Python ‘None’ Keyword: Comprehensive Guide
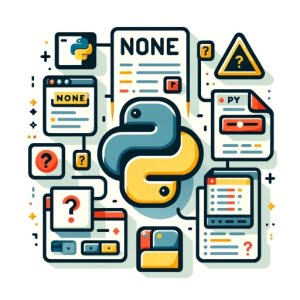
Have you ever stumbled upon the ‘None’ keyword in Python and wondered what it’s all about? You’re not alone. Many Python developers find themselves puzzled when they first encounter ‘None’. Think of ‘None’ as a placeholder in Python – it holds a place in your code when there’s nothing to be held.
‘None’ in Python is a unique creature, unlike anything in some other programming languages. It’s a special type of data, representing the absence of a value or a null value. It’s not zero, it’s not an empty string, it’s just… None.
In this guide, we’ll unravel the mystery of ‘None’ in Python, from its basic usage to more advanced applications. We’ll cover everything from understanding what ‘None’ is, how to use it, and even how to handle it in different scenarios.
So, let’s embark on this journey to master ‘None’ in Python!
TL;DR: What is ‘None’ in Python?
‘None’ in Python represents the absence of a value or a null value. It is an instance of the NoneType data type and is used to signify the absence of a value in a variable, function, etc. Here’s a simple example:
def function():
pass
result = function()
print(result)
# Output:
# None
In this example, we define a function that doesn’t return anything. When we call this function and assign its return value to the variable result
, the value of result
is ‘None’. This is because the function doesn’t return any value, hence ‘None’ is printed.
This is just a basic introduction to ‘None’ in Python. There’s much more to learn about ‘None’, its uses, and how to handle it in different scenarios. Continue reading for a more detailed understanding of ‘None’ and its uses in Python.
Table of Contents
- Unraveling ‘None’ in Python: A Beginner’s Perspective
- ‘None’ in Python: Functions, Variables, and OOP
- Expert-Level Handling of ‘None’ in Python
- Navigating ‘None’ Pitfalls in Python
- Python Data Types: The Unique Role of ‘None’
- The Power of ‘None’: Error Handling, Data Cleaning, and More
- Wrapping Up: Mastering ‘None’ in Python
Unraveling ‘None’ in Python: A Beginner’s Perspective
‘None’ is a unique and special data type in Python. It’s not a number, it’s not a string, it’s not a boolean – it’s simply ‘None’. The ‘None’ keyword is used to define a null value, or no value at all. It is not the same as 0, False, or an empty string. Here’s a simple example:
x = None
print(type(x))
# Output:
# <class 'NoneType'>
In this code block, we assign the value ‘None’ to the variable x
and then print the type of x
. The output shows that the type of x
is NoneType
, which is the data type of ‘None’ in Python.
Why Use ‘None’ in Python?
‘None’ is used for several reasons in Python programming. It can be used to initialize a variable that you don’t want to assign any value yet. For instance, if you’re creating a variable for a later computation but don’t have a value for it at the moment, you can initialize it with ‘None’.
x = None
# Some code here
x = 10
In this code snippet, x
is initially assigned with ‘None’, and later in the code, it gets a real value (10).
Another common use of ‘None’ is to denote the end of lists in Python or to mark default parameters of a function. But beware, using ‘None’ inappropriately can also lead to errors. For instance, trying to perform an operation on ‘None’ as if it were an integer or a string would result in a TypeError. We’ll discuss more about this in the ‘Troubleshooting and Considerations’ section.
In the next section, we’ll dive deeper into the advanced uses of ‘None’ in Python.
‘None’ in Python: Functions, Variables, and OOP
As we delve deeper into the world of Python, ‘None’ continues to play an essential role. It’s not just a placeholder for variables, but it also finds its place in functions, object-oriented programming (OOP), and more.
‘None’ in Functions
In Python functions, ‘None’ plays a crucial role. When a function doesn’t explicitly return a value, it returns ‘None’. Let’s look at an example:
def greet():
print('Hello, World!')
result = greet()
print(result)
# Output:
# Hello, World!
# None
In this example, the greet
function doesn’t return any value. It just prints ‘Hello, World!’. When we call this function and assign its return value to the result
variable, result
gets the value ‘None’. That’s because the greet
function doesn’t return any value, and hence Python returns ‘None’.
