Python to Lowercase: lower(), casefold(), and beyond
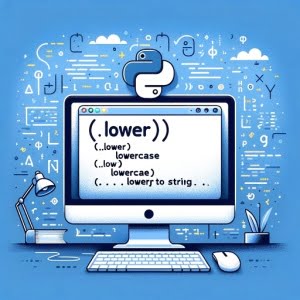
Struggling with converting strings to lowercase in Python? Just like a diligent editor, Python can effortlessly convert any string to lowercase. In this walkthrough, we will dive deep into the process of converting strings to lowercase in Python.
We cover basic usage, handling strings containing special characters, and even dealing with non-English alphabets. We will also explore some alternative approaches and discuss common issues you might encounter along the way.
TL;DR: How Do I Convert a String to Lowercase in Python?
You can use the
lower()
method in Python to convert a string to lowercase. Here’s a simple example:
text = 'Hello, World!'
lower_text = text.lower()
print(lower_text)
# Output:
# 'hello, world!'
In this example, we first declare a string text
with the value ‘Hello, World!’. We then use the lower()
method on the text
string to convert it to lowercase. The result is stored in the lower_text
variable, which we then print out. The output is ‘hello, world!’, which is the lowercase version of our original string.
This is a basic usage of the
lower()
method. Read on for a more detailed explanation and advanced usage scenarios.
Table of Contents
- Lowercase Conversion in Python: The Basics
- Lowercase Conversion with Special Characters
- Dealing with Non-English Alphabets
- Exploring Alternative Lowercase Conversion Methods
- Troubleshooting Python Lowercase Conversion
- Understanding Python’s String Data Type
- The Power of Lowercase Conversion in Python
- Python Lowercase Conversion: A Recap
Lowercase Conversion in Python: The Basics
Python’s lower()
method is a built-in function used to convert a string to lowercase. It’s simple and straightforward to use, making it perfect for beginners. Here’s how it works:
text = 'Python To Lowercase'
lower_text = text.lower()
print(lower_text)
# Output:
# 'python to lowercase'
In this example, we first declare a string text
with the value ‘Python To Lowercase’. We then use the lower()
method on the text
string to convert it to lowercase. The result is stored in the lower_text
variable, which we then print out. The output is ‘python to lowercase’, which is the lowercase version of our original string.
Advantages of lower()
Method
The lower()
method is a straightforward and efficient way to convert strings to lowercase in Python. It’s easy to understand and use, even for beginners. Additionally, since it’s a built-in method, no extra imports are needed.
Potential Pitfalls of lower()
Method
While the lower()
method is highly useful, it’s important to note that it only works with alphabetic characters. Non-alphabetic characters in the string remain unaffected. Moreover, it might not behave as expected with certain non-English alphabets or special characters. We’ll explore these issues in more depth in the ‘Advanced Use’ and ‘Troubleshooting and Considerations’ sections of this guide.
Lowercase Conversion with Special Characters
When dealing with strings that contain special characters or non-English alphabets, the lower()
method behaves slightly differently. Let’s take a look at an example to understand this better:
text = 'Python, To Lowercase! éÉ'
lower_text = text.lower()
print(lower_text)
# Output:
# 'python, to lowercase! éé'
In this example, we’ve included a comma, an exclamation mark, and two accented characters ‘é’ and ‘É’ in our original string. The lower()
method converts the uppercase ‘É’ to its lowercase equivalent ‘é’, while leaving the special characters (comma and exclamation mark) and the already lowercase ‘é’ unchanged.
Dealing with Non-English Alphabets
The lower()
method also works with strings containing characters from non-English alphabets. Here’s an example using Greek characters:
text = 'ΓΕΙΑ'
lower_text = text.lower()
print(lower_text)
# Output:
# 'γεια'
In this example, the lower()
method successfully converts the uppercase Greek word ‘ΓΕΙΑ’ (meaning ‘hello’) to its lowercase equivalent ‘γεια’. This illustrates the versatility of Python’s lower()
method in handling strings from various languages.
Exploring Alternative Lowercase Conversion Methods
While lower()
is a common method for string conversion in Python, there are other methods and third-party libraries that can be used for more specific needs. One such method is casefold()
.
Using casefold()
Method
casefold()
is similar to lower()
, but it is more aggressive. It’s designed to remove all case distinctions in a string. Here’s how it works:
text = 'Python, To Lowercase! ß'
casefold_text = text.casefold()
print(casefold_text)
# Output:
# 'python, to lowercase! ss'
In this example, the casefold()
method not only converts the regular characters to lowercase but also transforms the German letter ‘ß’ (sharp S) into ‘ss’. This is something that lower()
doesn’t do.
Advantages and Disadvantages of casefold()
casefold()
is more effective than lower()
in handling certain special characters and non-English alphabets. However, it might be overkill for simple use-cases and can potentially lead to unexpected results.
