first one. If you only want to replace the first occurrence, you would need to use the ‘count’ argument, like so:
# Replace only the first occurrence of 'apples'
new_text = text.replace('apples', 'oranges', 1)
print(new_text)
# Output: 'I love oranges. apples are my favorite fruit.'
In this case, only the first instance of ‘apples’ is replaced with ‘oranges’. Understanding these nuances will help you leverage Python’s replace()
method more effectively in your coding journey.
Advanced String Replacement Techniques
As you progress in your Python journey, you’ll encounter scenarios where a simple replace()
might not suffice. For instance, you might need to replace all occurrences of a substring, or combine the replace()
method with other string methods for more complex manipulations.
Replacing All Occurrences of a Substring
By default, the replace()
method replaces all occurrences of a substring. However, you can control the number of replacements using the optional ‘count’ parameter. If you want to replace all occurrences, you simply skip this parameter.
text = 'apple apple apple'
new_text = text.replace('apple', 'orange')
print(new_text)
# Output: 'orange orange orange'
In this example, all instances of ‘apple’ are replaced with ‘orange’.
Combining replace()
with Other String Methods
Python’s string methods can be combined for more advanced manipulations. For example, you might want to convert a string to lowercase before replacing substrings to ensure case-insensitive replacement.
text = 'Apple apple Apple'
new_text = text.lower().replace('apple', 'orange')
print(new_text)
# Output: 'orange orange orange'
In this case, we first convert the string to lowercase using the lower()
method, then replace ‘apple’ with ‘orange’. This ensures all instances of ‘apple’, regardless of case, are replaced.
These advanced techniques help you handle more complex scenarios, making the replace()
method a powerful tool in your Python toolkit.
Exploring Alternative Substring Replacement Methods
While Python’s replace()
method is an effective tool for replacing substrings, it’s not the only game in town. There are other techniques that you can use to replace substrings in Python, especially when dealing with more complex or specific scenarios. One such technique is using regular expressions.
Using Regular Expressions for Substring Replacement
Regular expressions (or regex) are a powerful tool used for matching and manipulating strings. Python’s re
module provides the sub()
function, which is similar to replace()
, but with more flexibility.
import re
text = 'Apple apple Apple'
new_text = re.sub('apple', 'orange', text, flags=re.I)
print(new_text)
# Output: 'orange orange orange'
In this example, we’re using the sub()
function to replace ‘apple’ with ‘orange’. The flags=re.I
argument makes the replacement case-insensitive. This is something that replace()
cannot do directly.
While regular expressions provide more flexibility, they also come with a steeper learning curve and can be overkill for simple substring replacements. Therefore, it’s recommended to stick with replace()
for straightforward cases and only use regular expressions when necessary.
By understanding and using these alternative methods, you can handle a wider range of string replacement scenarios in Python. The key is to choose the right tool for the job.
Troubleshooting Common String Replacement Issues
Python’s string replace()
method is generally straightforward, but there can be occasional hiccups. Let’s discuss some common issues you may encounter during string replacement, particularly when dealing with special characters, and their respective solutions.
Dealing with Special Characters
In Python, certain characters have special meanings, such as '\n'
for a newline or '\t'
for a tab. If you try to replace these using the replace()
method, you might not get the results you expect. Let’s look at an example:
text = 'Hello\nWorld'
new_text = text.replace('\n', ', ')
print(new_text)
# Output: 'Hello
World'
In this case, we wanted to replace the newline character '\n'
with a comma, but the output remains the same. This happens because in Python, '\\'
is an escape character that tells Python to treat the next character as a literal character, not a special one.
To correctly replace the newline character, we need to use a raw string, denoted by ‘r’ before the string, or double backslashes.
text = 'Hello\nWorld'
new_text = text.replace('\\n', ', ')
print(new_text)
# Output: 'Hello, World'
In this corrected example, we’re using double backslashes to indicate that we want to replace the literal '\n'
, not the newline character. This results in the expected output.
Understanding these nuances can help you avoid common pitfalls and effectively use Python’s replace()
method for string replacement.
Understanding Python Strings and Their Methods
Before diving deeper into Python’s replace()
method, it’s important to understand the basics of Python strings and their manipulation methods.