‘None’ in Object-Oriented Programming
In object-oriented programming (OOP), ‘None’ is used to represent the absence of a value for instance variables or to denote that a method doesn’t return anything. It can also be used as a default value for function arguments. Let’s consider an example:
class MyClass:
def __init__(self, var = None):
self.var = var
obj = MyClass()
print(obj.var)
# Output:
# None
In this example, the __init__
method of MyClass
has a parameter var
with a default value of ‘None’. When we create an object obj
of MyClass
without passing any argument, the instance variable obj.var
gets the value ‘None’.
In the next section, we’ll explore alternative approaches to handling ‘None’ in Python.
Expert-Level Handling of ‘None’ in Python
As you advance in Python, you’ll encounter situations where you need to handle ‘None’ more efficiently or in a different way. Let’s explore some of these alternative approaches.
‘is’ vs '=='
for Comparing with ‘None’
When comparing a variable with ‘None’, you might be tempted to use the '=='
operator. However, Python recommends using the ‘is’ operator. Here’s why:
x = None
print(x == None) # True
print(x is None) # True
y = 'None'
print(y == None) # False
print(y is None) # False
In this example, both '=='
and ‘is’ give the same result when comparing x
with ‘None’. However, when comparing y
(which is a string ‘None’) with ‘None’, both '=='
and ‘is’ return False. This is because '=='
compares the values, while ‘is’ checks if both the variables point to the same object.
Dealing with ‘None’ in Data Structures
When dealing with data structures like lists or dictionaries, ‘None’ can be used to represent the absence of a value. However, it’s important to handle ‘None’ correctly to avoid errors. For instance, trying to perform operations on ‘None’ as if it were a number or a string would result in a TypeError.
my_list = [1, None, 2]
print(my_list[1] + 1) # Raises TypeError: unsupported operand type(s) for +: 'NoneType' and 'int'
In this example, trying to add 1 to the ‘None’ value in the list raises a TypeError. To avoid this, you can check if a value is ‘None’ before performing operations on it.
if my_list[1] is not None:
print(my_list[1] + 1)
else:
print('None value encountered')
# Output:
# None value encountered
In this revised code, we first check if the value is ‘None’ before trying to add 1 to it. This way, we can avoid the TypeError.
In the next section, we’ll discuss troubleshooting and considerations when working with ‘None’ in Python.
While ‘None’ is a powerful tool in Python, it can also lead to a few common issues if not handled properly. Let’s explore these potential pitfalls and how to avoid them.
TypeError: NoneType object is not callable
One of the common errors you might encounter is a TypeError stating that ‘NoneType’ object is not callable. This error usually occurs when you try to use ‘None’ as a function. Here’s an example:
none_var = None
none_var()
# Output:
# TypeError: 'NoneType' object is not callable
In this code, we’re trying to use ‘None’ as if it were a function, which leads to a TypeError. To avoid this error, always ensure that a variable is callable before trying to call it.
TypeError: unsupported operand type(s) for +: ‘NoneType’ and ‘int’
Another common error is a TypeError that occurs when you try to perform an operation on ‘None’ as if it were an integer or a string. For instance:
x = None
print(x + 1)
# Output:
# TypeError: unsupported operand type(s) for +: 'NoneType' and 'int'
In this example, we’re trying to add 1 to ‘None’, which leads to a TypeError. To avoid this error, always check if a variable is ‘None’ before performing operations on it.
Key Takeaways
While ‘None’ can be a useful tool in Python, it’s important to use it appropriately to avoid errors. Always ensure that a variable isn’t ‘None’ before trying to use it as a function, or before performing operations on it as if it were an integer or a string. With these precautions, you can use ‘None’ effectively and avoid common pitfalls.
Python Data Types: The Unique Role of ‘None’
In Python, data types are the classification or categorization of data items. Python has various standard data types that define the operations possible on them and the storage method for each of them. These include numeric, sequence, set, and mapping types. Among these, ‘None’ holds a unique position.
‘None’: The Null in Python
In many programming languages, there’s a concept of a ‘null’ value. ‘Null’ typically signifies the absence of a value or that a data point doesn’t exist. In Python, ‘None’ serves this purpose. It’s a special constant in Python that represents the absence of a value or a null value. It’s an object of its own datatype, the NoneType.
x = None
print(type(x))
# Output:
# <class 'NoneType'>
In this code example, we assign ‘None’ to the variable x
and then print the type of x
. The output shows that the type of x
is NoneType
, which is the data type of ‘None’ in Python.