Using Third-Party Libraries
For even more advanced string manipulation, you might want to consider third-party Python libraries like unicodedata
. These libraries offer additional methods for string conversion and manipulation, but they might require more understanding of Python and its libraries to use effectively. As always, the best approach depends on your specific needs and the nature of your data.
Troubleshooting Python Lowercase Conversion
While Python’s lower()
and casefold()
methods are handy tools for converting strings to lowercase, they may not always work as expected, especially when dealing with multilingual strings or special characters.
Handling Case Conversion in Multilingual Strings
One common issue arises when converting uppercase characters in multilingual strings to lowercase. For instance, the Greek character ‘Σ’ corresponds to both ‘σ’ and ‘ς’ in lowercase, depending on its position in the word. Python’s lower()
method might not always handle such cases correctly.
text = 'ΠΑΡΑΔΕΙΣΗ'
lower_text = text.lower()
print(lower_text)
# Output:
# 'παραδειση'
In this example, the lower()
method converts the uppercase Greek word ‘ΠΑΡΑΔΕΙΣΗ’ (meaning ‘paradise’) to ‘παραδειση’. However, the correct conversion should be ‘παραδεισι’, with the final ‘Σ’ converted to ‘ς’ instead of ‘σ’.
Solutions and Workarounds
To handle such issues, you might need to implement custom conversion logic using Python’s string manipulation functions or use third-party libraries that provide more advanced case conversion features.
Remember, the best approach depends on the specific needs of your project and the nature of your data. Always test your code thoroughly with representative data to ensure it behaves as expected.
Understanding Python’s String Data Type
To fully comprehend the process of converting strings to lowercase in Python, it’s important to understand the basics of Python’s string data type.
What is a String in Python?
In Python, a string is a sequence of characters. It is an immutable data type, meaning that once a string is created, it cannot be changed. However, you can create a new string based on the original one with the desired modifications.
original = 'Python'
modified = original + ' Programming'
print(modified)
# Output:
# 'Python Programming'
In this example, we first declare a string original
with the value ‘Python’. We then create a new string modified
by appending ‘ Programming’ to original
. The output is ‘Python Programming’. Note that the original string remains unchanged.
How Does Python Handle Case in Strings?
Python distinguishes between uppercase and lowercase characters in strings. This is why we can convert strings to lowercase or uppercase. Internally, Python uses Unicode to represent characters, and each character has a unique Unicode code point. Uppercase and lowercase versions of the same letter have different code points, which is how Python differentiates between them.
text = 'Python'
print(ord('P'), ord('p'))
# Output:
# 80 112
In this example, we use the ord()
function to print the Unicode code points of ‘P’ and ‘p’. As you can see, they are different, which is why Python treats ‘P’ and ‘p’ as different characters.
The Power of Lowercase Conversion in Python
Converting strings to lowercase in Python isn’t just an academic exercise. It has real-world applications in fields like text processing and data cleaning. For instance, when processing user input or text data, converting everything to lowercase can help standardize the data and make it easier to work with.
user_input = 'Python To Lowercase'
standardized_input = user_input.lower()
print(standardized_input)
# Output:
# 'python to lowercase'
In this example, we take a user input string ‘Python To Lowercase’ and convert it to lowercase. This can help ensure consistency when comparing user input to stored data, regardless of how the user types it.
Exploring Related Concepts
Once you’ve mastered converting strings to lowercase in Python, you might want to explore related concepts like string manipulation and regular expressions. These topics can further enhance your text processing skills and open up new possibilities for handling and analyzing data.
Further Learning and Related Topics
Python is a powerful and versatile language with a rich set of features. To deepen your understanding of Python and its capabilities, consider delving into the following guides:
- Python Print Simplified: Quick Reference – Master the Python print() function to format and customize output in your scripts.
Join in Python – Discover Python’s join() method for concatenating elements of iterable objects into strings.
Python Split Function – Learn how to split strings in Python to extract meaningful information from textual data.
Understanding PEP 8: Your Guide to Writing Pythonic Code – A detailed guide on the PEP 8 styling convention.
PEP 8 – Style Guide for Python Code – Explore the official PEP 8 documentation on Python coding styles.
Case Styles in Python – Get a comprehensive look at different naming and case styles used in Python programming.
Python Lowercase Conversion: A Recap
Converting strings to lowercase is a fundamental operation in Python, with practical applications in text processing and data cleaning. This guide has provided a comprehensive overview of how to perform this operation using the lower()
method, as well as alternative methods like casefold()
.
We’ve seen that Python’s lower()
method is a straightforward and efficient tool for converting strings to lowercase. However, it’s important to be aware of potential issues when dealing with special characters or non-English alphabets. For these more complex scenarios, alternatives like casefold()
or third-party libraries might be more suitable.
Mastering string conversion is just one step in your Python journey. There are many more topics to explore, from string manipulation to regular expressions. As you continue to learn and practice, you’ll discover the full power and versatility of Python. Happy coding!