What is a Python String?
In Python, a string is a sequence of characters. It’s one of the basic data types in Python and can be created by enclosing characters in single or double quotes.
# Creating a string
str1 = 'Hello, Python string replace!'
print(str1)
# Output: 'Hello, Python string replace!'
Python String Methods
Python provides a variety of built-in methods for string manipulation. These methods allow you to change case, split a string, strip whitespace, and more. One such method is replace()
, which we’ve been discussing.
# Using a string method to convert a string to uppercase
str2 = str1.upper()
print(str2)
# Output: 'HELLO, PYTHON STRING REPLACE!'
In this example, we use the upper()
method to convert our string to uppercase.
Understanding these fundamentals provides a solid foundation for mastering more advanced concepts like Python’s replace()
method. With this knowledge, you can more effectively manipulate and handle strings in Python, optimizing your code and enhancing your programming skills.
The Power of Python String Replacement
Python string replacement is more than just a coding technique. It’s a powerful tool with wide-reaching applications in various fields, particularly in text processing and data cleaning.
String Replacement in Text Processing
In text processing, string replacement can be used to standardize text data, correct typos, or transform text to a desired format. For instance, you might want to replace all occurrences of ‘USA’ with ‘United States’ in a dataset.
text = 'I live in the USA.'
new_text = text.replace('USA', 'United States')
print(new_text)
# Output: 'I live in the United States.'
In this example, we replace ‘USA’ with ‘United States’, standardizing the country’s name in the text.
String Replacement in Data Cleaning
In data cleaning, string replacement plays a crucial role in preparing data for analysis. It can be used to handle missing values, eliminate unnecessary characters, or convert data to a suitable format.
data = 'Price: $100'
cleaned_data = data.replace('Price: $', '')
print(cleaned_data)
# Output: '100'
Here, we use string replacement to remove the ‘Price: $’ prefix, leaving only the numeric value. This cleaned data is now ready for numerical analysis.
Further Resources for Python Strings
While Python’s replace()
method is powerful, there are other related concepts worth exploring. Regular expressions, for instance, provide more flexibility in matching and replacing substrings. String formatting, on the other hand, allows you to dynamically insert values into a string.
For those interested in deepening their understanding of Python string manipulation, here are a few resources that you might find helpful:
- Unlocking the Potential of Python Strings in Programming: Unlock the full potential of Python strings in your programming projects, learning how to handle textual data like a pro.
Python Documentation: String Methods: In the official Python documentation, you can find a comprehensive list of string methods along with detailed explanations.
Python String Interpolation: Formatting Strings in Python: Learn how to interpolate variables and expressions into strings using different string interpolation techniques in Python, such as f-strings, format(), and %-formatting.
String Slicing in Python: Usage and Examples: This article provides an in-depth explanation of string slicing in Python, including various slicing techniques and examples to extract substrings from strings.
Python String replace() Method: A Comprehensive Guide: A comprehensive guide on w3schools.com that explains the replace() method in Python, used to replace occurrences of a specific value in a string.
Replacing Strings in Python: A Practical Guide: Real Python offers a practical guide on replacing strings in Python, covering different string manipulation techniques including replace().
Python String replace() Method: Tutorialspoint provides detailed information on the replace() method in Python, demonstrating how to replace substrings in a string.
Recap: Harnessing Python’s String Replace Method
Throughout this guide, we’ve explored the ins and outs of Python’s replace()
method, a powerful tool for replacing substrings in a string. We started with the basics, learning how to use replace()
in its simplest form.
We then delved into more advanced usage, understanding how to control the number of replacements and combine replace()
with other string methods for more complex manipulations. We also explored alternative methods like regular expressions for more flexible substring replacement.
We highlighted some common issues you might encounter when using replace()
, like dealing with special characters, and provided solutions for these problems. Finally, we discussed the wider applications of string replacement in fields like text processing and data cleaning, and encouraged further exploration of related concepts.
Python’s replace()
method is a versatile tool that can greatly enhance your text manipulation capabilities in Python. Whether you’re a beginner just starting out or an experienced Pythonista, mastering string replacement can open up new possibilities in your coding journey. Happy coding!