‘None’ as a Unique Python Entity
‘None’ is not the same as False, it’s not 0, and it’s certainly not some random empty string. It’s its own type — a sole singleton object that’s unique in its behavior. It’s used when you want to signify that a variable exists, but it doesn’t have any known value yet.
In the next section, we will discuss the importance of ‘None’ in error handling, data cleaning, and more.
The Power of ‘None’: Error Handling, Data Cleaning, and More
The use of ‘None’ in Python extends beyond just a placeholder for an absent value or a null value. It plays a significant role in various areas such as error handling, data cleaning, and more.
‘None’ in Error Handling
In error handling, ‘None’ is often used to represent the absence of an error. For instance, you might have a function that returns an error object if an error occurs, and ‘None’ if no errors occur.
def divide(a, b):
if b == 0:
return 'Error: Division by zero'
else:
return a / b
result = divide(10, 0)
if result is not None:
print(result)
else:
print('Operation successful')
# Output:
# Error: Division by zero
In this example, the divide
function returns an error message if the divisor is zero. If the division is successful, it returns the result of the division. After calling the function, we check if the result is ‘None’. If it’s not ‘None’, we print the result (which would be an error message in case of an error). If the result is ‘None’, we print ‘Operation successful’.
‘None’ in Data Cleaning
In data cleaning, ‘None’ is often used to represent missing data. For instance, you might have a list of data where ‘None’ represents missing values. By identifying ‘None’ values, you can handle missing data appropriately.
data = [1, None, 2, None, 3]
clean_data = [x for x in data if x is not None]
print(clean_data)
# Output:
# [1, 2, 3]
In this code, we have a list data
with some ‘None’ values. We create a new list clean_data
where we include only the values that are not ‘None’. This way, we can clean our data by removing the ‘None’ values.
Further Resources for Mastering ‘None’ in Python
To help you continue your journey in understanding ‘None’ in Python, here are some additional resources:
- IOFlood’s Python Keywords Article – Gain insights into Python’s foundational keywords for coding.
Understanding the “in” Keyword in Python – Learn how to use the “in” keyword in Python to check for membership in collections.
Python Null: Demystifying the None Keyword – Delve into the concept of null-like values in Python and how they differ from “None.”
Python Key Words Reference – A handy reference for Python’s reserved keywords from W3Schools.
Official Python Documentation on ‘None’ – In-depth view on ‘None’ keyword directly from Python’s official documentation.
Guide on ‘None’ in Python – Real Python provides a comprehensive understanding of Python’s ‘None’.
Wrapping Up: Mastering ‘None’ in Python
In this comprehensive guide, we’ve journeyed through the world of ‘None’ in Python, understanding its significance, usage, and the common issues that arise when dealing with it.
We began with the basics, understanding what ‘None’ represents in Python and how it’s used in beginner-level Python coding. We then ventured into more advanced territory, exploring ‘None’ usage in functions, variables, and object-oriented programming.
We also discussed alternative approaches to handling ‘None’, such as using ‘is’ vs '=='
for comparison and dealing with ‘None’ in data structures.
Along the way, we tackled common challenges you might face when using ‘None’, such as TypeError when trying to perform operations on ‘None’ as if it were an integer or a string. We provided solutions and workarounds for each issue, ensuring you’re well-equipped to handle any ‘None’-related problems that come your way.
Approach | Pros | Cons |
---|---|---|
Using ‘None’ | Signifies absence of value, useful in functions and OOP | Can lead to TypeError if not handled properly |
Using ‘is’ vs '==' | Correct way to compare with ‘None’ | May not be intuitive for beginners |
Handling ‘None’ in data structures | Useful to represent absence of data | Requires careful handling to avoid errors |
Whether you’re just starting out with Python or you’re an experienced Pythonista looking to deepen your understanding of ‘None’, we hope this guide has given you a comprehensive understanding of ‘None’ and its uses.
‘None’ in Python is a unique and powerful tool, and understanding how to use it effectively is an important part of mastering Python. Happy coding!